Service是什么?
服务实现了应用程序在后台运行,适合不需要和用户交互且还要长期运行的程序。
服务虽然不依赖于任何用户界面,但依赖创建服务的应用程序进程,当该应用程序被杀掉时,所依赖的服务也会停止运行。
服务不是运作在独立的进程当中,且并不会自动开启线程,代码默认运行在主线程中,我们需要在服务内部手动创建子线程,否则就有可能出现被主线程阻塞住的情况。
Service使用
选择包New→Service→Service,Exported表示是否允许其他程序访问这个服务,Enable表示是否启用这个服务,勾选,可选择重写:
- onCreate():服务第一次创建时调用
- onStartCommand():每次启动服务时调用
- onDestory():服务销毁时调用
启动服务:
Intent startIntent = new Intent(this, MyService.class);
startService(startIntent);
停止服务,此外还可以在Service内部调用stopSelf()停止服务:
Intent stopIntent = new Intent(this, MyService.class);
stopService(stopIntent);
Service和Activity绑定
修改activity_main.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/bind_service"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Bind Service" />
<Button
android:id="@+id/unbind_service"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="unbind Service" />
</LinearLayout>
新建MyService,创建内部类Binder用于管理Service,并在onBind()方法中返回:
public class MyService extends Service {
private DownLoadBinder mBinder = new DownLoadBinder();
class DownLoadBinder extends Binder {
public void startDownload() {
Log.d("MyService", "startDownload");
}
public int getProgress() {
Log.d("MyService", "startDownload");
return 0;
}
}
@Override
public IBinder onBind(Intent intent) {
return mBinder;
}
}
修改MainActivity,创建匿名内部类ServiceConnection实例,重写
- onServiceConnected():绑定时调用
- onServiceDisconnected():解绑时调用
在onServiceConnected()中获取管理Service的Binder,并调用相关方法。
调用bindService()进行绑定,第一个参数为Intent,第二个参数为ServiceConnection,第三个参数为BIND_AUTO_CREATE,表示在绑定后自动创建服务,会执行Service中的onCreate()方法,但不会执行onStartCommand()方法。
public class MainActivity extends AppCompatActivity {
private MyService.DownLoadBinder downLoadBinder;
private ServiceConnection connection = new ServiceConnection() {
@Override
public void onServiceConnected(ComponentName name, IBinder service) {
downLoadBinder = (MyService.DownLoadBinder) service;
downLoadBinder.startDownload();
downLoadBinder.getProgress();
}
@Override
public void onServiceDisconnected(ComponentName name) {
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button bind = findViewById(R.id.bind_service);
Button unbind = findViewById(R.id.unbind_service);
bind.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent bindIntent = new Intent(MainActivity.this, MyService.class);
bindService(bindIntent, connection, BIND_AUTO_CREATE);
}
});
unbind.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
unbindService(connection);
}
});
}
}
Service生命周期
当既调用startService()方法,又调用bindService()方法时,想要销毁Service就需要同时调用stopService()方法和unbindService()方法,onDestory()方法才会执行。
前台服务(报错未解决)
前台服务可以一直保持运行,不会因系统内存不足而回收,且会有运行图标在系统状态栏显示,下拉后可查看详细信息。
修改MyService中的onCreate()方法:
@Override
public void onCreate() {
super.onCreate();
Intent intent=new Intent(this,MainActivity.class);
PendingIntent pendingIntent=PendingIntent.getActivity(this,0,intent,0);
Notification notification=new NotificationCompat.Builder(this)
.setContentTitle("Title")
.setContentText("Text")
.setWhen(System.currentTimeMillis())
.setSmallIcon(R.mipmap.ic_launcher)
.setLargeIcon(BitmapFactory.decodeResource(getResources(), R.mipmap.ic_launcher))
.setContentIntent(pendingIntent)
.build();
startForeground(1,notification);
}
IntentService
服务的代码默认运行在主线程中,如果在服务内部处理耗时操作,就容易出现ANR(Application Not Responding),IntentServie提供了一个异步的、会自动停止的服务。
新建MyIntentService继承IntentService
- 重写无参构造函数调用父类有参构造函数
- 重写onHandleIntent()方法处理耗时操作(已自动开启线程)
- 重写onDestroy()方法查看是否会自动停止
public class MyIntentService extends IntentService {
public MyIntentService() {
super("MyIntentService");
}
@Override
protected void onHandleIntent(@Nullable Intent intent) {
Log.d("MyIntentService", String.valueOf(Thread.currentThread().getId()));
}
@Override
public void onDestroy() {
super.onDestroy();
Log.d("MyIntentService", "onDestroy: ");
}
}
在Mainfest.xml中注册:
<service android:name=".MyIntentService"/>
开启IntentService:
Log.d("MainActivity", String.valueOf(Thread.currentThread().getId()));
Intent intent = new Intent(MainActivity.this, MyIntentService.class);
startService(intent);
下图可以看到IntentService不在主线程且自动调用了onDonestroy()方法: 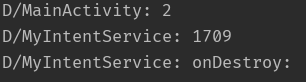
|