请求对象:StringRequest,JsonObjectRequest,JsonAarryRequest,ImageRequest
步骤:
1.创建一个请求队列RequestQueue
2.创建Request对象
3.将请求对象添加到请求队列中
具体实现:
1.在AndroidManifest.xml中添加网络访问权限:
<uses-permission android:name="android.permission.INTERNET" />
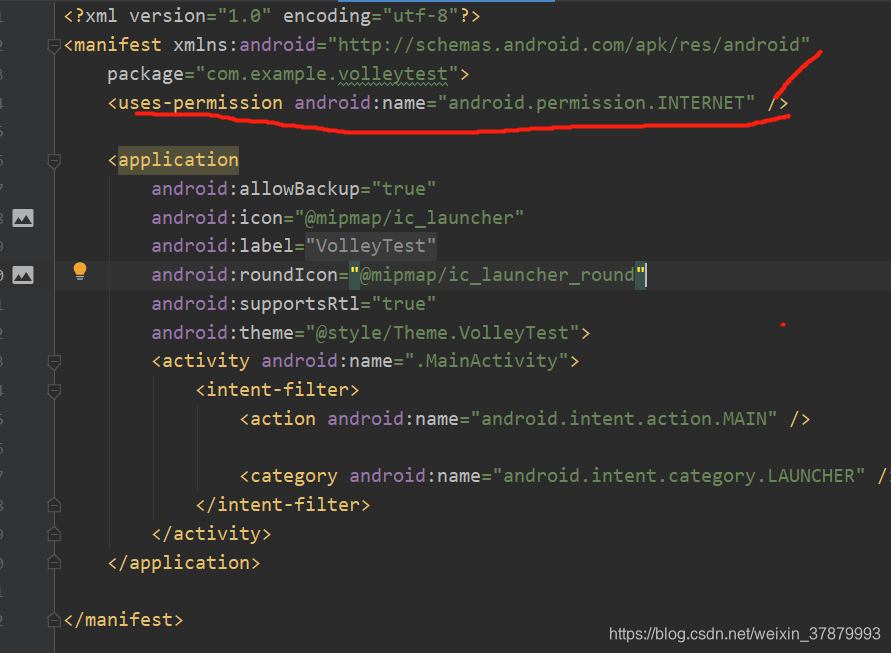
?2.在build.gradle:app中添加volley依赖
dependencies {
...
implementation 'com.android.volley:volley:1.1.1'
}
?3.在activity_main中绘制简单的UI
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical">
<ImageView
android:id="@+id/action_image"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@mipmap/ic_launcher"/>
<TextView
android:id="@+id/tv_image_url"
android:layout_width="match_parent"
android:text="请输入图片路径"
android:layout_height="50dp"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="加载网络图片"/>
</LinearLayout>
UI效果如图:
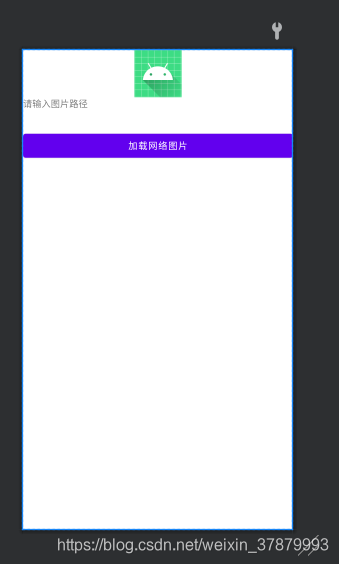
3.在MainActivity.kt(我用kotlin不是java)中使用volley框架发起请求
这是加载网络图片的方法
//加载网络图片
private fun loadImage(tv:EditText,iv:ImageView){
//创建网络队列请求
val requestQueue = Volley.newRequestQueue(this)
//获取图片url
val url = tv.text.toString()
//创建请求
val imageRequest = ImageRequest(url,Response.Listener<Bitmap>() {response ->
iv.setImageBitmap(response)
},0,0,Bitmap.Config.RGB_565,Response.ErrorListener{error->
Toast.makeText(this,"网络错误,$error",Toast.LENGTH_LONG).show()
})
//把imageRequest请求放到网络请求队列中
requestQueue.add(imageRequest)
}
所有的MainActivity.kt代码为:
class MainActivity : AppCompatActivity(), View.OnClickListener {
private lateinit var iv :ImageView
private lateinit var tv :EditText
private lateinit var bt :Button
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
iv = findViewById<ImageView>(R.id.action_image)
tv = findViewById<EditText>(R.id.tv_image_url)
bt = findViewById<Button>(R.id.bt)
bt.setOnClickListener(this)
}
override fun onClick(v: View?) {
loadImage(tv,iv)
}
//加载网络图片
private fun loadImage(tv:EditText,iv:ImageView){
//创建网络队列请求
val requestQueue = Volley.newRequestQueue(this)
//获取图片url
val url = tv.text.toString()
//创建请求
val imageRequest = ImageRequest(url,Response.Listener<Bitmap>() {response ->
iv.setImageBitmap(response)
},0,0,Bitmap.Config.RGB_565,Response.ErrorListener{error->
Toast.makeText(this,"网络错误,$error",Toast.LENGTH_LONG).show()
})
//把imageRequest请求放到网络请求队列中
requestQueue.add(imageRequest)
}
}
|