官方源码
https://github.com/google/charts/tree/master/charts_flutter 文档少
minimumPaddingBetweenLabelsPx 设置间距 BasicNumericTickFormatterSpec 处理横坐标value
效果图
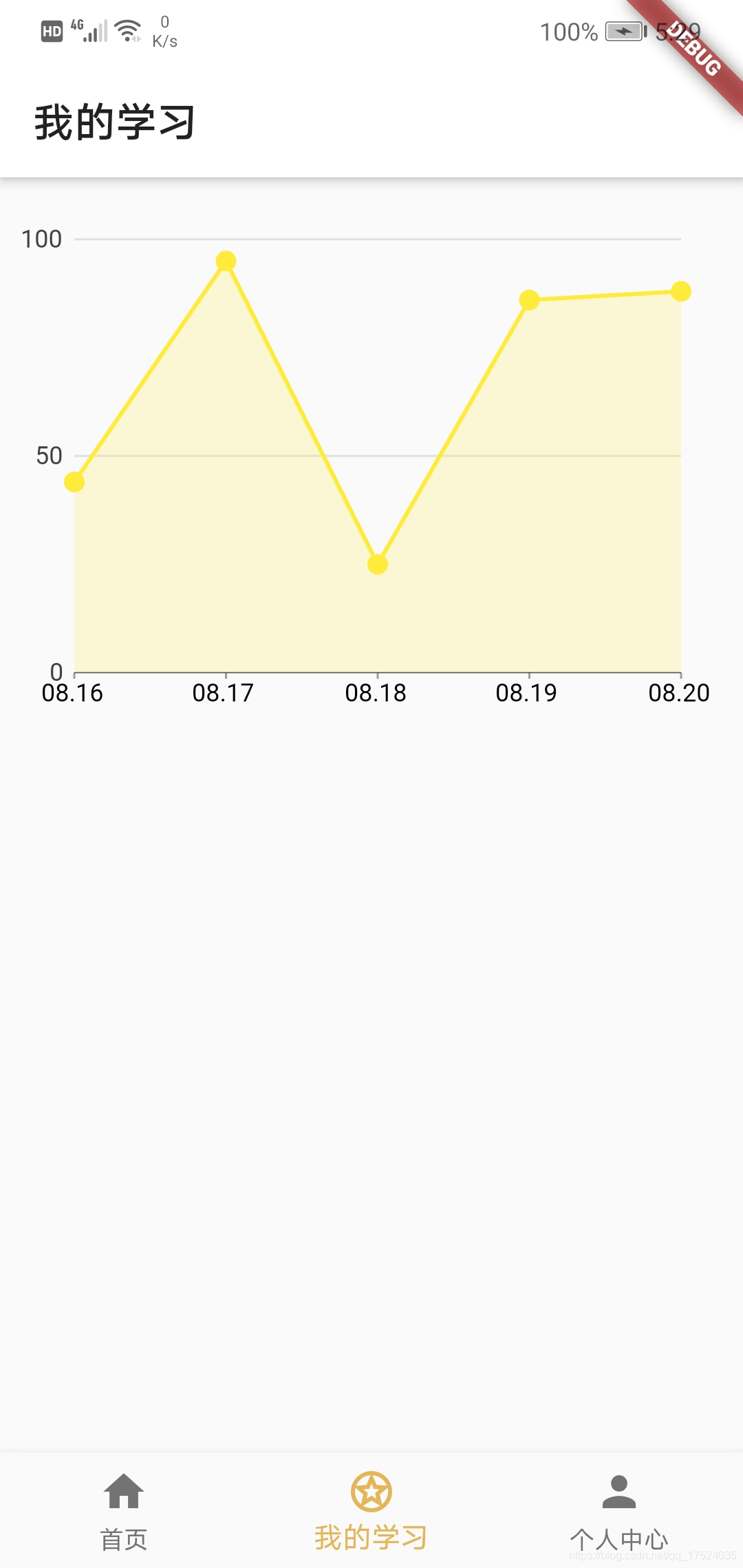
?
?
// Copyright 2018 the Charts project authors. Please see the AUTHORS file
// for details.
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
/// Line chart example
// EXCLUDE_FROM_GALLERY_DOCS_START
import 'dart:math';
import 'package:charts_flutter/flutter.dart' as charts;
// EXCLUDE_FROM_GALLERY_DOCS_END
import 'package:flutter/material.dart';
class PointsLineChart extends StatelessWidget {
final List<charts.Series<dynamic, num>> seriesList;
// final List<charts.Series> seriesList;
final bool animate;
PointsLineChart(this.seriesList, {this.animate = false});
/// Creates a [LineChart] with sample data and no transition.
// factory PointsLineChart.withSampleData() {
// return new PointsLineChart(
// _createSampleData(),
// // Disable animations for image tests.
// animate: false,
// );
// }
// EXCLUDE_FROM_GALLERY_DOCS_START
// This section is excluded from being copied to the gallery.
// It is used for creating random series data to demonstrate animation in
// the example app only.
factory PointsLineChart.withRandomData() {
return new PointsLineChart(_createRandomData());
}
/// Create random data.
static List<charts.Series<LinearSales, int>> _createRandomData() {
final random = new Random();
List<String> dateList = getDateList();
final data = [
new LinearSales(0, dateList[0], random.nextInt(100)),
new LinearSales(1, dateList[1], random.nextInt(100)),
new LinearSales(2, dateList[2], random.nextInt(100)),
new LinearSales(3, dateList[3], random.nextInt(100)),
new LinearSales(4, dateList[4], random.nextInt(100)),
];
return [
new charts.Series<LinearSales, int>(
id: 'Sales',
colorFn: (_, __) => charts.MaterialPalette.yellow.shadeDefault,
domainFn: (LinearSales sales, _) => sales.year,
measureFn: (LinearSales sales, _) => sales.sales,
data: data,
)
];
}
static var dateListTemp = [];
static List<String> getDateList() {
var dateTime2 = DateTime.now();
var dateTime = new DateTime(dateTime2.year, dateTime2.month, dateTime2.day - 4);
List<String> dateList = [];
for (int i = 0; i < 5; i++) {
var date = new DateTime(dateTime.year, dateTime.month, dateTime.day + i);
String month = date.month.toString();
String day = date.day.toString();
if (date.month < 10) {
month = "0" + month;
}
if (date.day < 10) {
day = "0" + day;
}
var s = month + "." + day;
dateList.add(s);
}
dateListTemp = dateList;
return dateList;
}
// EXCLUDE_FROM_GALLERY_DOCS_END
@override
Widget build(BuildContext context) {
return new charts.LineChart(
seriesList,
animate: animate,
defaultRenderer: charts.LineRendererConfig(
// 圆点大小
radiusPx: 5.0,
stacked: false,
// 线的宽度
strokeWidthPx: 2.0,
// 是否显示线
includeLine: true,
// 是否显示圆点
includePoints: true,
// 是否显示包含区域
includeArea: true,
// 区域颜色透明度 0.0-1.0
areaOpacity: 0.2,
),
domainAxis: new charts.NumericAxisSpec(
renderSpec: charts.SmallTickRendererSpec(
axisLineStyle: charts.LineStyleSpec(thickness: 0),
minimumPaddingBetweenLabelsPx: 10,
labelStyle: charts.TextStyleSpec(color: charts.Color.black),
labelRotation: 0),
showAxisLine: true,
tickFormatterSpec: charts.BasicNumericTickFormatterSpec((val) {
int number = 0;
print(val.toInt());
if (val.toInt() < 0) {
number = 0;
return "${val.toInt()}";
} else if (val.toInt() >= dateListTemp.length) {
number = dateListTemp.length - 1;
return "${val.toInt()}";
} else {
number = val.toInt();
return "${dateListTemp[number].substring(0)}";
}
// return "${val.toInt()}条";
})),
);
}
/// Create one series with sample hard coded data.
// static List<charts.Series<LinearSales, int>> _createSampleData() {
// List<String> dateList = getDateList();
// final data = [
// new LinearSales(0, dateList[0], 5),
// new LinearSales(1, dateList[1], 25),
// new LinearSales(2, dateList[2], 100),
// new LinearSales(3, dateList[3], 75),
// ];
//
// return [
// new charts.Series<LinearSales, int>(
// id: 'Sales',
// colorFn: (_, __) => charts.MaterialPalette.blue.shadeDefault,
// domainFn: (LinearSales sales, _) => sales.year,
// measureFn: (LinearSales sales, _) => sales.sales,
// data: data,
// )
// ];
// }
}
/// Sample linear data type.
class LinearSales {
final String name;
final int year;
final int sales;
LinearSales(this.year, this.name, this.sales);
}
|