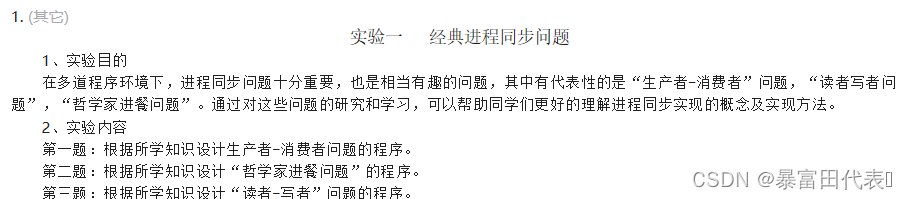 1.消费者生产者
import java.util.LinkedList;
import java.util.Queue;
public class 消费者生产者 {
public static void main(String[] args) {
Buffer buffer = new Buffer();
Consumer consumer = new Consumer(buffer);
Producer producer = new Producer(buffer);
producer.start();
consumer.start();
}
}
class Producer extends Thread{
private Buffer buffer;
public Producer(Buffer buffer) {
this.buffer = buffer;
}
public void run() {
for(int i=0;i<10;i++) {
try {
buffer.add(i);
Thread.sleep(500);
System.out.println("生产"+i);
} catch (InterruptedException e1) {
e1.printStackTrace();
}
}
}
}
class Consumer extends Thread{
private Buffer buffer;
public Consumer(Buffer buffer) {
this.buffer = buffer;
}
@Override
public void run() {
for(int i=0;i<10;i++) {
try {
int val =buffer.pull();
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("消费"+i);
}
}
}
class Buffer{
private Queue<Integer> queue = new LinkedList<>();
private int size=5;
public synchronized void add(int val) throws InterruptedException {
if(queue.size()>size) {
wait();
}
queue.add(val);
notify();
}
public synchronized int pull() throws InterruptedException {
if(queue.size()==0) {
wait();
}
int val=queue.poll();
notify();
return val;
}
}
2.哲学家进餐问题
package 实验一;
public class 哲学家进餐问题 {
public static void main(String[] args) {
Philosopher p1=new Philosopher(0);
Philosopher p2=new Philosopher(1);
Philosopher p3=new Philosopher(2);
Philosopher p4=new Philosopher(3);
Philosopher p5=new Philosopher(4);
new Thread(p1,"0").start();
new Thread(p2,"1").start();
new Thread(p3,"2").start();
new Thread(p4,"3").start();
new Thread(p5,"4").start();
}
}
class Philosopher extends Thread{
private int index;
public static Chopstick chop=new Chopstick();
public Philosopher(int index) {
this.index = index;
}
public synchronized void thinking(){
System.out.println("哲学家"+index+"在思考");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public synchronized void eating(){
System.out.println("哲学家"+index+"在吃饭");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
@Override
public void run() {
while(true){
thinking();
chop.takeChopsticks(index);
eating();
chop.putChopsticks(index);
}
}
}
class Chopstick {
public boolean[] isUsing=new boolean[5];
public synchronized void takeChopsticks(int index){
while(isUsing[index]||isUsing[(index+1)%5]){
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
isUsing[index]=true;
isUsing[(index+1)%5]=true;
System.out.println("哲学家"+index+"拿起筷子");
}
public synchronized void putChopsticks(int index){
isUsing[index]=false;
isUsing[(index+1)%5]=false;
System.out.println("哲学家"+index+"放下筷子");
notify();
}
}
3.读者写者问题
package 实验一;
import java.util.concurrent.Semaphore;
public class 读者写者问题 {
public static void main(String[] args) throws InterruptedException {
int writerNumber=3;
int readerNumber=7;
Disk disks=new Disk();
Thread[] writer_threads=new Thread[writerNumber];
Writer[] writers=new Writer[writerNumber];
Thread[] reader_threads=new Thread[readerNumber];
Reader[] readers=new Reader[readerNumber];
for (int i=0;i<writerNumber/2;i++)
{
writers[i]=new Writer(disks);
writer_threads[i]=new Thread(writers[i],Integer.toString(i));
writer_threads[i].start();
}
Thread.sleep(100);
for (int i=0;i<readerNumber;i++)
{
readers[i]=new Reader(disks);
reader_threads[i]=new Thread(readers[i],Integer.toString(i));
reader_threads[i].start();
}
for (int i=writerNumber/2;i<writerNumber;i++)
{
writers[i]=new Writer(disks);
writer_threads[i]=new Thread(writers[i],Integer.toString(i));
writer_threads[i].start();
}
}
}
class Disk extends Thread{
private String data_str;
private int reader_count;
private Semaphore write_mutex;
private Semaphore read_mutex;
private Semaphore read_count_mutex;
Disk()
{
write_mutex=new Semaphore(1);
read_mutex=new Semaphore(1000);
read_count_mutex=new Semaphore(1);
}
public void start_read() throws InterruptedException {
while (write_mutex.availablePermits()==0){ }
read_count_mutex.acquire();
reader_count++;
read_count_mutex.release();
read_mutex.acquire();
}
public void finish_read() throws InterruptedException {
read_count_mutex.acquire();
reader_count--;
read_count_mutex.release();
read_mutex.release();
}
public void start_write() throws InterruptedException {
write_mutex.acquire();
while (getReader_count()!=0){}
}
public void finish_write()
{
write_mutex.release();
}
public String read() throws InterruptedException {
return data_str;
}
public String write(String new_data) throws InterruptedException {
this.data_str=new_data;
return data_str;
}
public int getReader_count() {
return reader_count;
}
public void setReader_count(int reader_count) {
this.reader_count = reader_count;
}
}
class Reader extends Thread {
private Disk disk;
public Reader(Disk disk) {
this.disk = disk;
}
@Override
public void run() {
for (int i=10;i>=0;i--)
{
try {
read();
} catch (InterruptedException e) {
e.printStackTrace();
}
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private void read() throws InterruptedException {
disk.start_read();
String str=disk.read();
System.out.println("读操作:"+Thread.currentThread().getId()+" 现在的数据为:"+str);
disk.finish_read();
}
}
class Writer extends Thread{
private Disk disk;
public Writer(Disk disk) {
this.disk = disk;
}
public void run() {
for (int i=10;i>=0;i--)
{
try {
write();
} catch (InterruptedException e) {
e.printStackTrace();
}
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private void write() throws InterruptedException {
disk.start_write();
String str=disk.write("data+"+Thread.currentThread().getId());
System.out.println("写操作:"+Thread.currentThread().getId()+" 现在的数据为:"+str);
disk.finish_write();
}
}
|