所有学习flutter的人应该都要经过 Flutter中文网的吧
于是以下内容就中文网中的步骤记录一下自己图中遇到的问题以及解决方案。
配置环境
- 编程环境:
- 工具:Android Studio
- 操作系统: Windows 10 64位
- Git for Windows (Git命令行工具)
- VPN
- 配置系统环境变量
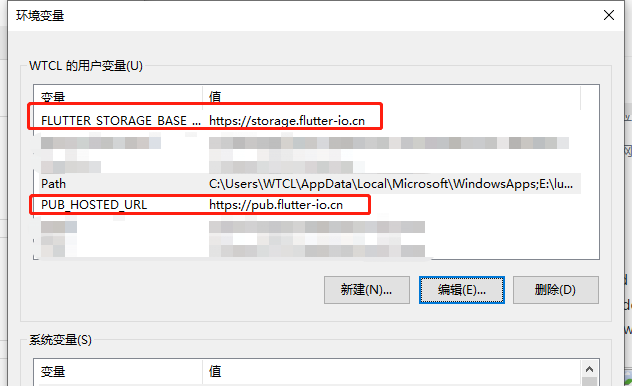
PUB_HOSTED_URL=https://pub.flutter-io.cn
FLUTTER_STORAGE_BASE_URL=https://storage.flutter-io.cn
- 官网 下载Flutter SDK ,解压
- 修改环境变量Path,追加安装fluttersdk的bin目录
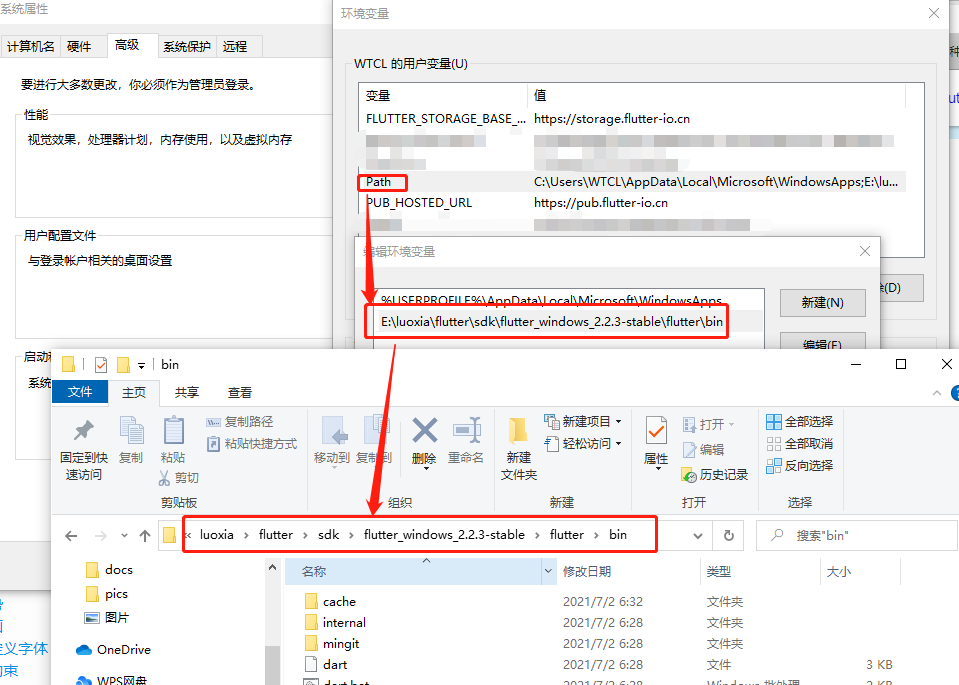 - 重启Windows
- 解压后并找到flutter文件夹中的flutter_console.bat并双击
- 打开cmd ,输入 flutter doctor 命令以检索依赖项是否安装(这个时间可能持续比较久,结果主要看粗体字)
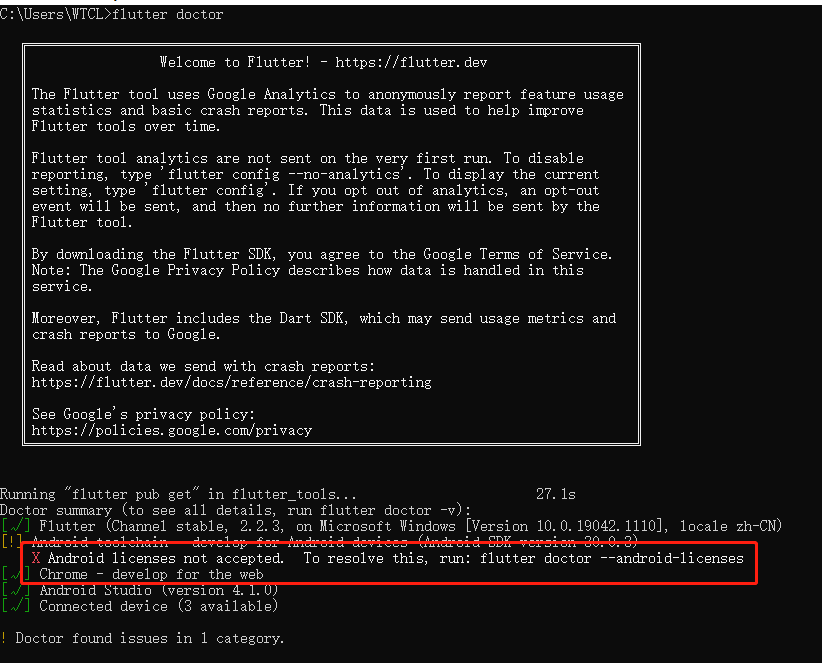
提示未接收Android协议,输入提示的命令一路 y 接收即可。
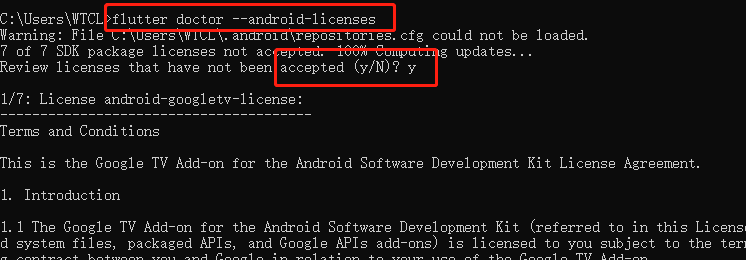
- 所有协议都接受后再次输入 flutter doctor ,让flutter 医生给你检查一下是否安装好
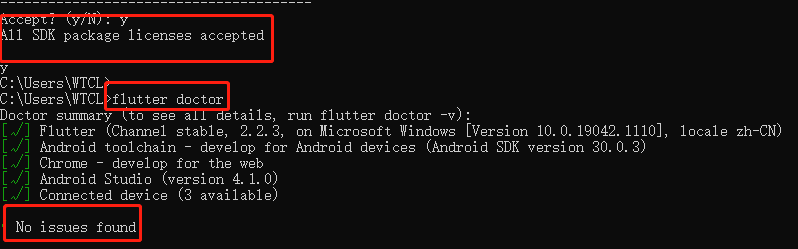
像上面全绿的那样安装ok了。
安装插件
- 在as中,File-Settings-Plugin-搜索Flutter和Dart安装并重启即可
写个简单的flutter应用
- File-New-New Flutter Project 创建一个flutter应用
- 我们目前只需要编写 main.dart 里面的代码即可,pubspec.yaml文件相当于Android项目的build.gradle文件,用于集成一些依赖
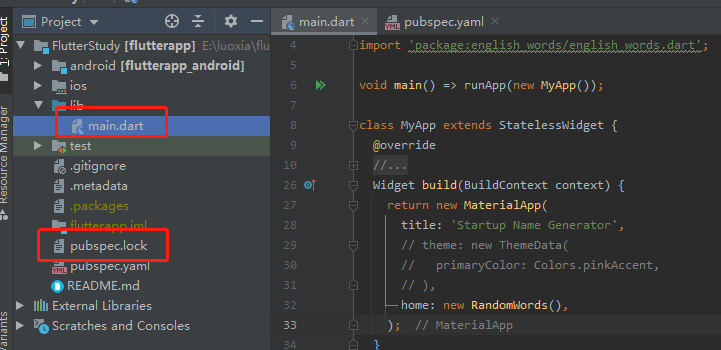 - 我们跟着中文网中的例子编写一个简单应用
// import 'dart:html';
import 'package:flutter/material.dart';
import 'package:english_words/english_words.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
// Widget build(BuildContext context) {
// final wordPair = new WordPair.random();
// return new MaterialApp(
// title: 'Welcome to Flutter',
// home: new Scaffold(
// appBar: new AppBar(
// title: new Text('Welcome to Flutter'),
// ),
// body: new Center(
// // child: new Text('Hello World'),
// // child: new Text(wordPair.asPascalCase),
// child: new RandomWords(),
// ),
// ),
// );
// }
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Startup Name Generator',
// theme: new ThemeData(
// primaryColor: Colors.pinkAccent,
// ),
home: new RandomWords(),
);
}
}
class RandomWords extends StatefulWidget {
@override
createState() => new RandomWordsState();
}
class RandomWordsState extends State<RandomWords> {
@override
Widget build(BuildContext context) {
// final wordPair = new WordPair.random();
// return new Text(wordPair.asPascalCase);
return new Scaffold(
appBar: new AppBar(
title: new Text('Startup Name Generator'),
actions: <Widget>[
new IconButton(icon: new Icon(Icons.list), onPressed: _pushSaved),
],
),
body: _buildSuggestions(),
);
}
void _pushSaved() {
Navigator.of(context).push(
new MaterialPageRoute(
builder: (context) {
final tiles = _saved.map(
(pair) {
return new ListTile(
title: new Text(
pair.asPascalCase,
style: _biggerFont,
),
);
},
);
final divided = ListTile.divideTiles(
context: context,
tiles: tiles,
).toList();
return new Scaffold(
appBar: new AppBar(
title: new Text('Saved Suggestions'),
),
body: new ListView(children: divided),
);
},
),
);
}
final _suggestions = <WordPair>[];
final _biggerFont = const TextStyle(fontSize: 18.0);
final _saved = new Set<WordPair>();
Widget _buildSuggestions() {
return new ListView.builder(
padding: const EdgeInsets.all(16.0),
// 对于每个建议的单词对都会调用一次itemBuilder,然后将单词对添加到ListTile行中
// 在偶数行,该函数会为单词对添加一个ListTile row.
// 在奇数行,该函数会添加一个分割线widget,来分隔相邻的词对。
// 注意,在小屏幕上,分割线看起来可能比较吃力。
itemBuilder: (context, i) {
// 在每一列之前,添加一个1像素高的分隔线widget
if (i.isOdd) return new Divider();
// 语法 "i ~/ 2" 表示i除以2,但返回值是整形(向下取整),比如i为:1, 2, 3, 4, 5
// 时,结果为0, 1, 1, 2, 2, 这可以计算出ListView中减去分隔线后的实际单词对数量
final index = i ~/ 2;
// 如果是建议列表中最后一个单词对
if (index >= _suggestions.length) {
// ...接着再生成10个单词对,然后添加到建议列表
_suggestions.addAll(generateWordPairs().take(10));
}
return _buildRow(_suggestions[index]);
});
}
Widget _buildRow(WordPair pair) {
final alreadySaved = _saved.contains(pair);
return new ListTile(
title: new Text(
pair.asPascalCase,
style: _biggerFont,
),
trailing: new Icon(
alreadySaved ? Icons.favorite : Icons.favorite_border,
color: alreadySaved ? Colors.red : null,
),
onTap: () {
setState(() {
if (alreadySaved) {
_saved.remove(pair);
} else {
_saved.add(pair);
}
});
},
);
}
}
- pubspec.yaml代码如下,注释部分可以忽略
name: flutterapp
description: A new Flutter application.
# The following line prevents the package from being accidentally published to
# pub.dev using `pub publish`. This is preferred for private packages.
publish_to: 'none' # Remove this line if you wish to publish to pub.dev
# The following defines the version and build number for your application.
# A version number is three numbers separated by dots, like 1.2.43
# followed by an optional build number separated by a +.
# Both the version and the builder number may be overridden in flutter
# build by specifying --build-name and --build-number, respectively.
# In Android, build-name is used as versionName while build-number used as versionCode.
# Read more about Android versioning at https://developer.android.com/studio/publish/versioning
# In iOS, build-name is used as CFBundleShortVersionString while build-number used as CFBundleVersion.
# Read more about iOS versioning at
# https://developer.apple.com/library/archive/documentation/General/Reference/InfoPlistKeyReference/Articles/CoreFoundationKeys.html
version: 1.0.0+1
environment:
sdk: ">=2.12.0 <3.0.0"
dependencies:
flutter:
sdk: flutter
# The following adds the Cupertino Icons font to your application.
# Use with the CupertinoIcons class for iOS style icons.
cupertino_icons: ^1.0.2
english_words: ^3.1.0
dev_dependencies:
flutter_test:
sdk: flutter
# For information on the generic Dart part of this file, see the
# following page: https://dart.dev/tools/pub/pubspec
# The following section is specific to Flutter.
flutter:
# The following line ensures that the Material Icons font is
# included with your application, so that you can use the icons in
# the material Icons class.
uses-material-design: true
# To add assets to your application, add an assets section, like this:
# assets:
# - images/a_dot_burr.jpeg
# - images/a_dot_ham.jpeg
# An image asset can refer to one or more resolution-specific "variants", see
# https://flutter.dev/assets-and-images/#resolution-aware.
# For details regarding adding assets from package dependencies, see
# https://flutter.dev/assets-and-images/#from-packages
# To add custom fonts to your application, add a fonts section here,
# in this "flutter" section. Each entry in this list should have a
# "family" key with the font family name, and a "fonts" key with a
# list giving the asset and other descriptors for the font. For
# example:
# fonts:
# - family: Schyler
# fonts:
# - asset: fonts/Schyler-Regular.ttf
# - asset: fonts/Schyler-Italic.ttf
# style: italic
# - family: Trajan Pro
# fonts:
# - asset: fonts/TrajanPro.ttf
# - asset: fonts/TrajanPro_Bold.ttf
# weight: 700
#
# For details regarding fonts from package dependencies,
# see https://flutter.dev/custom-fonts/#from-packages
- 好,然后点击运行,就可以得到一个无限下拉列表,带有收藏和取消收藏,以及收藏列表的功能拉,像这样。
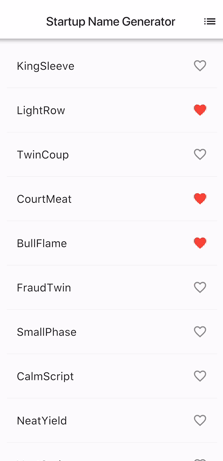
不得不感叹,用flutter写应用是不是都不用ui了?简单的icon 在flutter框架有集成,难一点的自己自定义…
过程中遇到的一些问题
1、报错提示:Error: Not found: 'dart:html’ 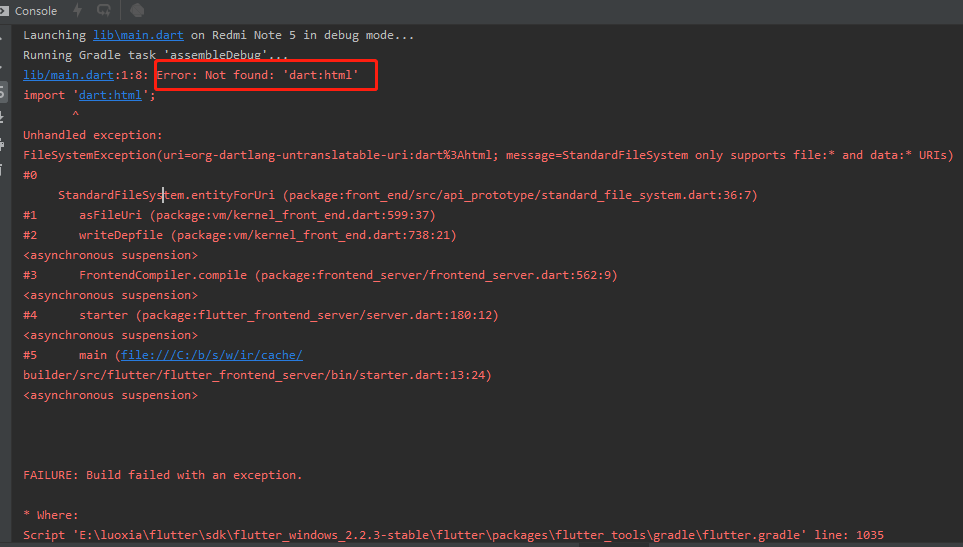
写代码过程中在dart.main中不小心导入了这个html
解决办法:删掉即可
2、报错提示:Error: Cannot run with sound null safety, because the following dependencies don’t support null safety:
版本问题,可以手动回滚版本到旧一点的去,也可以配置一下as,我这里选的是配置as
- as中点击Edit Configuration
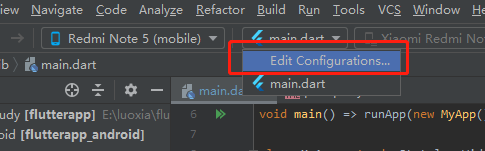 - 在Additional run args选项中输入 –no-sound-null-safety
- ok,再run一下试试
3、如果还遇到其他问题也不要紧,因为Flutter中文网的作者已经把每一步完成后的代码链接给到了我们,对照着看就能找到问题拉。这里不得不为中文网作者点一个大大的赞~~ 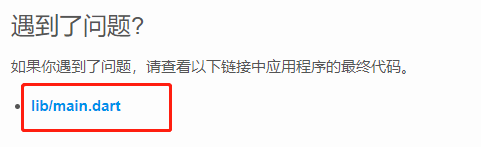
|