一、搜索框

components/SearchInput:
//components.js
Component({
options: {
addGlobalClass: true, // 自定义组件中不显示iconfont的解决
},
properties: {
title: {
type: String,
value: ''
}
},
})
<!-- SearchInput.wxml -->
<view class="searchContainer">
<text class="iconfont icon-sousuo"></text>
<navigator class="search" url="/pages/search/search" hover-class="none">{{title}}</navigator>
</view>
/* SearchInput.wxss */
.searchContainer {
display: flex;
padding: 0 20rpx;
width: 260rpx;
height: 50rpx;
line-height: 50rpx;
border: 1rpx solid var(--themeColor);
border-radius: 25rpx;
color: #666;
}
.searchContainer .search {
margin-left: 10rpx;
font-size: 25rpx;
}
使用:
xx.json页面中:
{
"usingComponents": {
"SearchInput": "../../components/SearchInput/SearchInput"
}
}
<!-- xx.wxml -->
<SearchInput title="搜索发现更多音乐"></SearchInput>
二、推荐歌曲,导航

components/Tabs:
// Tabs.js
Component({
properties: {
headerTitle: {
type: String,
value: ''
},
Moretitle: {
type: String,
value: ''
}
},
})
?Tabs.wxml:
<view class="header">
<view class="headerTitle">{{headerTitle}}</view>
<view class="headerMore">
<view class="moreTitle">{{Moretitle}}</view>
<view class="more">查看更多</view>
</view>
</view>
?Tabs.wxss:
.header .headerMore {
/* 由于子元素全部浮动,父元素要清除浮动,flex布局更好一些 */
overflow: hidden;
}
.header .headerMore .moreTitle {
float: left;
font-size: 30rpx;
}
.header .headerMore .more {
float: right;
border: 1rpx solid #ccc;
padding: 5rpx 15rpx;
border-radius: 15rpx;
}
使用:
index.json:
{
"usingComponents": {
"Tabs": "/components/Tabs/Tabs"
}
}
index.wxml:
<Tabs headerTitle="推荐歌曲" Moretitle="为你精心推荐"></Tabs>
三、搜索框另一种样式:
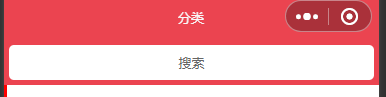
?components/SearchInput:
<!-- components/SearchInput/SearchInput.wxml -->
<view class="searchContainer">
<navigator class="search" url="/pages/search/search">搜索</navigator>
</view>
.searchContainer {
height: 90rpx;
padding: 10rpx;
background-color: #eb4450;
}
.searchContainer .search {
display: flex;
justify-content: center;
align-items: center;
border-radius: 10rpx;
height: 100%;
font-size: 26rpx;
color: #666;
background-color: #fff;
}
使用:
xx.json
{
"usingComponents": {
"SearchInput": "../../components/SearchInput/SearchInput"
},
"navigationBarTitleText": "分类"
}
xx.wxml:?
<SearchInput></SearchInput>
?四、导航栏切换
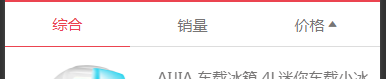
?
Component({
options: {
addGlobalClass: true, // 自定义组件中不显示iconfont的解决
},
// 组件的属性列表
properties: {
tabs: {
type: Array,
value: []
},
desc: {
type: Boolean,
value: true
}
},
//组件的方法列表
methods: {
changeNav(e) {
let index = e.currentTarget.dataset.index
// 触发父组件中的自定义事件
this.triggerEvent("itemChange", { index })
},
}
})
<view class="tabs">
<view class="title">
<view class="navItem {{item.isActive?'active':''}}" wx:for="{{tabs}}" wx:key="id" bindtap="changeNav" data-index="{{index}}">
<block wx:if="{{item.name=='价格'}}">
<text>{{item.name}}</text>
<text class="iconfont {{desc?'icon-jiangxu':'icon-shengxu'}}"></text>
</block>
<block wx:else>
{{item.name}}
</block>
</view>
</view>
<view class="content">
<slot></slot>
</view>
</view>
.tabs {
height: 90rpx;
border-bottom: 1rpx solid #ddd;
color: #777575;
font-size: 30rpx;
}
.tabs .title {
display: flex;
}
.tabs .title .navItem {
height: 90rpx;
display: flex;
flex: 1;
justify-content: center;
align-items: center;
}
.tabs .title .navItem.active {
color: var(--themeColor);
border-bottom: 1rpx solid var(--themeColor);
}
使用:
goods_list.json
{
"usingComponents": {
"searchInput": "../../components/SearchInput/SearchInput",
"Tabs": "../../components/Tabs/Tabs"
},
"navigationBarTitleText": "商品列表"
}
goods_list.wxml:
<Tabs tabs="{{tabs}}" desc="{{desc}}" binditemChange="itemChange">
<block wx:if="{{goodsList.length!==0}}">
<scroll-view class="scrollGoodsList" scroll-y bindscrolltolower="bindscrolltolower" bindrefresherrefresh="bindrefresherrefresh" refresher-enabled="{{refresherEnabled}}" refresher-triggered="{{refresherTriggered}}" scroll-top="{{scrollTop}}">
<view class="goodsContainer">
<navigator wx:for="{{goodsList}}" wx:key="goods_id" url="/pages/goods_detail/goods_detail?gid={{item.goods_id}}">
<view class="goodsImage">
<image mode="widthFix" src="{{item.goods_small_logo?item.goods_small_logo:noPic}}" />
</view>
<view class="goodsInfo">
<view class="title">{{item.goods_name}}</view>
<view class="price">¥ {{item.goods_price}}</view>
</view>
</navigator>
</view>
</scroll-view>
</block>
<block wx:else>
<image class="no-data" mode="widthFix" src="/static/images/no-data.png"></image>
<navigator class="goSelect" open-type="switchTab" url="/pages/category/main">
该分类暂无数据,换个试试吧~
</navigator>
</block>
</Tabs>
goods_list.js
Page({
data: {
tabs: [{
id: 0,
name: '综合',
isActive: true
}, {
id: 1,
name: '销量',
isActive: false
}, {
id: 2,
name: '价格',
isActive: false
}], // 导航栏
},
// 切换导航,子组件传递过来的
itemChange(e) {
let idx = e.detail.index
let tabs = JSON.parse(JSON.stringify(this.data.tabs))
tabs.map((item, index) => idx === index ? item.isActive = true : item.isActive = false)
this.setData({
tabs,
})
}
})
|