先看效果图:
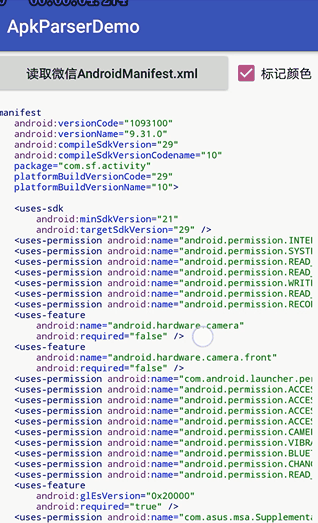
public class FastScrollView extends ScrollView {
private Rect mBarRect = new Rect();
private int mScrollExWidth;
private int mScrollExHeight;
private boolean mScrollStart;
private int dx, dy;
private int mRightPadding;
public FastScrollView(Context context) {
super(context);
init();
}
public FastScrollView(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public FastScrollView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
mScrollExWidth = dip2px(16); //扩展拖动区域宽度,可自行修改
mScrollExHeight = dip2px(50); //扩展拖动区域高度,可自行修改
}
@Override
public boolean dispatchTouchEvent(MotionEvent ev) {
switch (ev.getAction() & ev.getActionMasked()) {
case MotionEvent.ACTION_DOWN:
//Log.e("fly", "down");
mScrollStart = false;
if (canScroll()) {
dx = (int) ev.getX();
dy = (int) ev.getY();
float scrollPos = (float) getHeight() / getChildView().getHeight();
int barHeight = (int) (scrollPos * getHeight());
//Log.e("fly", scrollPos+", "+ barHeight);
mBarRect.left = getWidth() - mRightPadding - getVerticalScrollbarWidth();
mBarRect.right = getWidth() - mRightPadding;
mBarRect.top = (int) (getScrollY() * scrollPos);
mBarRect.bottom = mBarRect.top + barHeight;
//Dbg.print(mBarRect.left, mBarRect.right, mBarRect.top, mBarRect.bottom, dx, dy);
if (dx >= mBarRect.left - mScrollExWidth
&& dx < mBarRect.right
&& dy >= mBarRect.top - mScrollExHeight
&& dy < mBarRect.bottom + mScrollExHeight) {
//Log.e("fly", "mScrollStart");
mScrollStart = true;
}
}
break;
case MotionEvent.ACTION_MOVE:
if (mScrollStart) {
int offsetY = (int) ev.getY() - dy;
//Log.e("fly", "move: " + ev.getY());
int top = mBarRect.top + offsetY;
float scrollPos = (float) top / getHeight();
int scrollY = (int) (scrollPos * getChildView().getHeight());
if (scrollY < 0) {
scrollY = 0;
}
if (scrollY > getChildView().getHeight() - getHeight()) {
scrollY = getChildView().getHeight() - getHeight();
}
setScrollY(scrollY);
return true;
}
break;
}
return super.dispatchTouchEvent(ev);
}
private boolean canScroll() {
View child = getChildView();
if (child != null && child.getHeight() > getHeight()) {
return true;
} else {
return false;
}
}
private View getChildView() {
if (getChildCount() > 0) {
return getChildAt(0);
} else {
return null;
}
}
private int dip2px(float dpValue) {
final float scale = getContext().getResources().getDisplayMetrics().density;
return (int) (dpValue * scale + 0.5f);
}
/**
* 这里不用view的paddding,用于预留特殊空隙
*
* @param mRightPadding
*/
public void setRightPadding(int mRightPadding) {
this.mRightPadding = mRightPadding;
}
}
布局中设置了大滚动条样式,滚动时更加明显:
<com.zwxuf.apkparserdemo.FastScrollView
android:id="@+id/mScrollView"
android:layout_width="0dp"
android:layout_weight="1"
android:scrollbarSize="10dp"
android:paddingRight="10dp"
android:scrollbarThumbVertical="@drawable/fast_scroll_thumb_drawable"
android:layout_height="match_parent">
<HorizontalScrollView
android:layout_width="match_parent"
android:layout_height="match_parent" >
<EditText
android:id="@+id/et_xml"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="@null"
android:editable="false"
android:textColor="#000000"
android:textSize="10sp"
android:typeface="monospace"/>
</HorizontalScrollView>
</com.zwxuf.apkparserdemo.FastScrollView>
同理,可以依此制作快速滚动的 HorizontalScrollView。
|