MongoDB 学习
第一章: MongoDB学习(一) 详细安装教程 win10
第二章:MongoDB学习(二)sql语法
第三章:MongoDB学习(三)SpringBoot整合MongoDB
连接工具
连接工具可以选择上一章节中Mongodb自带的MongodbCompass。我这里采用的是Navicat 15。 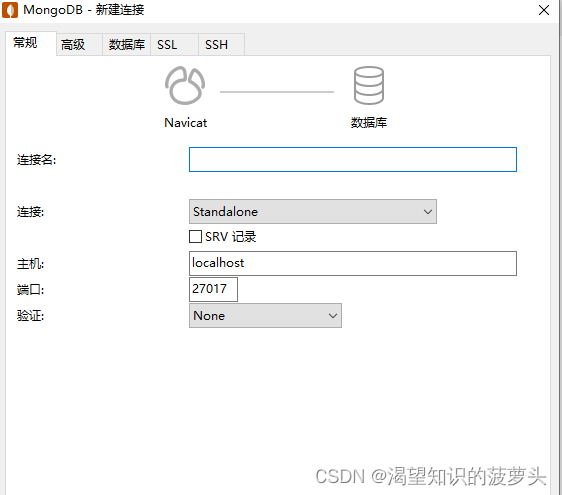
和关系型数据库的对比
MongoDB是非关系型数据库当中最像关系型数据库的,所以我们通过它与关系型数据库的对比,来了解下它的概念。
SQL概念 | MongoDB概念 | 说明 |
---|
database | database | 数据库 | table | collection | 数据库表/集合 | row | document | 行/文档 | column | field | 字段/域 | index | index | 索引 | primary key | primary key | 主键/MongoDB自动将_id字段设置为主键 |
语法
1. 数据库(database)操作
创建数据库 使用use命令去创建数据库,当插入第一条数据时会创建数据库,例如创建一个test数据库;
> use test
switched to db test
> db.article.insert({name:"MongoDB 教程"})
WriteResult({ "nInserted" : 1 })
> show dbs
admin 0.000GB
config 0.000GB
local 0.000GB
test 0.000GB
删除数据库 使用db对象中的dropDatabase()方法来删除;
> db.dropDatabase()
{ "dropped" : "test", "ok" : 1 }
> show dbs
admin 0.000GB
config 0.000GB
local 0.000GB
2. 集合(collection)操作
创建集合 使用db对象中的createCollection()方法来创建集合,例如创建一个student集合;
> use test
switched to db test
> db.createCollection("student")
{ "ok" : 1 }
> show collections
student
删除集合 使用collection对象的drop()方法来删除集合,例如删除一个student集合;
> db.student.drop()
true
> show collections
3. 文档(document)操作
3.1 插入数据(文档)
db.collection.insert(document);
db.student.insert([
{
'name':'小红',
'age':7,
'class':'小(二)班',
'grade':[88,99,85]
},
{
'name':'小明',
'age':8,
'class':'小(二)班',
'grade':[83,90,85]
},
{
'name':'张华',
'age':8,
'class':'小(一)班',
'grade':[90,85,90]
}
]);
3.2 查询数据(文档)
db.article.find({})
db.student.find();
db.student.find({'class':'小(二)班'});
db.student.distinct('class');
db.student.find({age: {$gt: 7}});
db.student.find({age: {$lt: 8}});
db.student.find({age: {$gte: 7}});
db.student.find({age: {$lte: 8}});
db.student.find({age: {$gte: 7, $lt: 8}});
db.student.find({name: /小/});
db.student.find({name: /^小/});
db.student.find({name:{$regex:'小'}});
db.student.find({},{name: 1, age: 1});
db.student.find().sort({age: 1});
db.student.find().sort({age: -1});
db.student.find().limit(2);
db.student.find().sort({age: -1}).limit(2);
db.student.find().skip(2);
db.student.find().limit(3).skip(1);
db.student.find({$or: [{name: /小/},{age: 8}]});
db.student.findOne();
db.student.find().limit(1);
db.student.find({}).count();
db.student.aggregate([{
$match: {
age: {
$gt: 8
}
}
}, {
$group: {
"_id": {
"class": "$class",
"age": "$age"
},
"total": {
"$sum": 1
}
}
}, {
$project: {
"_id": 0,
"name": "$_id.class",
"age": "$_id.age",
"count": "$total"
}
}])
3.3 更新数据(文档)
db.collection.update(
<query>,
<update>,
{
multi: <boolean>
}
)
db.student.update({'name':'小红'},{$set:{'age':9}},{multi:true});
db.student.save(
{
'_id': ObjectId('62a154ad345f0000ab005e14'),
'name':'小红',
'age':9,
'class':'小(二)班',
'grade':[88,99,85]
});
3.4 删除数据(文档)
db.collection.remove(
<query>,
{
justOne: <boolean>
}
)
db.student.remove({'name':'小红'});
|