项目结构
1.实体model模块 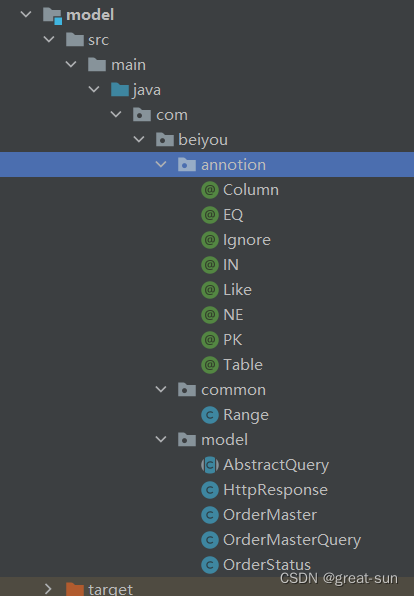 2.功能模块sb-mybatis 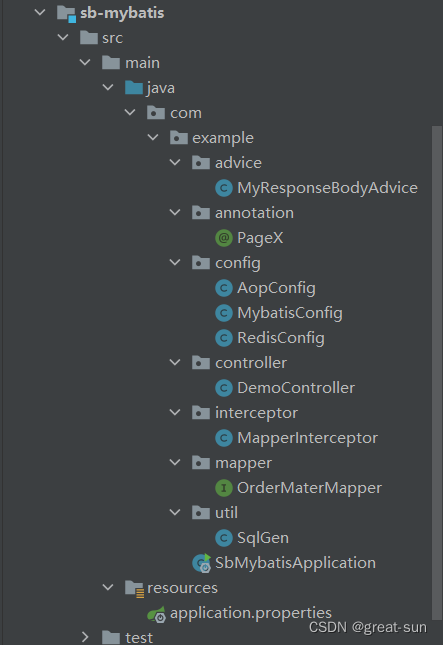 实际操作 1.引入依赖
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper</artifactId>
<version>5.1.10</version>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
1.自定义注解分页PageX
package com.example.annotation;
import java.lang.annotation.*;
@Documented
@Target({ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
public @interface PageX {
}
2.自定义封装SQL语句注解 Column列
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface Column {
String value() default "";
}
EQ等于
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface Table {
String value() default "";
}
NQ 不等于
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface NE {
String value() default "";
}
IN 条件in
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface IN {
String value() default "";
}
Like 条件like
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface Like {
String value() default "";
}
PK 主键
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface PK {
String value() default "";
boolean autoIncrement() default true;
}
Table 表名
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ElementType.TYPE})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface Table {
String value() default "";
}
Ignore 忽略不封装
package com.beiyou.annotion;
import java.lang.annotation.*;
@Target({ElementType.FIELD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface Ignore {
String value() default "";
}
3.实体 Range查询范围的类,SQL语句封装
package com.beiyou.common;
import java.io.Serializable;
public class Range<T> implements Serializable {
private T left;
private boolean leftEQ;
private T right;
private boolean rightEQ;
public Range() {
}
public Range(T left, boolean leftEQ, T right, boolean rightEQ) {
this.left = left;
this.leftEQ = leftEQ;
this.right = right;
this.rightEQ = rightEQ;
}
public T getLeft() {
return left;
}
public void setLeft(T left) {
this.left = left;
}
public boolean isLeftEQ() {
return leftEQ;
}
public void setLeftEQ(boolean leftEQ) {
this.leftEQ = leftEQ;
}
public T getRight() {
return right;
}
public void setRight(T right) {
this.right = right;
}
public boolean isRightEQ() {
return rightEQ;
}
public void setRightEQ(boolean rightEQ) {
this.rightEQ = rightEQ;
}
public static <T> Range.RangeBuilder<T> builder() {
return new Range.RangeBuilder<T>();
}
public static class RangeBuilder<T> implements Serializable {
private T left;
private boolean leftEQ = true;
private T right;
private boolean rightEQ = true;
RangeBuilder() {
}
public Range.RangeBuilder<T> left(T left) {
this.left = left;
return this;
}
public Range.RangeBuilder<T> leftEQ(boolean leftEQ) {
this.leftEQ = leftEQ;
return this;
}
public Range.RangeBuilder<T> right(T right) {
this.right = right;
return this;
}
public Range.RangeBuilder<T> rightEQ(boolean rightEQ) {
this.rightEQ = rightEQ;
return this;
}
public Range<T> build() {
return new Range<T>(this.left, this.leftEQ, this.right, this.rightEQ);
}
}
}
HttpResponse统一返回值
package com.beiyou.model;
import lombok.Data;
import java.io.Serializable;
@Data
public class HttpResponse<T> implements Serializable {
private int code;
private String message;
private T data;
}
测试分页的实体
package com.beiyou.model;
import com.beiyou.annotion.*;
import com.beiyou.common.Range;
import lombok.Builder;
import lombok.Data;
@Data
@Table("orderMaster")
@Builder
public class OrderMasterQuery extends AbstractQuery {
@EQ
private Long id;
@IN
@Column("id")
private Long[] ids;
@Like
private String receiver;
@NE
private String phoneNumber;
@EQ
@Column("phoneNumber")
private String phoneNumberEQ;
@Column("id")
private Range<Long> idRange;
@Ignore
private String sourceFrom;
}
分页当前页,当前页数量
package com.beiyou.model;
import java.io.Serializable;
public abstract class AbstractQuery implements Serializable {
private Integer pageIndex;
private Integer pageSize;
private String orderByBlock;
public Integer getPageIndex() {
return pageIndex;
}
public void setPageIndex(Integer pageIndex) {
this.pageIndex = pageIndex;
}
public Integer getPageSize() {
return pageSize;
}
public void setPageSize(Integer pageSize) {
this.pageSize = pageSize;
}
public String getOrderByBlock() {
return orderByBlock;
}
public void setOrderByBlock(String orderByBlock) {
this.orderByBlock = orderByBlock;
}
}
4.配置分页插件
package com.example.config;
import com.github.pagehelper.PageInterceptor;
import org.apache.ibatis.session.SqlSessionFactory;
import org.mybatis.spring.SqlSessionFactoryBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.DataSource;
@Configuration
public class MybatisConfig {
@Bean
public SqlSessionFactory getSqlSessionFactory(DataSource ds) throws Exception {
SqlSessionFactoryBean factory = new SqlSessionFactoryBean();
factory.setDataSource(ds);
factory.setPlugins(new PageInterceptor());
SqlSessionFactory object = factory.getObject();
return object;
}
}
5.配置redis序列化,解决乱码
package com.example.config;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
public class RedisConfig {
@Bean(name = "redisTemplate")
public RedisTemplate<Object, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<Object, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
Jackson2JsonRedisSerializer jacksonSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jacksonSerializer.setObjectMapper(om);
StringRedisSerializer stringRedisSerializer = new StringRedisSerializer();
template.setKeySerializer(stringRedisSerializer);
template.setValueSerializer(jacksonSerializer);
template.setHashKeySerializer(stringRedisSerializer);
template.setHashValueSerializer(jacksonSerializer);
template.afterPropertiesSet();
return template;
}
}
6.配置application.properties
#连接数据库
spring.datasource.url=jdbc:mysql://localhost:3303/mall?useUnicode=true&characterEncoding=UTF-8&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=@root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
logging.level.com.example=debug
#配置自定义注解
pointcut.property=@annotation(com.example.annotation.PageX)
#redis配置
#redis服务器地址
spring.redis.host=192.168.43.1
#端口号
spring.redis.port=6379
#redis服务器密码默认为空
#spring.redis.password=
#redis最大连接数
spring.redis.lettuce.pool.max-active=16
#连接池最大阻塞等待时间,(使用负数表示没有限制)
spring.redis.lettuce.pool.max-wait=-1
#连接池的最大空闲时间,默认为8
spring.redis.lettuce.pool.max-idle=8
#连接池的最小空闲时间,默认为8
spring.redis.lettuce.pool.min-idle=0
#连接超时时间
spring.redis.timeout=30000
7.编写分页拦截器
package com.example.interceptor;
import cn.hutool.core.util.ObjectUtil;
import cn.hutool.json.JSONUtil;
import com.beiyou.model.AbstractQuery;
import com.github.pagehelper.PageHelper;
import lombok.extern.slf4j.Slf4j;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import java.lang.reflect.Method;
@Slf4j
public class MapperInterceptor implements MethodInterceptor {
@Override
public Object invoke(MethodInvocation invocation) throws Throwable {
Method method = invocation.getMethod();
Object[] arguments = invocation.getArguments();
Object arg0 = arguments[0];
if (arg0 instanceof AbstractQuery) {
AbstractQuery query = (AbstractQuery) arg0;
Integer pageIndex = query.getPageIndex();
Integer pageSize = query.getPageSize();
if (ObjectUtil.isNotEmpty(pageIndex) && ObjectUtil.isNotEmpty(pageSize)) {
PageHelper.startPage(pageIndex, pageSize);
}
}
Object value = invocation.proceed();
log.info("method:{},args:{},value:{}", method.getName(), JSONUtil.toJsonStr(arguments), value);
return value;
}
}
8.注册拦截器
package com.example.config;
import com.example.interceptor.MapperInterceptor;
import org.springframework.aop.aspectj.AspectJExpressionPointcutAdvisor;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class AopConfig {
@Value("${pointcut.property}")
private String expression;
@Bean
public AspectJExpressionPointcutAdvisor aspectJExpressionPointcutAdvisor() {
AspectJExpressionPointcutAdvisor advisor = new AspectJExpressionPointcutAdvisor();
advisor.setExpression(expression);
advisor.setAdvice(new MapperInterceptor());
return advisor;
}
}
9.集成分页做统一返回值
package com.example.advice;
import cn.hutool.core.lang.Dict;
import cn.hutool.core.util.StrUtil;
import cn.hutool.json.JSONUtil;
import com.beiyou.model.HttpResponse;
import com.github.pagehelper.Page;
import org.springframework.core.MethodParameter;
import org.springframework.http.MediaType;
import org.springframework.http.converter.StringHttpMessageConverter;
import org.springframework.http.server.ServerHttpRequest;
import org.springframework.http.server.ServerHttpResponse;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
import org.springframework.web.servlet.mvc.method.annotation.ResponseBodyAdvice;
import javax.servlet.http.HttpServletRequest;
@RestControllerAdvice(basePackages = {"com.example.controller"})
public class MyResponseBodyAdvice implements ResponseBodyAdvice {
@Override
public boolean supports(MethodParameter returnType, Class converterType) {
return true;
}
@ExceptionHandler(value = Exception.class)
public Object defaultErrorHandler(HttpServletRequest req, Exception ex) {
HttpResponse<Object> httpResponse = new HttpResponse<>();
httpResponse.setCode(1);
httpResponse.setMessage(ex.getMessage());
return httpResponse;
}
@Override
public Object beforeBodyWrite(Object body,
MethodParameter returnType,
MediaType selectedContentType,
Class selectedConverterType,
ServerHttpRequest request,
ServerHttpResponse response) {
HttpResponse<Object> httpResponse = new HttpResponse<>();
String message = StrUtil.EMPTY;
httpResponse.setCode(0);
httpResponse.setMessage(message);
if (body instanceof Page) {
Page page = (Page) body;
int pages = page.getPages();
long total = page.getTotal();
Dict dic = Dict.create()
.set("total", total)
.set("pages", pages)
.set("items", page);
httpResponse.setData(dic);
} else {
httpResponse.setData(body);
}
if (selectedConverterType == StringHttpMessageConverter.class) {
return JSONUtil.toJsonStr(httpResponse);
} else {
return httpResponse;
}
}
}
10.封装SQL语句
package com.example.util;
import cn.hutool.core.annotation.AnnotationUtil;
import cn.hutool.core.lang.Assert;
import cn.hutool.core.util.ClassUtil;
import cn.hutool.core.util.ReflectUtil;
import cn.hutool.core.util.StrUtil;
import com.beiyou.annotion.*;
import com.beiyou.common.Range;
import com.beiyou.model.AbstractQuery;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.util.HashMap;
import java.util.HashSet;
public class SqlGen {
public static final String select = "select";
public static final String insert = "insert";
public static final String update = "update";
public static HashMap<String, String> selectMap = new HashMap<>();
private static final HashSet<String> pageFileds = new HashSet<String>() {
{
add("pageIndex");
add("pageSize");
add("orderByBlock");
}
};
public String update(Object model) {
Class<?> modelClass = model.getClass();
StringBuilder sb = new StringBuilder();
sb.append(" <script>");
sb.append(" update ");
String tableName = AnnotationUtil.getAnnotationValue(modelClass, Table.class, "value");
Assert.notEmpty(tableName, "{} 表名未设置", modelClass.getName());
sb.append(tableName);
sb.append(" set ");
String idString = "";
Field[] fields = ReflectUtil.getFields(modelClass);
for (Field field : fields) {
Ignore ignore = AnnotationUtil.getAnnotation(field, Ignore.class);
if (ignore != null) {
continue;
}
String fieldName = field.getName();
String cloumnName = fieldName;
String cloumnName1 = AnnotationUtil.getAnnotationValue(field, Column.class, "value");
if (StrUtil.isNotEmpty(cloumnName1)) {
cloumnName = cloumnName1;
}
PK pk = AnnotationUtil.getAnnotation(field, PK.class);
if (pk != null) {
idString = StrUtil.format(" {} = #{{}}", cloumnName, fieldName);
continue;
}
sb.append(StrUtil.format(" {} = #{{}},", cloumnName, fieldName));
}
sb.deleteCharAt(sb.toString().length() - 1);
sb.append(" where ");
sb.append(idString);
sb.append("</script>");
System.out.println(sb.toString());
return sb.toString().trim();
}
public String insert(Object model) {
Class<?> modelClass = model.getClass();
StringBuilder sb = new StringBuilder();
sb.append(" <script>");
sb.append(" insert into ");
String tableName = AnnotationUtil.getAnnotationValue(modelClass, Table.class, "value");
Assert.notEmpty(tableName, "{}没有设置数据库对应的表名", modelClass);
sb.append(tableName);
sb.append(" ( ");
Field[] fields = ReflectUtil.getFields(modelClass);
for (Field field : fields) {
Ignore ignore = AnnotationUtil.getAnnotation(field, Ignore.class);
if (ignore != null) {
continue;
}
PK pk = AnnotationUtil.getAnnotation(field, PK.class);
if (pk != null && pk.autoIncrement() == true) {
continue;
}
String fieldName = field.getName();
String columnName = fieldName;
Column column = AnnotationUtil.getAnnotation(field, Column.class);
if (column != null) {
columnName = column.value();
}
sb.append(columnName);
sb.append(",");
}
sb.deleteCharAt(sb.toString().length() - 1);
sb.append(")");
sb.append(" values ");
sb.append(" ( ");
fields = ReflectUtil.getFields(modelClass);
for (Field field : fields) {
Ignore ignore = AnnotationUtil.getAnnotation(field, Ignore.class);
if (ignore != null) {
continue;
}
PK pk = AnnotationUtil.getAnnotation(field, PK.class);
if (pk != null && pk.autoIncrement() == true) {
continue;
}
String filedName = field.getName();
sb.append(StrUtil.format("#{{}}", filedName));
sb.append(",");
}
sb.deleteCharAt(sb.toString().length() - 1);
sb.append(" ) ");
sb.append(" </script> ");
System.out.println(sb.toString());
return sb.toString().trim();
}
public String select(AbstractQuery query) {
String queryClassName = query.getClass().getName();
if (selectMap.containsKey(queryClassName)) {
return selectMap.get(queryClassName);
}
String modelClassName = StrUtil.sub(queryClassName, 0, queryClassName.length() - 5);
String tableName = "";
Class<?> modelClass = ClassUtil.loadClass(modelClassName);
Table table = AnnotationUtil.getAnnotation(modelClass, Table.class);
if (table != null) {
tableName = table.value();
} else {
table = AnnotationUtil.getAnnotation(query.getClass(), Table.class);
if (table != null) {
tableName = table.value();
}
}
Assert.notNull(tableName, "{} 没有设置具体表名", modelClassName);
StringBuilder sb = new StringBuilder();
sb.append(" <script> ");
sb.append(" select ");
Field[] fields0 = ReflectUtil.getFields(modelClass);
for (Field field : fields0) {
Ignore ignore = AnnotationUtil.getAnnotation(field, Ignore.class);
if (ignore != null) {
continue;
}
String fieldName = field.getName();
String columnName = fieldName;
Column column = AnnotationUtil.getAnnotation(field, Column.class);
if (column != null) {
columnName = column.value();
}
if (fieldName.equalsIgnoreCase(columnName)) {
sb.append(StrUtil.format(" {},", columnName));
} else {
sb.append(StrUtil.format("{} as {},", columnName, fieldName));
}
}
sb.deleteCharAt(sb.toString().length() - 1);
sb.append(" from ");
sb.append(tableName);
sb.append(" <where> ");
sb.append(" 1 = 1");
Field[] fields = ReflectUtil.getFields(query.getClass());
for (Field field : fields) {
if (pageFileds.contains(field.getName())) {
continue;
}
Ignore ignore = AnnotationUtil.getAnnotation(field, Ignore.class);
if (ignore != null) {
continue;
}
String filedName = field.getName();
String columnName = filedName;
Column column = AnnotationUtil.getAnnotation(field, Column.class);
if (column != null) {
columnName = column.value();
}
Annotation[] anns = AnnotationUtil.getAnnotations(field, true);
if (anns.length == 0 || (anns.length == 1 && column != null)) {
if (!ClassUtil.isAssignable(Range.class, field.getType())) {
sb.append(String.format("\n<if test=\"%s != null\">" +
" \n <![CDATA[ AND %s = #{%s} ]]>\n" +
" \n</if>", filedName, columnName, filedName));
continue;
}
}
EQ eq = AnnotationUtil.getAnnotation(field, com.beiyou.annotion.EQ.class);
if (eq != null) {
if (eq != null) {
sb.append(String.format("\n<if test=\"%s != null\">" +
" \n <![CDATA[ AND %s = #{%s} ]]>\n" +
" \n</if>", filedName, columnName, filedName));
}
}
IN in = AnnotationUtil.getAnnotation(field, com.beiyou.annotion.IN.class);
if (in != null) {
sb.append(StrUtil.format(" <if test='{} != null and {}.length > 0 '> \n", filedName, filedName));
sb.append(StrUtil.format("and {} in \n", columnName));
sb.append(StrUtil.format(" <foreach item='item' collection='{}' open='(' close=')' separator=','> \n", filedName));
sb.append(StrUtil.format(" #{item}\n"));
sb.append(StrUtil.format(" </foreach> \n"));
sb.append(StrUtil.format(" </if> \n"));
}
Like like = AnnotationUtil.getAnnotation(field, Like.class);
if (like != null) {
sb.append(StrUtil.format("\n<if test=\"{} != null and {} !='' \">" +
" \n <![CDATA[ AND {} like concat('%',#{{}},'%') ]]>\n" +
" \n</if>", filedName, filedName, columnName, filedName));
}
NE ne = AnnotationUtil.getAnnotation(field, NE.class);
if (ne != null) {
sb.append(String.format("\n<if test=\"%s != null\">" +
" \n <![CDATA[ AND %s != #{%s} ]]>\n" +
" \n</if>", filedName, columnName, filedName));
}
if (ClassUtil.isAssignable(Range.class, field.getType())) {
sb.append(StrUtil.format(" <choose>\n" +
" <when test=\"{} != null and {}.left != null and {}.leftEQ == true\">\n" +
" <![CDATA[ AND {} >= #{{}.left} ]]>\n" +
" </when>\n" +
" <when test=\"{} != null and {}.left != null and {}.leftEQ == false\">\n" +
" <![CDATA[ AND {} > #{{}.left} ]]>\n" +
" </when>\n" +
" </choose>", filedName, filedName, filedName, columnName, filedName, filedName, filedName, filedName, columnName, filedName, filedName, filedName, filedName, columnName, filedName, filedName, filedName, filedName, columnName, filedName));
sb.append(StrUtil.format(" <choose>\n" +
" <when test=\"{} != null and {}.right != null and {}.rightEQ == true\">\n" +
" <![CDATA[ AND {} <= #{{}.right} ]]>\n" +
" </when>\n" +
" <when test=\"{} != null and {}.right != null and {}.rightEQ == false\">\n" +
" <![CDATA[ AND {} < #{{}.right} ]]>\n" +
" </when>\n" +
" </choose>", filedName, filedName, filedName, columnName, filedName, filedName, filedName, filedName, columnName, filedName, filedName, filedName, filedName, columnName, filedName, filedName, filedName, filedName, columnName, filedName));
}
}
sb.append(" </where> ");
sb.append(StrUtil.format("\n<if test=\"{} != null and {} !='' \">" +
" \n <![CDATA[ order by ${{}} ]]>\n" +
" \n</if>", "orderByBlock", "orderByBlock", "orderByBlock"));
sb.append(" </script>");
String sql = sb.toString().trim();
selectMap.putIfAbsent(queryClassName, sql);
return sql;
}
}
11.mapper层数据库操作
package com.example.mapper;
import com.beiyou.model.OrderMaster;
import com.beiyou.model.OrderMasterQuery;
import com.example.annotation.PageX;
import com.example.util.SqlGen;
import org.apache.ibatis.annotations.*;
import java.util.List;
@Mapper
public interface OrderMaterMapper {
@PageX
@SelectProvider(type = SqlGen.class, method = SqlGen.select)
List<OrderMaster> queryByOrderMasterQuery(OrderMasterQuery query);
@SelectKey(keyColumn = "id", keyProperty = "id", statement = "select last_insert_id()", before = false, resultType = Long.class)
@InsertProvider(type = SqlGen.class, method = SqlGen.insert)
Integer insertOrderMaster(OrderMaster orderMaster);
@UpdateProvider(type = SqlGen.class, method = SqlGen.update)
Integer updateOrderMaster(OrderMaster orderMaster);
}
12.测试的controller
package com.example.controller;
import cn.hutool.core.util.ArrayUtil;
import cn.hutool.core.util.ObjectUtil;
import com.beiyou.model.OrderMaster;
import com.beiyou.model.OrderMasterQuery;
import com.example.mapper.OrderMaterMapper;
import com.github.pagehelper.Page;
import com.github.pagehelper.PageHelper;
import lombok.extern.slf4j.Slf4j;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.TimeUnit;
@Slf4j
@RestController
public class DemoController {
@Resource
private OrderMaterMapper orderMaterMapper;
@GetMapping("/test/update")
public Integer testUpdate() {
OrderMasterQuery query = OrderMasterQuery.builder().id(7L).build();
List<OrderMaster> orderMasters = orderMaterMapper.queryByOrderMasterQuery(query);
OrderMaster one = orderMasters.get(0);
one.setAddress1(one.getAddress1() + "--up");
Integer ret = orderMaterMapper.updateOrderMaster(one);
return ret;
}
@Resource(name = "redisTemplate")
private ValueOperations<String, OrderMaster> valueOps;
public final String orderPre = "order#";
@PostMapping("/test/insert")
public Integer testInser(@RequestBody OrderMaster orderMaster) {
Integer ret = orderMaterMapper.insertOrderMaster(orderMaster);
String key = orderPre + orderMaster.getId();
valueOps.set(key, orderMaster);
return ret;
}
@PostMapping("/test/sqlgen2")
public List<OrderMaster> testSqlGen2(@RequestBody OrderMasterQuery query) {
List<OrderMaster> orderMasters = orderMaterMapper.queryByOrderMasterQuery(query);
return orderMasters;
}
@PostMapping("/test/sqlgen")
public List<OrderMaster> testSqlGen() {
OrderMasterQuery query = OrderMasterQuery.builder().phoneNumber("18676644557").build();
List<OrderMaster> orderMasters = orderMaterMapper.queryByOrderMasterQuery(query);
return orderMasters;
}
@PostMapping("/test/QueryByOrderMasterQuery")
public List<OrderMaster> testByOrderMasterQuery(@RequestBody OrderMasterQuery query) {
List<OrderMaster> retList = new ArrayList<>();
boolean b = ObjectUtil.isNotEmpty(query.getId());
if (b == true) {
OrderMaster orderMaster = valueOps.get(orderPre + query.getId());
if (orderMaster != null) {
retList.add(orderMaster);
log.debug("直接从redis获取,没有经过数据库");
return retList;
}
}
Long[] ids = query.getIds();
ArrayList<Long> notInRedisIds = new ArrayList<>();
for (Long id : ids) {
OrderMaster orderMaster = valueOps.get(orderPre + id);
if (orderMaster != null) {
retList.add(orderMaster);
} else {
notInRedisIds.add(id);
}
}
if (ObjectUtil.isEmpty(notInRedisIds)) {
return retList;
}
query.setIds(ArrayUtil.toArray(notInRedisIds, Long.class));
log.debug("筛选后的ids:{}", query.getIds());
List<OrderMaster> orderMasters = orderMaterMapper.queryByOrderMasterQuery(query);
log.debug("经过数据库");
for (OrderMaster orderMaster : orderMasters) {
valueOps.set(orderPre + orderMaster.getId(), orderMaster, 7, TimeUnit.DAYS);
log.debug("通过数据库查得:{}", orderMaster.getId());
}
return orderMasters;
}
}
|