?
一、基本框架
1.顶部top.xml的实现:
顶部的实现过程:最外层为水平的LinearLayout,在其下添加一个TextView即可。
如图:
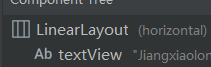
代码如下:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:background="@color/black"
android:layout_height="wrap_content">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="1"
android:gravity="center"
android:text="Jiangxiaolong app"
android:textColor="@color/white"
android:textSize="30sp" />
</LinearLayout>
实现效果如图:
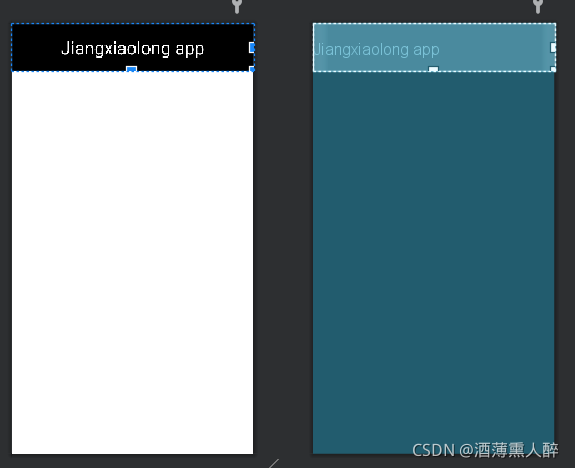
?2.底部bottom.xml的实现:
底部需要四个部分,每个部分包括一个imageview和textview,imageview要在textview上方。
如图:
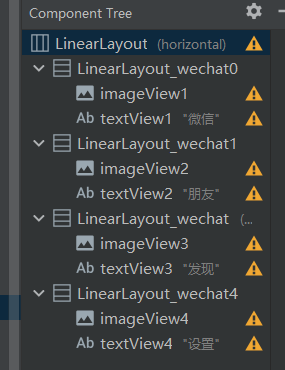
?代码如下:
值得注意的是:贴图的时候要使用Android:src,否则可能会报错
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:background="@color/black"
android:layout_height="70dp">
<LinearLayout
android:id="@+id/LinearLayout_wechat0"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/weiixn4" />
<TextView
android:id="@+id/textView1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="微信"
android:textColor="@color/white"
android:textSize="25sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/LinearLayout_wechat1"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/friend2"/>
<TextView
android:id="@+id/textView2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="朋友"
android:textColor="@color/white"
android:textSize="25sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/LinearLayout_wechat"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:src="@drawable/find2"/>
<TextView
android:id="@+id/textView3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="发现"
android:textColor="@color/white"
android:textSize="25sp" />
</LinearLayout>
<LinearLayout
android:id="@+id/LinearLayout_wechat4"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:orientation="vertical">
<ImageView
android:id="@+id/imageView4"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_weight="1"
android:src="@drawable/config2"/>
<TextView
android:id="@+id/textView4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:text="设置"
android:textColor="@color/white"
android:textSize="25sp" />
</LinearLayout>
</LinearLayout>
实现效果如图:
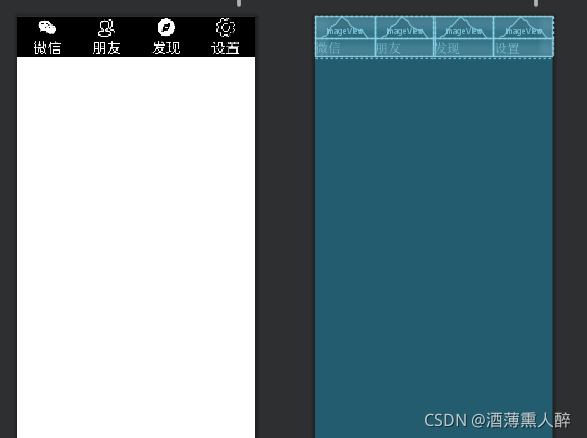
?
?3.总框架activity_main.xml:
使用垂直布局的LinearLayout,其下添加一个FramLayout。并将top.xml和bottom.xml包括进去即可。
如图:
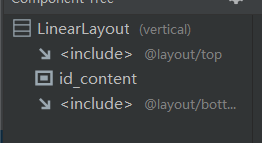
代码如下:
注意:top.xml要在framelayout上方,bottom.xml在其下方
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity" >
<include layout="@layout/top" />
<FrameLayout
android:id="@+id/id_content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1">
</FrameLayout>
<include
layout="@layout/bottom"
/>
</LinearLayout>
?4.基本框架实现效果
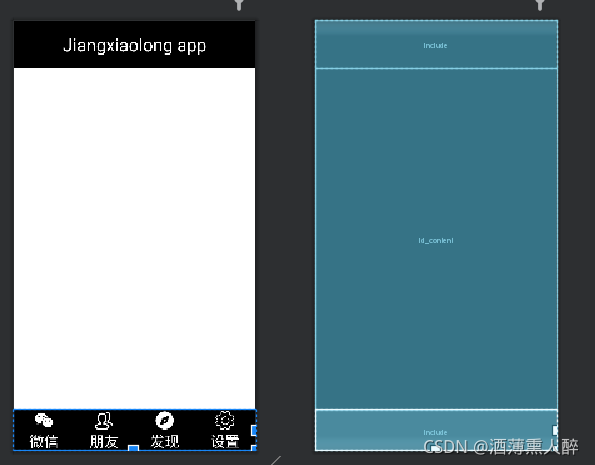
?
二.四个组件及切换动作的实现
1.四个组件的布局显示
在layout文件夹中新建四个文件。每一个xml文件下属一个textview。
如图:
?
四个组件的代码如下:
contentweixin.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="30dp"
android:text="这里是聊天界面" />
</androidx.constraintlayout.widget.ConstraintLayout>
friendweixin.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="30dp"
android:text="这里是朋友" />
</androidx.constraintlayout.widget.ConstraintLayout>
findweixin.xml
?
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="30dp"
android:text="这里是发现" />
</androidx.constraintlayout.widget.ConstraintLayout>
configweixin.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textSize="30dp"
android:text="这里是设置" />
</androidx.constraintlayout.widget.ConstraintLayout>
2.定义调用四个界面的类
使用inflater将四个xml文件压缩到文件中。
content.java
package com.example.myapplication1;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class contentweixin extends Fragment {
public contentweixin() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.contentweixin, container, false);
}
}
friend.java
package com.example.myapplication1;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class friend extends Fragment {
public friend() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.friendweixin, container, false);
}
}
findweixin.java
package com.example.myapplication1;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class findweixin extends Fragment {
public findweixin() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.findweixin, container, false);
}
}
configweixin.java
package com.example.myapplication1;
import android.app.Fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class configweixin extends Fragment {
public configweixin() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.configweixin, container, false);
}
}
3.注意:可能存在的错误来源于包
将相关的包改为:
import android.app.Fragment;
import android.app.FragmentManager;
import android.app.FragmentTransaction;
4.切换功能的实现
主要是各个部分的显示和隐藏,这由两个函数来实现.
显示:
private void showfragment(int i){
FragmentTransaction transaction=fragmentManager.beginTransaction();
hideFragment(transaction);
switch (i){
case 0:
transaction.show(contentweixin);
break;
case 1:
transaction.show(friendweixin);
break;
case 2:
transaction.show(findweixn);
break;
case 3:
transaction.show(configweixin);
break;
default:
break;
}
transaction.commit();
}
隐藏:
private void hideFragment( FragmentTransaction transaction){
transaction.hide(friendweixin);
transaction.hide(findweixn);
transaction.hide(contentweixin);
transaction.hide(configweixin);
三.主程序中关于界面显示功能的实现
1.在activity初始化的时候调用的OnCreat()函数
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
linearLayout1=findViewById(R.id.LinearLayout_wechat);
linearLayout2=findViewById(R.id.LinearLayout_wechat0);
linearLayout3=findViewById(R.id.LinearLayout_wechat1);
linearLayout4=findViewById(R.id.LinearLayout_wechat4);
imageView1=findViewById(R.id.imageView1);
imageView2=findViewById(R.id.imageView2);
imageView3=findViewById(R.id.imageView3);
imageView4=findViewById(R.id.imageView4);
linearLayout1.setOnClickListener(this);
linearLayout2.setOnClickListener(this);
linearLayout3.setOnClickListener(this);
linearLayout4.setOnClickListener(this);
initFragment();
}
2.初始化函数initFragment()
private void initFragment(){
fragmentManager=getFragmentManager();
FragmentTransaction transaction=fragmentManager.beginTransaction();
transaction.add(R.id.id_content, configweixin);
transaction.add(R.id.id_content,contentweixin);
transaction.add(R.id.id_content,findweixn);
transaction.add(R.id.id_content,friendweixin);
hideFragment(transaction);
transaction.commit();
}
3.获取组件信息的函数OnClick()
public void onClick(View view) {
switch (view.getId()){
case R.id.LinearLayout_wechat0:
reImage(0);
showfragment(0);
break;
case R.id.LinearLayout_wechat1:
reImage(1);
showfragment(1);
break;
case R.id.LinearLayout_wechat:
reImage(2);
showfragment(2);
break;
case R.id.LinearLayout_wechat4:
reImage(3);
showfragment(3);
break;
default:
break;
}
}
4.显示颜色区别的函数reimage()
和onclick函数一起使用
主要是使用了两组颜色不同的图片,通过颜色变化来显示正在使用那个组件。
public void reImage(int i)
{
imageView1.setImageResource(R.drawable.weiixn4);
imageView2.setImageResource(R.drawable.friend2);
imageView3.setImageResource(R.drawable.find2);
imageView4.setImageResource(R.drawable.config2);
switch (i)
{
case 0:
imageView1.setImageResource((R.drawable.weixin1));
break;
case 1:
imageView2.setImageResource(R.drawable.friend1);
break;
case 2:
imageView3.setImageResource(R.drawable.find1);
break;
case 3:
imageView4.setImageResource(R.drawable.config1);
break;
default:
break;
}
}
四.效果演示
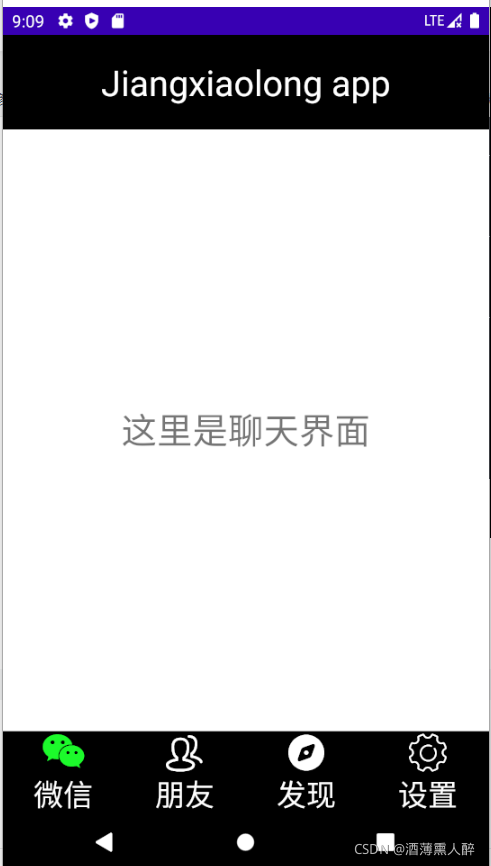 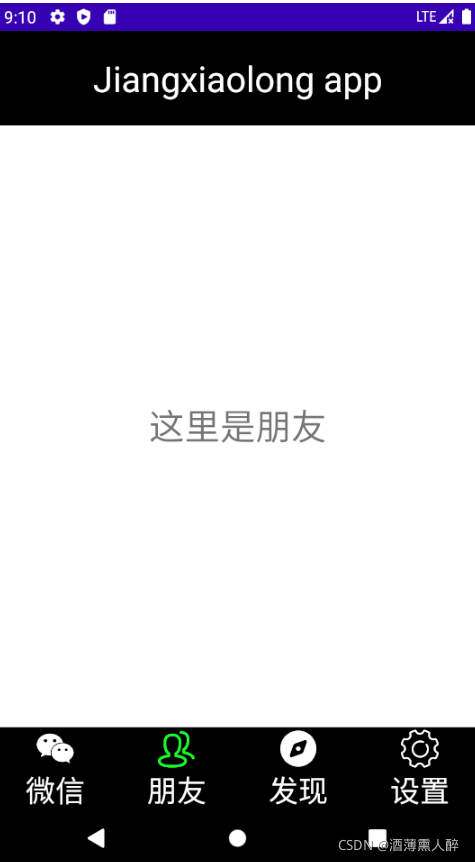 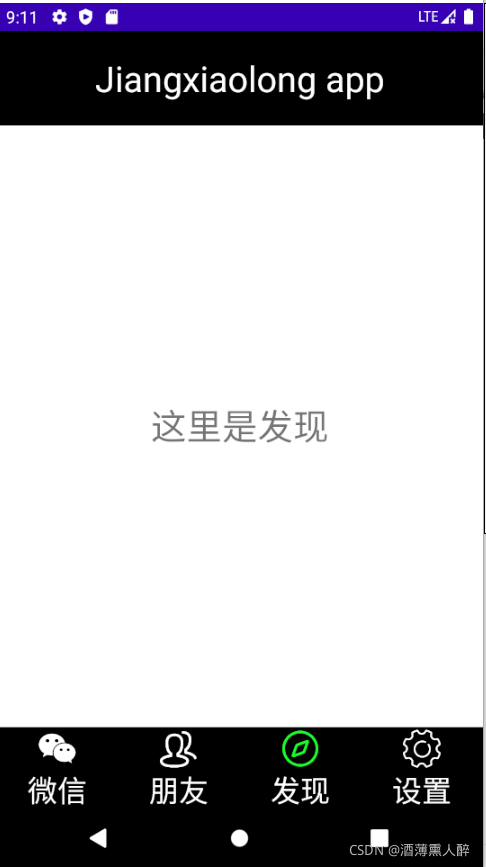 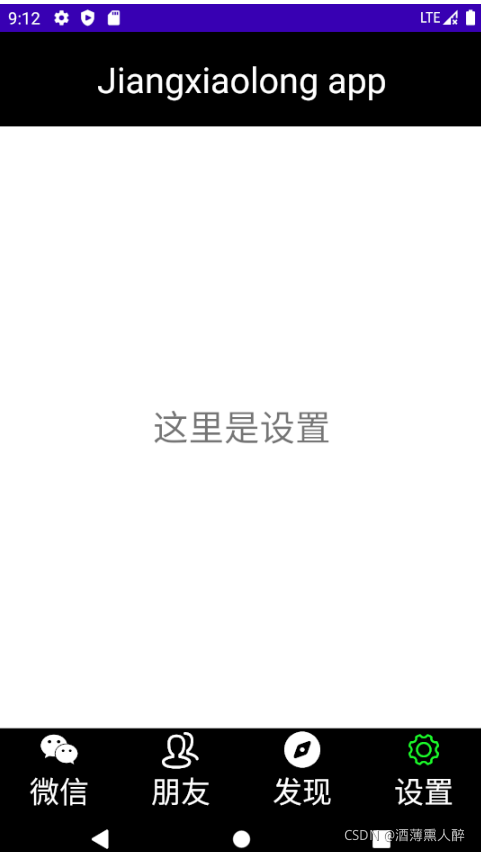
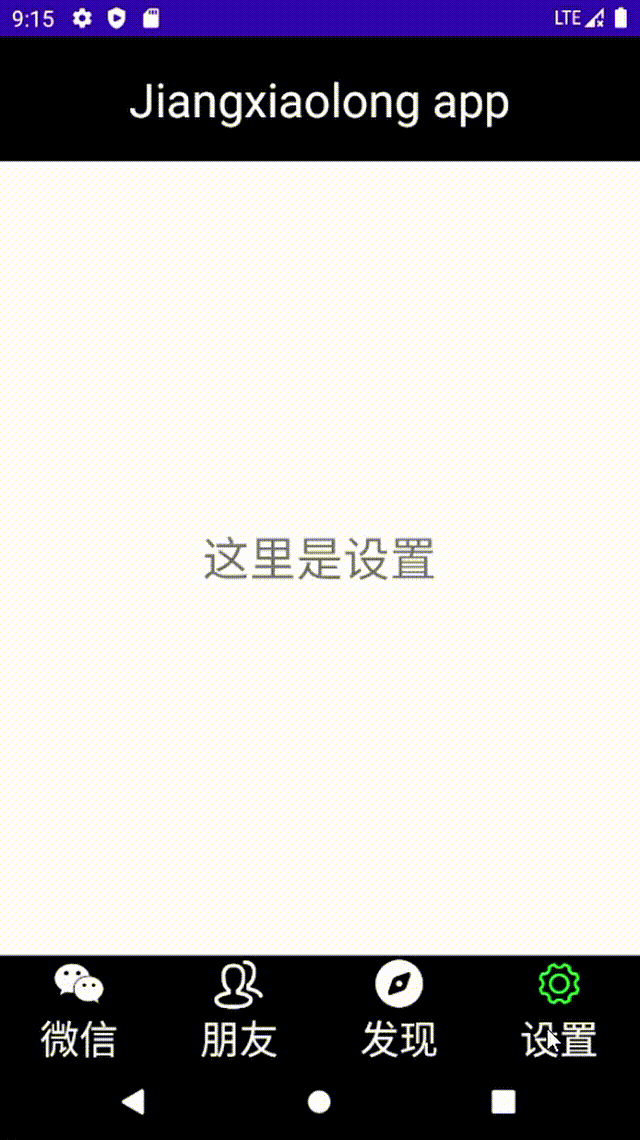
?
?五.原码地址
https://gitee.com/mansions007/MyApplication1
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
?
|