?一、运行界面
顶部的cx对应微信的顶部
中间的fragment对应微信的各种功能
最下面的菜单栏完成各个功能的切换
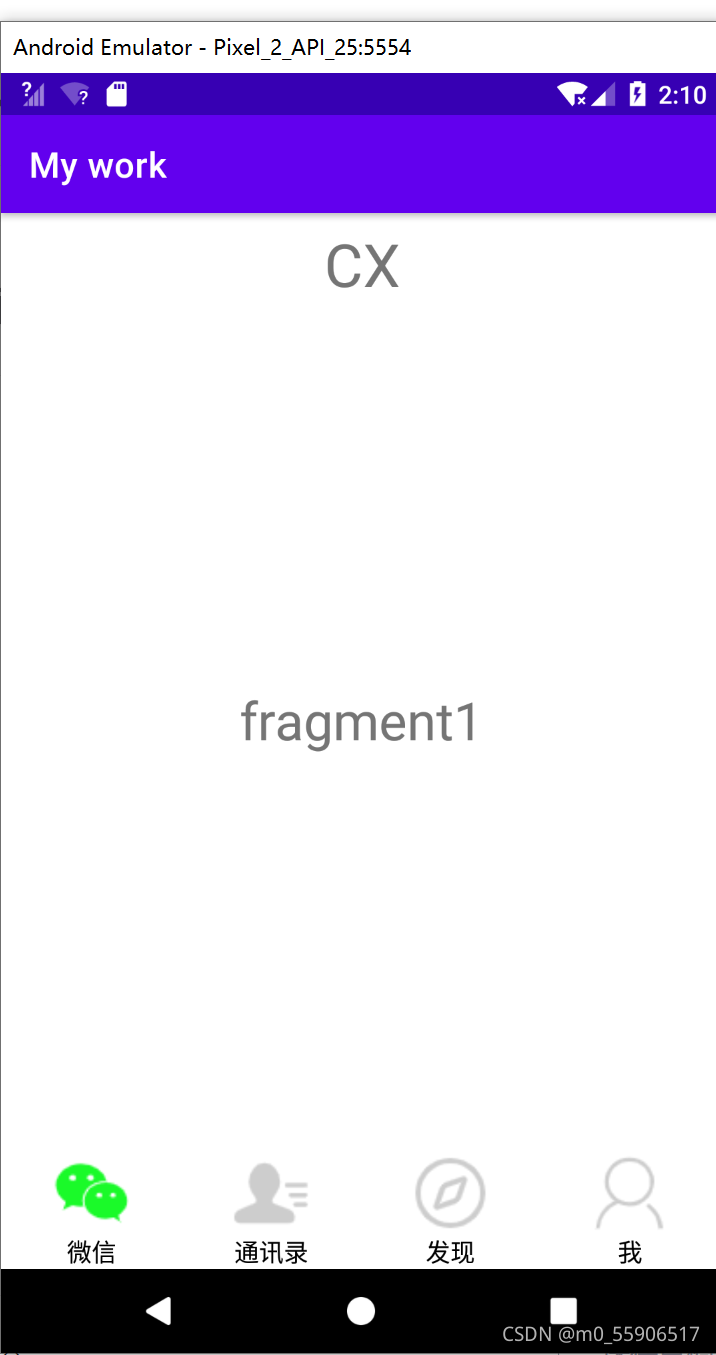
二、实现的大致思路
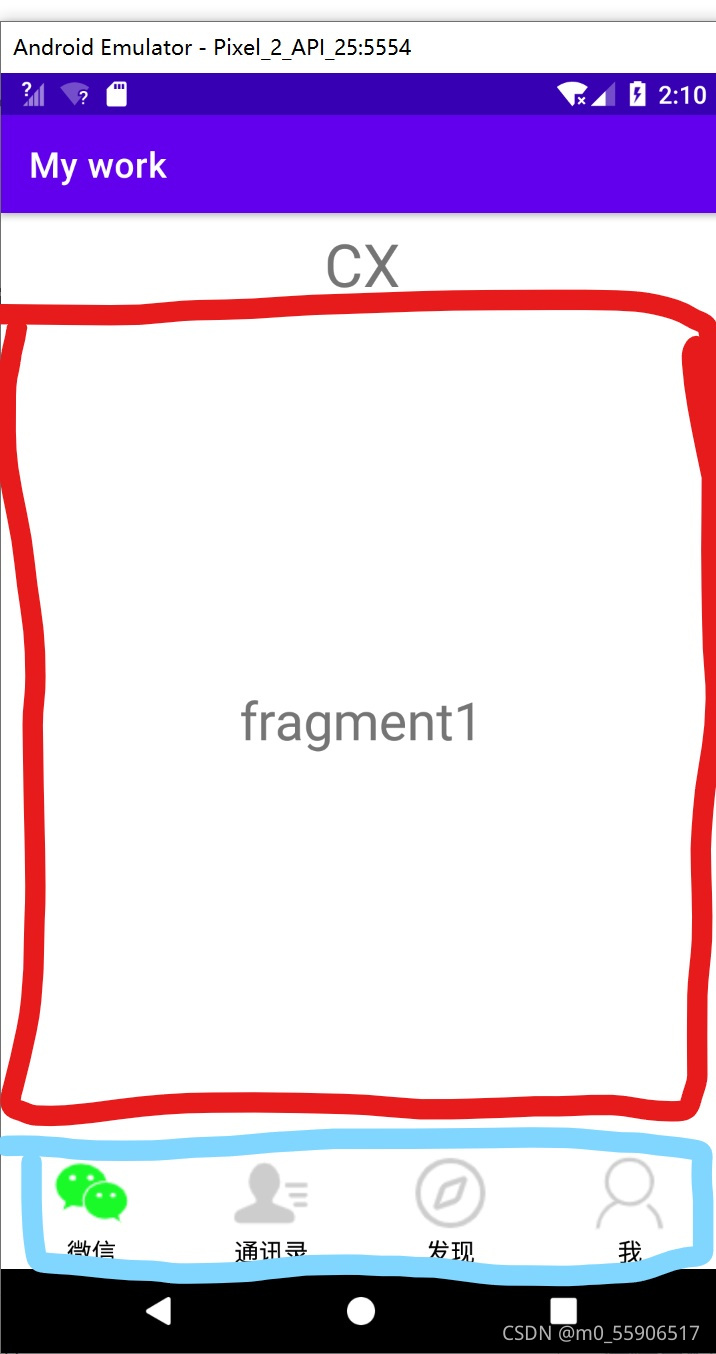
?红色框通过4个fragment完成,每个fragment的设计基本一样。
下方底部的菜单栏通过linearlayout里面包含四个小LinearLayout,每个LinearLayout里面含有一个ImageView和一个TextView,TextView写入功能,ImageView放入对应的ui图片,ui图片我是在iconfont里面找的,通过点击下方菜单实现fragment的切换。
三、具体实现
?activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<include
layout="@layout/bottom"
android:layout_width="405dp"
android:layout_height="84dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.545"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="@+id/textView"
app:layout_constraintVertical_bias="1.0"></include>
<TextView
android:id="@+id/textView"
android:layout_width="410dp"
android:layout_height="53dp"
android:gravity="center"
android:text="CX"
android:textSize="34sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<FrameLayout
android:id="@+id/content"
android:layout_width="406dp"
android:layout_height="590dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.3"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="@+id/textView"
app:layout_constraintVertical_bias="0.42">
</FrameLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
?bottom.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/linearLayout"
android:layout_width="410dp"
android:layout_height="75dp"
android:layout_gravity="bottom"
android:background="@color/white"
android:orientation="horizontal">
<LinearLayout
android:id="@+id/linearLayout1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:src="@drawable/a1"
tools:src="@drawable/a1" />
<TextView
android:id="@+id/textView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="微信"
android:textColor="@color/black" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:src="@drawable/a2"
tools:src="@drawable/a2" />
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:gravity="center"
android:text="通讯录"
android:textColor="@color/black" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout3"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:src="@drawable/a3"
tools:src="@drawable/a3" />
<TextView
android:id="@+id/textView3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="发现"
android:textColor="@color/black" />
</LinearLayout>
<LinearLayout
android:id="@+id/linearLayout4"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_gravity="bottom"
android:layout_weight="1"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:adjustViewBounds="true"
android:scaleType="centerCrop"
android:src="@drawable/a4"
tools:src="@drawable/a4" />
<TextView
android:id="@+id/textView4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="我"
android:textColor="@color/black" />
</LinearLayout>
</LinearLayout>
?fragment.xml
?剩下3个都是一样
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/frameLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".weixinfragment">
<!-- TODO: Update blank fragment layout -->
<TextView
android:id="@+id/textView01"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:text="fragment1"
android:textSize="30dp" />
</LinearLayout>
?具体代码实现
MainActivity.java
package com.example.mywork;
import androidx.appcompat.app.AppCompatActivity;
import android.app.Fragment;
import android.app.FragmentManager;
import android.app.FragmentTransaction;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
//implement View.OnClickListener 这个为了实现不用给每个控件设置监听,简化代码
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
private Fragment weixin0fragment=new weixinfragment();
private Fragment weixin1fragment=new weixinfragment1();
private Fragment weixin2fragment=new weixinfragment2();
private Fragment weixin3fragment=new weixinfragment3();
private LinearLayout linearLayout1,linearLayout2,linearLayout3,linearLayout4;
private ImageView imageView1,imageView2,imageView3,imageView4;
private FragmentManager fragmentMananger;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
linearLayout1=findViewById(R.id.linearLayout1);
linearLayout2=findViewById(R.id.linearLayout2);
linearLayout3=findViewById(R.id.linearLayout3);
linearLayout4=findViewById(R.id.linearLayout4);
imageView1=findViewById(R.id.imageView1);
imageView2=findViewById(R.id.imageView2);
imageView3=findViewById(R.id.imageView3);
imageView4=findViewById(R.id.imageView4);
linearLayout1.setOnClickListener(this);
linearLayout2.setOnClickListener(this);
linearLayout3.setOnClickListener(this);
linearLayout4.setOnClickListener(this);
initfragment();
//设置进去默认第一个fragment显示在最上面,不然会4个重叠在一起显示
showfragment(0);
//四个菜单的图标初始都是灰色,点击后会调用另一张图片,这张图片是绿色的
imageView1.setImageResource(R.drawable.p5);
}
private void initfragment(){
fragmentMananger=getFragmentManager();
//在activity中管理fragment,需要使用FragmentManager. 通过调用activity的getFragmentManager()取得它的实例
FragmentTransaction transaction= fragmentMananger.beginTransaction();
//对fragment进行增加删除隐藏等方法需要用到transaction
transaction.add(R.id.content, weixin0fragment);
transaction.add(R.id.content, weixin1fragment);
transaction.add(R.id.content, weixin2fragment);
transaction.add(R.id.content, weixin3fragment);
transaction.commit();
}//实现fragment的初始化
private void hidefragment(FragmentTransaction transaction){
transaction.hide( weixin0fragment);
transaction.hide( weixin1fragment);
transaction.hide( weixin2fragment);
transaction.hide( weixin3fragment);
}//隐藏fragment
public void onClick(View v){
hidebot();
switch (v.getId()){
case R.id.linearLayout1:imageView1.setImageResource(R.drawable.p5);showfragment(0);break;
case R.id.linearLayout2:imageView2.setImageResource(R.drawable.p6);showfragment(1);break;
case R.id.linearLayout3:imageView3.setImageResource(R.drawable.p7);showfragment(2);break;
case R.id.linearLayout4:imageView4.setImageResource(R.drawable.p8);showfragment(3);break;
default:break;
}
}//再更改图片之前先设置图片为灰色,在调用绿色的菜单的图标,然后显示出对应的fragment
private void showfragment(int i) {
FragmentTransaction transaction=fragmentMananger.beginTransaction();
hidefragment(transaction);
switch(i){
case 0:transaction.show(weixin0fragment);break;
case 1:transaction.show(weixin1fragment);break;
case 2:transaction.show(weixin2fragment);break;
case 3:transaction.show(weixin3fragment);break;
default:break;
}transaction.commit();
}//显示出点击的菜单栏对应的fragment,先隐藏所有的fragment,再显示出对应的fragment
private void hidebot(){
imageView1.setImageResource(R.drawable.a1);
imageView2.setImageResource(R.drawable.a2);
imageView3.setImageResource(R.drawable.a3);
imageView4.setImageResource(R.drawable.a4);
}//设置所有菜单的图片为灰色
}
?fragment.java
package com.example.mywork;
import android.os.Bundle;
import android.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
/**
* A simple {@link Fragment} subclass.
* Use the {@link weixinfragment#newInstance} factory method to
* create an instance of this fragment.
*/
public class weixinfragment extends Fragment {
// TODO: Rename parameter arguments, choose names that match
// the fragment initialization parameters, e.g. ARG_ITEM_NUMBER
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
// TODO: Rename and change types of parameters
private String mParam1;
private String mParam2;
public weixinfragment() {
// Required empty public constructor
}
/**
* Use this factory method to create a new instance of
* this fragment using the provided parameters.
*
* @param param1 Parameter 1.
* @param param2 Parameter 2.
* @return A new instance of fragment weixinfragment.
*/
// TODO: Rename and change types and number of parameters
public static weixinfragment newInstance(String param1, String param2) {
weixinfragment fragment = new weixinfragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
return inflater.inflate(R.layout.fragment_weixinfragment, container, false);
}
}
github网址:c43n/cxapp (github.com)
|