import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
import java.util.concurrent.TimeUnit;
@Service
public class RedisUtil {
@Autowired
RedisTemplate redisTemplate;
public <T> T getObject(String key, Class<T> clazz) {
Object valueObj = redisTemplate.opsForValue().get(key);
if (valueObj == null) {
return null;
}
if (clazz.isInstance(valueObj)) {
return (T) valueObj;
} else if (clazz == Long.class && valueObj instanceof Integer) {
Integer obj = (Integer) valueObj;
return (T) Long.valueOf(obj.longValue());
}
return null;
}
public void setObject(String key, Object value, Long second) {
redisTemplate.opsForValue().set(key, value, second, TimeUnit.SECONDS);
}
public void setObjectForever(String key, Object value) {
redisTemplate.opsForValue().set(key, value);
}
public Long increment(String key, Long second) {
Long value = redisTemplate.opsForValue().increment(key);
redisTemplate.expire(key, second, TimeUnit.SECONDS);
return value;
}
public void decrement(String key) {
redisTemplate.opsForValue().decrement(key);
}
public boolean setIfAbsent(String key, Long second) {
return redisTemplate.opsForValue().setIfAbsent(key, "1", second, TimeUnit.SECONDS);
}
}
import cn.hutool.core.date.DateUtil;
import com.easy.hotel.pms.service.impl.RedisUtil;
import lombok.AllArgsConstructor;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
import java.util.Date;
@Component
@AllArgsConstructor
public class RedisGeoCommunityRunner {
private final RedisUtil redisUtil;
private static String redisGeoCommunityCount = "REDIS_GEO_COMMUNITY_COUNT";
/*
@Scheduled是设计来定期执行操作的,不是只执行一次。
ps.定时器是跑在服务端的任务调度,为什么会和客户端的请求联系起来呢?你的描述是不是有些问题
如果只想执行一次,
1.粗暴的方式是在这个scheduler里设置标识位,代码进行判断,执行过了就直接返回了
2.cron表达式具体到时间,如0 0 21 18 8 ? 2020,这种方式的肯定只执行一次
3.如果是项目启动时需要做一些操作,web.xml里可以配置应用启、停的监听
4.如果是bean组件实例化后进行一次性操作,@PostConstruct注解可以满足
5.如果想项目启动x秒后,进行一次性操作,quartz有SimpleTrigger接口,可以配置repeatCount=1
*/
@Scheduled(cron = "*/5 * * * * ?")//每隔5秒执行一次
public void totalIndex() {
try {
System.out.println(com.easy.hotel.pms.util.DateUtil.toDateTimeStr(DateUtil.toLocalDateTime(new Date())) );
Integer count = redisUtil.getObject(redisGeoCommunityCount, Integer.class);
if(count == null){
redisUtil.setObjectForever(redisGeoCommunityCount,1);
count = redisUtil.getObject(redisGeoCommunityCount, Integer.class);
}
if(count > 1){
return ;
}else{
redisUtil.setObjectForever(redisGeoCommunityCount,2);
}
execteOnlyOnce( );
} catch (Exception e) {
e.printStackTrace();
}
}
private void execteOnlyOnce( ) {
System.out.println("只执行一次");
}
}
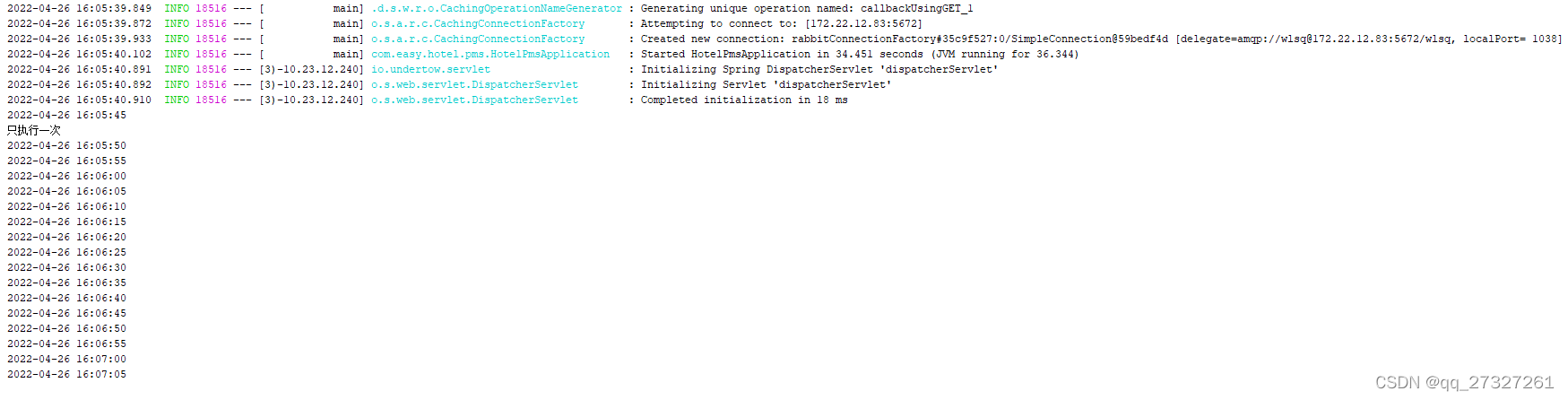
?
|