1、数据库驱动
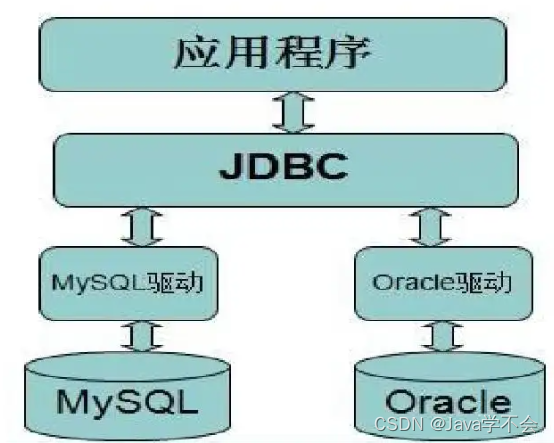
2、JDBC
- SUN公司为了简化开发人员的(对数据的统一)操作,提供了一个(Java操作数据库的)规范,俗称JDBC
- 对于开发人员,只需要掌握JDBC接口操作即可
3、第一个JDBC程序
-
创建测试数据库 CREATE DATABASE `jdbcStudy` CHARACTER SET utf8 COLLATE utf8_general_ci;
USE `jdbcStudy`;
CREATE TABLE `users`(
`id` INT PRIMARY KEY,
`NAME` VARCHAR(40),
`PASSWORD` VARCHAR(40),
`email` VARCHAR(60),
birthday DATE
);
INSERT INTO `users`(`id`,`NAME`,`PASSWORD`,`email`,`birthday`)
VALUES('1','zhangsan','123456','zs@sina.com','1980-12-04'),
('2','lisi','123456','lisi@sina.com','1981-12-04'),
('3','wangwu','123456','wangwu@sina.com','1979-12-04')
-
导入数据库驱动 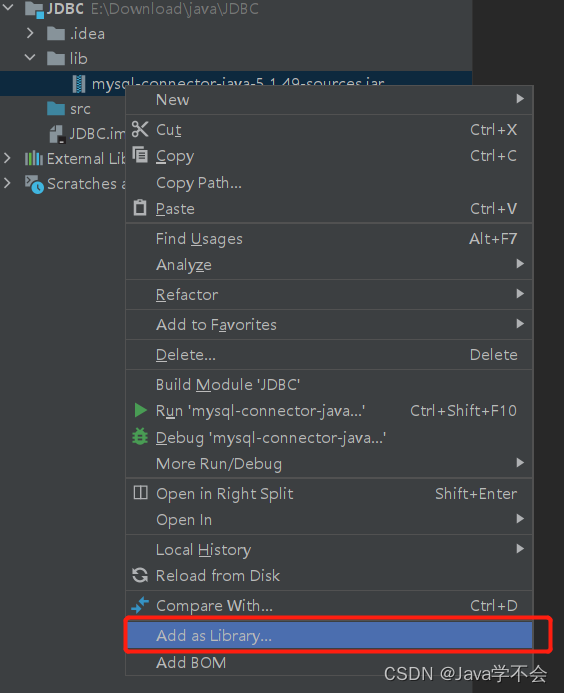 -
编写测试代码 package demo;
import java.sql.*;
public class JdbcFirstDemo {
public static void main( String[] args ) throws ClassNotFoundException, SQLException {
Class.forName("com.mysql.jdbc.Driver");
String url = "jdbc:mysql://localhost:3306/jdbcstudy?useUnicode=true&characterEncoding=utf8&useSSL=false";
String username = "root";
String password = "123456";
Connection connection = DriverManager.getConnection(url, username, password);
Statement statement = connection.createStatement();
String sql="SELECT * FROM users";
ResultSet resultSet = statement.executeQuery(sql);
while (resultSet.next()){
System.out.println("id="+resultSet.getObject(1));
System.out.println("name="+resultSet.getObject(2));
System.out.println("pwd="+resultSet.getObject(3));
System.out.println("email="+resultSet.getObject(4));
System.out.println("birthday="+resultSet.getObject(5));
System.out.println("======================");
}
resultSet.close();
statement.close();
connection.close();
}
}
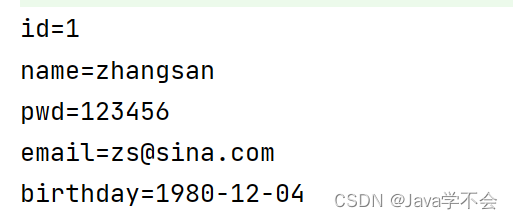
4、步骤总结
- 加载驱动
- 连接数据库 DriverManager
- 获得执行sql的对象 Statement
- 获得返回的结果集
5、JDBC对象解释
- Class.forName(“com.mysql.jdbc.Driver”); //加载驱动
- connection: 数据库对象,获得一个数据库
- statement: 执行SQL语句
- statement.executeQuery(sql) //查询语句,返回ResultSet结果集
- statement.executeUpdate(sql) //更新语句,返回更新的行数
- statement.execute(sql) //任何SQL语句
- ResultSet: SQL查询结果集,根据数据库的类型
- resultSet.next() //指针,移动到下一个数据
- resultSet.getInt(参数); //获得整型数据,可以是下标(从1开始),也可以是"表字段名称"
- resultSet.getString(参数); //获得字符串数据,可以是下标(从1开始),也可以是"表字段名称"
- resultSet.getObject(参数); //获得任意型数据,可以是下标(从1开始),也可以是"表字段名称"
- 释放资源:
- resultSet.close();
- statement.close();
- connection.close();
6、写工具类
-
在src目录下创建 db.properties 文件 driver=com.mysql.jdbc.Driver
url=jdbc:mysql://localhost:3306/jdbcstudy?useUnicode=true&characterEncoding=utf8&useSSL=false
username = root
password = 123456
-
创建工具类 JdbcUtils package demo;
import java.io.IOException;
import java.io.InputStream;
import java.sql.*;
import java.util.Properties;
public class JdbcUtils {
private static String driver=null;
private static String url=null;
private static String username=null;
private static String password=null;
static {
try{
InputStream in = JdbcUtils.class.getClassLoader().getResourceAsStream("db.properties");
Properties properties = new Properties();
properties.load(in);
driver= properties.getProperty("driver");
url= properties.getProperty("url");
username= properties.getProperty("username");
password= properties.getProperty("password");
Class.forName(driver);
}catch (IOException e){
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
public static Connection getConnection() throws SQLException {
return DriverManager.getConnection(url, username, password);
}
public static void release( Connection conn, Statement st,ResultSet rs ){
if(rs!=null){
try {
rs.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(st!=null){
try {
st.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn!=null){
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
-
使用工具类 package demo;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class TestInsert {
public static void main( String[] args ) {
Connection conn=null;
Statement st=null;
ResultSet rs=null;
try {
conn = JdbcUtils.getConnection();
st = conn.createStatement();
String sql="INSERT\tINTO users(`id`,`NAME`,`PASSWORD`,`email`,`birthday`) " +
"VALUES(4,'Java学不会','123456','2929054638@qq.com','2022-07-02')";
int i = st.executeUpdate(sql);
if(i>0){
System.out.println("插入成功");
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JdbcUtils.release(conn,st,rs);
}
}
}
7、JDBC操作事务
7.1、创建表
create table account(
id int primary key auto_increment,
name varchar(40),
money float
);
insert into account(name,money) values ('A',1000);
insert into account(name,money) values ('B',1000);
insert into account(name,money) values ('C',1000);
7.2、事务
-
要么都成功,要么都失败 -
ACID原则:
- 原子性:要么全部成功,要么全部失败
- 一致性:总数不变
- 隔离性:多个线程互不干扰
- 脏读:一个事务读取了另一个没有提交的事务
- 不可重复读:在同一个事务内,重复读取表中的数据,表数据发生了改变
- 虚读(幻读):在一个事务内,读取到了别人插入的数据,导致前后读出来结果不一致
- 持久性:一旦提交不可逆,持久化到数据库
package demo01;
import demo.JdbcUtils;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class TestTransaction01 {
public static void main( String[] args ) {
Connection conn=null;
PreparedStatement st=null;
ResultSet rs=null;
try {
conn = JdbcUtils.getConnection();
conn.setAutoCommit(false);
String sql1="update account set money = money-100 where name ='A'";
st = conn.prepareStatement(sql1);
st.execute();
int x = 1/0;
String sql2="update account set money = money+100 where name ='B'";
st = conn.prepareStatement(sql2);
st.execute();
conn.commit();
System.out.println("操作成功");
} catch (SQLException throwables) {
try {
conn.rollback();
} catch (SQLException e) {
e.printStackTrace();
}
} finally {
JdbcUtils.release(conn,st,rs);
}
}
}
7.3、总结
- 开始事务: conn.setAutoCommit(false);
- 一组业务执行完毕,提交事务
- 可以在catch语句中显示的定义,回滚语句,默认失败就会回滚
8、数据库连接池
- 数据库连接——执行完毕——释放
- 连接——释放十分浪费系统资源
- 池化技术:准备一些预先的资源,过来就连接预先准备好的
- 最小连接数
- 最大连接数
- 等待超时
- 编写连接池,实现一个接口 DataSource
- 开源数据源实现
- DBCP
- C3P0
- Druid
- 使用了这些数据库连接池只有,在项目开发中就不需要编写连接数据库的代码了
8.1 、DBCP:
-
创建 dbcpconfig.properties 文件 #连接设置 这里面的名词,是DBCP数据源中定义好的
driverClassName=com.mysql.jdbc.Driver
url=jdbc:mysql://localhost:3306/jdbcstudy?userUnicode=true&characterEncoding=utf8&useSSL=false
username=root
password=123456
#<!-- 初始化连接 -->
initialSize=10
#最大连接数量
maxActive=50
#<!-- 最大空闲连接 -->
maxIdle=20
#<!-- 最小空闲连接 -->
minIdle=5
#<!-- 超时等待时间以毫秒为单位 6000毫秒/1000等于60秒 -->
maxWait=60000
#JDBC驱动建立连接时附带的连接属性属性的格式必须为这样:【属性名=property;】
#注意:"user" 与 "password" 两个属性会被明确地传递,因此这里不需要包含他们。
connectionProperties=useUnicode=true;characterEncoding=utf8
#指定由连接池所创建的连接的自动提交(auto-commit)状态。
defaultAutoCommit=true
#driver default 指定由连接池所创建的连接的只读(read-only)状态。
#如果没有设置该值,则“setReadOnly”方法将不被调用。(某些驱动并不支持只读模式,如:Informix)
defaultReadOnly=
#driver default 指定由连接池所创建的连接的事务级别(TransactionIsolation)。
#可用值为下列之一:(详情可见javadoc。)NONE,READ_UNCOMMITTED, READ_COMMITTED, REPEATABLE_READ, SERIALIZABLE
defaultTransactionIsolation=READ_COMMITTED
-
工具类 package demo02;
import demo.JdbcUtils;
import org.apache.commons.dbcp2.BasicDataSource;
import org.apache.commons.dbcp2.BasicDataSourceFactory;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Properties;
public class JdbcUtils_DBCP {
private static BasicDataSource dataSource = null;
static {
try{
InputStream in = JdbcUtils.class.getClassLoader().getResourceAsStream("dbcpconfig.properties");
Properties properties = new Properties();
properties.load(in);
dataSource = BasicDataSourceFactory.createDataSource(properties);
}catch (Exception e){
}
}
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
public static void release( Connection conn, Statement st, ResultSet rs ){
if(rs!=null){
try {
rs.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(st!=null){
try {
st.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn!=null){
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
-
测试代码 package demo02;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class TestDBCP {
public static void main( String[] args ) {
Connection conn=null;
Statement st=null;
ResultSet rs=null;
try {
conn = JdbcUtils_DBCP.getConnection();
st = conn.createStatement();
String sql="INSERT INTO users(`id`,`NAME`,`PASSWORD`,`email`,`birthday`) " +
"VALUES(6,'JavaStudy','123456','2929054638@qq.com','2022-07-02')";
int i = st.executeUpdate(sql);
if(i>0){
System.out.println("插入成功");
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JdbcUtils_DBCP.release(conn,st,null);
}
}
}
8.2、c3p0
-
创建 c3p0-config.xml 文件 <?xml version="1.0" encoding="UTF-8"?>
<c3p0-config>
<default-config>
<property name="driverClass">com.mysql.jdbc.Driver</property>
<property name="jdbcUrl">jdbc:mysql://localhost:3306/jdbcstudy?userUnicode=true&characterEncoding=utf8&useSSL=false&</property>
<property name="user">root</property>
<property name="password">123456</property>
<property name="acquiredIncrement">5</property>
<property name="initialPoolSize">10</property>
<property name="minPoolSize">5</property>
<property name="maxPoolSize">20</property>
</default-config>
<name-config name="MySQL">
<property name="driverClass">com.mysql.jdbc.Driver</property>
<property name="jdbcUrl">jdbc:mysql://localhost:3306/jdbcstudy?userUnicode=true&characterEncoding=utf8&useSSL=false&</property>
<property name="user">root</property>
<property name="password">123456</property>
<property name="acquiredIncrement">5</property>
<property name="initialPoolSize">10</property>
<property name="minPoolSize">5</property>
<property name="maxPoolSize">20</property>
</name-config>
</c3p0-config>
-
工具类: package demo02;
import com.mchange.v2.c3p0.ComboPooledDataSource;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class JdbcUtils_c3p0 {
private static ComboPooledDataSource dataSource = null;
static {
try{
dataSource = new ComboPooledDataSource();
}catch (Exception e){
}
}
public static Connection getConnection() throws SQLException {
return dataSource.getConnection();
}
public static void release( Connection conn, Statement st, ResultSet rs ){
if(rs!=null){
try {
rs.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(st!=null){
try {
st.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
if(conn!=null){
try {
conn.close();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
}
}
-
测试代码: package demo02;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
public class Testc3p0 {
public static void main( String[] args ) {
Connection conn=null;
Statement st=null;
ResultSet rs=null;
try {
conn = JdbcUtils_c3p0.getConnection();
st = conn.createStatement();
String sql="INSERT INTO users(`id`,`NAME`,`PASSWORD`,`email`,`birthday`) " +
"VALUES(10,'JavaStudy','123456','2929054638@qq.com','2022-07-02')";
int i = st.executeUpdate(sql);
if(i>0){
System.out.println("插入成功");
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
JdbcUtils_DBCP.release(conn,st,null);
}
}
}
|