一、需求
JAVA实现如下图所示的“标签”列表接口: 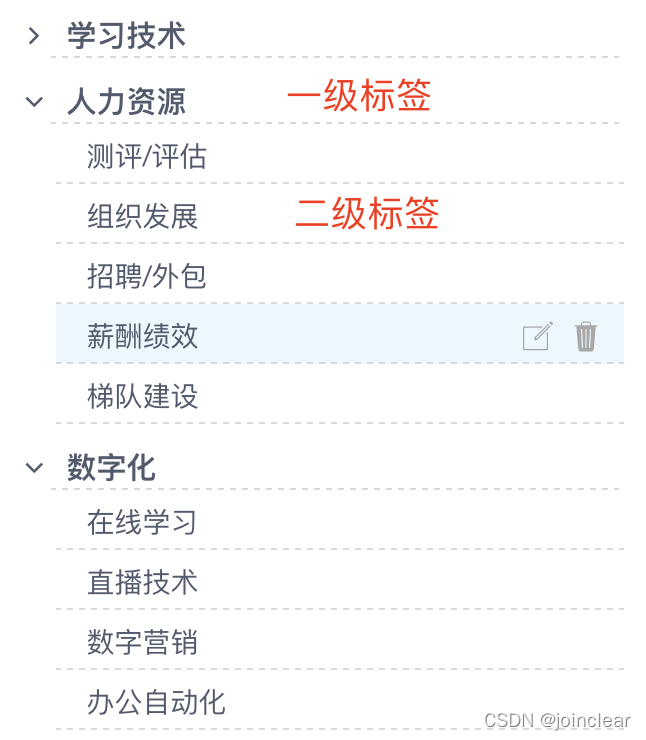 学习技术、人力资源、数字化为“一级标签”,下面的为“二级子标签”。
二、数据库表设计
一级标签表db_label:
DROP TABLE IF EXISTS `db_label`;
CREATE TABLE `db_label` (
`id` bigint(20) NOT NULL AUTO_INCREMENT,
`name` varchar(100) CHARACTER SET utf8mb4 NOT NULL DEFAULT '' COMMENT '名称',
`createtime` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP COMMENT '创建时间',
`modifytime` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP COMMENT '修改时间',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='一级标签表';
二级标签表db_label_sub:
DROP TABLE IF EXISTS `db_label_sub`;
CREATE TABLE `db_label_sub` (
`id` bigint(20) NOT NULL AUTO_INCREMENT,
`label_id` bigint(20) NOT NULL COMMENT '一级标签id',
`name` varchar(100) CHARACTER SET utf8mb4 NOT NULL DEFAULT '' COMMENT '名称',
`createtime` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP COMMENT '创建时间',
`modifytime` datetime NOT NULL DEFAULT CURRENT_TIMESTAMP COMMENT '修改时间',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=1 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_bin COMMENT='二级标签表';
三、DTO
一级标签DTO:
@Getter
@Setter
public class LabelDto extends Serializable {
private static final long serialVersionUID = 1L;
private String name;
private List<LabelSubDto> labelSubDtoList;
}
二级标签DTO:
@Getter
@Setter
public class LabelSubDto extends Serializable {
private static final long serialVersionUID = 1L;
private Long labelId;
private String name;
}
四、接口
@PostMapping("/list")
@ResponseBody
public Result<List<LabelDto>> list(){
return Result.success(service.list());
}
五、mybatis实现映射
这种 一级标签DTO里面包含二级标签列表List的情况,要使用<resultMap>中的 <collection>来实现,使用 <collection>可以解决这种关联的一对多关系。
LabelMapper.xml文件如下:
<resultMap id="LabelResultMap" type="com.test.bi.bo.LabelDto">
<id column="id" jdbcType="BIGINT" property="id" />
<result column="name" jdbcType="VARCHAR" property="name" />
<result column="createtime" jdbcType="TIMESTAMP" property="createtime" />
<result column="modifytime" jdbcType="TIMESTAMP" property="modifytime" />
<collection property="LabelSubDtoList" ofType="com.test.bi.bo.LabelSubDto">
<id column="bid" jdbcType="BIGINT" property="id" />
<result column="label_id" jdbcType="BIGINT" property="labelId" />
<result column="bname" jdbcType="VARCHAR" property="name" />
<result column="bcreatetime" jdbcType="TIMESTAMP" property="createtime" />
<result column="bmodifytime" jdbcType="TIMESTAMP" property="modifytime" />
</collection>
</resultMap>
<select id="list" resultMap="LabelResultMap">
SELECT a.*,
b.id as bid, b.label_id, b.name as bname
FROM db_label a
Left JOIN db_label_sub b
ON a.id = b.label_id
</select>
注意:
<collection property="LabelSubDtoList" ofType="com.test.bi.bo.LabelSubDto">
property值对应LabelDto里面的:
private List<LabelSubDto> labelSubDtoList;
}
|