首先声明两个Label
let titleLabel = UILabel()
let bodyLabel = UILabel()
在viewDidLoad中,将titleLabel和bodyLabel的属性设置好,然后创建一个StackView,将两个label放在里面,UIStackView是一个简化的界面,用于帮助程序猿将一队视图按竖直或者水平排列。将stackView设置为竖直排列,并将其放在视图中间。
titleLabel.backgroundColor = .red
bodyLabel.backgroundColor = .green
titleLabel.text = "title"
bodyLabel.text = "body"
let stackView = UIStackView(arrangedSubviews: [titleLabel,bodyLabel])
view.addSubview(stackView)
stackView.axis = .vertical
stackView.translatesAutoresizingMaskIntoConstraints = false
stackView.centerXAnchor.constraint(equalTo: view.centerXAnchor).isActive = true
stackView.centerYAnchor.constraint(equalTo: view.centerYAnchor).isActive = true
现在运行后,发现两个标签一上一下显示在屏幕中间了。 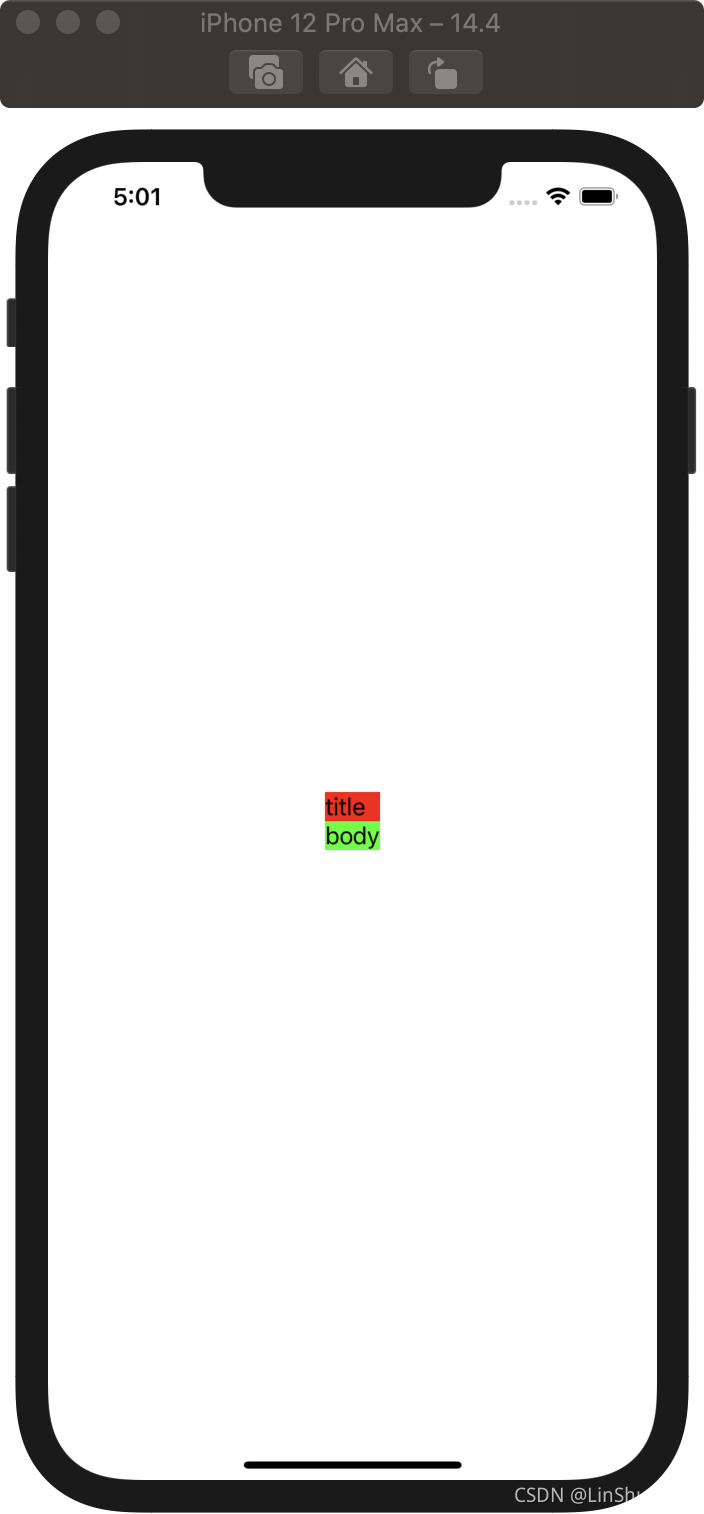 为标签添加一些有意义的字,并且改变字体大小,然后给stackView一个宽度。
titleLabel.backgroundColor = .red
titleLabel.text = "welcome to company XYZ,"
titleLabel.numberOfLines = 0
titleLabel.font = UIFont(name: "Futura", size: 34)
bodyLabel.backgroundColor = .green
bodyLabel.text = "Hello there! Thanks so much for downloading our brand new app and giving us a try. Make sure to leave us a good review in the AppStore."
bodyLabel.numberOfLines = 0
let stackView = UIStackView(arrangedSubviews: [titleLabel,bodyLabel])
view.addSubview(stackView)
stackView.axis = .vertical
stackView.translatesAutoresizingMaskIntoConstraints = false
stackView.centerXAnchor.constraint(equalTo: view.centerXAnchor).isActive = true
stackView.centerYAnchor.constraint(equalTo: view.centerYAnchor).isActive = true
stackView.widthAnchor.constraint(equalTo: view.widthAnchor, multiplier: 0.8).isActive = true
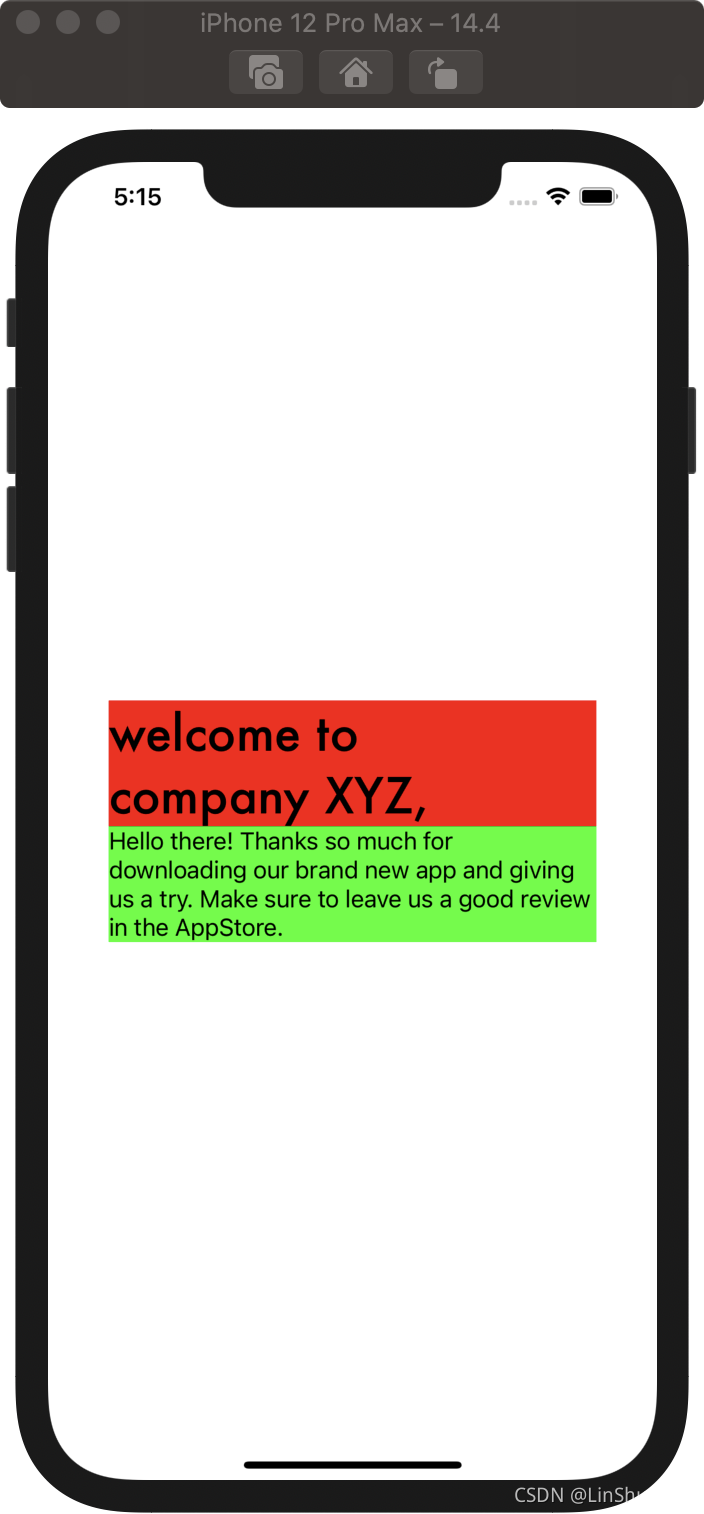 用refactor将两个label的设置和stackView的设置分离出去变为两个方法,接下来就可以开始写链接动画了。
为view添加一个点击手势
view.addGestureRecognizer(UITapGestureRecognizer(target: self, action: #selector(handleTap)))
添加响应方法,这样运行后发现titleLabel会先向左移30,然后向上移动后消失。这里的参数:
- duration:表示动画执行时间。
- delay:动画延迟时间。
- usingSpringWithDamping: 参数的范围为0.0f到1.0f,数值越小「弹簧」的振动效果越明显。可以视为弹簧的劲度系数
- initialSpringVelocity: 表示动画的初始速度,数值越大一开始移动越快。
- options:可选项,一些可选的动画效果,包括重复等。
- animations:表示执行的动画内容,包括透明度的渐变,移动,缩放。
- completion:表示执行完动画后执行的内容。
@objc func handleTap() {
UIView.animate(withDuration: 0.5, delay: 0, usingSpringWithDamping: 0.5, initialSpringVelocity: 0.5, options: .curveEaseOut) {
self.titleLabel.transform = CGAffineTransform(translationX: -30, y: 0)
} completion: { (_) in
UIView.animate(withDuration: 0.5, delay: 0, usingSpringWithDamping: 1, initialSpringVelocity: 1, options: .curveEaseOut, animations: {
self.titleLabel.transform = self.titleLabel.transform.translatedBy(x: 0, y: -200)
self.titleLabel.alpha = 0
}, completion: nil)
}
}
为bodyLabel也添加上动画,设置延迟为0.5秒。
UIView.animate(withDuration: 0.5, delay: 0.5, usingSpringWithDamping: 0.5, initialSpringVelocity: 0.5, options: .curveEaseOut) {
self.bodyLabel.transform = CGAffineTransform(translationX: -30, y: 0)
} completion: { (_) in
UIView.animate(withDuration: 0.5, delay: 0, usingSpringWithDamping: 1, initialSpringVelocity: 1, options: .curveEaseOut, animations: {
self.bodyLabel.transform = self.bodyLabel.transform.translatedBy(x: 0, y: -200)
self.bodyLabel.alpha = 0
}, completion: { (_) in
self.view.isUserInteractionEnabled = true
})
}
这样一个链式动画就完成啦。 完整代码
import UIKit
class ViewController: UIViewController {
let titleLabel = UILabel()
let bodyLabel = UILabel()
fileprivate func setupLabels() {
// Do any additional setup after loading the view.
titleLabel.text = "welcome to company XYZ,"
titleLabel.numberOfLines = 0
titleLabel.font = UIFont(name: "Futura", size: 34)
bodyLabel.text = "Hello there! Thanks so much for downloading our brand new app and giving us a try. Make sure to leave us a good review in the AppStore."
bodyLabel.numberOfLines = 0
}
fileprivate func setUpViews() {
let stackView = UIStackView(arrangedSubviews: [titleLabel,bodyLabel])
view.addSubview(stackView)
stackView.axis = .vertical
stackView.translatesAutoresizingMaskIntoConstraints = false
stackView.centerXAnchor.constraint(equalTo: view.centerXAnchor).isActive = true
stackView.centerYAnchor.constraint(equalTo: view.centerYAnchor).isActive = true
stackView.spacing = 8
stackView.widthAnchor.constraint(equalTo: view.widthAnchor, multiplier: 0.8).isActive = true
}
override func viewDidLoad() {
super.viewDidLoad()
setupLabels()
setUpViews()
// animation
view.addGestureRecognizer(UITapGestureRecognizer(target: self, action: #selector(handleTap)))
}
@objc func handleTap() {
view.isUserInteractionEnabled = false
UIView.animate(withDuration: 0.5, delay: 0, usingSpringWithDamping: 0.5, initialSpringVelocity: 0.5, options: .curveEaseOut) {
self.titleLabel.transform = CGAffineTransform(translationX: -30, y: 0)
} completion: { (_) in
UIView.animate(withDuration: 0.5, delay: 0, usingSpringWithDamping: 1, initialSpringVelocity: 1, options: .curveEaseOut, animations: {
self.titleLabel.transform = self.titleLabel.transform.translatedBy(x: 0, y: -200)
self.titleLabel.alpha = 0
}, completion: nil)
}
UIView.animate(withDuration: 0.5, delay: 0.5, usingSpringWithDamping: 0.5, initialSpringVelocity: 0.5, options: .curveEaseOut) {
self.bodyLabel.transform = CGAffineTransform(translationX: -30, y: 0)
} completion: { (_) in
UIView.animate(withDuration: 0.5, delay: 0, usingSpringWithDamping: 1, initialSpringVelocity: 1, options: .curveEaseOut, animations: {
self.bodyLabel.transform = self.bodyLabel.transform.translatedBy(x: 0, y: -200)
self.bodyLabel.alpha = 0
}, completion: { (_) in
self.view.isUserInteractionEnabled = true
})
}
}
}
|