简述
????????AlertDialog可以在当前的界面上显示一个对话框,这个对话框是置顶于所有界面元素之上的,能够屏蔽掉其他控件的交互能力,因此AlertDialog一般是用于提示一些非常重要的内容或者警告信息。 ????????对应手机上就是弹出框。通常和单击事件连用。 正常的创建步骤 AlertDialog不需要布局因此不需要再布局文件中定义。 一、构造AlertDialog.Builder对象
AlertDialog.Builder defaultBuilder = new AlertDialog.Builder(MainActivity.this);
二、调用setTitle、setMessage、setIcon等方法构造对话框的标题、信息和图标等内容
defaultBuilder.setTitle("默认样式");
defaultBuilder.setMessage("这是什么样式?");
三、调用setPositive/Negative/NeutralButton()方法设置选项
defaultBuilder.setPositiveButton("默认样式",new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"选对了",Toast.LENGTH_SHORT).show();
}
});
四、显示
defaultBuilder.show();
通常有四种默认样式、单选弹出框、 多选弹出框、列表对话框、自定义弹出框 布局文件 四个按钮对应对应四个弹出类型
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FFFFFFFF"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="标题"
android:textSize="30sp"
android:textColor="#000"
android:layout_marginTop="20dp"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="自定义的AlertDialog"
android:textSize="20sp"
android:textColor="#000"
android:layout_marginTop="20dp"
/>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginTop="20dp"
android:background="#414E5E"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/btn_cancel"
android:layout_width="0dp"
android:layout_weight="1"
android:text="取消"
android:backgroundTint="#F8F3F3"
android:textColor="#0781B8"
android:textSize="20sp"
android:layout_height="wrap_content" />
<View
android:layout_width="1dp"
android:background="#E4E5E6"
android:layout_height="match_parent" />
<Button
android:id="@+id/btn_save"
android:layout_width="0dp"
android:layout_weight="1"
android:backgroundTint="#F8F3F3"
android:text="保存"
android:textColor="#0781B8"
android:textSize="20sp"
android:layout_height="wrap_content" />
</LinearLayout>
</LinearLayout>
默认样式
AlertDialog.Builder defaultBuilder = new AlertDialog.Builder(MainActivity.this);
defaultBuilder.setTitle("默认样式");
defaultBuilder.setMessage("这是什么样式?");
defaultBuilder.setPositiveButton("默认样式",new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"选对了",Toast.LENGTH_SHORT).show();
}
});
defaultBuilder.setNegativeButton("多选样式", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"选错了",Toast.LENGTH_SHORT).show();
}
});
defaultBuilder.setNeutralButton("我再想想", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"那你再想想",Toast.LENGTH_SHORT).show();
}
});
defaultBuilder.show();
效果 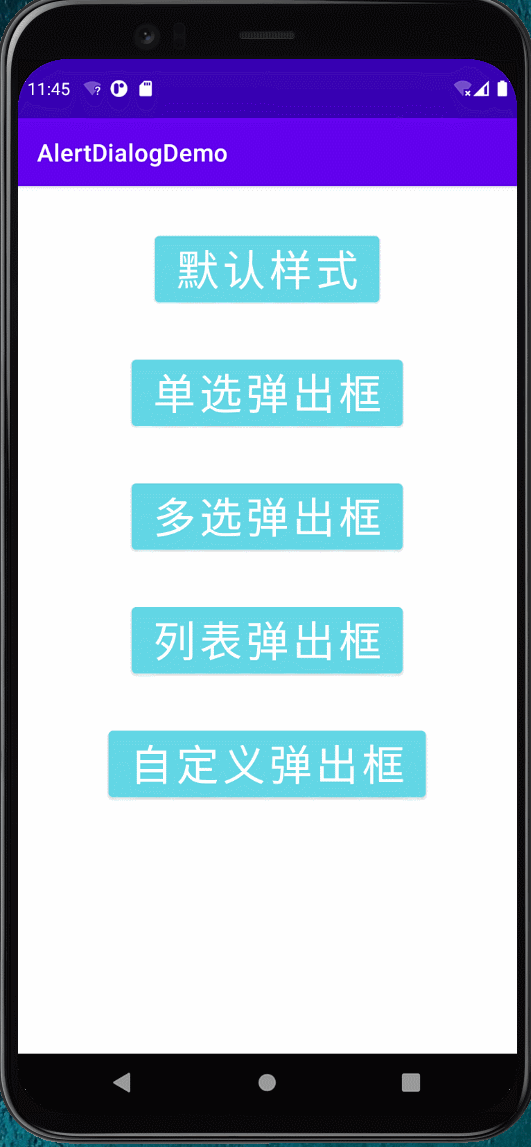
单选弹出框
public void singleAlertDialog(){
final String[] sex = {"男","女"};
AlertDialog.Builder singleBuilder = new AlertDialog.Builder(MainActivity.this);
singleBuilder.setTitle("请选择性别");
singleBuilder.setSingleChoiceItems(sex,0, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,sex[which],Toast.LENGTH_SHORT).show();
dialog.dismiss();
}
});
singleBuilder.show();
}
效果 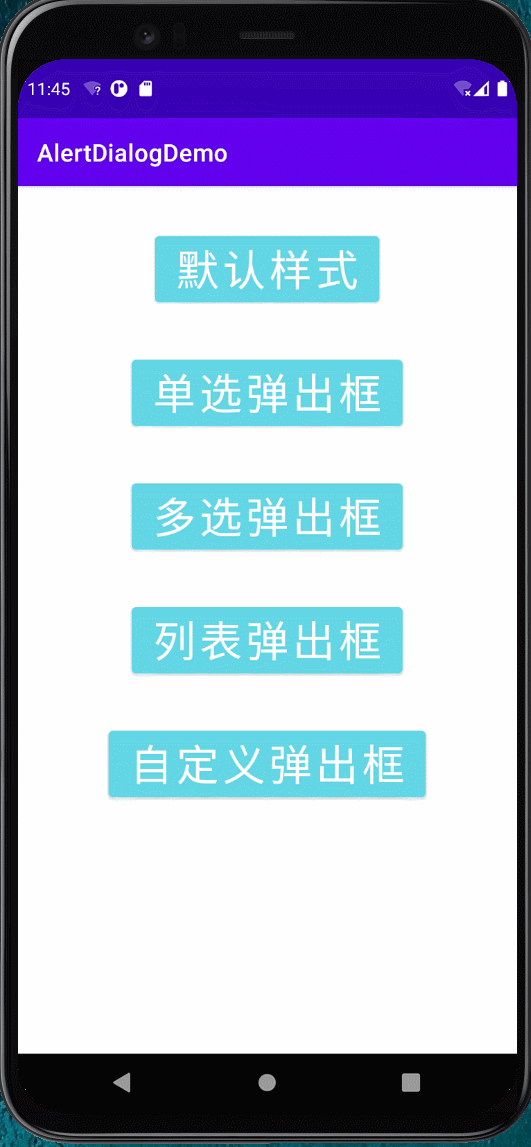
多选弹出框
public void multipleAlertDialog(){
final String[] book = {"从删库到跑路","深入理解计算机系学生秃头的原因","程序员的构造和解释","算法导论论不懂","计算机网络联不通"};
boolean[] begin = {false,false,false,false,false};
ArrayList<String> bookList = new ArrayList<String>();
AlertDialog.Builder multipleAlertDialog = new AlertDialog.Builder(MainActivity.this);
multipleAlertDialog.setTitle("想要读什么?");
multipleAlertDialog.setMultiChoiceItems(book, begin, new DialogInterface.OnMultiChoiceClickListener() {
@Override
public void onClick(DialogInterface dialog, int which, boolean isChecked) {
if(isChecked){
bookList.add(book[which]);
Toast.makeText(MainActivity.this,"任意选中就true",Toast.LENGTH_SHORT).show();
}else {
Toast.makeText(MainActivity.this,"任意取消就false",Toast.LENGTH_SHORT).show();
bookList.remove(book[which]);
}
}
});
multipleAlertDialog.setPositiveButton("选好了", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,bookList.toString(),Toast.LENGTH_SHORT).show();
}
});
multipleAlertDialog.setNeutralButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"不准备读一读吗?",Toast.LENGTH_SHORT).show();
}
});
multipleAlertDialog.show();
}
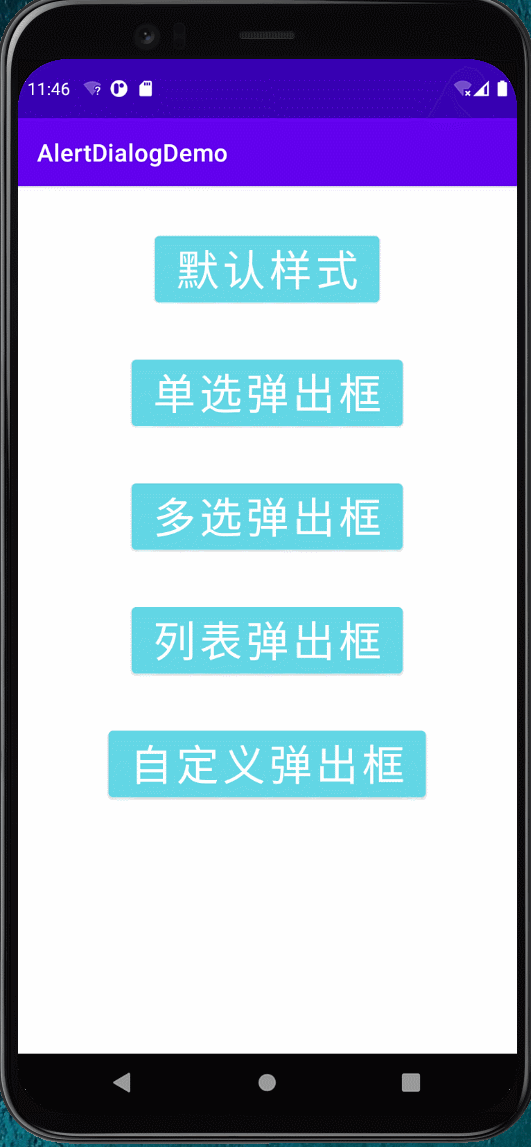
列表对话框
public void tableAlertDialog(){
AlertDialog.Builder tableBuilder = new AlertDialog.Builder(MainActivity.this);
final String[] course = {"C++","Java","Go","php","Pyhton"};
tableBuilder.setTitle("你喜欢那个语言?");
tableBuilder.setItems(course, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,course[which],Toast.LENGTH_SHORT).show();
}
});
tableBuilder.show();
}
效果 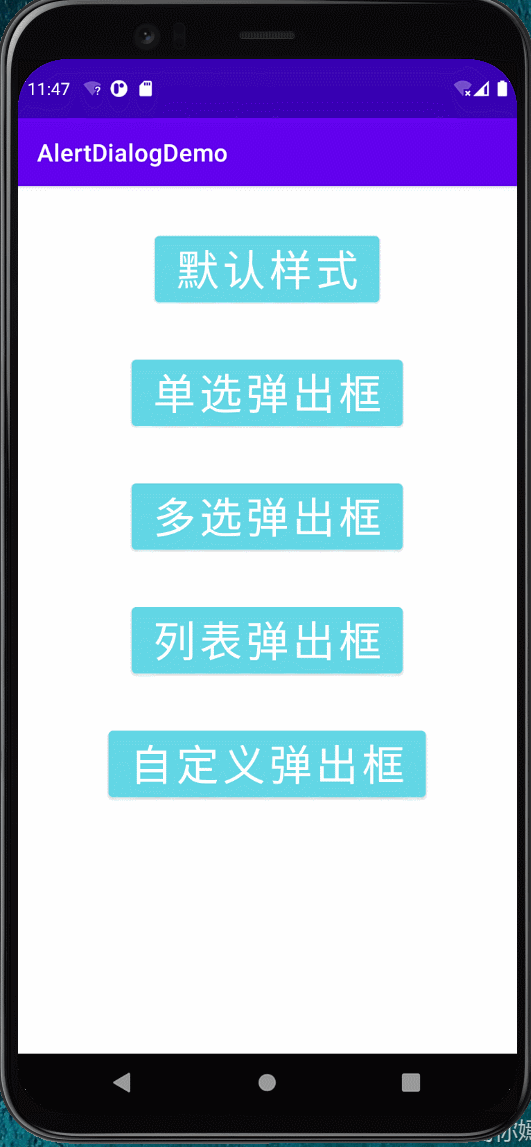
自定义弹出框
本质上就是弹出一个你自己定义的页面 布局alertdialog_custom
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FFFFFFFF"
android:orientation="vertical">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="标题"
android:textSize="30sp"
android:textColor="#000"
android:layout_marginTop="20dp"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="自定义的AlertDialog"
android:textSize="20sp"
android:textColor="#000"
android:layout_marginTop="20dp"
/>
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_marginTop="20dp"
android:background="#414E5E"
/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<Button
android:id="@+id/btn_cancel"
android:layout_width="0dp"
android:layout_weight="1"
android:text="取消"
android:backgroundTint="#F8F3F3"
android:textColor="#0781B8"
android:textSize="20sp"
android:layout_height="wrap_content" />
<View
android:layout_width="1dp"
android:background="#E4E5E6"
android:layout_height="match_parent" />
<Button
android:id="@+id/btn_save"
android:layout_width="0dp"
android:layout_weight="1"
android:backgroundTint="#F8F3F3"
android:text="保存"
android:textColor="#0781B8"
android:textSize="20sp"
android:layout_height="wrap_content" />
</LinearLayout>
</LinearLayout>
代码
public void customizeAlertDialog(){
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
AlertDialog dialog = builder.create();
View dialogView = LayoutInflater.from(MainActivity.this).inflate(R.layout.alertdialog_custom,null);
dialog.setView(dialogView);
dialog.show();
Button btnCancel,btnSave;
btnCancel = dialogView.findViewById(R.id.btn_cancel);
btnSave = dialogView.findViewById(R.id.btn_save);
btnCancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this,"你选择了取消",Toast.LENGTH_SHORT).show();
dialog.dismiss();
}
});
btnSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this,"你选择了保存",Toast.LENGTH_SHORT).show();
dialog.dismiss();
}
});
}
效果 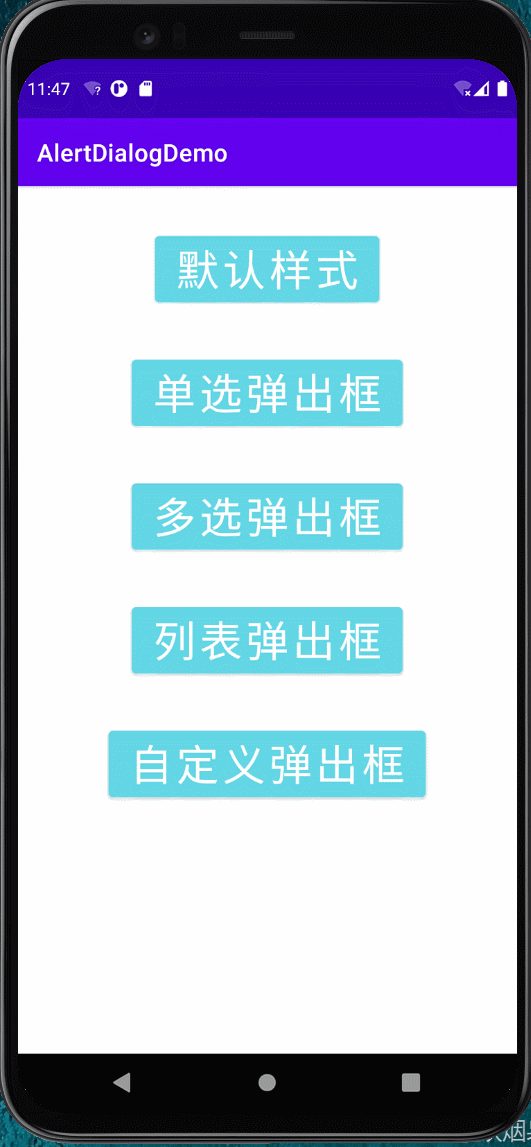 注意:宽度是固定的 想要修改宽度需要代码设置
dialog.getWindow().setLayout(width,height);
完整代码
import androidx.appcompat.app.AlertDialog;
import androidx.appcompat.app.AppCompatActivity;
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity {
Button mBtnDefault,mBtnSingle,mBtnMultiple,mBtnTable,mBtnCustomize;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mBtnDefault = findViewById(R.id.btnDefault);
mBtnSingle = findViewById(R.id.btnSingle);
mBtnMultiple = findViewById(R.id.btnMultiple);
mBtnTable = findViewById(R.id.btnTable);
mBtnCustomize = findViewById(R.id.btnCustomize);
mBtnDefault.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
defaultAlertDialog();
}
});
mBtnSingle.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
singleAlertDialog();
}
});
mBtnMultiple.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
multipleAlertDialog();
}
});
mBtnTable.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
tableAlertDialog();
}
});
mBtnCustomize.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
customizeAlertDialog();
}
});
}
public void defaultAlertDialog(){
AlertDialog.Builder defaultBuilder = new AlertDialog.Builder(MainActivity.this);
defaultBuilder.setTitle("默认样式");
defaultBuilder.setMessage("这是什么样式?");
defaultBuilder.setPositiveButton("默认样式",new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"选对了",Toast.LENGTH_SHORT).show();
}
});
defaultBuilder.setNegativeButton("多选样式", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"选错了",Toast.LENGTH_SHORT).show();
}
});
defaultBuilder.setNeutralButton("我再想想", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"那你再想想",Toast.LENGTH_SHORT).show();
}
});
defaultBuilder.show();
}
public void singleAlertDialog(){
final String[] sex = {"男","女"};
AlertDialog.Builder singleBuilder = new AlertDialog.Builder(MainActivity.this);
singleBuilder.setTitle("请选择性别");
singleBuilder.setSingleChoiceItems(sex,0, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,sex[which],Toast.LENGTH_SHORT).show();
dialog.dismiss();
}
});
singleBuilder.show();
}
public void multipleAlertDialog(){
final String[] book = {"从删库到跑路","深入理解计算机系学生秃头的原因","程序员的构造和解释","算法导论论不懂","计算机网络联不通"};
boolean[] begin = {false,false,false,false,false};
ArrayList<String> bookList = new ArrayList<String>();
AlertDialog.Builder multipleAlertDialog = new AlertDialog.Builder(MainActivity.this);
multipleAlertDialog.setTitle("想要读什么?");
multipleAlertDialog.setMultiChoiceItems(book, begin, new DialogInterface.OnMultiChoiceClickListener() {
@Override
public void onClick(DialogInterface dialog, int which, boolean isChecked) {
if(isChecked){
bookList.add(book[which]);
Toast.makeText(MainActivity.this,"任意选中就true",Toast.LENGTH_SHORT).show();
}else {
Toast.makeText(MainActivity.this,"任意取消就false",Toast.LENGTH_SHORT).show();
bookList.remove(book[which]);
}
}
});
multipleAlertDialog.setPositiveButton("选好了", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,bookList.toString(),Toast.LENGTH_SHORT).show();
}
});
multipleAlertDialog.setNeutralButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,"不准备读一读吗?",Toast.LENGTH_SHORT).show();
}
});
multipleAlertDialog.show();
}
public void tableAlertDialog(){
AlertDialog.Builder tableBuilder = new AlertDialog.Builder(MainActivity.this);
final String[] course = {"C++","Java","Go","php","Pyhton"};
tableBuilder.setTitle("你喜欢那个语言?");
tableBuilder.setItems(course, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Toast.makeText(MainActivity.this,course[which],Toast.LENGTH_SHORT).show();
}
});
tableBuilder.show();
}
public void customizeAlertDialog(){
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
AlertDialog dialog = builder.create();
View dialogView = LayoutInflater.from(MainActivity.this).inflate(R.layout.alertdialog_custom,null);
dialog.setView(dialogView);
dialog.show();
Button btnCancel,btnSave;
btnCancel = dialogView.findViewById(R.id.btn_cancel);
btnSave = dialogView.findViewById(R.id.btn_save);
btnCancel.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this,"你选择了取消",Toast.LENGTH_SHORT).show();
dialog.dismiss();
}
});
btnSave.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(MainActivity.this,"你选择了保存",Toast.LENGTH_SHORT).show();
dialog.dismiss();
}
});
}
}
|