相对于AlertDialog的使用,PopupWindow的使用也比较简单,这里主要介绍的是PopupWindow的基础使用包括在使用过程中的一些注意事项,做个笔记。
效果展示:
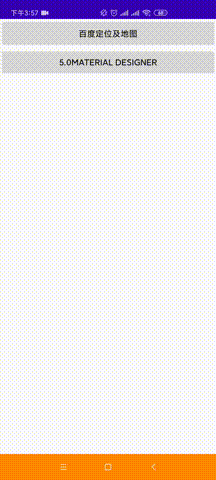
PopupWindow的基础方法
创建popupWindow实例
popupView = LayoutInflater.from(this).inflate(R.layout.popupwindow_view, null)
popupWindow = PopupWindow()
设置展示的视图
popupWindow?.contentView = popupView
设置宽度和高度
popupWindow?.width = ViewGroup.LayoutParams.WRAP_CONTENT
popupWindow?.height = ViewGroup.LayoutParams.WRAP_CONTENT
设置外部区域可以点击取消popupWindow
popupWindow?.isOutsideTouchable = true
设置背景
设置popupWindow是否可以聚焦
popupWindow?.isFocusable = true
设置弹窗弹出的动画高度
popupWindow?.elevation = 100f
设置popupWindow是否可以触摸
popupWindow?.isTouchable = true
设置触摸监听
popupWindow?.setTouchInterceptor { _, _ ->
Toast.makeText(this,"触发事件",Toast.LENGTH_SHORT).show()
false
}
设置取消事件监听
popupWindow?.setOnDismissListener {
Toast.makeText(this,"PopupWindow被关闭",Toast.LENGTH_SHORT).show()
}
PopupWindow的展示
方式一:
popupWindow?.showAsDropDown(view,0,0, Gravity.BOTTOM)
方式二:
popupWindow?.showAtLocation(view, Gravity.TOP, 0, 0)
当然,在实际的开发过程中我们并不能仅仅满足于如何简单使用,更多的时候我们需要去考虑兼容性与扩展性的问题,所以,在这里,我对PopupWindow做了一个简单的封装,如下所示:
简单封装
创建PopupWindow的管理类,即PopupWindowManager类
public class PopupWindowManager {
private int mPopupWindowWidth = ViewGroup.LayoutParams.MATCH_PARENT;
private int mPopupWindowHeight = ViewGroup.LayoutParams.WRAP_CONTENT;
private MyPopupWindow mPopupWindow;
private static volatile PopupWindowManager mInstance;
public static PopupWindowManager getInstance() {
if (mInstance == null) {
synchronized (PopupWindowManager.class) {
if (mInstance == null) {
mInstance = new PopupWindowManager();
}
}
}
return mInstance;
}
private PopupWindowManager() {
mPopupWindow = new MyPopupWindow();
}
private PopupWindow getMyPopupWindow() {
return mPopupWindow;
}
public PopupWindowManager init(View contentView) {
return init(contentView, mPopupWindowWidth, mPopupWindowHeight);
}
public PopupWindowManager init(View contentView, int width, int height) {
this.mPopupWindowWidth = width;
this.mPopupWindowHeight = height;
mPopupWindow.setContentView(contentView);
mPopupWindow.setOutsideTouchable(true);
mPopupWindow.setFocusable(true);
mPopupWindow.setElevation(0);
mPopupWindow.setTouchable(true);
setBackgroundDrawable();
mPopupWindow.setWidth(mPopupWindowWidth);
mPopupWindow.setHeight(mPopupWindowHeight);
mPopupWindow.setAnimationStyle(R.style.popup_window_anim_style);
return this;
}
private PopupWindowManager setBackgroundDrawable() {
ColorDrawable dw = new ColorDrawable(Color.parseColor("#50000000"));
mPopupWindow.setBackgroundDrawable(dw);
return this;
}
public PopupWindowManager setBackgroundDrawable(int color) {
ColorDrawable dw = new ColorDrawable(color);
mPopupWindow.setBackgroundDrawable(dw);
return this;
}
public PopupWindowManager setAnimationStyle(int animationStyle){
mPopupWindow.setAnimationStyle(animationStyle);
return this;
}
public PopupWindowManager setOutsideTouchable(boolean isOutsideTouchable) {
mPopupWindow.setOutsideTouchable(isOutsideTouchable);
return this;
}
public PopupWindowManager setFocusable(boolean isFocusable) {
mPopupWindow.setFocusable(isFocusable);
return this;
}
public PopupWindowManager setElevation(float elevation) {
mPopupWindow.setElevation(elevation);
return this;
}
public PopupWindowManager setTouchable(boolean touchable) {
mPopupWindow.setTouchable(touchable);
return this;
}
public PopupWindowManager setOnDismissListener(PopupWindow.OnDismissListener onDismissListener) {
mPopupWindow.setOnDismissListener(onDismissListener);
return this;
}
public PopupWindowManager setTouchInterceptor(View.OnTouchListener listener) {
mPopupWindow.setTouchInterceptor(listener);
return this;
}
public void showAsDropDown(View anchor) {
mPopupWindow.showAsDropDown(anchor);
}
public void showAsDropDown(View anchor, int xoff, int yoff) {
mPopupWindow.showAsDropDown(anchor, xoff, yoff);
}
public void showAsDropDown(View anchor, int xoff, int yoff, int gravity) {
mPopupWindow.showAsDropDown(anchor, xoff, yoff, gravity);
}
public void showAtLocation(View parent, int gravity, int x, int y) {
mPopupWindow.showAtLocation(parent, gravity, x, y);
}
public void close() {
if (mPopupWindow != null && mPopupWindow.isShowing()) {
mPopupWindow.dismiss();
}
}
}
新建类来继承自PopupWindow
public class MyPopupWindow extends PopupWindow {
@Override
public void showAsDropDown(View anchor) {
handlerHeight(anchor);
super.showAsDropDown(anchor);
}
@Override
public void showAsDropDown(View anchor, int xoff, int yoff) {
handlerHeight(anchor);
super.showAsDropDown(anchor, xoff, yoff);
}
@Override
public void showAsDropDown(View anchor, int xoff, int yoff, int gravity) {
handlerHeight(anchor);
super.showAsDropDown(anchor, xoff, yoff, gravity);
}
private void handlerHeight(View anchor) {
if (Build.VERSION.SDK_INT >= 24) {
Rect rect = new Rect();
anchor.getGlobalVisibleRect(rect);
int heightPixels = anchor.getResources().getDisplayMetrics().heightPixels;
int h = heightPixels - rect.bottom + getStatusHeight(anchor.getContext());
setHeight(h);
}
}
private int getStatusHeight(Context context) {
int statusHeight = -1;
try {
Class<?> clazz = Class.forName("com.android.internal.R$dimen");
Object object = clazz.newInstance();
int height = Integer.parseInt(clazz.getField("status_bar_height").get(object).toString());
statusHeight = context.getResources().getDimensionPixelSize(height);
} catch (Exception e) {
e.printStackTrace();
}
return statusHeight;
}
}
最后进行调用:
val popView = LayoutInflater.from(this).inflate(R.layout.pop_view, null)
PopupWindowManager.getInstance().init(popView).setOnDismissListener {
PopupWindowManager.getInstance().close()
}.setTouchInterceptor { v, event ->
PopupWindowManager.getInstance().close()
false
}.showAsDropDown(view)
到这里,popupWindow的简单使用就完成了。最后来讲一下在使用popupWindow的过程中需要注意的几点:
(1)必须手动给popupWindow设置宽度和高度,否则popupWindow不显示。 (2)在手机系统的API大于24的时候全屏展示的时候会完全填充整个屏幕,而不是在目标View的下方正常显示。 (3)在有些手机上面,全屏展示的时候底部会留白,其实是因为StatusBar的高度没有计算进去,需要我们自己手动计算出去。 (4)当我们设置了setFocusable为true的时候,点击屏幕事件会交由onTouchListener处理。
|