一、navigation说明
????????navigation是2018年谷歌推出的Android Jetpack应用开发架构中的一个导航组件。学习kotlin时看到有这么个组件,没用过,就新建一个项目看了一下效果,一下子就爱了,太方便了,以前需要很多代码很多界面才能实现的app使用方法导航功能和底部或顶部导航功能,使用navigation很简单方便的就能实现了。
二、navigation使用方法
? ? ? ? 1、导入库文件:
//java中使用
implementation 'androidx.navigation:navigation-fragment:2.2.2'
implementation 'androidx.navigation:navigation-ui:2.2.2'
//kotlin中使用
implementation 'androidx.navigation:navigation-fragment-ktx:2.2.2'
implementation 'androidx.navigation:navigation-ui-ktx:2.2.2'
? ? ? ? 2、手动新建几个Fragment子类,这里写了一个,可以参考:
/**
*
* 类描述:主页fragment
* 备注:学习kotlin,学习jetpack中的navigation
* R.layout.fragment_home:布局文件
* 具体的界面和逻辑代码根据需求编写
*/
class HomeFragment :Fragment(R.layout.fragment_home){
}
? ? ? ??
????????3、在资源文件res下新建一个navigation文件夹,注意是文件夹,不是文件,新建时记得类型选择navigation。
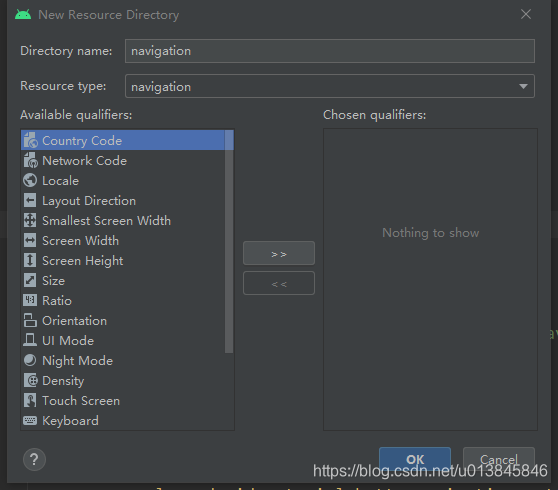
????????4、在navigation文件夹下新建一个文件,名字自定义,我定义的navigation_demo.xml。
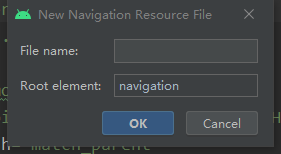
? ? ? ?5、navigation_demo文件中:
<?xml version="1.0" encoding="utf-8"?>
<navigation xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/demo"<!-- 这个属性是你这个xml文件navigation的id,很重要,我们需要在activity的xml布局里引用,记得写上不要忘记-->
app:startDestination="@id/home"><!--首次加载的第一个页面,很重要,一般就是第一个fragment-->
<fragment android:id="@+id/home"<!-- 每一个fragment节点都需要有自己的id,很重要. 我们需要在后面的节点上使用这些id指定跳转目标-->
android:name="com.demo.kotlin.demo.ui.capital.fragment.HomeFragment"<!--这个节点所对应的fragment(需要你导入指定的fragment文件路径),这个很重要-->
android:label="@string/home"<!--标签,显示的文字,非必须-->
tools:layout="@layout/fragment_home"/><!--HomeFragment对应的布局文件-->
<action
android:id="@+id/action_home_to_sort"<!--这个跳转动作的id, 这个id我们将在后面的代码中调用,用于执行fragment的跳转-->
app:destination="@id/imooc_sort" <!--跳转的目标fragment-->
/>
<fragment android:id="@+id/sort"
android:name="com.demo.kotlin.demo.ui.capital.fragment.SortFragment"
android:label="@string/sort"
tools:layout="@layout/fragment_home"/>
<fragment android:id="@+id/find"
android:name="com.demo.kotlin.demo.ui.capital.fragment.FindFragment"
android:label="@string/find"
tools:layout="@layout/fragment_home"/>
<fragment android:id="@+id/learn"
android:name="com.demo.kotlin.demo.ui.capital.fragment.LearnFragment"
android:label="@string/learn"
tools:layout="@layout/fragment_home"/>
<fragment android:id="@+id/user"
android:name="com.demo.kotlin.demo.ui.capital.fragment.UserFragment"
android:label="@string/user"
tools:layout="@layout/fragment_home"/>
</navigation>
? ? ? ? 注意:有些属性事必须写的,有的可以不写。
? ? ? ? 6、activity的布局中添加Fragment,有几点需要注意的,已写在注释中:
<fragment
android:id="@+id/fragment_demo"<!--id-->
android:name="androidx.navigation.fragment.NavHostFragment"<!--这个非常
重要,这是告知fragment需要使用navigation模式的关键属性,另外它是固定死的.你必需写.-->
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
app:defaultNavHost="true"<!-- 这是实现物理按键(比如返回键),
是按一下退出一个fragment 还是直接退出这个Activity的关键属性-->
app:navGraph="@navigation/navigation_demo"<!--很重要,这就是我们前面创建
的navigation的xml文件-->
/>
? ? ? ? 就这样,一个简单的使用navigation demo就完成了。
? ? ? ? 7、Fragment之间的跳转:
//tv是fragment_home中的一个TextView
//action_home_to_sort就是navigation文件夹下文件中一个action节点下的一个属性
binding.tv.setOnClickListener {
findNavController().navigate(R.id.action_home_to_sort)
}
findNavController().popBackStack() //第二个fragment返回上一个Fragment
? ? ? ? 到这里,使用以上功能就可以实现一个新安装的app的导航界面了。
? ? ? ? 8、Fragment经常和导航栏一起配合使用,在navigation中,也有这个功能。
? ? ? ? 首先新建一个menu文件夹,文件夹中新建一个menu._demo.xml(名字自定义)文件:
<?xml version="1.0" encoding="utf-8"?>
<menu xmlns:android="http://schemas.android.com/apk/res/android">
<!--android:id的值必须和navigation_demo.xml文件中fragment的id保持一致-->
<!--android:icon是图标,我使用的是矢量图,一张图,选中和未选中状态不一样,没有使用以前的select-->
<item
android:id="@id/home"
android:icon="@drawable/svg_home_n"
android:title="@string/home"/>
<item
android:id="@id/sort"
android:icon="@drawable/svg_sort_n"
android:title="@string/sort"/>
<item
android:id="@id/find"
android:icon="@drawable/svg_find_n"
android:title="@string/find"/>
<item
android:id="@id/learn"
android:icon="@drawable/svg_learn_n"
android:title="@string/learn"/>
<item
android:id="@id/user"
android:icon="@drawable/svg_user_n"
android:title="@string/user"/>
</menu>
? ? ? ? activity的布局文件中增加以下代码:
<com.google.android.material.bottomnavigation.BottomNavigationView
android:id="@+id/nav_view"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="bottom"
app:menu="@menu/menu_demo"/>
? ? ? ? 有一个问题,我这么做,调试的时候,导航栏图片选中和没选择都是正常的,但是title选中的时候显示,没选中的时候有title的位置,但是没有显示出来,不知道哪里做错了。
备注:这是看着慕课网上的学习视频总结的,有想看相关视频,可以上慕课网上找一下。
|