效果
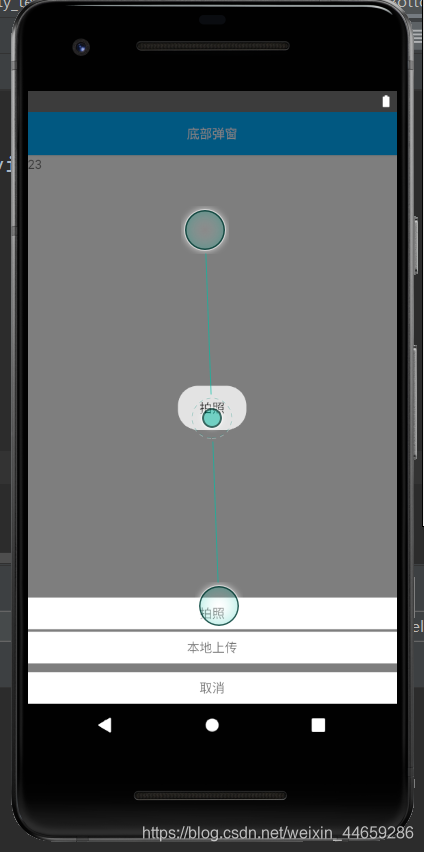
PopWinBottomLayout
package com.coral3.common_module.components;
import android.app.Activity;
import android.content.Context;
import android.os.Handler;
import android.os.Message;
import android.util.AttributeSet;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.FrameLayout;
import android.widget.LinearLayout;
import android.widget.PopupWindow;
import android.widget.TextView;
import androidx.annotation.Nullable;
import com.coral3.common_module.R;
import com.coral3.common_module.utils.WinManagerUtil;
import java.util.HashMap;
import java.util.Map;
public class PopWinBottomLayout extends LinearLayout implements View.OnClickListener{
private Context mContext;
private TextView tvCancel, takePhoto, localPhoto;
private PopupWindow window;
private View view;
private Boolean popWinIsShow = false;
private ICallback mICallback;
private FrameLayout bgView;
private Handler handler = new Handler(new Handler.Callback(){
@Override
public boolean handleMessage(Message message) {
if(message.what == 0x00) popWinIsShow = false;
return false;
}
});
public PopWinBottomLayout(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
mContext = context;
initView();
initPop();
initLisener();
}
private void initView(){
view = LayoutInflater.from(mContext).inflate(R.layout.layout_pop_window_bottom, null);
tvCancel = view.findViewById(R.id.tv_cancel);
takePhoto = view.findViewById(R.id.tv_take_photo);
localPhoto = view.findViewById(R.id.tv_local_photo);
}
private void initLisener(){
window.setOnDismissListener(new PopupWindow.OnDismissListener() {
@Override
public void onDismiss() {
if(bgView != null) bgView.setVisibility(View.GONE);
handler.sendEmptyMessageDelayed(0x00, 100);
}
});
tvCancel.setOnClickListener(this);
takePhoto.setOnClickListener(this);
localPhoto.setOnClickListener(this);
}
private void initPop(){
window = new PopupWindow(this);
window.setContentView(view);
window.setHeight(ViewGroup.LayoutParams.WRAP_CONTENT);
window.setWidth(WinManagerUtil.getWidth((Activity) mContext));
window.setOutsideTouchable(true);
}
public void showPopView(){
if(this != null && window != null){
window.showAtLocation(this, Gravity.BOTTOM,0,0);
popWinIsShow = true;
if(null != bgView) bgView.setVisibility(View.VISIBLE);
}
}
public void hidePopView(){
if(window != null){
window.dismiss();
popWinIsShow = false;
if(bgView != null) bgView.setVisibility(View.GONE);
}
}
public Boolean getPopWinStatus(){
return popWinIsShow;
}
public void setICallback(ICallback iCallback){
mICallback = iCallback;
}
@Override
public void onClick(View view) {
int id = view.getId();
if(id == R.id.tv_take_photo){
if(null != mICallback) mICallback.onItemClick(Click.TAKE_PHOTO.getCode(), Click.TAKE_PHOTO.getName());
}else if(id == R.id.tv_local_photo){
if(null != mICallback) mICallback.onItemClick(Click.LOCAL_UPLOAD.getCode(), Click.LOCAL_UPLOAD.getName());
}
if(id == R.id.tv_cancel) hidePopView();
}
public interface ICallback{
void onItemClick(int pos, String name);
}
public void setBg(FrameLayout bg){
bgView = bg;
}
public enum Click {
TAKE_PHOTO(0, "拍照"), LOCAL_UPLOAD(1, "本地上传");
private final Integer code;
private final String name;
public Integer getCode() {
return code;
}
public String getName() {
return name;
}
Click(Integer code, String name) {
this.code = code;
this.name = name;
}
private static Map<Integer, Click> KEY_MAP = new HashMap<>();
static {
for (Click value : Click.values()) {
KEY_MAP.put(value.getCode(), value);
}
}
public static Click getType(Integer code) {
return KEY_MAP.get(code);
}
}
}
TestActivity
package com.coral3.ah.ui;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.FrameLayout;
import android.widget.LinearLayout;
import com.coral3.ah.R;
import com.coral3.common_module.components.PopWinBottomLayout;
import com.coral3.common_module.utils.ToastUtil;
public class TestActivity extends AppCompatActivity implements View.OnClickListener, PopWinBottomLayout.ICallback{
private Button btnPopButton;
private PopWinBottomLayout popWinBottomLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_test);
initView();
initListener();
}
private void initView(){
btnPopButton = findViewById(R.id.btn_pop_bottom);
popWinBottomLayout = findViewById(R.id.pop_win_bottom);
popWinBottomLayout.setBg(findViewById(R.id.fl_bg));
}
private void initListener(){
btnPopButton.setOnClickListener(this);
popWinBottomLayout.setICallback(this);
}
@Override
public void onClick(View view) {
int id = view.getId();
if(id == R.id.btn_pop_bottom) {
if(popWinBottomLayout.getPopWinStatus()){
popWinBottomLayout.hidePopView();
}else{
popWinBottomLayout.showPopView();
}
}
}
@Override
public void onItemClick(int pos, String name) {
ToastUtil.showMsg(this, name);
}
}
custom_pop_bottom_item.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="vertical"
android:gravity="center_vertical"
android:layout_height="wrap_content">
<TextView
android:id="@+id/tv_divide"
android:layout_width="match_parent"
android:background="#ffffff"
android:layout_height="1dp"/>
<TextView
android:id="@+id/tv_fruit"
android:gravity="center"
android:padding="10dp"
android:layout_weight="1"
android:textColor="#fff"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
activity_test.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".ui.TestActivity">
<FrameLayout
android:id="@+id/fl_bg"
android:visibility="gone"
android:background="#000"
android:alpha="0.5"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
<LinearLayout
android:id="@+id/ll_container"
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="wrap_content">
<Button
android:text="底部弹窗"
android:id="@+id/btn_pop_bottom"
android:background="@color/theme_color"
android:textColor="@color/white"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<TextView
android:text="23"
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
<com.coral3.common_module.components.PopWinBottomLayout
android:id="@+id/pop_win_bottom"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
</RelativeLayout>
|