AIDL File: ILights.aidl
package android.hardware.light;
import android.hardware.light.HwLightState; import android.hardware.light.HwLight;
/** ?* Allows controlling logical lights/indicators, mapped to LEDs in a ?* hardware-specific manner by the HAL implementation. ?*/ @VintfStability interface ILights { ? ? /** ? ? ?* Set light identified by id to the provided state. ? ? ?* ? ? ?* If control over an invalid light is requested, this method exists with ? ? ?* EX_UNSUPPORTED_OPERATION. Control over supported lights is done on a ? ? ?* device-specific best-effort basis and unsupported sub-features will not ? ? ?* be reported. ? ? ?* ? ? ?* @param id ID of logical light to set as returned by getLights() ? ? ?* @param state describes what the light should look like. ? ? ?*/ ? ? void setLightState(in int id, in HwLightState state);
? ? /** ? ? ?* Discover what lights are supported by the HAL implementation. ? ? ?* ? ? ?* @return List of available lights ? ? ?*/ ? ? HwLight[] getLights(); }
ILights的类结构
ILights.java是个 Interface class, 其中包含了内部类Sub, 而Proxy又是Sub的内部类,Sub和Proxy都 implement ILights接口,但Proxy没有继承Binder, Proxy是通过成员变量mRemote去请求服务。
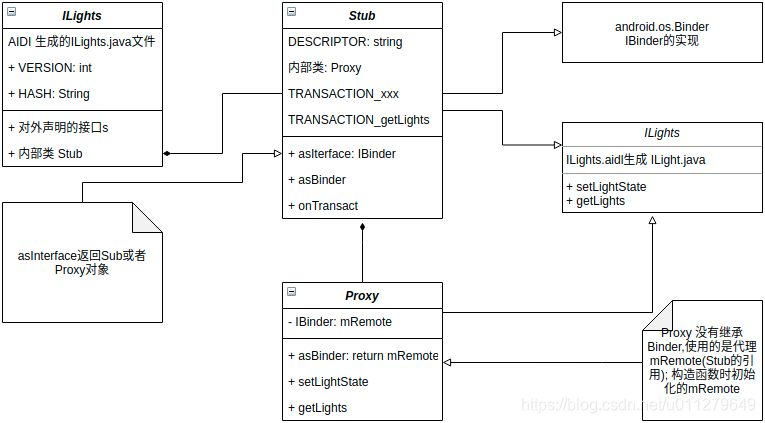
?代理类的创建肯定要得到Stub的应用,Proxy接口函数的实现通过mRemote.transact(Stub.TRANSACTION_setLightState, _data, _reply, 0) 调用到 Stub的实现, 这是标准的Client/Server架构和 代理设计模式
// 因为可能跨进程传输(即使不跨进程)也要进行打包解包(编解码)防止数据传输过程错误
?@Override
public boolean onTransact(int code, android.os.Parcel data, android.os.Parcel reply, int flags) throws android.os.RemoteException ? ? { ? ? ? java.lang.String descriptor = DESCRIPTOR; ? ? ? switch (code) ? ? ? { ? ? ? ? case TRANSACTION_setLightState: ? ? ? ? { ? ? ? ? ? data.enforceInterface(descriptor); ? ? ? ? ? int _arg0; ? ? ? ? ? _arg0 = data.readInt(); ? ? ? ? ? android.hardware.light.HwLightState _arg1; ? ? ? ? ? if ((0!=data.readInt())) { ? ? ? ? ? ? _arg1 = android.hardware.light.HwLightState.CREATOR.createFromParcel(data); ? ? ? ? ? } ? ? ? ? ? else { ? ? ? ? ? ? _arg1 = null; ? ? ? ? ? } ? ? ? ? ? this.setLightState(_arg0, _arg1); // server端去完成接口具体的实现 ? ? ? ? ? reply.writeNoException(); ? ? ? ? ? return true; ? ? ? ? } ? ? }
生成的ILights.java
/* ?* This file is auto-generated. ?DO NOT MODIFY. ?*/ package android.hardware.light; public interface ILights extends android.os.IInterface { ? /** ? ?* The version of this interface that the caller is built against. ? ?* This might be different from what {@link #getInterfaceVersion() ? ?* getInterfaceVersion} returns as that is the version of the interface ? ?* that the remote object is implementing. ? ?*/ ? public static final int VERSION = 1; ? public static final String HASH = "33fec8401b6e66bddaeff251e1a2a0f4fa0d3bee"; ? /** Default implementation for ILights. */ ? public static class Default implements android.hardware.light.ILights ? { ? ? @Override public void setLightState(int id, android.hardware.light.HwLightState state) throws android.os.RemoteException ? ? { ? ? } ? ? @Override public android.hardware.light.HwLight[] getLights() throws android.os.RemoteException ? ? { ? ? ? return null; ? ? } ? ? @Override ? ? public int getInterfaceVersion() { ? ? ? return 0; ? ? } ? ? @Override ? ? public String getInterfaceHash() { ? ? ? return ""; ? ? } ? ? @Override ? ? public android.os.IBinder asBinder() { ? ? ? return null; ? ? } ? }
? /** Local-side IPC implementation stub class. */ ? public static abstract class Stub extends android.os.Binder implements android.hardware.light.ILights ? { ? ? private static final java.lang.String DESCRIPTOR = "android$hardware$light$ILights".replace('$', '.'); ? ? /** Construct the stub at attach it to the interface. */ ? ? public Stub() ? ? { ? ? ? this.markVintfStability(); ? ? ? this.attachInterface(this, DESCRIPTOR); ? ? } ? ? /** ? ? ?* Cast an IBinder object into an android.hardware.light.ILights interface, ? ? ?* generating a proxy if needed. ? ? ?*/ ? ? public static android.hardware.light.ILights asInterface(android.os.IBinder obj) ? ? { ? ? ? if ((obj==null)) { ? ? ? ? return null; ? ? ? } ? ? ? android.os.IInterface iin = obj.queryLocalInterface(DESCRIPTOR); ? ? ? if (((iin!=null)&&(iin instanceof android.hardware.light.ILights))) { ? ? ? ? return ((android.hardware.light.ILights)iin); ? ? ? } ? ? ? return new android.hardware.light.ILights.Stub.Proxy(obj); ? ? } ? ? @Override public android.os.IBinder asBinder() ? ? { ? ? ? return this; ? ? } ? ? @Override public boolean onTransact(int code, android.os.Parcel data, android.os.Parcel reply, int flags) throws android.os.RemoteException ? ? { ? ? ? java.lang.String descriptor = DESCRIPTOR; ? ? ? switch (code) ? ? ? { ? ? ? ? case INTERFACE_TRANSACTION: ? ? ? ? { ? ? ? ? ? reply.writeString(descriptor); ? ? ? ? ? return true; ? ? ? ? } ? ? ? ? case TRANSACTION_setLightState: ? ? ? ? { ? ? ? ? ? data.enforceInterface(descriptor); ? ? ? ? ? int _arg0; ? ? ? ? ? _arg0 = data.readInt(); ? ? ? ? ? android.hardware.light.HwLightState _arg1; ? ? ? ? ? if ((0!=data.readInt())) { ? ? ? ? ? ? _arg1 = android.hardware.light.HwLightState.CREATOR.createFromParcel(data); ? ? ? ? ? } ? ? ? ? ? else { ? ? ? ? ? ? _arg1 = null; ? ? ? ? ? } ? ? ? ? ? this.setLightState(_arg0, _arg1); ? ? ? ? ? reply.writeNoException(); ? ? ? ? ? return true; ? ? ? ? } ? ? ? ? case TRANSACTION_getLights: ? ? ? ? { ? ? ? ? ? data.enforceInterface(descriptor); ? ? ? ? ? android.hardware.light.HwLight[] _result = this.getLights(); ? ? ? ? ? reply.writeNoException(); ? ? ? ? ? reply.writeTypedArray(_result, android.os.Parcelable.PARCELABLE_WRITE_RETURN_VALUE); ? ? ? ? ? return true; ? ? ? ? } ? ? ? ? case TRANSACTION_getInterfaceVersion: ? ? ? ? { ? ? ? ? ? data.enforceInterface(descriptor); ? ? ? ? ? reply.writeNoException(); ? ? ? ? ? reply.writeInt(getInterfaceVersion()); ? ? ? ? ? return true; ? ? ? ? } ? ? ? ? case TRANSACTION_getInterfaceHash: ? ? ? ? { ? ? ? ? ? data.enforceInterface(descriptor); ? ? ? ? ? reply.writeNoException(); ? ? ? ? ? reply.writeString(getInterfaceHash()); ? ? ? ? ? return true; ? ? ? ? } ? ? ? ? default: ? ? ? ? { ? ? ? ? ? return super.onTransact(code, data, reply, flags); ? ? ? ? } ? ? ? } ? ? }
? ? private static class Proxy implements android.hardware.light.ILights ? ? { ? ? ? private android.os.IBinder mRemote; ? ? ? Proxy(android.os.IBinder remote) ? ? ? { ? ? ? ? mRemote = remote; ? ? ? } ? ? ? private int mCachedVersion = -1; ? ? ? private String mCachedHash = "-1"; ? ? ? @Override public android.os.IBinder asBinder() ? ? ? { ? ? ? ? return mRemote; ? ? ? } ? ? ? public java.lang.String getInterfaceDescriptor() ? ? ? { ? ? ? ? return DESCRIPTOR; ? ? ? } ? ? ? @Override public void setLightState(int id, android.hardware.light.HwLightState state) throws android.os.RemoteException ? ? ? { ? ? ? ? android.os.Parcel _data = android.os.Parcel.obtain(); ? ? ? ? android.os.Parcel _reply = android.os.Parcel.obtain(); ? ? ? ? try { ? ? ? ? ? _data.writeInterfaceToken(DESCRIPTOR); ? ? ? ? ? _data.writeInt(id); ? ? ? ? ? if ((state!=null)) { ? ? ? ? ? ? _data.writeInt(1); ? ? ? ? ? ? state.writeToParcel(_data, 0); ? ? ? ? ? } ? ? ? ? ? else { ? ? ? ? ? ? _data.writeInt(0); ? ? ? ? ? } ? ? ? ? ? boolean _status = mRemote.transact(Stub.TRANSACTION_setLightState, _data, _reply, 0); ? ? ? ? ? if (!_status && getDefaultImpl() != null) { ? ? ? ? ? ? getDefaultImpl().setLightState(id, state); ? ? ? ? ? ? return; ? ? ? ? ? } ? ? ? ? ? _reply.readException(); ? ? ? ? } ? ? ? ? finally { ? ? ? ? ? _reply.recycle(); ? ? ? ? ? _data.recycle(); ? ? ? ? } ? ? ? } ? ? ? @Override public android.hardware.light.HwLight[] getLights() throws android.os.RemoteException ? ? ? { ? ? ? ? android.os.Parcel _data = android.os.Parcel.obtain(); ? ? ? ? android.os.Parcel _reply = android.os.Parcel.obtain(); ? ? ? ? android.hardware.light.HwLight[] _result; ? ? ? ? try { ? ? ? ? ? _data.writeInterfaceToken(DESCRIPTOR); ? ? ? ? ? boolean _status = mRemote.transact(Stub.TRANSACTION_getLights, _data, _reply, 0); ? ? ? ? ? if (!_status && getDefaultImpl() != null) { ? ? ? ? ? ? return getDefaultImpl().getLights(); ? ? ? ? ? } ? ? ? ? ? _reply.readException(); ? ? ? ? ? _result = _reply.createTypedArray(android.hardware.light.HwLight.CREATOR); ? ? ? ? } ? ? ? ? finally { ? ? ? ? ? _reply.recycle(); ? ? ? ? ? _data.recycle(); ? ? ? ? } ? ? ? ? return _result; ? ? ? } ? ? ? @Override ? ? ? public int getInterfaceVersion() throws android.os.RemoteException { ? ? ? ? if (mCachedVersion == -1) { ? ? ? ? ? android.os.Parcel data = android.os.Parcel.obtain(); ? ? ? ? ? android.os.Parcel reply = android.os.Parcel.obtain(); ? ? ? ? ? try { ? ? ? ? ? ? data.writeInterfaceToken(DESCRIPTOR); ? ? ? ? ? ? boolean _status = mRemote.transact(Stub.TRANSACTION_getInterfaceVersion, data, reply, 0); ? ? ? ? ? ? if (!_status) { ? ? ? ? ? ? ? if (getDefaultImpl() != null) { ? ? ? ? ? ? ? ? return getDefaultImpl().getInterfaceVersion(); ? ? ? ? ? ? ? } ? ? ? ? ? ? } ? ? ? ? ? ? reply.readException(); ? ? ? ? ? ? mCachedVersion = reply.readInt(); ? ? ? ? ? } finally { ? ? ? ? ? ? reply.recycle(); ? ? ? ? ? ? data.recycle(); ? ? ? ? ? } ? ? ? ? } ? ? ? ? return mCachedVersion; ? ? ? } ? ? ? @Override ? ? ? public synchronized String getInterfaceHash() throws android.os.RemoteException { ? ? ? ? if ("-1".equals(mCachedHash)) { ? ? ? ? ? android.os.Parcel data = android.os.Parcel.obtain(); ? ? ? ? ? android.os.Parcel reply = android.os.Parcel.obtain(); ? ? ? ? ? try { ? ? ? ? ? ? data.writeInterfaceToken(DESCRIPTOR); ? ? ? ? ? ? boolean _status = mRemote.transact(Stub.TRANSACTION_getInterfaceHash, data, reply, 0); ? ? ? ? ? ? if (!_status) { ? ? ? ? ? ? ? if (getDefaultImpl() != null) { ? ? ? ? ? ? ? ? return getDefaultImpl().getInterfaceHash(); ? ? ? ? ? ? ? } ? ? ? ? ? ? } ? ? ? ? ? ? reply.readException(); ? ? ? ? ? ? mCachedHash = reply.readString(); ? ? ? ? ? } finally { ? ? ? ? ? ? reply.recycle(); ? ? ? ? ? ? data.recycle(); ? ? ? ? ? } ? ? ? ? } ? ? ? ? return mCachedHash; ? ? ? } ? ? ? public static android.hardware.light.ILights sDefaultImpl; ? ? } ? ? static final int TRANSACTION_setLightState = (android.os.IBinder.FIRST_CALL_TRANSACTION + 0); ? ? static final int TRANSACTION_getLights = (android.os.IBinder.FIRST_CALL_TRANSACTION + 1); ? ? static final int TRANSACTION_getInterfaceVersion = (android.os.IBinder.FIRST_CALL_TRANSACTION + 16777214); ? ? static final int TRANSACTION_getInterfaceHash = (android.os.IBinder.FIRST_CALL_TRANSACTION + 16777213);
? ? public static boolean setDefaultImpl(android.hardware.light.ILights impl) { ? ? ? // Only one user of this interface can use this function ? ? ? // at a time. This is a heuristic to detect if two different ? ? ? // users in the same process use this function. ? ? ? if (Stub.Proxy.sDefaultImpl != null) { ? ? ? ? throw new IllegalStateException("setDefaultImpl() called twice"); ? ? ? } ? ? ? if (impl != null) { ? ? ? ? Stub.Proxy.sDefaultImpl = impl; ? ? ? ? return true; ? ? ? } ? ? ? return false; ? ? } ? ? public static android.hardware.light.ILights getDefaultImpl() { ? ? ? return Stub.Proxy.sDefaultImpl; ? ? } ? } ? public void setLightState(int id, android.hardware.light.HwLightState state) throws android.os.RemoteException; ? public android.hardware.light.HwLight[] getLights() throws android.os.RemoteException; ? public int getInterfaceVersion() throws android.os.RemoteException; ? public String getInterfaceHash() throws android.os.RemoteException; }
|