第一步,我们先准备一个待集成的aar文件。我这里采用Androidstudio创建一个module,然后assemble一个libfoo.aar文件,具体代码如下:
package com.droid.libfoo;
public class Foo {
public int sum(int a, int b) {
return a + b;
}
}
第二步,创建一个flutter 插件工程:
flutter create --template=plugin foo_plugin
创建完成之后,用Androidstudio打开这个flutter工程,并在Android手机上运行下,确保该工程可以正常的构建和运行。
?第三步,import libfoo.aar 到这个插件工程,操作如下:
1. 在 ProjectRoot/android/下创建 libs文件夹,并拷贝 libfoo.aar 到其中
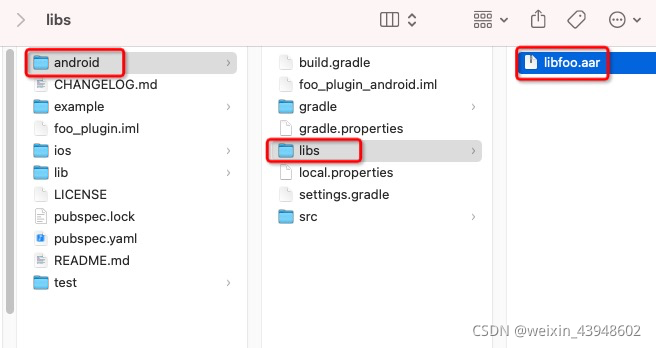
2. 修改build.gradle,集成libfoo.aar?
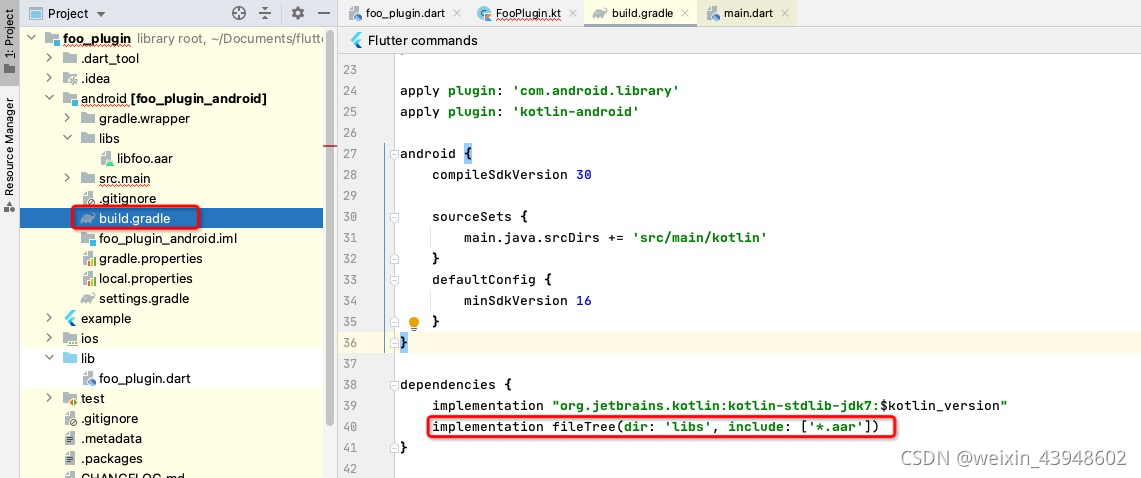
?3. 修改完成之后,开始构建,会遇到如下的错误:
FAILURE: Build failed with an exception.
* What went wrong:
Execution failed for task ':foo_plugin:bundleDebugAar'.
> Direct local .aar file dependencies are not supported when building an AAR. The resulting AAR would be broken because the classes and Android resources from any local .aar file dependencies would not be packaged in the resulting AAR. Previous versions of the Android Gradle Plugin produce broken AARs in this case too (despite not throwing this error). The following direct local .aar file dependencies of the :foo_plugin project caused this error: /Users/cjl/Documents/flutterproj/foo_plugin/android/libs/libfoo.aar
* Try:
Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output. Run with --scan to get full insights.
* Get more help at https://help.gradle.org
BUILD FAILED in 5s
Exception: Gradle task assembleDebug failed with exit code 1
FAILURE: Build failed with an exception.
* What went wrong: Execution failed for task ':foo_plugin:bundleDebugAar'. > Direct local .aar file dependencies are not supported when building an AAR. The resulting AAR would be broken because the classes and Android resources from any local .aar file dependencies would not be packaged in the resulting AAR. Previous versions of the Android Gradle Plugin produce broken AARs in this case too (despite not throwing this error). The following direct local .aar file dependencies of the :foo_plugin project caused this error: /Users/cjl/Documents/flutterproj/foo_plugin/android/libs/libfoo.aar
* Try: Run with --stacktrace option to get the stack trace. Run with --info or --debug option to get more log output. Run with --scan to get full insights.
* Get more help at https://help.gradle.org
BUILD FAILED in 5s Exception: Gradle task assembleDebug failed with exit code 1
4. 这个时候还需要进一步更新 build.gradle, 更新之后的build.gradle 如下:
group 'com.example.foo_plugin'
version '1.0-SNAPSHOT'
buildscript {
ext.kotlin_version = '1.3.50'
repositories {
google()
jcenter()
}
dependencies {
classpath 'com.android.tools.build:gradle:4.1.0'
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
}
}
rootProject.allprojects {
repositories {
google()
jcenter()
flatDir {
dirs project(':foo_plugin').file('libs')
}
}
}
apply plugin: 'com.android.library'
apply plugin: 'kotlin-android'
android {
compileSdkVersion 30
sourceSets {
main.java.srcDirs += 'src/main/kotlin'
}
defaultConfig {
minSdkVersion 16
}
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
// implementation fileTree(dir: 'libs', include: ['*.aar'])
implementation(name: 'libfoo', ext: 'aar')
}
主要修改点参考下面的贴图:
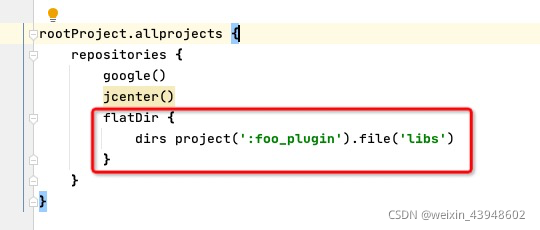
?
这个时候,我们再次构建运行,结果是成功的。
第三步:添加 channel method 代码:
import 'dart:async';
import 'package:flutter/services.dart';
class FooPlugin {
static const MethodChannel _channel =
const MethodChannel('foo_plugin');
static Future<String?> get platformVersion async {
final String? version = await _channel.invokeMethod('getPlatformVersion');
return version;
}
static Future<int?> sum(int a, int b) async {
final int? value = await _channel.invokeMethod('sum', [a, b]);
return value;
}
}
?
package com.example.foo_plugin
import androidx.annotation.NonNull
import com.droid.libfoo.Foo
import io.flutter.embedding.engine.plugins.FlutterPlugin
import io.flutter.plugin.common.MethodCall
import io.flutter.plugin.common.MethodChannel
import io.flutter.plugin.common.MethodChannel.MethodCallHandler
import io.flutter.plugin.common.MethodChannel.Result
/** FooPlugin */
class FooPlugin: FlutterPlugin, MethodCallHandler {
/// The MethodChannel that will the communication between Flutter and native Android
///
/// This local reference serves to register the plugin with the Flutter Engine and unregister it
/// when the Flutter Engine is detached from the Activity
private lateinit var channel : MethodChannel
override fun onAttachedToEngine(@NonNull flutterPluginBinding: FlutterPlugin.FlutterPluginBinding) {
channel = MethodChannel(flutterPluginBinding.binaryMessenger, "foo_plugin")
channel.setMethodCallHandler(this)
}
override fun onMethodCall(@NonNull call: MethodCall, @NonNull result: Result) {
if (call.method == "getPlatformVersion") {
result.success("Android ${android.os.Build.VERSION.RELEASE}")
} else if (call.method == "sum") {
val a: Int = (call.arguments as ArrayList<Int>)[0]
val b: Int = (call.arguments as ArrayList<Int>)[1]
result.success(Foo().sum(a, b))
} else {
result.notImplemented()
}
}
override fun onDetachedFromEngine(@NonNull binding: FlutterPlugin.FlutterPluginBinding) {
channel.setMethodCallHandler(null)
}
}
第四步:测试验证。 测试验证的代码非常简单,这里就不赘述了。
第五步:附上工程源码:?https://github.com/jaylon-pp/foo_plugin
|