第一部分:技术要求
Java知识。 GUI图形界面。 JDBC知识。 MySQL知识。
第二部分:软件结构
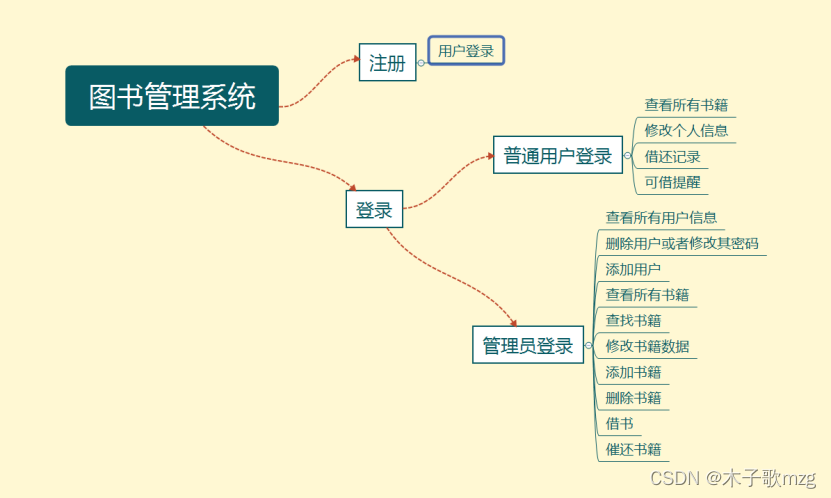
第三部分:部分页面展示
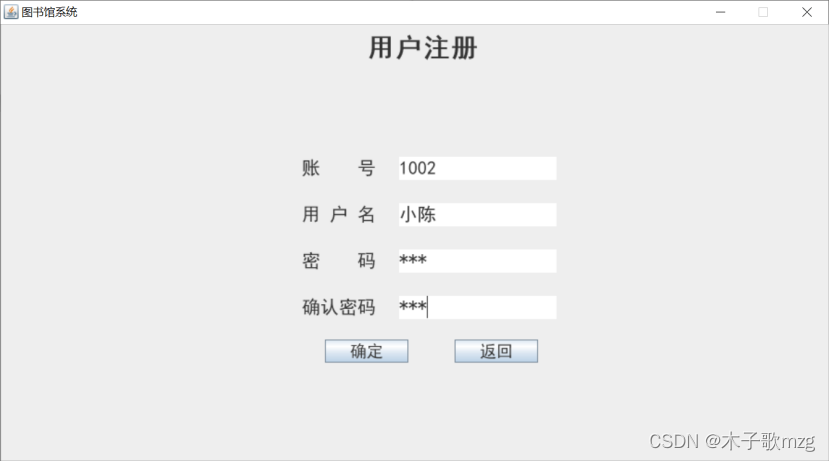 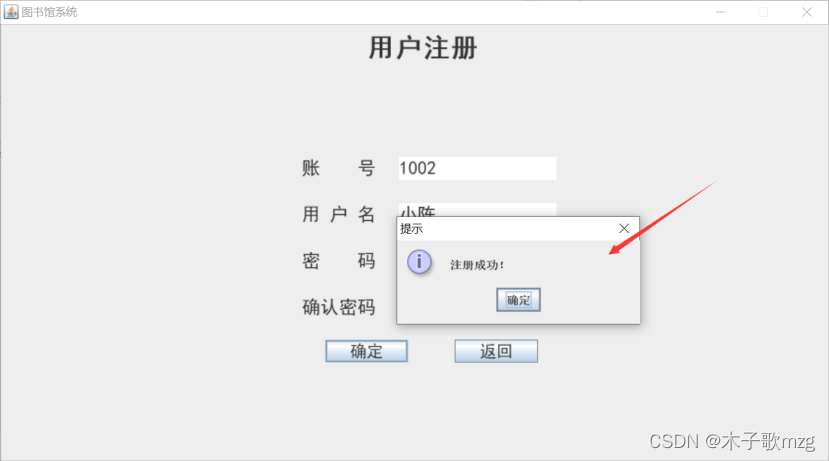
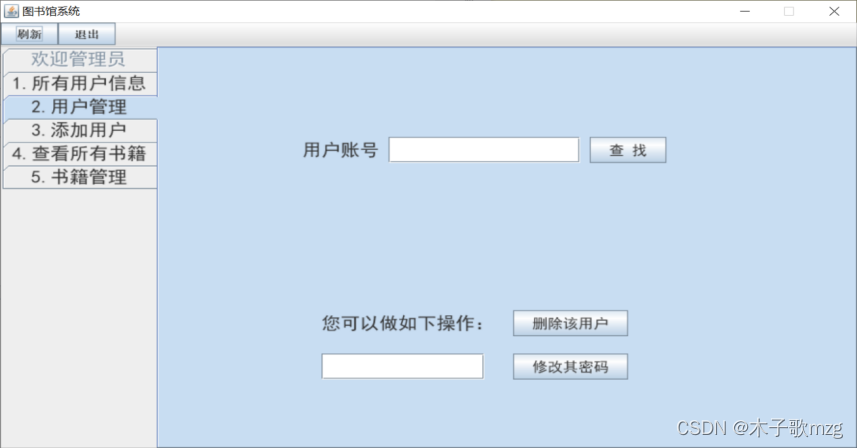 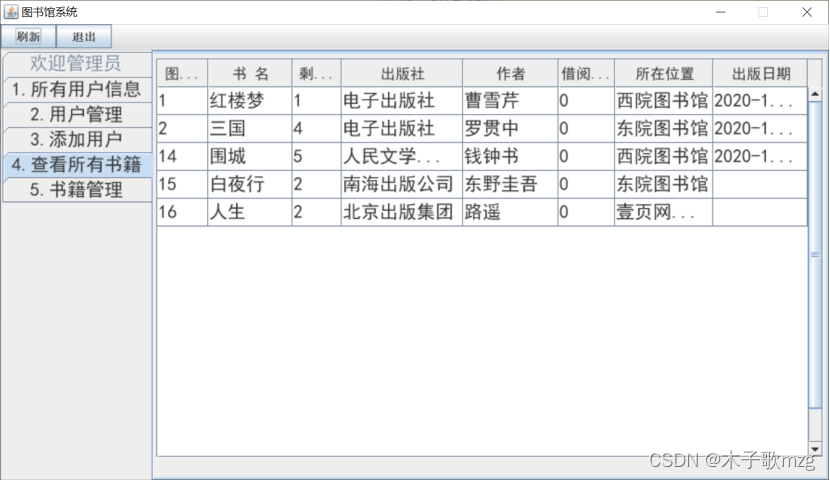
第四部分:数据库
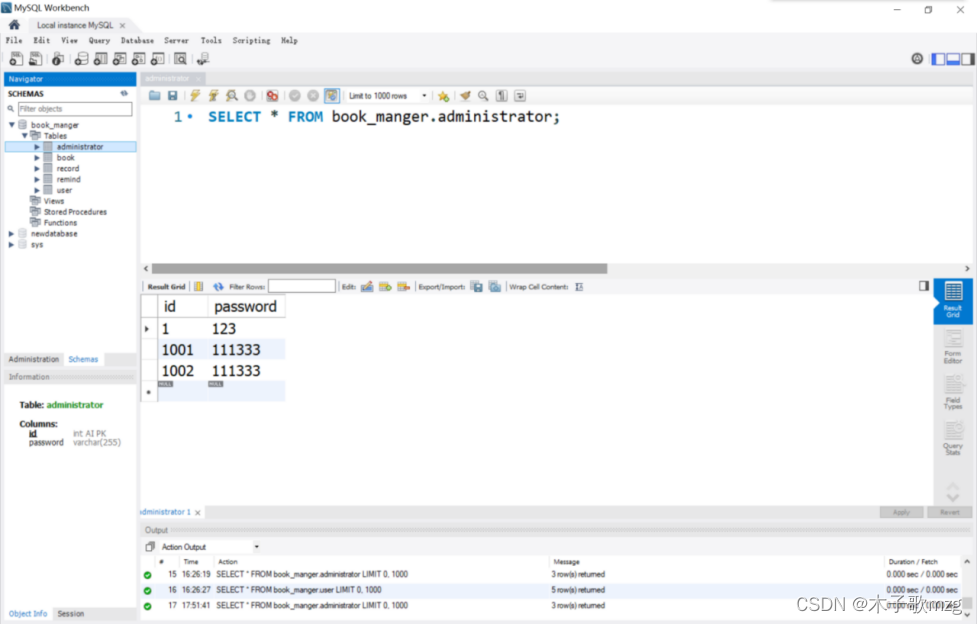
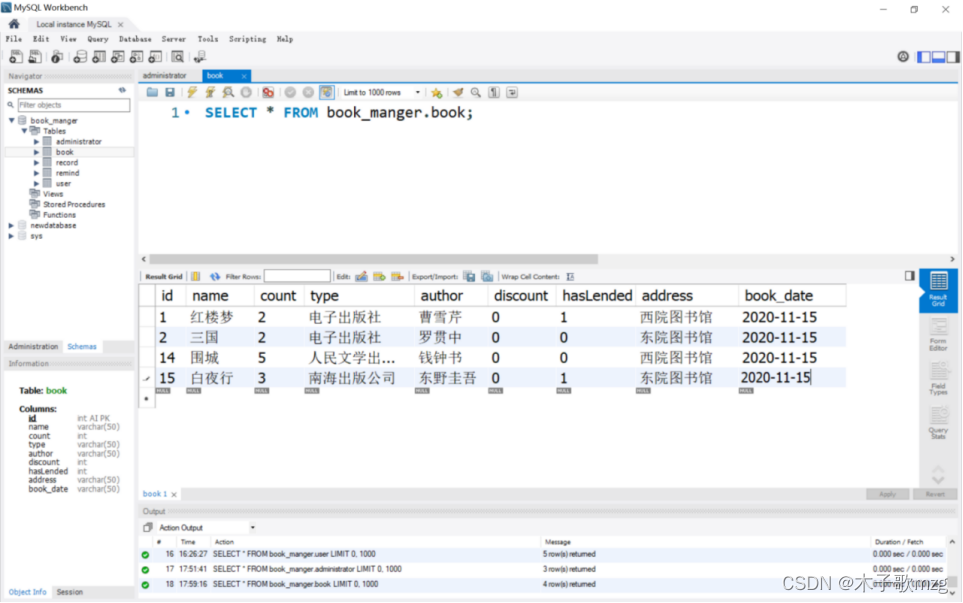 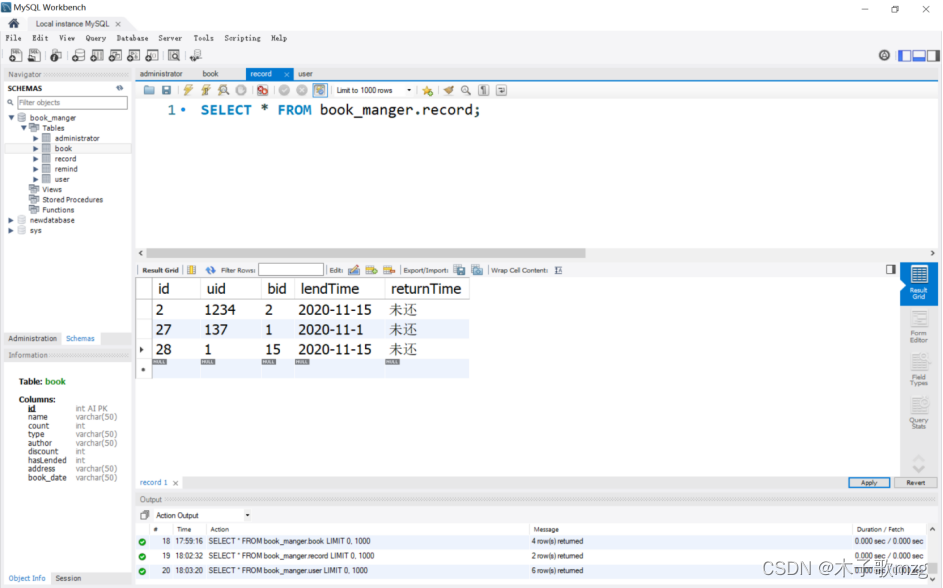
第五部分:部分代码展示
service类:
package service;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import util.DateUtil;
import modle.Book;
import modle.Record;
import dao.BaseDaoImpl;
import dao.BookDaoImpl;
import dao.RecordDaoImpl;
public class BookService {
private static BookService bookService;
static {
bookService = new BookService();
}
private BookDaoImpl bookDao;
private RecordDaoImpl recordDao;
private BookService() {
bookDao = BookDaoImpl.getInstance();
recordDao = RecordDaoImpl.getInstance();
}
public static BookService getInstance() {
return bookService;
}
public static final String CAN = "可借";
public static final String CANT = "无库存";
public boolean lendBook(int uId, String name){
Book bk = bookDao.searchBook(name);
if(bk.getCount() == bk.getHasLended()) {
return false;
}
Record rd = new Record(uId,bk.getId(), DateUtil.getDate(), "未还");
bk.setHasLended(bk.getHasLended() + 1);
bk.setDiscount(bk.getDiscount());
Connection conn = BaseDaoImpl.getConn();
try {
conn.setAutoCommit(false);
} catch (SQLException e1) {
e1.printStackTrace();
}
boolean res = bookDao.updateBook(bk, conn);
if(!res) {
try {
conn.commit();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return false;
}
res = recordDao.insertRecord(rd, conn);
if (!res) {
try {
conn.rollback();
conn.commit();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return false;
} else {
try {
conn.commit();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return true;
}
}
public boolean returnBook(int uId, String name){
Book bk = bookDao.searchBook(name);
if (bk.getId() <= 0){
return false;
}
bk.setHasLended(bk.getHasLended() - 1);
Connection conn = BaseDaoImpl.getConn();
try {
conn.setAutoCommit(false);
} catch (SQLException e1) {
e1.printStackTrace();
}
boolean res = recordDao.updataRecord(uId, bk.getId(), DateUtil.getDate(), conn);
if(!res) {
try {
conn.commit();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return false;
}
res = bookDao.updateBook(bk, conn);
if(!res) {
try {
conn.rollback();
conn.commit();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return false;
} else {
try {
conn.commit();
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return true;
}
}
public Object[][] queryAllBooks() {
List<Book> list = bookDao.queryAllBooks();
Object[][] obj = new Object[list.size()][7];
int i = 0;
for (Book book: list) {
obj[i][0] = book.getHasLended() < book.getCount() ? CAN : CANT;
obj[i][1] = book.getName();
obj[i][2] = book.getCount() - book.getHasLended();
obj[i][3] = book.getType();
obj[i][4] = book.getAuthor();
obj[i][5] = book.getDiscount();
obj[i][6] = book.getAddress();
i++;
}
return obj;
}
public Object[][] queryBooks() {
List<Book> list = bookDao.queryAllBooks();
Object[][] obj = new Object[list.size()][8];
int i = 0;
for (Book book: list) {
obj[i][0] = book.getId();
obj[i][1] = book.getName();
obj[i][2] = book.getCount() - book.getHasLended();
obj[i][3] = book.getType();
obj[i][4] = book.getAuthor();
obj[i][5] = book.getDiscount();
obj[i][6] = book.getAddress();
obj[i][7] = book.getBookDate();
i++;
}
return obj;
}
public boolean insertBook(String name, int count, String type,
String author, String address) {
if (bookDao.searchBook(name).getId() > 0) {
return false;
}
return bookDao.insertBook(name, count, type, author, address);
}
}
package service;
import util.ShowMessageUtil;
import dao.AdminDaoImpl;
import dao.UserDaoImpl;
import modle.Admin;
import modle.User;
public class LoginService {
private static LoginService loginService;
private UserDaoImpl userDao;
private AdminDaoImpl admDao;
private RemindService remindService;
static {
loginService = new LoginService();
}
public static LoginService getInstance() {
return loginService;
}
private LoginService() {
userDao = UserDaoImpl.getInstance();
admDao = AdminDaoImpl.getInstance();
remindService = RemindService.getInstance();
}
public User userLogin(int id, String password) {
User u = userDao.queryUser(id, password);
if (u != null) {
String remind = remindService.getRemindByUid(id);
if(remind != null && !remind.equals("")) {
ShowMessageUtil.winMessage("您预约的书" + remind + "需要尽快归还!\n赶紧去看看吧!");
}
}
return u;
}
public Admin adminLogin(int id, String password) {
return admDao.queryAdmin(id, password);
}
}
package service;
import java.util.List;
import modle.Book;
import dao.RemindDaoImpl;
public class RemindService {
public static final String PRE = "《";
public static final String TAIL = "》";
public static final String SPACE = "、";
public static final String SPLIT = ",";
private static RemindService remindService;
static {
remindService = new RemindService();
}
private RemindDaoImpl remindDao;
private RemindService() {
remindDao = RemindDaoImpl.getInstance();
}
public static RemindService getInstance() {
return remindService;
}
public String getRemindByUid(int id) {
List<Book> books = remindDao.getRemindByUid(id);
StringBuilder str = new StringBuilder();
StringBuilder ids = new StringBuilder();
if (books.size() > 0) {
str.append(PRE).append(books.get(0).getName()).append(TAIL);
ids.append(books.get(0).getId());
}
for(int j = 1; j < books.size(); j++) {
str.append(SPACE).append(PRE);
str.append(books.get(j).getName()).append(TAIL);
ids.append(SPLIT);
ids.append(books.get(j).getId());
}
if (ids.length() > 0) {
remindDao.updateRemindBatch(ids.toString());
}
return str.toString();
}
}
dao类:
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import modle.*;
public class AdminDaoImpl {
private static AdminDaoImpl adminDaoImpl;
static {
adminDaoImpl = new AdminDaoImpl();
}
private AdminDaoImpl() {}
public static AdminDaoImpl getInstance() {
return adminDaoImpl;
}
public Admin queryAdmin(int id, String password) {
String sql = "select * from Administrator where id = ? and password = ?";
Connection conn = BaseDaoImpl.getConn();
ResultSet rs = null;
Admin admin = null;
PreparedStatement psts = null;
try {
psts = conn.prepareStatement(sql);
psts.setObject(1, id);
psts.setObject(2, password);
rs = psts.executeQuery();
while (rs.next()) {
int return_id = rs.getInt("id");
String return_password = rs.getString("password");
admin = new Admin(return_id, return_password);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
psts.close();
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return admin;
}
}
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import modle.Book;
public class BookDaoImpl {
private static BookDaoImpl bookDao;
static {
bookDao = new BookDaoImpl();
}
private BookDaoImpl() {}
public static BookDaoImpl getInstance() {
return bookDao;
}
public boolean insertBook(String name, int count, String type,
String author, String address) {
String sql = "insert into " +
"Book(name, count, type, author, discount, hasLended, address)" +
"values(?,?,?,?,?,?,?)";
Connection conn = BaseDaoImpl.getConn();
PreparedStatement psts = null;
try {
psts = conn.prepareStatement(sql);
psts.setObject(1, name);
psts.setObject(2, count);
psts.setObject(3, type);
psts.setObject(4, author);
psts.setObject(5, 0);
psts.setObject(6, 0);
psts.setObject(7, address);
return psts.executeUpdate() > 0;
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
psts.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return false;
}
public List<Book> queryAllBooks() {
String sql = "select * from Book";
Connection conn = BaseDaoImpl.getConn();
ResultSet rs = null;
List<Book> list = new ArrayList<Book>();
PreparedStatement psts = null;
try {
psts = conn.prepareStatement(sql);
rs = psts.executeQuery();
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int count = rs.getInt("count");
String type = rs.getString("type");
String author = rs.getString("author");
int discount = rs.getInt("discount");
int hasLended = rs.getInt("hasLended");
String address = rs.getString("address");
String book_date = rs.getString("book_date");
Book book = new Book(id, name, count,type,author,discount,hasLended,address,book_date);
list.add(book);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
psts.close();
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return list;
}
public boolean delBook(String name) {
String sql="delete from Book where name = '" + name + "'";
Connection conn = BaseDaoImpl.getConn();
PreparedStatement psts = null;
try {
psts = conn.prepareStatement(sql);
int res = psts.executeUpdate();
if(res > 0) {
return true;
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
psts.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return false;
}
public boolean updateBook(Book book, Connection conn) {
boolean isRelease = false;
String sql = "update Book set ";
if (book.getCount() != 0) {
sql += "count="+book.getCount() + ",";
}
if (book.getDiscount() != 0) {
sql += "discount="+book.getDiscount() + ",";
}
if (book.getHasLended() != 0) {
sql += "hasLended="+book.getHasLended() + ",";
}
if (book.getType() != null) {
sql += "type='"+book.getType() + "',";
}
if (book.getAddress() != null) {
sql += "address='"+book.getAddress() + "' ,";
}
if (book.getAuthor() != null) {
sql += "author='"+book.getAuthor() + "' ,";
}
sql = sql.substring(0, sql.length() - 1);
sql += " where name='" + book.getName() + "'";
System.out.println(sql);
if(conn == null) {
conn = BaseDaoImpl.getConn();
isRelease = true;
}
PreparedStatement psts = null;
try {
psts = conn.prepareStatement(sql);
int res = psts.executeUpdate();
if(res > 0) return true;
} catch (SQLException e) {
e.printStackTrace();
} finally{
try {
if (isRelease && conn != null) conn.close();
if (psts != null) psts.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return false;
}
public Book searchBook(String name) {
String sql = "select * from Book where name = '" + name + "'";
Connection conn = BaseDaoImpl.getConn();
ResultSet rs = null;
Book book = new Book();
PreparedStatement psts = null;
try {
psts = conn.prepareStatement(sql);
rs = psts.executeQuery();
if (rs.next()) {
book.setId(rs.getInt("id"));
book.setName(rs.getString("name"));
book.setCount(rs.getInt("count"));
book.setType(rs.getString("type"));
book.setAuthor(rs.getString("author"));
book.setDiscount(rs.getInt("discount"));
book.setHasLended(rs.getInt("hasLended"));
book.setAddress(rs.getString("address"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
psts.close();
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return book;
}
public Book queryBook(int bId) {
String sql = "select * from Book where id = " + bId;
Connection conn = BaseDaoImpl.getConn();
ResultSet rs = null;
Book book = new Book();
PreparedStatement psts = null;
try {
psts = conn.prepareStatement(sql);
rs = psts.executeQuery();
book.setId(rs.getInt("id"));
book.setName(rs.getString("name"));
book.setCount(rs.getInt("count"));
book.setType(rs.getString("type"));
book.setAuthor(rs.getString("author"));
book.setDiscount(rs.getInt("discount"));
book.setHasLended(rs.getInt("hasLended"));
book.setAddress(rs.getString("address"));
} catch (SQLException e) {
e.printStackTrace();
} finally {
try {
conn.close();
psts.close();
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
return book;
}
}
第六部分:完整代码
点击链接即可下载:https://download.csdn.net/download/qq_47471385/85101745
|