本文有借鉴别人的代码,但是也是自己理解之后写出来的,文中有注释,并且有个别文件也用红框和黄字体讲解,并且能解决其他文件90%看不懂的问题,如果还是看不懂清留言,源码在文章末尾,附上了全部代码,这个注册系统比较简陋,供大家学习参考。 # 一、系统简介(思维导图) 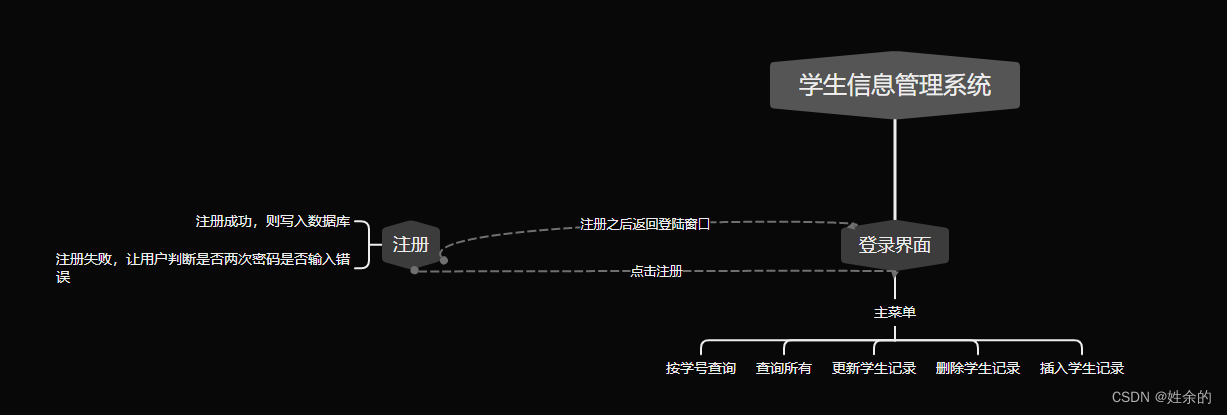
**这是一个简单的思维导图大家将就着看 **不知道思维导图能不能显示出来,可能有点问题,但是复制链接能访问,如果有需要我会补上
二、JDBC模块
1、声明所需
public static final String JDBC_DRIVER = "com.mysql.cj.jdbc.Driver";
public static final String DB_URL = "jdbc:mysql://localhost:3306/java_sims?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=UTC";
public static final String username = "root";
public static final String password = "111111";
private Connection connection = null;
private PreparedStatement pStatement = null;
private ResultSet rSet = null;
以上代码是调用JDBC 的jar包 并且设置参数和初始化,版本低一点的JDBC 包可以删除第一行中间的.jc 有一点要注意的是 代码中 java_sims 是我的数据库名,还有在 java_sims 之后的可以不写,但是有可能会报错,还是建议写上至于参数是false 还是true 看你报错的地方让你设置什么 然后就是数据库账号和密码 记得根据自己的写昂
然后就是一些先行初始化的东西
2、加载驱动
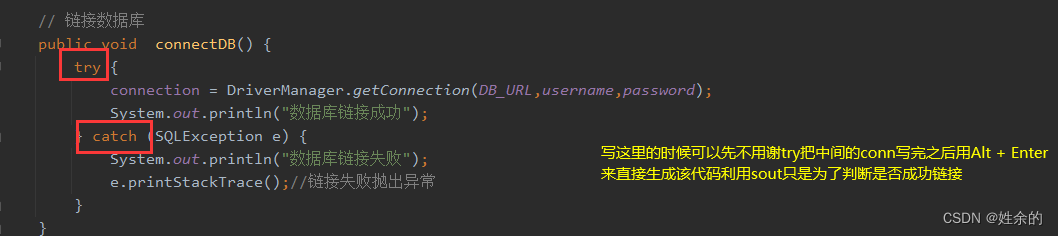 在这里我们能看见一个conn 这个是调用数据库驱动,用来链接数据库。
3、关闭资源
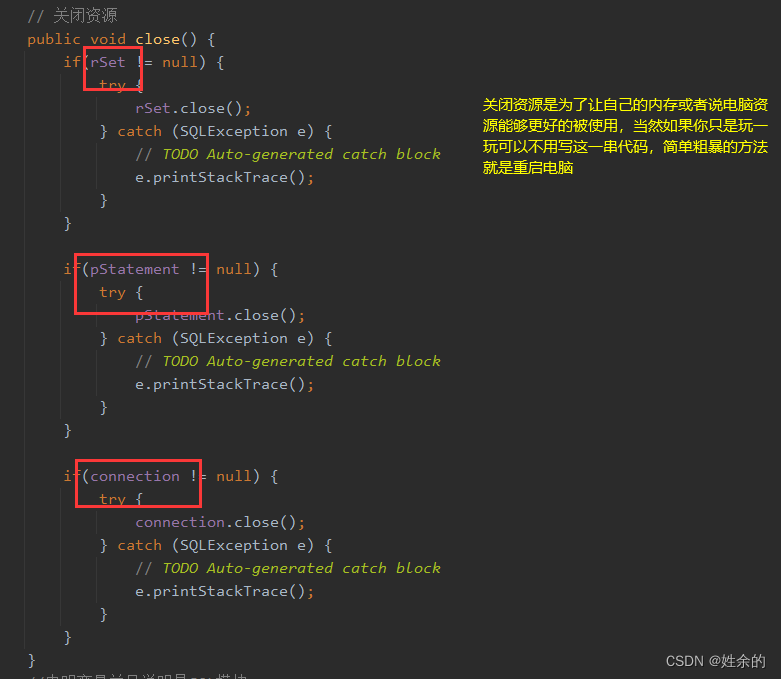
4、插入记录
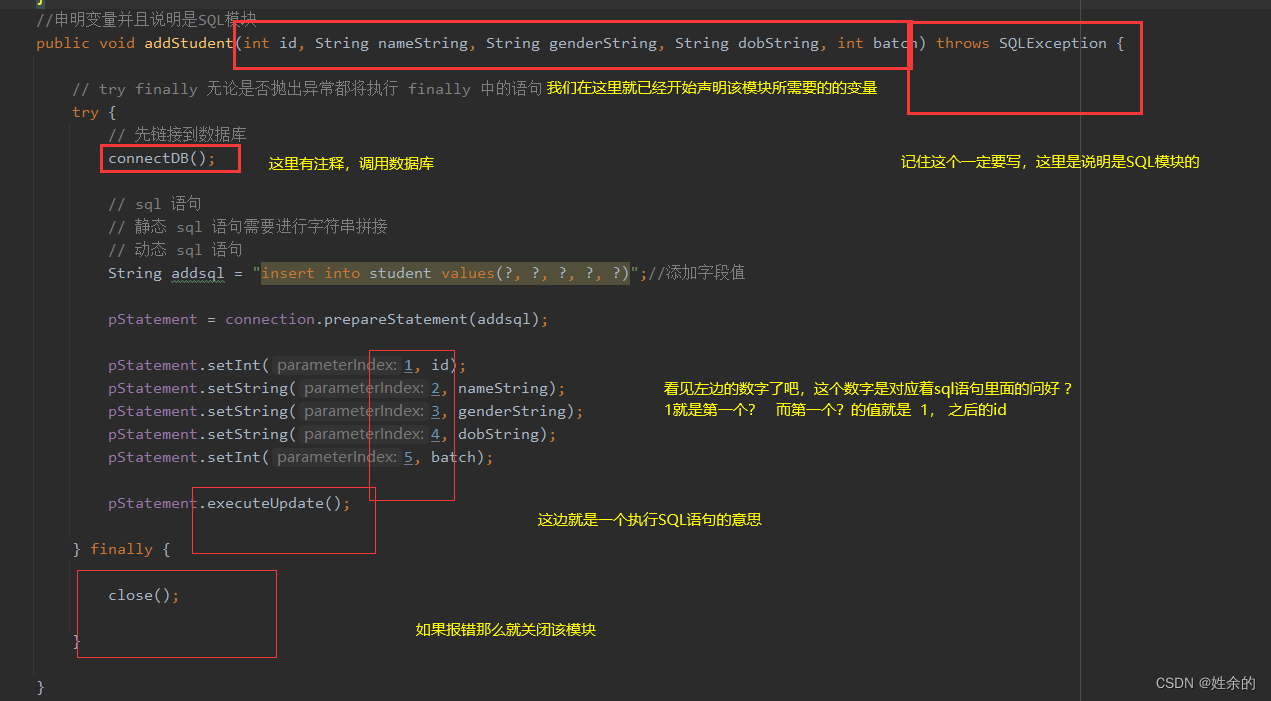
5、更新记录
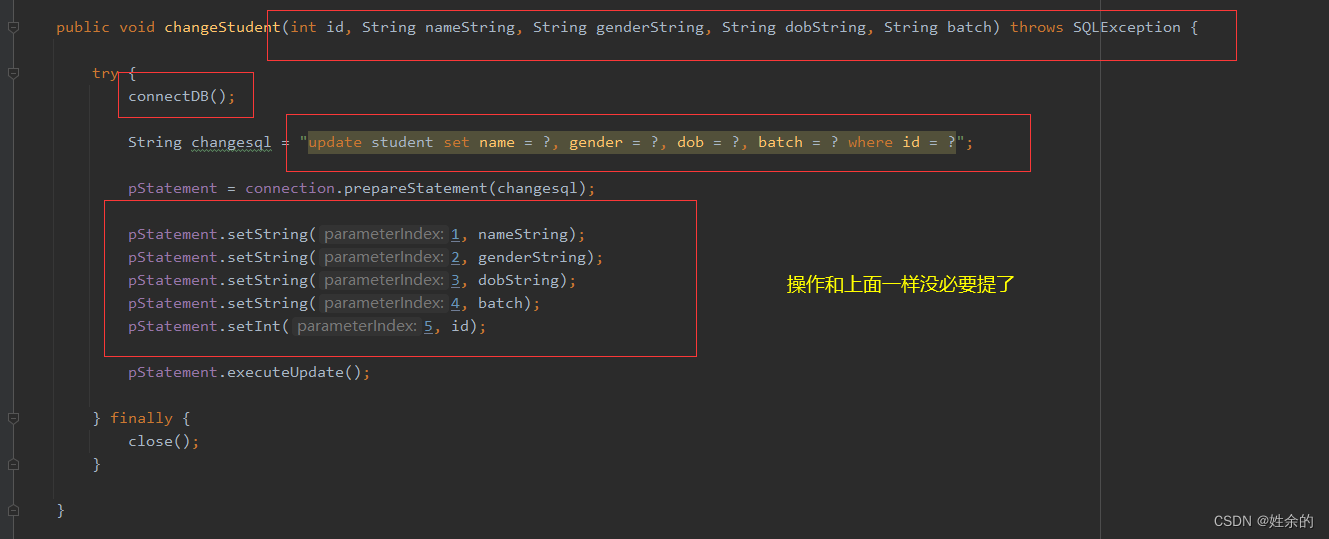
6、删除记录
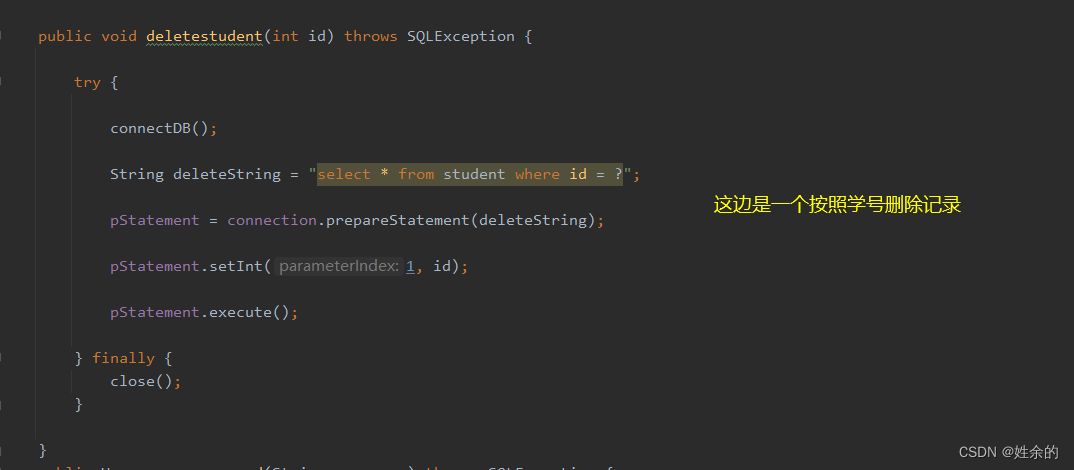
7、按学号查询学生记录
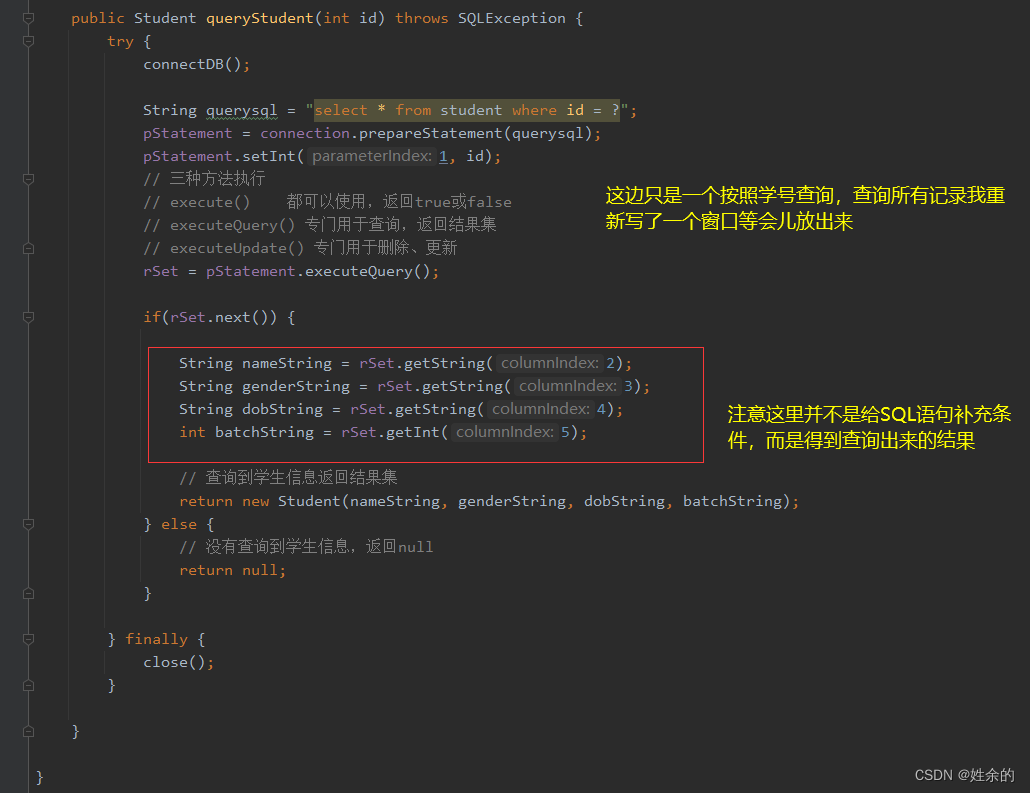
三、Swing(所有的窗口)
Swing+JDBC 因为这里我有一个文件包含了JDBC和Swing所以先把它拿来讲解
1)先是JDBC 部分
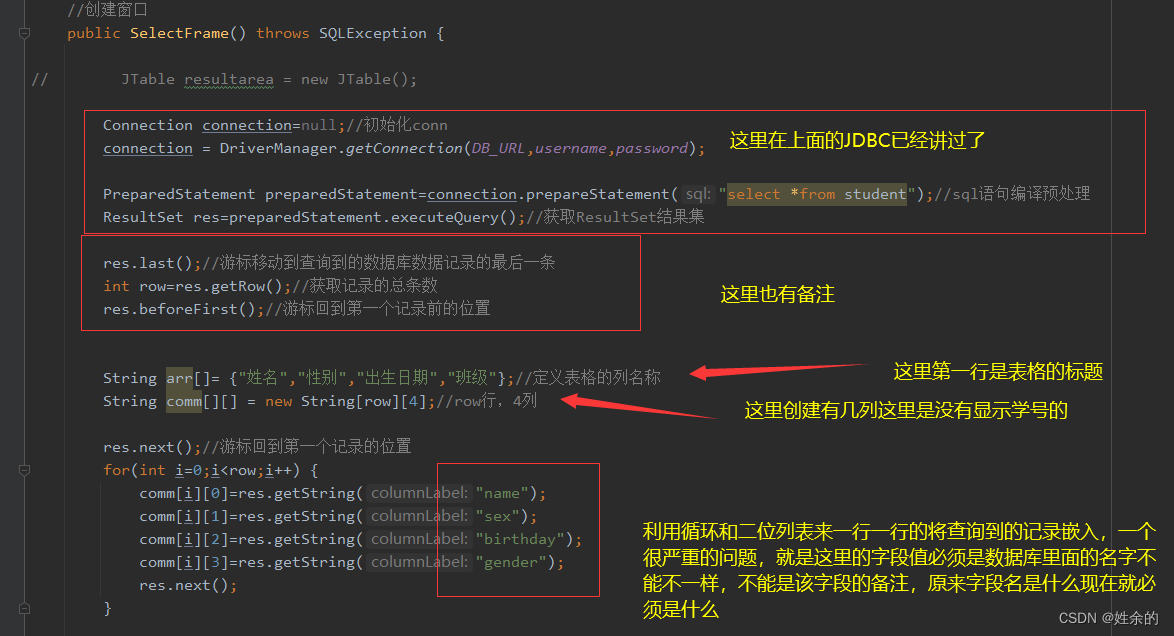
2)然后就是Swing 部分
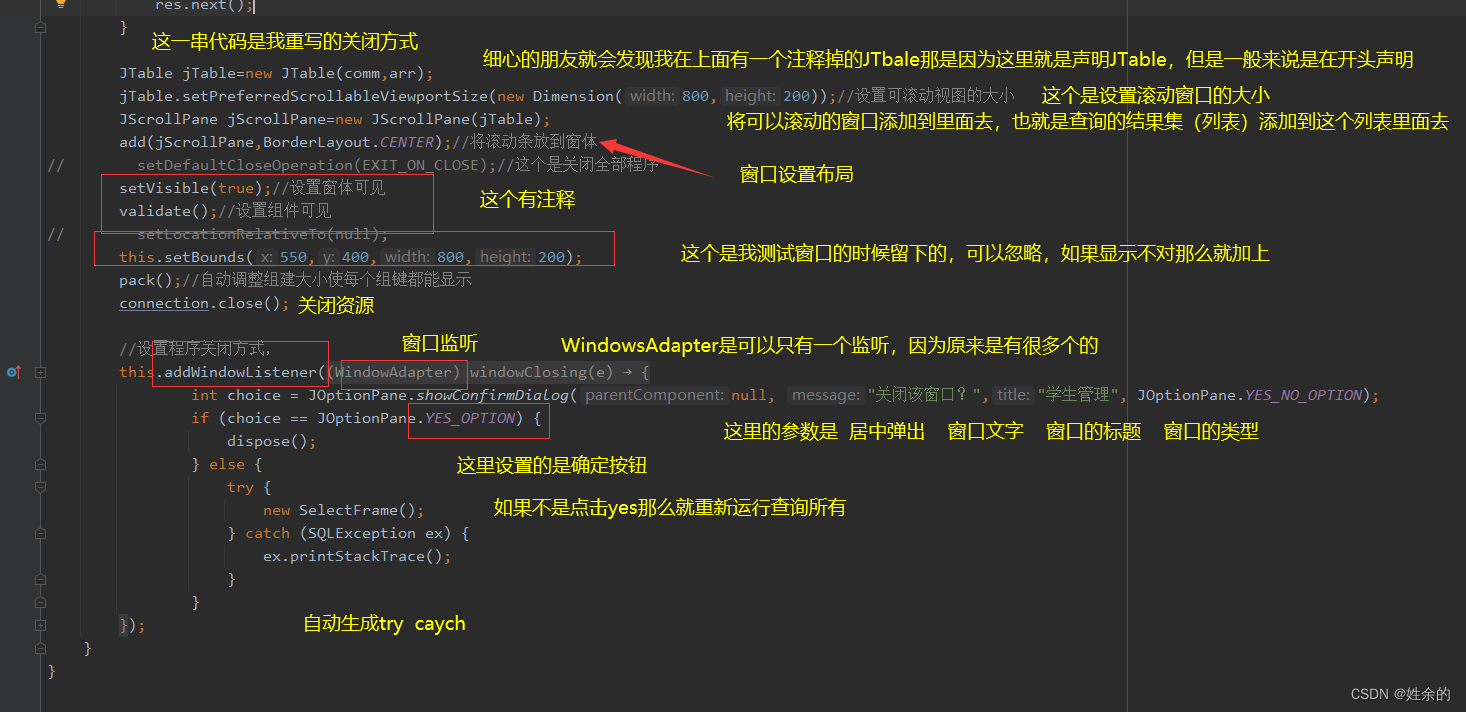
四、接下来就是放源码
我会将源码分JDBC 和Swing 放在两个标题下,每个代码上面都有一个标题看表示文件名 在放JDBC 和Swing 源码前有两个文件需要构造
Student
package StudentManager;
public class Student {
private String name;
private String gender;
private String dob;
private int batch;
public Student(String name, String gender, String dob, int batch) {
this.name = name;
this.gender = gender;
this.dob = dob;
this.batch = batch;
}
public String getName() {
return name;
}
public String getGender() {
return gender;
}
public String getDob() {
return dob;
}
public int getBatch() {
return batch;
}
}
User
package StudentManager;
public class User {
private String username;
private String password;
public User(String username, String password){
this.username = username;
this.password = password;
};
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
还有一个文件需要写就是注册文字的监听
Regevent
package StudentManager;
import StudentManager.test.RegisterFrame;
import javax.swing.*;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
public class Regevent implements MouseListener {
@Override
public void mouseClicked(MouseEvent e) {
if(e.getButton()==MouseEvent.BUTTON1){
JOptionPane.showMessageDialog(null, "hello");
Register r = new Register("用户注册");
}
}
@Override
public void mousePressed(MouseEvent e) {
System.out.println("按住经过");
}
@Override
public void mouseReleased(MouseEvent e) {
System.out.println("按住鼠标");
}
@Override
public void mouseEntered(MouseEvent e) {
System.out.println("滑动鼠标");
}
@Override
public void mouseExited(MouseEvent e) {
System.out.println("鼠标退出");
}
}
JDBC代码
SQLHelp
package StudentManager;
import java.sql.*;
public class SQLHelp {
public static final String JDBC_DRIVER = "com.mysql.cj.jdbc.Driver";
public static final String DB_URL = "jdbc:mysql://localhost:3306/java_sims?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=UTC";
public static final String username = "root";
public static final String password = "111111";
private Connection connection = null;
private PreparedStatement pStatement = null;
private ResultSet rSet = null;
static {
try {
Class.forName(JDBC_DRIVER);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public void connectDB() {
try {
connection = DriverManager.getConnection(DB_URL,username,password);
System.out.println("数据库链接成功");
} catch (SQLException e) {
System.out.println("数据库链接失败");
e.printStackTrace();
}
}
public void close() {
if(rSet != null) {
try {
rSet.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(pStatement != null) {
try {
pStatement.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if(connection != null) {
try {
connection.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public void addStudent(int id, String nameString, String genderString, String dobString, int batch) throws SQLException {
try {
connectDB();
String addsql = "insert into student values(?, ?, ?, ?, ?)";
pStatement = connection.prepareStatement(addsql);
pStatement.setInt(1, id);
pStatement.setString(2, nameString);
pStatement.setString(3, genderString);
pStatement.setString(4, dobString);
pStatement.setInt(5, batch);
pStatement.executeUpdate();
} finally {
close();
}
}
public void addManeger(String username, String password) throws SQLException {
try {
connectDB();
String addsql = "insert into m_user values(?, ?)";
pStatement = connection.prepareStatement(addsql);
pStatement.setString(1, username);
pStatement.setString(2, password);
pStatement.executeUpdate();
} finally {
close();
}
}
public void changeStudent(int id, String nameString, String genderString, String dobString, String batch) throws SQLException {
try {
connectDB();
String changesql = "update student set name = ?, gender = ?, dob = ?, batch = ? where id = ?";
pStatement = connection.prepareStatement(changesql);
pStatement.setString(1, nameString);
pStatement.setString(2, genderString);
pStatement.setString(3, dobString);
pStatement.setString(4, batch);
pStatement.setInt(5, id);
pStatement.executeUpdate();
} finally {
close();
}
}
public void deletestudent(int id) throws SQLException {
try {
connectDB();
String deleteString = "select * from student where id = ?";
pStatement = connection.prepareStatement(deleteString);
pStatement.setInt(1, id);
pStatement.execute();
} finally {
close();
}
}
public User userpassweord(String username) throws SQLException {
try {
connectDB();
String slelectusername = "select * from m_user where username = ?";
pStatement = connection.prepareStatement(slelectusername);
pStatement.setString(1, username);
rSet = pStatement.executeQuery();
if (rSet.next()) {
String usernameString = rSet.getString(1);
String passwordString = rSet.getString(2);
return new User(usernameString, passwordString);
}else {
return null;
}
} finally {
close();
}
}
public Student queryStudent(int id) throws SQLException {
try {
connectDB();
String querysql = "select * from student where id = ?";
pStatement = connection.prepareStatement(querysql);
pStatement.setInt(1, id);
rSet = pStatement.executeQuery();
if(rSet.next()) {
String nameString = rSet.getString(2);
String genderString = rSet.getString(3);
String dobString = rSet.getString(4);
int batchString = rSet.getInt(5);
return new Student(nameString, genderString, dobString, batchString);
} else {
return null;
}
} finally {
close();
}
}
}
SelectFrame
- 因为JDBC本来是写在一起的这里我单独写了一个文件,我就把它并在JDBC内容里面
package StudentManager;
import javax.swing.*;
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.sql.*;
public class SelectFrame extends JFrame {
public static final String JDBC_DRIVER = "com.mysql.cj.jdbc.Driver";
public static final String DB_URL = "jdbc:mysql://localhost:3306/java_sims?useSSL=false&allowPublicKeyRetrieval=true&serverTimezone=UTC";
public static final String username = "root";
public static final String password = "111111";
public SelectFrame() throws SQLException {
Connection connection=null;
connection = DriverManager.getConnection(DB_URL,username,password);
PreparedStatement preparedStatement=connection.prepareStatement("select *from student");
ResultSet res=preparedStatement.executeQuery();
res.last();
int row=res.getRow();
res.beforeFirst();
String arr[]= {"姓名","性别","出生日期","班级"};
String comm[][] = new String[row][4];
res.next();
for(int i=0;i<row;i++) {
comm[i][0]=res.getString("name");
comm[i][1]=res.getString("sex");
comm[i][2]=res.getString("birthday");
comm[i][3]=res.getString("gender");
res.next();
}
JTable jTable=new JTable(comm,arr);
jTable.setPreferredScrollableViewportSize(new Dimension(800,200));
JScrollPane jScrollPane=new JScrollPane(jTable);
add(jScrollPane,BorderLayout.CENTER);
setVisible(true);
validate();
this.setBounds(550,400,800,200);
pack();
connection.close();
this.addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
int choice = JOptionPane.showConfirmDialog(null, "关闭该窗口?","学生管理", JOptionPane.YES_NO_OPTION);
if (choice == JOptionPane.YES_OPTION) {
dispose();
} else {
try {
new SelectFrame();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
}
});
}
}
Swing
Start
这个文件是启动文件哈,启动程序的时候用这个启动
package StudentManager;
public class Start {
public static void main(String[] args) {
LoginStar l = new LoginStar();
}
}
LoginStar
package StudentManager;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class LoginStar extends JFrame {
JLabel bgimg;
JLabel username;
JLabel password;
JTextField usernametext;
JPasswordField passwordtext;
JButton login;
JLabel register;
final int WIDTH = 300;
final int HEIGHT = 300;
JPanel p;
Regevent regeveent;
public LoginStar() {
init();
setResizable(false);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
validate();
setVisible(true);
}
private void init() {
p = new JPanel();
p.setBounds(0, 0, WIDTH, HEIGHT);
p.setSize(300,300);
p.setLayout(null);
Toolkit kit = Toolkit.getDefaultToolkit();
Dimension screenSize = kit.getScreenSize();
int width = screenSize.width;
int height = screenSize.height;
int x = (width - WIDTH) / 2;
int y = (height - HEIGHT) / 2;
this.setBounds(x, y, WIDTH, HEIGHT);
this.setTitle("登录");
this.add(p);
username = new JLabel("账号");
password = new JLabel("密码");
login = new JButton("登录");
register = new JLabel("注册");
usernametext = new JTextField(20);
passwordtext = new JPasswordField(20);
passwordtext.setEchoChar('*');
passwordtext.setBounds(125, 120, 120, 25);
usernametext.setBounds(125, 90, 120, 25);
password.setBounds(60, 120, 60, 25);
username.setBounds(60, 90, 60, 25);
login.setBounds(90,180,60,25);
register.setBounds(10,200,100,100);
p.add(username);
p.add(password);
p.add(usernametext);
p.add(passwordtext);
p.add(login);
p.add(register);
login.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String strusername = usernametext.getText();
String strpassword = new String(passwordtext.getPassword());
SQLHelp sqlHelp = new SQLHelp();
try {
User users = sqlHelp.userpassweord(strusername);
String username = users.getUsername();
String password = users.getPassword();
System.out.println(username);
System.out.println(password);
if (strusername.equals(username) && strpassword.equals(password)){
JOptionPane.showMessageDialog(null,"登录成功","登陆成功提示窗口",JOptionPane.INFORMATION_MESSAGE);
MainFrame main = new MainFrame();
main.setVisible(true);
}else {
JOptionPane.showMessageDialog(null,"登录失败,若没有账号点击左下角注册","登录失败提示窗口",JOptionPane.INFORMATION_MESSAGE);
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
});
allEvent();
}
void allEvent(){
regeveent = new Regevent();
register.addMouseListener(regeveent);
}
}
Register
package StudentManager;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class Register extends JFrame {
JLabel username;
JLabel password;
JLabel passwordAgain;
JTextField usernameFile;
JPasswordField passwordFile;
JPasswordField PasswordFileAgain;
JButton sign;
JPanel p;
JPanel p1;
final int WIDTH = 410;
final int HEIGHT = 610;
public Register() {
init();
setResizable(false);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
validate();
setVisible(true);
Toolkit kit = Toolkit.getDefaultToolkit();
Dimension screenSize = kit.getScreenSize();
int width = screenSize.width;
int height = screenSize.height;
int x = (width - WIDTH) / 2;
int y = (height - HEIGHT) / 2;
setBounds(x, y, WIDTH, HEIGHT);
setTitle("账号注册");
}
void init() {
p = new JPanel();
p.setLayout(null);
p.setVisible(true);
p1 = new JPanel();
p1.setVisible(true);
p1.setLayout(null);
p1.setBounds(0, 0, 400, 600);
username = new JLabel("账号:");
password = new JLabel("密码:");
passwordAgain = new JLabel("确认密码:");
usernameFile = new JTextField(20);
passwordFile = new JPasswordField(20);
PasswordFileAgain = new JPasswordField(20);
passwordFile.setEchoChar('*');
PasswordFileAgain.setEchoChar('*');
sign = new JButton("注册");
sign.setBounds(160, 300, 100, 50);
username.setBounds(130, 150, 40, 20);
password.setBounds(130, 200, 40, 20);
passwordAgain.setBounds(130, 250, 120, 20);
usernameFile.setBounds(200, 150, 100, 20);
passwordFile.setBounds(200, 200, 100, 20);
PasswordFileAgain.setBounds(200, 250, 100, 20);
p1.add(username);
p1.add(password);
p1.add(passwordAgain);
p1.add(usernameFile);
p1.add(passwordFile);
p1.add(PasswordFileAgain);
p1.add(sign);
p.add(p1);
this.add(p);
sign.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String strusername = usernameFile.getText();
String strpassword = new String(passwordFile.getPassword());
String strpasswordAgain = new String(PasswordFileAgain.getPassword());
SQLHelp sqlHelp = new SQLHelp();
try {
User users = sqlHelp.userpassweord(strusername);
if (users == null) {
if (strpassword.equals(strpasswordAgain)) {
System.out.println("注册成功");
String username = strusername;
String password = strpassword;
sqlHelp.addManeger(username,password);
JOptionPane.showMessageDialog(null, "注册成功", "点击确定后返回登录界面", JOptionPane.INFORMATION_MESSAGE);
} else {
System.out.println("注册失败");
JOptionPane.showMessageDialog(null, "注册失败,请检查两次密码是否相同", "登录失败提示窗口", JOptionPane.INFORMATION_MESSAGE);
}
}else{
System.out.println("已有账号无法注册");
JOptionPane.showMessageDialog(null,"注册失败了,已拥有该账号,请检查密码是否输入错误,目前不支持找回密码","账号已有窗口",JOptionPane.INFORMATION_MESSAGE);
}
} catch (SQLException ex) {
ex.printStackTrace();
}
}
});
}
public static void main(String[] args) {
new Register();
}
}
MainFrame
package StudentManager;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class MainFrame extends JFrame {
private JPanel contentPane;
public MainFrame() {
setResizable(false);
setTitle("学生管理系统");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 450, 500);
setLocationRelativeTo(null);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(null);
JLabel lblNewLabel = new JLabel("学生管理系统");
lblNewLabel.setForeground(Color.RED);
lblNewLabel.setFont(new Font("宋体", Font.BOLD, 25));
lblNewLabel.setBounds(140, 10, 163, 44);
contentPane.add(lblNewLabel);
JButton addButton = new JButton("添加学生");
addButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new AddFrame().setVisible(true);
}
});
addButton.setFont(new Font("宋体", Font.PLAIN, 18));
addButton.setBounds(167, 64, 114, 37);
contentPane.add(addButton);
JButton changeButton = new JButton("修改信息");
changeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new ChangeFrame().setVisible(true);
}
});
changeButton.setFont(new Font("宋体", Font.PLAIN, 18));
changeButton.setBounds(167, 121, 114, 37);
contentPane.add(changeButton);
JButton deleteButton = new JButton("删除学生");
deleteButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new DeleteFrame().setVisible(true);
}
});
deleteButton.setFont(new Font("宋体", Font.PLAIN, 18));
deleteButton.setBounds(167, 180, 114, 37);
contentPane.add(deleteButton);
JButton queryButton = new JButton("查询信息");
queryButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new QueryFrame().setVisible(true);
}
});
queryButton.setFont(new Font("宋体", Font.PLAIN, 18));
queryButton.setBounds(167, 240, 114, 37);
contentPane.add(queryButton);
JButton selectButton = new JButton("查询所有学生记录");
selectButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
try {
SelectFrame s = new SelectFrame();
} catch (SQLException ex) {
ex.printStackTrace();
}
}
});
selectButton.setBounds(134,300,180,37);
selectButton.setFont(new Font("宋体", Font.PLAIN, 18));
contentPane.add(selectButton);
}
}
QueryFrame
package StudentManager;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class QueryFrame extends JFrame {
private JPanel contentPane;
private JTextField idField;
private JTextField nameField;
private JTextField genderField;
private JTextField dobField;
private JTextField batchField;
private JTextField searchField;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
QueryFrame frame = new QueryFrame();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public QueryFrame() {
setResizable(false);
setTitle("查询信息");
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setBounds(100, 100, 450, 470);
setLocationRelativeTo(null);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(new BoxLayout(contentPane, BoxLayout.Y_AXIS));
JPanel panel = new JPanel();
contentPane.add(panel);
panel.setLayout(null);
JLabel lblNewLabel = new JLabel("请输入要查找的学生的学号:");
lblNewLabel.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel.setBounds(71, 0, 208, 29);
panel.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("学号:");
lblNewLabel_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1.setBounds(71, 105, 50, 30);
panel.add(lblNewLabel_1);
idField = new JTextField();
idField.setEditable(false);
idField.setBounds(143, 99, 240, 45);
panel.add(idField);
idField.setColumns(10);
JLabel lblNewLabel_1_1 = new JLabel("姓名:");
lblNewLabel_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1.setBounds(71, 160, 50, 30);
panel.add(lblNewLabel_1_1);
nameField = new JTextField();
nameField.setColumns(10);
nameField.setBounds(143, 154, 240, 45);
panel.add(nameField);
JLabel lblNewLabel_1_1_1 = new JLabel("性别:");
lblNewLabel_1_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1.setBounds(71, 215, 50, 30);
panel.add(lblNewLabel_1_1_1);
genderField = new JTextField();
genderField.setColumns(10);
genderField.setBounds(143, 209, 240, 45);
panel.add(genderField);
JLabel lblNewLabel_1_1_1_1 = new JLabel("出生日期:");
lblNewLabel_1_1_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1_1.setBounds(41, 270, 80, 30);
panel.add(lblNewLabel_1_1_1_1);
dobField = new JTextField();
dobField.setColumns(10);
dobField.setBounds(143, 264, 240, 45);
panel.add(dobField);
JLabel lblNewLabel_1_1_1_2 = new JLabel("班级:");
lblNewLabel_1_1_1_2.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1_2.setBounds(71, 325, 50, 30);
panel.add(lblNewLabel_1_1_1_2);
batchField = new JTextField();
batchField.setColumns(10);
batchField.setBounds(143, 319, 240, 45);
panel.add(batchField);
searchField = new JTextField();
searchField.setBounds(71, 39, 208, 45);
panel.add(searchField);
searchField.setColumns(10);
JButton searchButton = new JButton("查找");
searchButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int id = Integer.parseInt(searchField.getText());
try {
SQLHelp sqlHelp = new SQLHelp();
Student student = sqlHelp.queryStudent(id);
if(student != null) {
idField.setText(String.valueOf(id));
nameField.setText(student.getName());
genderField.setText(student.getGender());
dobField.setText(student.getDob());
batchField.setText(String.valueOf(student.getBatch()));
} else {
JOptionPane.showMessageDialog(QueryFrame.this, "无此学生");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
});
searchButton.setFont(new Font("宋体", Font.PLAIN, 18));
searchButton.setBounds(289, 42, 97, 39);
panel.add(searchButton);
}
}
DeleteFrame
package StudentManager;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class QueryFrame extends JFrame {
private JPanel contentPane;
private JTextField idField;
private JTextField nameField;
private JTextField genderField;
private JTextField dobField;
private JTextField batchField;
private JTextField searchField;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
QueryFrame frame = new QueryFrame();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public QueryFrame() {
setResizable(false);
setTitle("查询信息");
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setBounds(100, 100, 450, 470);
setLocationRelativeTo(null);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(new BoxLayout(contentPane, BoxLayout.Y_AXIS));
JPanel panel = new JPanel();
contentPane.add(panel);
panel.setLayout(null);
JLabel lblNewLabel = new JLabel("请输入要查找的学生的学号:");
lblNewLabel.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel.setBounds(71, 0, 208, 29);
panel.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("学号:");
lblNewLabel_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1.setBounds(71, 105, 50, 30);
panel.add(lblNewLabel_1);
idField = new JTextField();
idField.setEditable(false);
idField.setBounds(143, 99, 240, 45);
panel.add(idField);
idField.setColumns(10);
JLabel lblNewLabel_1_1 = new JLabel("姓名:");
lblNewLabel_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1.setBounds(71, 160, 50, 30);
panel.add(lblNewLabel_1_1);
nameField = new JTextField();
nameField.setColumns(10);
nameField.setBounds(143, 154, 240, 45);
panel.add(nameField);
JLabel lblNewLabel_1_1_1 = new JLabel("性别:");
lblNewLabel_1_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1.setBounds(71, 215, 50, 30);
panel.add(lblNewLabel_1_1_1);
genderField = new JTextField();
genderField.setColumns(10);
genderField.setBounds(143, 209, 240, 45);
panel.add(genderField);
JLabel lblNewLabel_1_1_1_1 = new JLabel("出生日期:");
lblNewLabel_1_1_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1_1.setBounds(41, 270, 80, 30);
panel.add(lblNewLabel_1_1_1_1);
dobField = new JTextField();
dobField.setColumns(10);
dobField.setBounds(143, 264, 240, 45);
panel.add(dobField);
JLabel lblNewLabel_1_1_1_2 = new JLabel("班级:");
lblNewLabel_1_1_1_2.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1_2.setBounds(71, 325, 50, 30);
panel.add(lblNewLabel_1_1_1_2);
batchField = new JTextField();
batchField.setColumns(10);
batchField.setBounds(143, 319, 240, 45);
panel.add(batchField);
searchField = new JTextField();
searchField.setBounds(71, 39, 208, 45);
panel.add(searchField);
searchField.setColumns(10);
JButton searchButton = new JButton("查找");
searchButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int id = Integer.parseInt(searchField.getText());
try {
SQLHelp sqlHelp = new SQLHelp();
Student student = sqlHelp.queryStudent(id);
if(student != null) {
idField.setText(String.valueOf(id));
nameField.setText(student.getName());
genderField.setText(student.getGender());
dobField.setText(student.getDob());
batchField.setText(String.valueOf(student.getBatch()));
} else {
JOptionPane.showMessageDialog(QueryFrame.this, "无此学生");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
});
searchButton.setFont(new Font("宋体", Font.PLAIN, 18));
searchButton.setBounds(289, 42, 97, 39);
panel.add(searchButton);
}
}
ChangeFrame
package StudentManager;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class ChangeFrame extends JFrame {
private JPanel contentPane;
private JTextField idField;
private JTextField nameField;
private JTextField genderField;
private JTextField dobField;
private JTextField batchField;
private JTextField searchField;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
ChangeFrame frame = new ChangeFrame();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public ChangeFrame() {
setResizable(false);
setTitle("修改信息");
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setBounds(100, 100, 450, 470);
setLocationRelativeTo(null);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(new BoxLayout(contentPane, BoxLayout.Y_AXIS));
JPanel panel = new JPanel();
contentPane.add(panel);
panel.setLayout(null);
JLabel lblNewLabel = new JLabel("请输入要修改的学生的学号:");
lblNewLabel.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel.setBounds(71, 0, 208, 29);
panel.add(lblNewLabel);
JLabel lblNewLabel_1 = new JLabel("学号:");
lblNewLabel_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1.setBounds(71, 105, 50, 30);
panel.add(lblNewLabel_1);
idField = new JTextField();
idField.setEditable(false);
idField.setBounds(143, 99, 240, 45);
panel.add(idField);
idField.setColumns(10);
JLabel lblNewLabel_1_1 = new JLabel("姓名:");
lblNewLabel_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1.setBounds(71, 160, 50, 30);
panel.add(lblNewLabel_1_1);
nameField = new JTextField();
nameField.setColumns(10);
nameField.setBounds(143, 154, 240, 45);
panel.add(nameField);
JLabel lblNewLabel_1_1_1 = new JLabel("性别:");
lblNewLabel_1_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1.setBounds(71, 215, 50, 30);
panel.add(lblNewLabel_1_1_1);
genderField = new JTextField();
genderField.setColumns(10);
genderField.setBounds(143, 209, 240, 45);
panel.add(genderField);
JLabel lblNewLabel_1_1_1_1 = new JLabel("出生日期:");
lblNewLabel_1_1_1_1.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1_1.setBounds(41, 270, 80, 30);
panel.add(lblNewLabel_1_1_1_1);
dobField = new JTextField();
dobField.setColumns(10);
dobField.setBounds(143, 264, 240, 45);
panel.add(dobField);
JLabel lblNewLabel_1_1_1_2 = new JLabel("班级:");
lblNewLabel_1_1_1_2.setFont(new Font("宋体", Font.PLAIN, 16));
lblNewLabel_1_1_1_2.setBounds(71, 325, 50, 30);
panel.add(lblNewLabel_1_1_1_2);
batchField = new JTextField();
batchField.setColumns(10);
batchField.setBounds(143, 319, 240, 45);
panel.add(batchField);
JButton changeButton = new JButton("修改");
changeButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent arg0) {
int id = Integer.parseInt(searchField.getText());
String nameString = nameField.getText();
String gendeString = genderField.getText();
String dobString = dobField.getText();
String batch = batchField.getText();
SQLHelp sqlHelp = new SQLHelp();
try {
sqlHelp.changeStudent(id, nameString, gendeString, dobString, batch);
JOptionPane.showMessageDialog(ChangeFrame.this, "修改成功!");
} catch (SQLException e) {
JOptionPane.showMessageDialog(ChangeFrame.this, "修改失败!");
e.printStackTrace();
}
}
});
changeButton.setFont(new Font("宋体", Font.PLAIN, 18));
changeButton.setBounds(182, 389, 97, 33);
panel.add(changeButton);
searchField = new JTextField();
searchField.setBounds(71, 39, 208, 45);
panel.add(searchField);
searchField.setColumns(10);
JButton searchButton = new JButton("查找");
searchButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e){
int id = Integer.parseInt(searchField.getText());
try {
SQLHelp sqlHelp = new SQLHelp();
Student student = sqlHelp.queryStudent(id);
if(student != null) {
idField.setText(String.valueOf(id));
nameField.setText(student.getName());
genderField.setText(student.getGender());
dobField.setText(student.getDob());
batchField.setText(String.valueOf(student.getBatch()));
} else {
JOptionPane.showMessageDialog(ChangeFrame.this, "无此用户");
}
} catch (SQLException e1) {
e1.printStackTrace();
}
}
});
searchButton.setFont(new Font("宋体", Font.PLAIN, 18));
searchButton.setBounds(289, 42, 97, 39);
panel.add(searchButton);
}
}
AddFrame
package StudentManager;
import javax.swing.*;
import javax.swing.border.EmptyBorder;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.sql.SQLException;
public class AddFrame extends JFrame {
private JPanel contentPane;
private JTextField idField;
private JTextField nameField;
private JTextField genderField;
private JTextField dobField;
private JTextField batchField;
public static void main(String[] args) {
EventQueue.invokeLater(new Runnable() {
public void run() {
try {
AddFrame frame = new AddFrame();
frame.setVisible(true);
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
public AddFrame() {
setResizable(false);
setTitle("添加学生");
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setBounds(100, 100, 450, 470);
setLocationRelativeTo(null);
contentPane = new JPanel();
contentPane.setBorder(new EmptyBorder(5, 5, 5, 5));
setContentPane(contentPane);
contentPane.setLayout(new BoxLayout(contentPane, BoxLayout.Y_AXIS));
JPanel panel = new JPanel();
contentPane.add(panel);
panel.setLayout(null);
JLabel TitleLabel = new JLabel("请输入新学生的信息:");
TitleLabel.setFont(new Font("宋体", Font.BOLD, 20));
TitleLabel.setBounds(71, 34, 220, 45);
panel.add(TitleLabel);
JLabel idLabel = new JLabel("学号:");
idLabel.setFont(new Font("宋体", Font.PLAIN, 16));
idLabel.setBounds(71, 105, 50, 30);
panel.add(idLabel);
idField = new JTextField();
idField.setBounds(143, 99, 240, 45);
panel.add(idField);
idField.setColumns(10);
JLabel nameLabel = new JLabel("姓名:");
nameLabel.setFont(new Font("宋体", Font.PLAIN, 16));
nameLabel.setBounds(71, 160, 50, 30);
panel.add(nameLabel);
nameField = new JTextField();
nameField.setColumns(10);
nameField.setBounds(143, 154, 240, 45);
panel.add(nameField);
JLabel genderLabel = new JLabel("性别:");
genderLabel.setFont(new Font("宋体", Font.PLAIN, 16));
genderLabel.setBounds(71, 215, 50, 30);
panel.add(genderLabel);
genderField = new JTextField();
genderField.setColumns(10);
genderField.setBounds(143, 209, 240, 45);
panel.add(genderField);
JLabel dobLabel = new JLabel("出生日期:");
dobLabel.setFont(new Font("宋体", Font.PLAIN, 16));
dobLabel.setBounds(41, 270, 80, 30);
panel.add(dobLabel);
dobField = new JTextField();
dobField.setColumns(10);
dobField.setBounds(143, 264, 240, 45);
panel.add(dobField);
JLabel batchLabel = new JLabel("班级:");
batchLabel.setFont(new Font("宋体", Font.PLAIN, 16));
batchLabel.setBounds(71, 325, 50, 30);
panel.add(batchLabel);
batchField = new JTextField();
batchField.setColumns(10);
batchField.setBounds(143, 319, 240, 45);
panel.add(batchField);
JButton addButton = new JButton("添加");
addButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int id = Integer.parseInt(idField.getText());
String nameString = nameField.getText();
String genderString = genderField.getText();
String dobfieldString = dobField.getText();
int batch = Integer.parseInt(batchField.getText());
System.out.println(id + "\t" + nameString + "\t" + genderString + "\t" + dobfieldString + "\t" + batch);
SQLHelp sqlHelp = new SQLHelp();
try {
sqlHelp.addStudent(id, nameString, genderString, dobfieldString, batch);
JOptionPane.showMessageDialog(AddFrame.this, "添加成功!");
} catch (SQLException e1) {
if(e1.getSQLState().equals("23000")) {
JOptionPane.showMessageDialog(AddFrame.this, "添加失败!该学生已存在");
}
e1.printStackTrace();
}
}
});
addButton.setFont(new Font("宋体", Font.PLAIN, 18));
addButton.setBounds(182, 389, 97, 33);
panel.add(addButton);
}
}
我在这设置一个标题
作者写这边博客也不容易,对你有帮助就来个一键三连咋样? 对了有个不懂的地方可以留言或者私信,我看见了会回你的~ 芭芭拉,闪耀登场~
|