android 学习日记 smartLayout 底部选项卡
前言
最近在做项目时,用的最原始的底部选项卡的代码,又臭又长,发现了一个比较节省代码的控件,再次分享一下。
一、使用步骤
大致分为以下步骤:
1.导入相应依赖
2.编写smartLayout用到的布局样式文件
3.编写碎片文件
4.编写activity主页面 确定数据源 --> 引用适配器 --> smartLayout装载适配器
代码如下:
1.导入依赖
implementation 'com.ogaclejapan.smarttablayout:library:1.6.1@aar'
implementation 'com.ogaclejapan.smarttablayout:utils-v4:1.6.1@aar'
implementation 'androidx.legacy:legacy-support-v4:1.0.0'
2、编写smartLayout用到的布局样式文件 smart_item_style.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/imageView"
android:layout_width="30dp"
android:layout_height="30dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/tab_ic_home" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="商店"
android:textColor="#6D6D6D"
android:textSize="15sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
</androidx.constraintlayout.widget.ConstraintLayout>
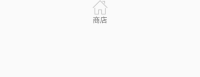 另外不要忘了写一个 点击时切换菜单时高亮显示的xml文件 需要两个图片文件,一个是点击时的图片样式 另一个是静态时的图片样式
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:drawable="@drawable/ic_home_normal" android:state_selected="true" />
<item android:drawable="@drawable/ic_home_selected" android:state_selected="false" />
</selector>
再写一个变换图片下面的字体颜色的xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:state_selected="true"
android:color="@color/purple_200" />
<item
android:state_selected="false"
android:color="@color/black" />
</selector>
 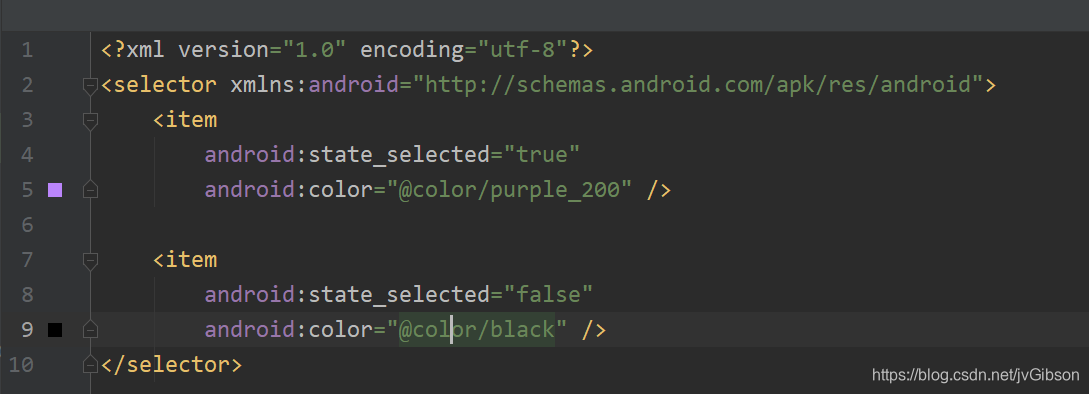
3、编写碎片文件
按照项目需求来编写 ,需要几个就写几个 这里只给出一个示例
package com.example.practice.fragment;
import androidx.fragment.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import com.example.practice.R;
public class ShopFragment extends Fragment {
View view ;
@Override
public View onCreateView(LayoutInflater inflater , ViewGroup Container , Bundle savedInstanceState) {
view = inflater.inflate(R.layout.activity_shop_fragment,null);
return view ;
}
}
4、编写activity主页面
确定数据源 --> 引用适配器 --> smartLayout装载适配器
package com.example.practice;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
import androidx.viewpager.widget.PagerAdapter;
import androidx.viewpager.widget.ViewPager;
import com.example.practice.fragment.ShopFragment;
import com.ogaclejapan.smarttablayout.SmartTabLayout;
import com.ogaclejapan.smarttablayout.utils.v4.FragmentPagerItemAdapter;
import com.ogaclejapan.smarttablayout.utils.v4.FragmentPagerItems;
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
private SmartTabLayout smartTabLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
final LayoutInflater inflater = LayoutInflater.from(this);
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
init();
final int[] tabIcons = {R.drawable.tab_ic_home, R.drawable.tab_ic_orders, R.drawable.tab_ic_me};
final String[] tabTitles = {"主页", "订单", "个人中心"};
FragmentPagerItems pages = FragmentPagerItems.with(this)
.add("主页", ShopFragment.class)
.add("订单", ShopFragment.class)
.add("我的", ShopFragment.class)
.create();
FragmentPagerItemAdapter adapter = new FragmentPagerItemAdapter(
getSupportFragmentManager(),
pages);
viewPager.setOffscreenPageLimit(pages.size());
viewPager.setAdapter(adapter);
smartTabLayout.setCustomTabView(new SmartTabLayout.TabProvider() {
@Override
public View createTabView(ViewGroup container, int position, PagerAdapter adapter) {
View view = inflater.inflate(R.layout.smart_item_style, container, false);
ImageView iconView = (ImageView) view.findViewById(R.id.imageView);
iconView.setImageResource(tabIcons[position % tabIcons.length]);
TextView titleView = (TextView) view.findViewById(R.id.textView2);
titleView.setText(tabTitles[position % tabTitles.length]);
return view;
}
});
smartTabLayout.setViewPager(viewPager);
}
private void init() {
viewPager = findViewById(R.id.viewpager);
smartTabLayout = findViewById(R.id.smarttablayout);
}
}
|