【27.EditText软键盘】
EditText初始不弹出软键盘,只有光标显示,点击再弹出 解决方法1: 在清单activity属性中设置
android:windowSoftInputMode="stateHidden"
解决方法2:
InputMethodManager inputMethodManager = (InputMethodManager)this.getSystemService(Context.INPUT_METHOD_SERVICE);
inputMethodManager.hideSoftInputFromWindow(et.getWindowToken(), 0);
解决方法3: 系统默认第一个EditText是获得焦点的,解决办法,增加一个不显示的view强制获得焦点,比如
<View
android:layout_width="0dip"
android:layout_height="0dip"
android:focusableInTouchMode="true" />
【隐藏软键盘-未验证】
public void hideSoftInput() {
InputMethodManager imm = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE);
if (getCurrentFocus() != null) {
imm.hideSoftInputFromWindow(getCurrentFocus().getWindowToken(), 0);
}
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
if (ev.getAction() == MotionEvent.ACTION_DOWN) {
View v = getCurrentFocus();
if (ToolUtil.isShouldHideInput(v, ev)) {
InputMethodManager imm = (InputMethodManager) getSystemService(Context.INPUT_METHOD_SERVICE);
if (imm != null) {
imm.hideSoftInputFromWindow(v.getWindowToken(), 0);
}
}
return super.dispatchTouchEvent(ev);
}
if (getWindow().superDispatchTouchEvent(ev)) {
return true;
}
return onTouchEvent(ev);
}
public void showInputMethod(){
if (getCurrentFocus() != null){
InputMethodManager imm = (InputMethodManager) getSystemService(INPUT_METHOD_SERVICE);
imm.showSoftInputFromInputMethod(getCurrentFocus().getWindowToken(),0);
}
}
【软键盘不遮挡输入框】 https://blog.csdn.net/u012523122/article/details/52101303/
方法一:在你的activity中的oncreate中setContentView之前写上这个代码
getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_ADJUST_PAN);
方法二:在项目的AndroidManifest.xml文件中界面对应的里加入
android:windowSoftInputMode="stateVisible|adjustResize"
这样会让屏幕整体上移。
如果加上的 是 android:windowSoftInputMode="adjustPan"这样键盘就会覆盖屏幕。
各值的含义:
stateUnspecified:软键盘的状态并没有指定,系统将选择一个合适的状态或依赖于主题的设置
stateUnchanged:当这个activity出现时,软键盘将一直保持在上一个activity里的状态,无论是隐藏还是显示
stateHidden:用户选择activity时,软键盘总是被隐藏
stateAlwaysHidden:当该Activity主窗口获取焦点时,软键盘也总是被隐藏的
stateVisible:软键盘通常是可见的
stateAlwaysVisible:用户选择activity时,软键盘总是显示的状态
adjustUnspecified:默认设置,通常由系统自行决定是隐藏还是显示
adjustResize:该Activity总是调整屏幕的大小以便留出软键盘的空间
adjustPan:当前窗口的内容将自动移动以便当前焦点从不被键盘覆盖和用户能总是看到输入内容的部分
方法三:(用的此处)
把顶级的layout替换成ScrollView,或者说在顶级的Layout上面再加一层ScrollView。
这样就会把软键盘和输入框一起滚动了,软键盘会一直处于底部。
一般顶级View需要加上 android:fitsSystemWindows="true"
【28.定时器】(注意不是延时器)
1.Handler类的postDelayed方法:
Handler mHandler = new Handler();
Runnable r = new Runnable() {
@Override
public void run() {
mHandler.postDelayed(this, 1000);
}
};
主线程中调用: mHandler.postDelayed(r, 100);
2.用handler+timer+timeTask方法:
Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
if (msg.what == 1){
}
super.handleMessage(msg);
}
};
Timer timer = new Timer();
TimerTask timerTask = new TimerTask() {
@Override
public void run() {
Message message = new Message();
message.what = 1;
handler.sendMessage(message);
}
};
主线程中调用:timer.schedule(timerTask,1000,500);
3.Thread+handler方法:
Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
if (msg.what == 1){
}
super.handleMessage(msg);
}
};
class MyThread extends Thread {
@Override
public void run() {
while (true){
try {
Thread.sleep(1000);
Message msg = new Message();
msg.what = 1;
handler.sendMessage(msg);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
主线程中调用:new Thread(new MyThread()).start();
【29.延时器】
1.Handler的postDelayed方法:
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
}
}, 1000);
2.timer + TimerTask方法:
Timer timer = new Timer();
timer.schedule(new TimerTask() {
@Override
public void run() {
}
},1000);
3.Thread方法:
new Thread(new MyThread()).start();
new Thread(new Runnable() {
@Override
public void run() {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}).start();
【30.TextView 上下滑动 左右滑动设置】
1.垂直滑动
android:scrollbars = "vertical"
2.水平滑动
android:scrollbars = "horizontal"
android:scrollbars="none"
必须在Java添加(否则无法滑动)
ArrowKeyMovementMethod.getInstance()
tx.setMovementMethod(ScrollingMovementMethod.getInstance()); /垂直
要加:android:ellipsize=“none” android:singleLine=“true” 3.设置滚动条一直存在
android:fadeScrollbars="false"
4.自定义滚动条属性
android:scrollbarThumbVertical="@drawable/bar"
5.跑马灯:
android:singleLine="true"
android:ellipsize="marquee"
https://blog.csdn.net/beiminglei/article/details/9317997
//设置字符宽度(其实em是一个印刷排版的单位,表示字宽的单位)为10,
//一般一个汉字为一个宽度,
android:maxEms="10"
//值为1-5时,m = n..
6-11时,m = n+1。
12-18时,m = n+2。
【31.防止快速点击】
private static long lastClick = 0;
private boolean fastClick() {
if (System.currentTimeMillis() - lastClick <= 1000) {
return false;
}
lastClick = System.currentTimeMillis();
return true;
}
【32.EditText 输入内容】
代码中
editText.setInputType(8194);
android:digits="1234567890." ==== editText.setInputType(EditorInfo.TYPE_CLASS_PHONE);
InputType.TYPE_CLASS_NUMBER 数字类型 1234567890
InputType.TYPE_CLASS_DATETIME 日期类型 1234567890/-
editText.setKeyListener(DigitsKeyListener.getInstance("0123456789-"));
android:inputType="none"--输入普通字符
android:inputType="text"--输入普通字符
android:inputType="textCapCharacters"--输入普通字符
android:inputType="textCapWords"--单词首字母大小
android:inputType="textCapSentences"--仅第一个字母大小
android:inputType="textAutoCorrect"--前两个自动完成
android:inputType="textAutoComplete"--前两个自动完成
android:inputType="textMultiLine"--多行输入
android:inputType="textImeMultiLine"--输入法多行(不一定支持)
android:inputType="textNoSuggestions"--不提示
android:inputType="textUri"--URI格式
android:inputType="textEmailAddress"--电子邮件地址格式
android:inputType="textEmailSubject"--邮件主题格式
android:inputType="textShortMessage"--短消息格式
android:inputType="textLongMessage"--长消息格式
android:inputType="textPersonName"--人名格式
android:inputType="textPostalAddress"--邮政格式
android:inputType="textPassword"--密码格式
android:inputType="textVisiblePassword"--密码可见格式
android:inputType="textWebEditText"--作为网页表单的文本格式
android:inputType="textFilter"--文本筛选格式
android:inputType="textPhonetic"--拼音输入格式
android:inputType="number"--数字格式
android:inputType="numberSigned"--有符号数字格式
android:inputType="numberDecimal"--可以带小数点的浮点格式
android:inputType="phone"--拨号键盘
android:inputType="datetime"
android:inputType="date"--日期键盘
android:inputType="time"--时间键盘
EditText有默认高度直接设置setHeight没作用。
LinearLayout.LayoutParams layoutParams = new LinearLayout.LayoutParams(LinearLayout.LayoutParams.WRAP_CONTENT,
DensityUtil.dip2px(context, 25));
layoutParams.setMargins(0, 0, 0, 0);
editText.setIncludeFontPadding(false);
editText.setFilters(new InputFilter[]{new InputFilter.LengthFilter(10)});
【kotlin版:editText.filters = arrayOf(InputFilter.LengthFilter(24))】
editText.setInputType(InputType.TYPE_CLASS_NUMBER);
editText.setTextColor(context.getResources().getColor(R.color.color_888888));
editText.setPadding(10, 0, 0, 1);
editText.setLayoutParams(layoutParams);
editText.setBackground(null);
样式:
android:background="@null"//光标的样式没有改变,下划线消失了,边距也没有了
游标样式
<EditText
android:text=" "
android:textCursorDrawable="@drawable/color_cursor"
将textCursorDrawable设置为@null,表示去除系统默认的样式
隐藏光标的属性是android:cursorVisible,光标的颜色是跟文字android:textColor保持一致
sendText.setSelection(sendText.getText().length());
sendText.setSelection(0, sendText.getText().length());
下划线修改样式:https://www.jb51.net/article/121010.htm
<style name="MyEditText" parent="Theme.AppCompat.Light">
<item name="colorControlNormal">@color/indigo</item>
<item name="colorControlActivated">@color/pink</item>
</style>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Hint text"
android:theme="@style/MyEditText"/>
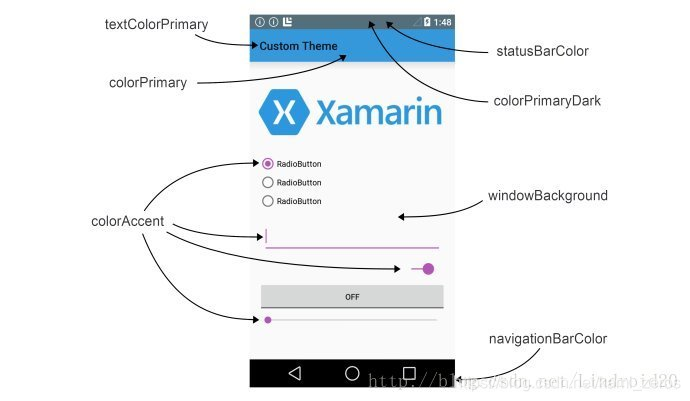
et_underline_unselected.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:bottom="0dp"
android:left="-2dp"
android:right="-2dp"
android:top="-2dp">
<shape>
<solid android:color="@android:color/transparent" />
<stroke
android:width="1dp"
android:color="@android:color/darker_gray" />
<padding android:bottom="4dp" />
</shape>
</item>
</layer-list>
et_underline_selected.xml
<?xml version="1.0" encoding="utf-8"?>
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<item
android:bottom="0dp"
android:left="-2dp"
android:right="-2dp"
android:top="-2dp">
<shape>
<solid android:color="@android:color/transparent" />
<stroke
android:color="@android:color/holo_green_light"
android:width="2dp" />
<padding android:bottom="4dp" />
</shape>
</item>
</layer-list>
<EditText
android:id="@+id/editText1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:background="@null"
android:hint="自定义EditText下划线1"
android:textCursorDrawable="@drawable/cursor_color" />
editText1.setBackgroundResource(R.drawable.et_underline_unselected);
editText1.setOnFocusChangeListener(new View.OnFocusChangeListener() {
@Override
public void onFocusChange(View v, boolean hasFocus) {
if (hasFocus) {
Log.e(TAG, "EditText1获得焦点");
editText1.setBackgroundResource(R.drawable.et_underline_selected);
} else {
Log.e(TAG, "EditText1失去焦点");
editText1.setBackgroundResource(R.drawable.et_underline_unselected);
}
}
});
et_underline_selector.xml
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_focused="false" android:drawable="@drawable/et_underline_unselected"/>
<item android:state_focused="true" android:drawable="@drawable/et_underline_selected"/>
</selector>
<EditText
android:id="@+id/editText1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="3dp"
android:background="@drawable/et_underline_selector"
android:hint="自定义EditText下划线1"
android:textCursorDrawable="@drawable/cursor_color" />
cursor_color.xml
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<size android:width="2dp" />
<solid android:color="@android:color/holo_blue_light" />
</shape>
【33.AndroidBase64加解密】
Android项目引用不到以下两个java类
import sun.misc.BASE64Decoder;
import sun.misc.BASE64Encoder;
Android有自己的base64类
import android.util.Base64
String str = "Hello!";
String strBase64 = Base64.encodeToString(str.getBytes(), Base64.DEFAULT);
String str2 = new String(Base64.decode(strBase64.getBytes(), Base64.DEFAULT));
【34.Java中字符串拼接-删除最后一个字符】
str.split("\|"); //转义字符
StringBuilder builder = new StringBuilder();
for (int i = 0; i < 5; i++) {
builder.append("aaa").append(",");
}
System.out.println(builder.toString());
if (builder.length() <= 0) {
return;
}
if ("".contentEquals(time)) {
return;
}
String aaa = builder.substring(0, builder.length() - 1);
System.out.println(aaa);
builder = builder.deleteCharAt(builder.length() - 1);
System.out.println(builder.toString());
builder = builder.deleteCharAt(builder.lastIndexOf(","));
System.out.println(builder);
builder.setLength(builder.length() - 1);
System.out.println(builder);
StringJoiner joiner = new StringJoiner(",");
for (int i = 0; i < 5; i++) {
joiner.add("bbb");
}
System.out.println(joiner);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 5; i++) {
sb.append(",").append(i);
}
sb = sb.deleteCharAt(0);
System.out.println(sb);
原始:aaa,aaa,aaa,aaa,aaa,
substring:aaa,aaa,aaa,aaa,aaa
deleteCharAt + length:aaa,aaa,aaa,aaa,aaa
deleteCharAt + lastIndexOf:aaa,aaa,aaa,aaaaaa
setLength:aaa,aaa,aaa,aaaaa
Joiner:bbb,bbb,bbb,bbb,bbb
前缀:0,1,2,3,4
【JS中字符串截取】
var str = 'Hello World!';
str=str.slice(0,str.length-1)
str=str.substr(0,str.length-1)
str=str.substring(0,str.length-1)
str=str.substring(0, str.lastIndexOf('!'));
str = str.substr(1);
【35.日期字符串比较大小】
public static boolean compareNowDate1(String t1, String t2) {
int result = t1.compareTo(t2);
return result > 0;
}
public static boolean compareNowDate1(String t1, String t2) {
SimpleDateFormat format = new SimpleDateFormat("yyyyMMdd");
try {
Date d1 = format.parse(t1);
Date d2 = format.parse(t2);
long result = d1.getTime() - d2.getTime();
return result > 0;
} catch (ParseException e) {
e.printStackTrace();
}
return false;
}
public static boolean compareNowDate1(String t1, String t2) {
int int1 = Integer.parseInt(t1);
int int2 = Integer.parseInt(t2);
int result = int1 - int2;
return result > 0;
}
【番外:删除git账号】
[生成公钥:ssh-keygen -t rsa -c “xxxx@xxx.com”]三次回车 git 提取项目Authentication failed 和Incorrect username or password ( access token )的问题 接着怎么提取,都是这样,也不是会再次提示输入账号和密码; 解决办法: 1.控制面板进入用户账户 2.点击管理windows凭据 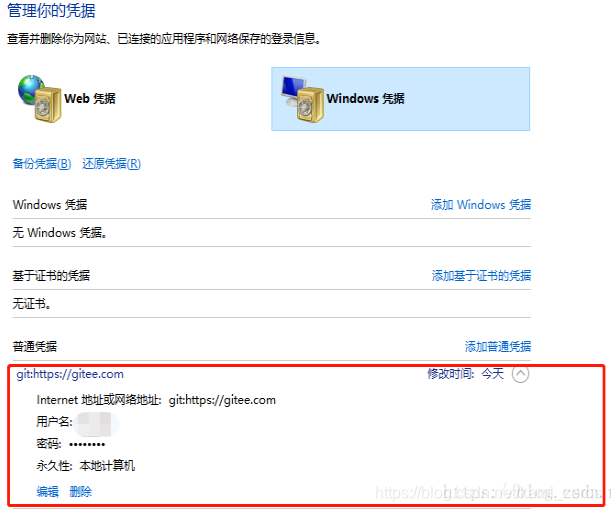
【36.隐藏App界面及图标】
<application
...
android:theme="@android:style/Theme.NoDisplay">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
<!--无启动页面-->
<data
android:host=".app"
android:pathPrefix="/openwith"
android:scheme="myapp" />
</intent-filter>
</activity>
</application>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
...
android:theme="@android:style/Theme.NoDisplay">
</LinearLayout>
【39.绘制view宽高】
https://blog.csdn.net/chenbaige/article/details/77991594 在activity中获取view 的尺寸大小(宽度和高度),若只写getWidth()、getHeight()等方法是获取不到View的宽度和高度大小的 疑问解答: 实际情况下,View的measure过程和Activity的生命周期方法不是同步执行的,因此无法保证Activity在执行完某个生命周期方法时View已经测量完毕了,这种情况下,获取到的尺寸大小就是0。
@Override
public void onWindowFocusChanged(boolean hasFocus) {
super.onWindowFocusChanged(hasFocus);
if (hasFocus) {
int width = bbb.getMeasuredWidth();
int height = bbb.getMeasuredHeight();
ZLog.e("1.view宽:" + width + " view高:" + height);
}
}
view.post(new Runnable() {
@Override
public void run() {
int width = bbb.getMeasuredWidth();
int height = bbb.getMeasuredHeight();
ZLog.e("2.view宽:" + width + " view高:" + height);
}
});
ViewTreeObserver observer = view.getViewTreeObserver();
observer.addOnGlobalLayoutListener(new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
view.getViewTreeObserver().removeOnGlobalLayoutListener(this);
int width = view.getMeasuredWidth();
int height = view.getMeasuredHeight();
ZLog.e("3.view宽:" + width + " view高:" + height);
aaa.setMaxWidth(widthP - width - 10);
}
});
int widthV = View.MeasureSpec.makeMeasureSpec(0, View.MeasureSpec.EXACTLY);
int heigtV = View.MeasureSpec.makeMeasureSpec(0, View.MeasureSpec.EXACTLY);
bbb.measure(widthV, heigtV);
int width1 = bbb.getMeasuredWidth();
int height1 = bbb.getMeasuredHeight();
ZLog.e("4.view宽:" + width1 + " view高:" + height1);
int width22 = View.MeasureSpec.makeMeasureSpec((1 << 30) - 1, View.MeasureSpec.AT_MOST);
int height22 = View.MeasureSpec.makeMeasureSpec((1 << 30) - 1, View.MeasureSpec.AT_MOST);
bbb.measure(width22, height22);
int height222 = bbb.getMeasuredHeight();
int width222 = bbb.getMeasuredWidth();
ZLog.e("5.view宽:" + width222 + " view高:" + height222);
【我用的此】
int spec = View.MeasureSpec.makeMeasureSpec(0, View.MeasureSpec.UNSPECIFIED);
bbb.measure(spec, spec);
int width11 = bbb.getMeasuredWidth();
int height11 = bbb.getMeasuredHeight();
ZLog.e("4.1 view宽:" + width11 + " view高:" + height11);
【40.基本类型转字符串】
float+“”这个有个+号,是在常量池里操作的,会生成两个。所以 String.valueOf(float)相对好一点。 另外:+与StringBuider的对比 https://mp.weixin.qq.com/s/dc7HW0SqEcJknMIqoQZnlg
【41.TextView变色】
https://weilu.blog.csdn.net/article/details/52863741
text.setText(Html.fromHtml("商品编码<font color=red>*</font>:"));
text.setText(Html.fromHtml(String.format("商品编码<font color=red>*</font>:%s","22")));
SpannableStringBuilder builder = new SpannableStringBuilder(bar_code);
int index = bar_code.indexOf(etScan);
builder.setSpan(new ForegroundColorSpan(Color.RED), index,
index + etScan.length(), Spannable.SPAN_EXCLUSIVE_EXCLUSIVE);
textView.setText(builder);
android:autoLink 设置是否当文本为URL链接/email/号码/map时,文本显示为可点击的链接。
可选值:none/web/Email/phone/map/all
android:lineSpacingExtra="8dp"
lineSpacingMultiplier属性,它代表行间距倍数,默认为1.0f,是一个相对高度值。
android:text="揽件方式:上门取件\n快递公司:顺丰快递"
【42.限制EditText输入的小数的位数】
①xml中限制输入的类型:
android:inputType="numberDecimal"
②重写InputFilter
public class DecimalDigitsInputFilter implements InputFilter {
private final int decimalDigits;
public DecimalDigitsInputFilter(int digits) {
this.decimalDigits = digits;
}
@Override
public CharSequence filter(CharSequence source, int start, int end, Spanned dest, int dstart, int dend) {
if (dest.length() == 0 && source.equals(".")) {
return "0.";
}
String dValue = dest.toString();
String[] splitArray = dValue.split("\\.");
if (splitArray.length > 1) {
String dotValue = splitArray[1];
if (dotValue.length() == decimalDigits && dest.length() - dstart <= decimalDigits) {
return "";
}
}
return null;
}
}
③设置小数点位数,这里传进去的是2位
edit.setFilters(new InputFilter[]{new DecimalDigitsInputFilter(2)});
【TextView代码设置展开与省略】
textView.setOnClickListener(v -> {
if (isShowDes) {
textView.setEllipsize(TextUtils.TruncateAt.END);
textView.setSingleLine();
} else {
textView.setEllipsize(null);
textView.setSingleLine(false);
}
isShowDes = !isShowDes;
});
|