内容简介
源码地址:https://gitee.com/liu_peilin/android-development-learning 1.实现APP门户界面框架设计,至少包含4个tab页,能实现tab页之间的点击切换; 2.使用布局(layouts)和分段(fragment),对控件进行点击监听 3.使用git将项目传至gitee。
需求分析
UI设计
以手机微信主界面为例: ??应将主界面分为三个版块,top,content,bottom; 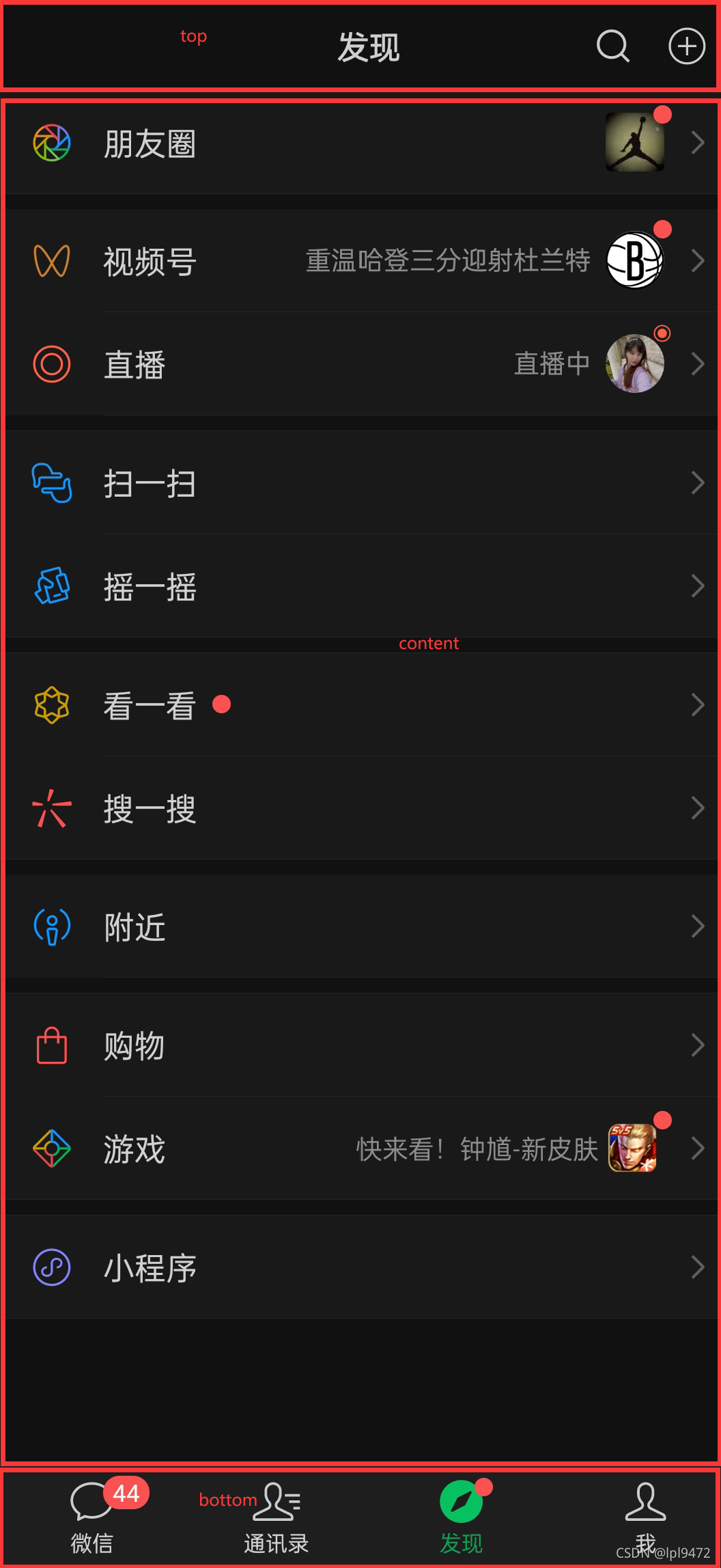
top
??在top版块应该展示出该主界面的标题,即wechat,在AS中,可在layout使用textview部件居中展示该文字效果。
content
??在该板块,应该能够根据底部功能栏的切换而随之切换。因此,在AS中,可以使用FrameLayout实现该功能,在FrameLayout中包含四个fragment部件对应四个功能栏即可。
bottom
??在bottom版块中,进一步分析应将其水平划分为四个LinearLayout,在每一个LinearLayout中应该再垂直划分为两个LinearLayout,如下图所示: 
后端功能设计
top
??该版块在本项目中固定为wechat,因此不做改变。
content
??该版块应能监听到bottom栏的点击事件,以此来切换该板块所展示的fragment。
bottom
??在该板块中,应该设置将四个水平方向上的LinearLayout设置点击监听事件,以此来改变content板块中的fragment
代码模块讲解
layout
activity_main.xml
??引入其他的xml文件应使用include语法; ??该界面的整体布局应该是vertical LinearLayout。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"
android:orientation="vertical">
<include layout="@layout/top"/>
<FrameLayout
android:id="@+id/content"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1">
</FrameLayout>
<include layout="@layout/bottom"/>
</LinearLayout>
bottom.xml
??下面代码以单个水平方向上的LinearLayout为例: ??imagView在此即为图标展示部件,具体图标可在iconfont官网下载。 ??需要将LinearLayout设置为可点击,否则无法监听到点击事件
<LinearLayout
android:id="@+id/LinearLayout_weixinFragment"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:clickable="true"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView1"
android:layout_width="wrap_content"
android:layout_height="40sp"
android:src="@drawable/wechat" />
<TextView
android:id="@+id/textView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="微信"
android:textColor="@color/white"
android:textSize="20sp" />
</LinearLayout>
MainAvticity
onCreate
??通过findViewById函数来找到创建各个fragment的对象,以便之后通过代码对四个fragment对象进行控制;又通过setOnClickListener函数对四个fragment对象设置点击监听事件。
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
supportRequestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
LinearLayout1 = findViewById(R.id.LinearLayout_weixinFragment);
LinearLayout2 = findViewById(R.id.LinearLayout_weixinContacts);
LinearLayout3 = findViewById(R.id.LinearLayout_weixinFind);
LinearLayout4 = findViewById(R.id.LinearLayout_weixinMe);
LinearLayout1.setOnClickListener(this);
LinearLayout2.setOnClickListener(this);
LinearLayout3.setOnClickListener(this);
LinearLayout4.setOnClickListener(this);
initFragment();#初始化fragment
showFragment(0);#展示weixinFragment页面
}
initFragment
??顾名思义,初始化fragment,FragmentTransaction可对fragment部件进行添加,替换,移除等操作
private void initFragment(){
fragmentManager = getFragmentManager();
FragmentTransaction transaction = fragmentManager.beginTransaction();
transaction.add(R.id.content,weixinFragment);
transaction.add(R.id.content,weixinContacts);
transaction.add(R.id.content,weixinFind);
transaction.add(R.id.content,weixinMe);
transaction.commit();
}
hideFragment
??隐藏所有的fragment部件。
public void hideFragment(FragmentTransaction transaction){
transaction.hide(weixinFragment);
transaction.hide(weixinContacts);
transaction.hide(weixinFind);
transaction.hide(weixinMe);
}
showFragment
??通过switch函数,以及transaction的show方法对四个fragment执行切换操作,当i变化时,所显示的fragment也随之变化
private void showFragment(int i){
FragmentTransaction transaction = fragmentManager.beginTransaction();
hideFragment(transaction);
switch (i){
case 0:
transaction.show(weixinFragment);
textView1.setText("WX");
break;
case 1:
transaction.show(weixinContacts);
textView2.setText("WC");
break;
case 2:
transaction.show(weixinFind);
textView3.setText("WF");
break;
case 3:
transaction.show(weixinMe);
textView4.setText("WM");
break;
default:
break;
}
transaction.commit();
}
onClick
??在写setOnClickListener函数时执行onClick的复写方法,通过监听所点击LinearLayout的id,来执行showFragment展示所对应的Fragment页面。
@Override
public void onClick(View v) {
switch (v.getId()){
case R.id.LinearLayout_weixinFragment:
showFragment(0);
break;
case R.id.LinearLayout_weixinContacts:
showFragment(1);
break;
case R.id.LinearLayout_weixinFind:
showFragment(2);
break;
case R.id.LinearLayout_weixinMe:
showFragment(3);
break;
default:
break;
}
}
fragment.java文件
??要将fragment装换成对象进行控制,需要创建fragment.java文件 ??以weixinFragment为例:
package com.example.mywechat;
import android.os.Bundle;
import android.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class weixinFragment extends Fragment {
public weixinFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_weixin, container, false);
}
}
AS项目上传gitee
git下载及安装
??具体安装教程可见链接:https://blog.csdn.net/mukes/article/details/115693833
gitee创建项目仓库
??具体选项如下图所示,然后点击创建即可: 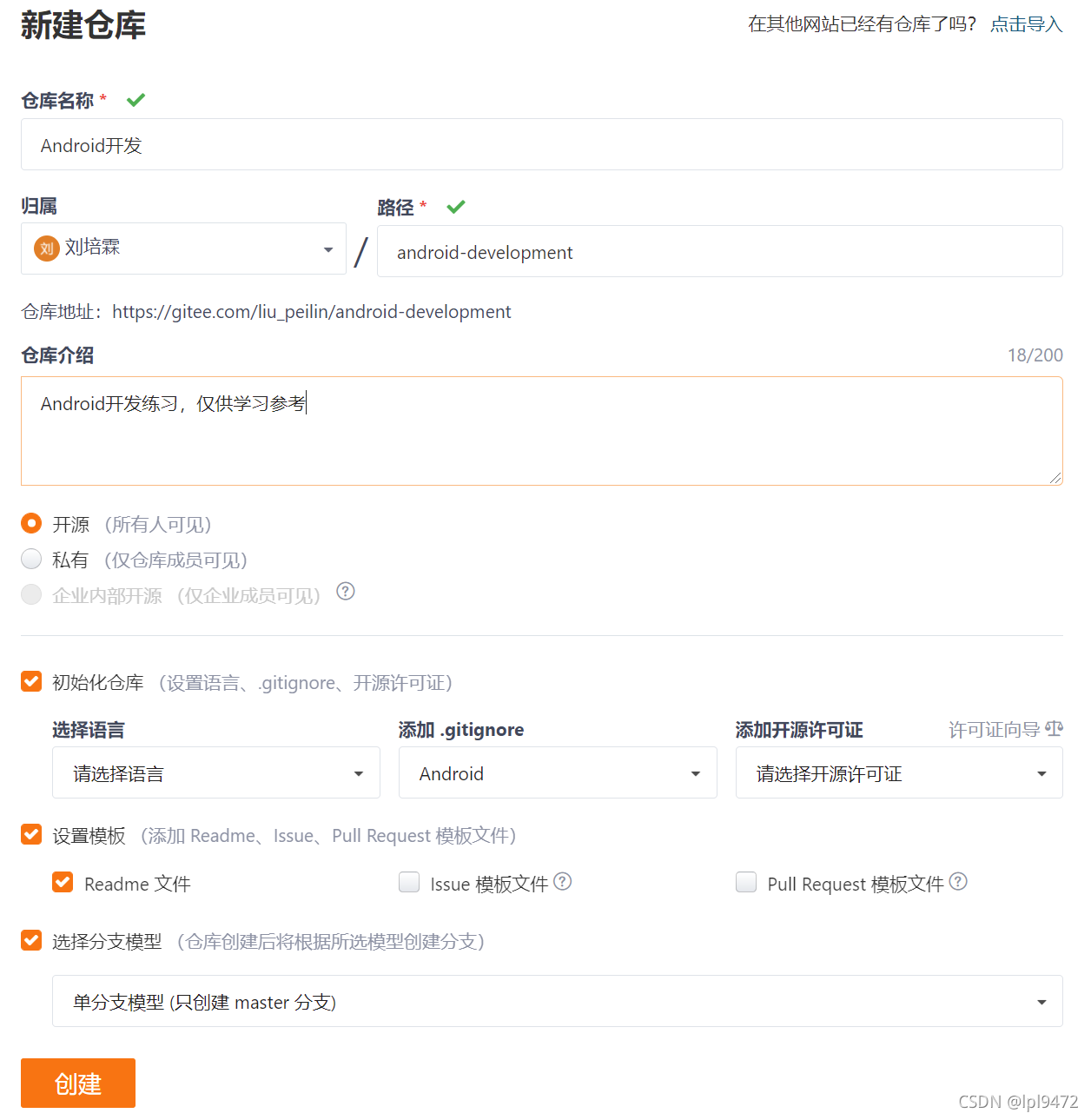
AS本地项目拉取至gitee仓库中
1.创建一个git repository 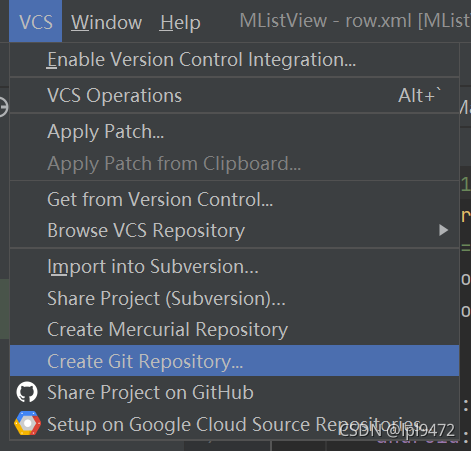 点击后,选择自己的本地项目文件夹 2.如下图所示,将自己的本地项目添加到暂存区 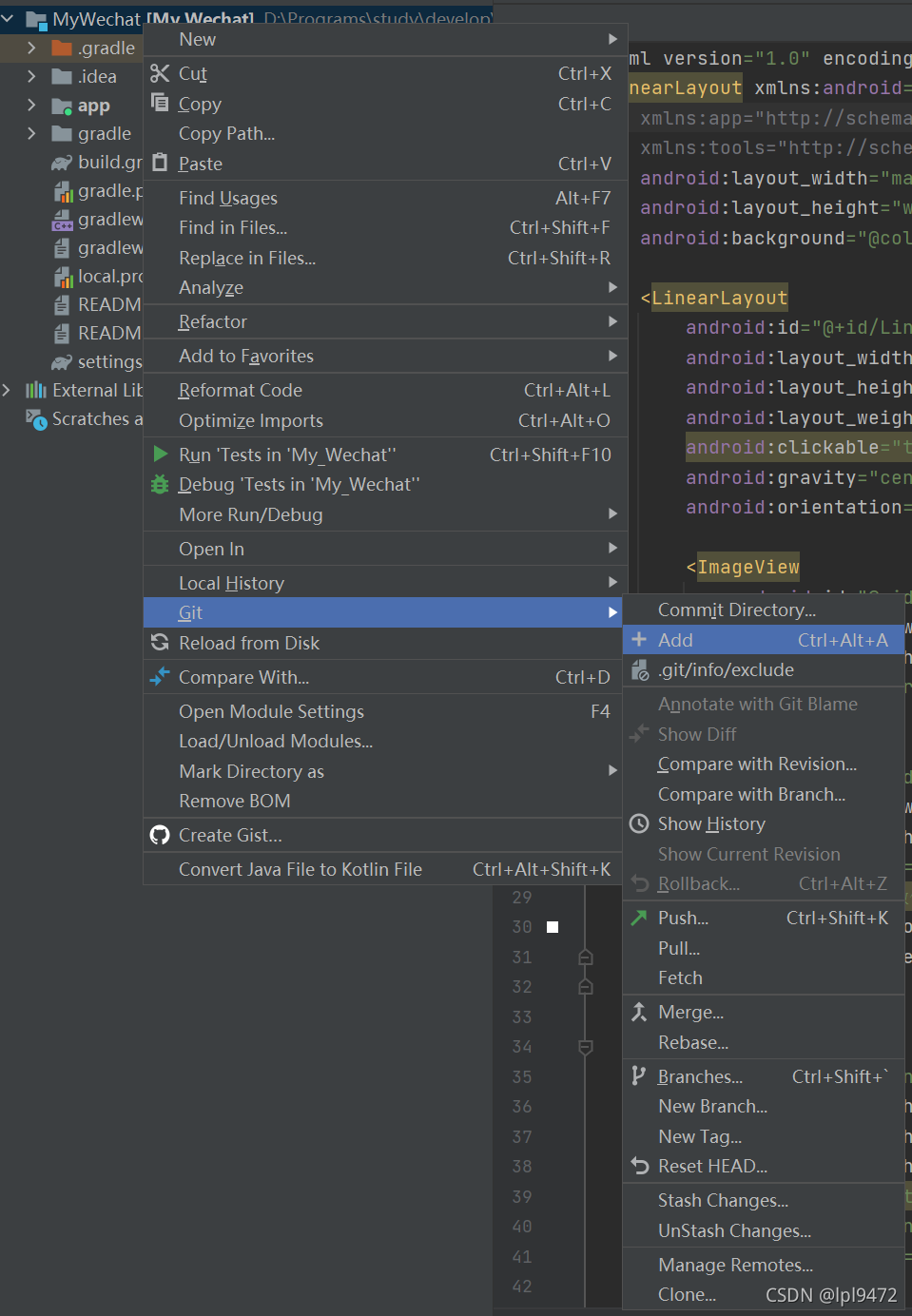 3.如下图所示,对远程仓库地址进行管理: 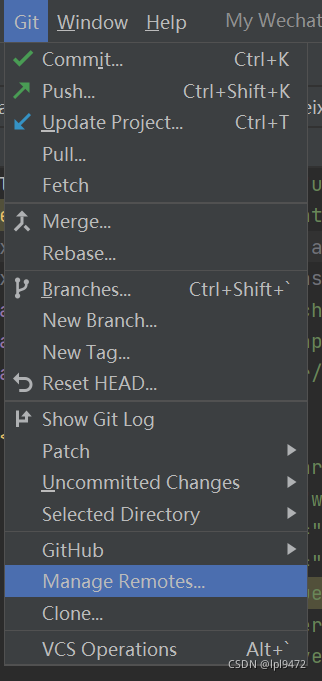 将自己的git仓库中的https连接复制之后填入AS中的URL,name默认为origin。 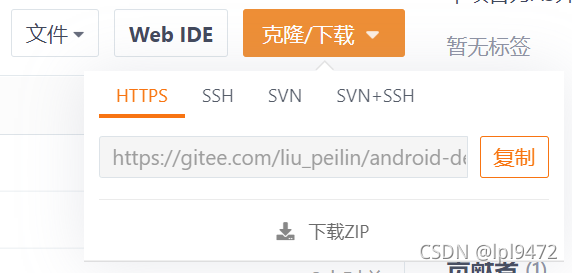
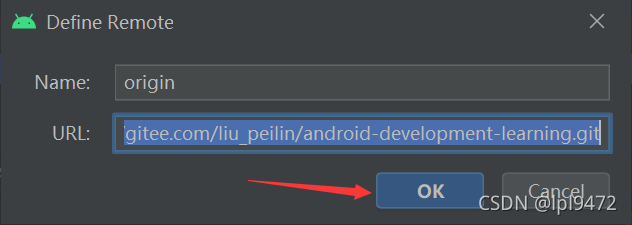
4.如下图所示,将AS项目提交至本地仓库 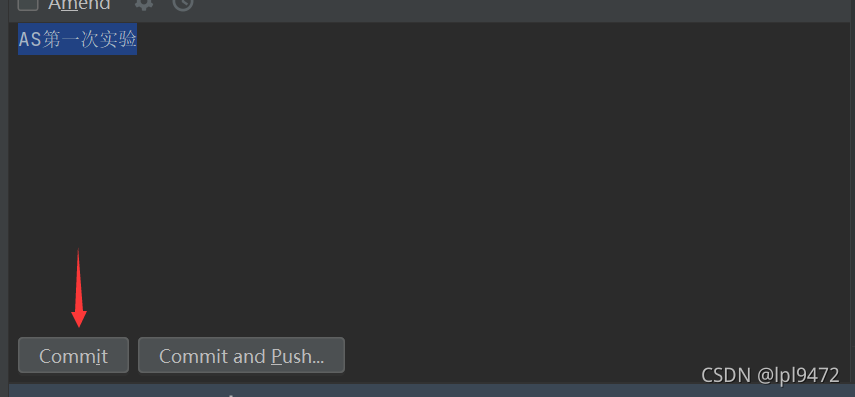 5.由于第一次push之前需要进行pull操作,因此,应在terminal命令行执行pull操作(可能会遇到合并冲突的情况,需要将AS项目自身所带的冲突文件(我的是.gitignore文件)删除后,再次提交,再进行pull操作即可) 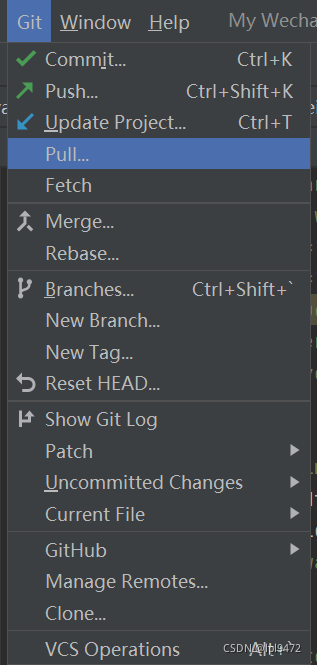 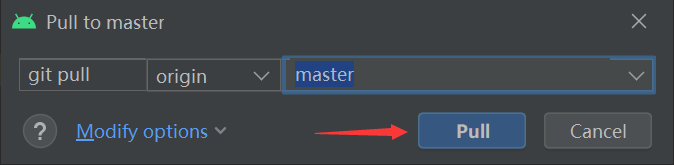 6.执行push操作即可完成 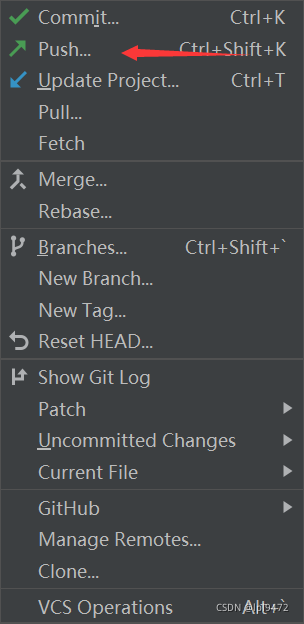 7.查看是否push完成 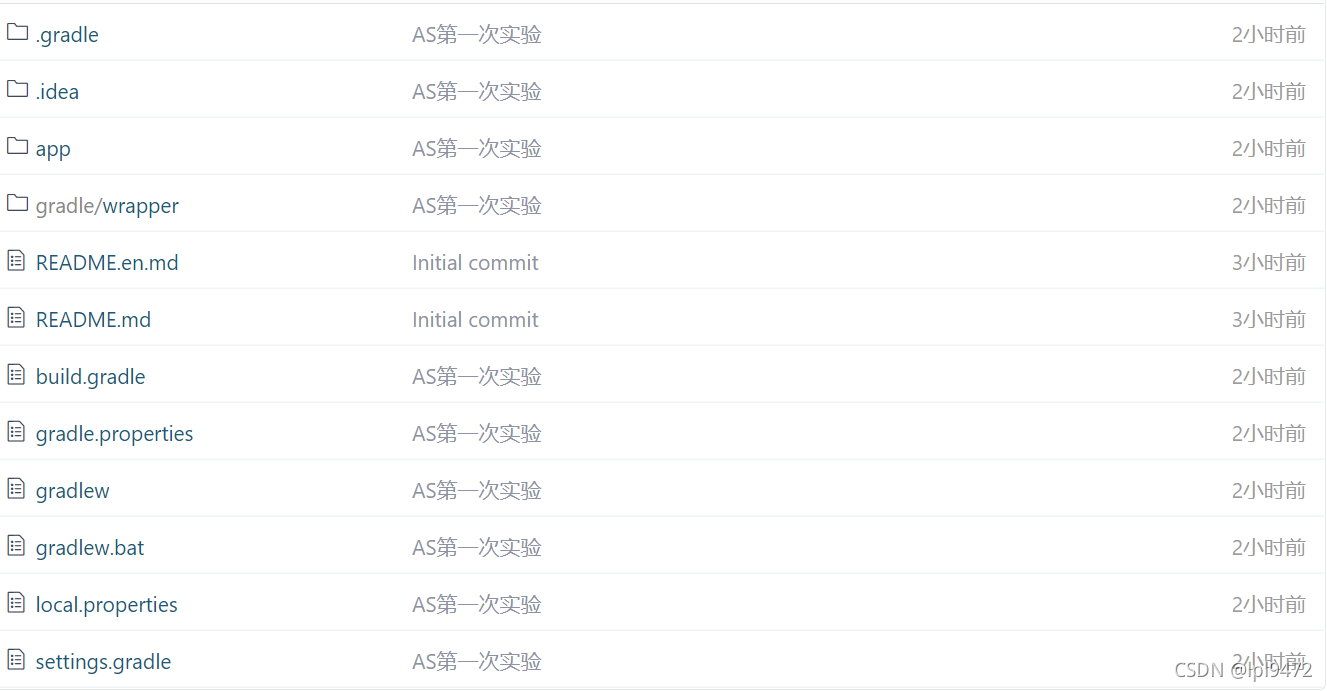 项目文件都在即push完成。
|