权限
- 内容提供器作用:
不同与文件与SharedPreferences的全局可读写,内容提供器可选择只对哪一部分进行共享 - 现有提供器读取和操作数据,创建提供器给数据提供外部接口
普通权限和危险权限
- 普通:不威胁用户安全和隐私,系统自动授权;
- 危险:用户手动授权,运行时权限看起来用的是权限名,实际对应权限组的其他权限也会同时被授权
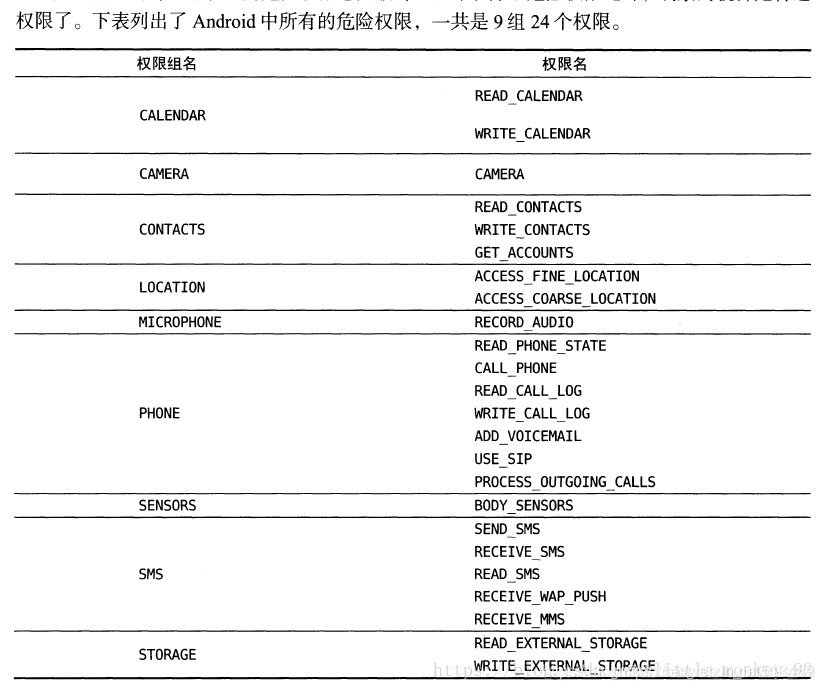
在程序运行时申请权限
public void onClick(View v){
if(ContextCompat.checkSelfPermission(MainActivity.this,Manifext.permission.CALL_PHONE)!=PackageManager.PERMISSION_GRANTED){
ActivityCompat.requestPermissions(MainActivity.this,new String[]{Manifest.permission.CALL_PHONE},1);
}else{
call();
}
}
private void call(){
try{
Intent intent=new Intent(Intent.ACTION_CALL);
intent.setData(Uri.parse("tel:10086"));
startActivity(intent);
}catch{
e.printSatckTrace();
}
}
public void onRequestPermissionResult(int requestCode,String[] permissions,int[] grantResults){
switch(requestCode){
case 1:
if(grandeResults.length>0&&grandsResults[0]==PackageManager.PERMISSION_GRANTED){
call();
}else{
Toast.makeText(this,"you denied the permission",Toast.LENGTH_SHORT).show();
}
break;
default;
}
}
}
<uses-permission android:name="android.permission.CALL_PHONE"/>
访问其他程序中的数据
ContentResolver
Uri
content://com.example.app.provider/table1
content://com.example.app.provider/table2
- Uri是提供器唯一的标识符,ContentResolver 以Uri 作为唯一参数,传入时要解析成Uri对象
Uri uri=Uri.parse("content://com.example.app.provider/table");
查询
Cursor cursor=getContentResolver().query(
uri,
projection,
selection,
selectionArgs,
sortOrder);
if(cursor!=null){
while(cursor.moveText()){
String column1=cursor.getString(cursor.getColumnIndex("column1"));
int colunm2=cursor.getInt(cursor.getColumnIndex("column2"));
}
cursor.close();
}
增加
ContentValues values=new ContentValues();
values.put("column1","text");
values.put("column2",1);
getContentResolver().insert(uri,values);
改变
ContentValues values=new ContentValues();
values.put("column1","");
getContentResolver().update(uri,values,"column1=? and column2=?",new String[]{"text","1"});
删除
getContentResolver().detele(uri,"column2=?",new String[]{"1"});
创建内容提供器
*:表示匹配 任意长度 的 任意字符
#:表示匹配 任意长度数字
vnd.android.cursor.dir/vnd.com.example.app.provider.table1
vnd.android.cursor.item/vnd.com.example.app.provider.table1
onCreate()
public boolean onCreate(){
return false;
}
query()
- 5个参数:uri,projection,selection,selectionArgs,sortOrder
public Cursor query(Uri uri,String[] projection,String selection,String[] selectionArgs,String sortOrder){
return null;
}
insert
public Uri insert(Uri uri,ContentValues values){
return null;
}
update
- 4个参数:uri,values,selection,selectionArgs
public int update(Uri uri,ContentValues values,String selection,String[] selectionArgs){
return 0;
}
delete
- 3个参数:uri,selection,selectionArgs
public int delete(Uri uri,String selection,String[] selectioArgs){
return 0;
}
getType()
public class MyProvider extends ContentProvider{
...
public String getType(Uri uri){
switch(uriMatcher.match(uri)){
case TABLE1_DIR:
return "vnd.android.cursor.dir/vnd.com.example.app.provider.table1";
case TABLE1_ITEM:
return "vnd.android.cursor.item/vnd.com.example.app.provider.table1";
case TABLE2_DIR:
return "vnd.android.cursor.dir/vnd.com.example.app.provider.table2"
case TABLE2_ITEM:
"vnd.android.cursor.item/vnd.com.example.app.provider.table2"
default:
break
}
return null;
}
}
跨程序访问数据
- 相当于以上知识的一次总结运用,虽然代码很长,但是内容简单,有很多相似的部分~
public class DatbaseProvider extends ContentProvider{
public static final int BOOK_DIR=0;
public static final int BOOK_ITEM=1;
public static final int CATEGORY_DIR=2;
public static final int CATEGORY_ITEM=3;
public static final String AUTHORITY="com.example.databasetest.provider";
private static UriMatcher uriMatcher;
private MyDatabaseHelper dbHelper;
static {
uriMatcher =new UriMatcher(UriMatcher.NO_MATCH);
uriMatcher.addURI(AUTHORITY,"book",BOOK_DIR);
uriMatcher.addURI(AUTHORITY,"book/#",BOOK_ITEM);
uriMatcher.addURI(AUTHORITY,"category",CATEGORY_DIR);
uriMatcher.addURI(AUTHORITY,"category/#",CATEGORY_ITEM);
}
public boolean onCreate(){
dbHelper =new MyDatabaseHelper(getContext(),"BookStore.db",null,2);
return true;
}
public Cursor query(Uri uri,String[] projection,String selection,String selectionArgs,String sortOrder){
SQLiteDatabase sb=dbHelper.getReadableDatabase();
Cursor cursor=null;
switch(uriMatcher.match(uri)){
case BOOK_DIR:
cursor=db.query("Book",projection,selection,selectionArgs,null,null,sortOrder);
break;
case BOOK_ITEM:
String bookId=uri.getPathSegments().get(1);
cursor=db.query("Book",projection,"id=?",new String[]{bookId},null,null,sortOrder);
break;
case CATEGORY_DIR:
cursor=db.query("Category",projection,selection,selectionArgs,null,null,sortOrder);
break;
case CATEGORY_ITEM:
cursor=db.query("Category",projection,"id=?".new String[]{categotyId},null,null,sortOrder);
break;
default:
break;
}
return cursor;
}
public Uri insert(Uri uri,ContentValues values){
SQLiteDatabase db=dbHelper.getWritableDatabase();
Uri uriReturn=null;
switch(uriMatcher.match(uri)){
case BOOK_DIR:
case BOOK_ITEM:
long newBookId=db.insert("Book",null,values);
uriReturn =Uri.parse("content://"+AUTHORITY+"/book/"+newBookId);
break;
case CATEGORY_DIR:
case CATEGORY_ITEM:
long newCategoryId=db.insert("Category",null,values);
uriReturn=Uri.parse("content://"+AUTHORITY+"/category/"+newCategoryId);
break;
default:
break
}
return uriReturn;
}
public int update(Uri uri,ContentValues values,String selection,String[] selectionArgs){
SQLiteDatabase db=dbHelper.getWritableDatabase();
int updatedRows;
switch(uriMatcher.match(uri)){
case BOOK_DIR:
updateRows=db.update("Book",values,selection,selctionArgs);
break;
case BOOK_ITEM:
String bookId=uri.getPathSegments().get(1);
updateRows=db.update("Book",values,"id=?",new String[]{bookId});
case CATEGORY_DIR:
updateRows=db.update("Category",values,selection,selectionArgs);
break;
case CATEGORY_ITEM:
String categoryId=uri.getPathSegments().get(1);
updateRows=db.update("Category",values,"id=?",new String[]{categoryId});
break;
default:
break;
}
return updateRows;
}
public int delete(Uri uri,String selection,String[] selectionArgs){
SQLiteDatabase db=dbHelper.getWritableDatabase();
int updatedRows;
switch(uriMatcher.match(uri)){
case BOOK_DIR:
updateRows=db.delete("Book",selection,selctionArgs);
break;
case BOOK_ITEM:
String bookId=uri.getPathSegments().get(1);
updateRows=db.delete("Book","id=?",new String[]{bookId});
break;
case CATEGORY_DIR:
updateRows=db.delete("Category",selection,selectionArgs);
break;
case CATEGORY_ITEM:
String categoryId=uri.getPathSegments().get(1);
updateRows=db.delete("Category","id=?",new String[]{categoryId});
break;
default:
break;
}
return updateRows;
}
public String getType(Uri uri){
switch(uriMatcher.match(uri)){
case TABLE1_DIR:
return "vnd.android.cursor.dir/vnd.com.example.databasetest.provider.table1";
case TABLE1_ITEM:
return "vnd.android.cursor.item/vnd.com.example.databasetest.provider.table1";
case TABLE2_DIR:
return "vnd.android.cursor.dir/vnd.com.example.databasetest.provider.table2"
case TABLE2_ITEM:
return "vnd.android.cursor.item/vnd.com.example.databasetest.provider.table2"
default:
break
}
return null;
}
}
Git进阶版
忽略文件
/src/test
/src/androidTest
查看修改内容
git status
git diff
git diff app/src/main/java/com/example/providertest/ManiActivity.java
撤销未提交修改
git reset HEAD app/src/main/java/com/example/providertest/MainActivity.java
查看提交记录
git log
git log 1fa380b..... -1
git log 1fa380b..... -1 -p
这篇博客分享了Android里的权限机制,学习了如何申请权限,如何访问其他程序的数据,如何建内容提供器共享数据~~小小浅谈,如果对你有用,感谢您百忙中点个赞~?
|