一、微信界面的功能
1、可展示出四个可切换界面:微信、联系人、朋友圈、设置; 2、上方栏标题居中,界面中间显示内容,内容随下方栏的选择而切换,下方栏可点击切换,点击过的界面的图标为绿色,没有点击的界面的图标为灰色; 3.主要从top、bottom、中间布局以及MainActivity四个方面分析。
二、top布局
在res->layout新建top.xml文件,将将标题栏的内容居中,并将背景色调为黑色,文字调为白色。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="@color/black"
android:gravity="center"
android:orientation="vertical">
<TextView
android:id="@+id/textView6"
android:layout_width="wrap_content"
android:layout_height="40dp"
android:text="WeChat"
android:textColor="@color/white"
android:layout_gravity="center"
android:textSize="30sp" />
</LinearLayout>
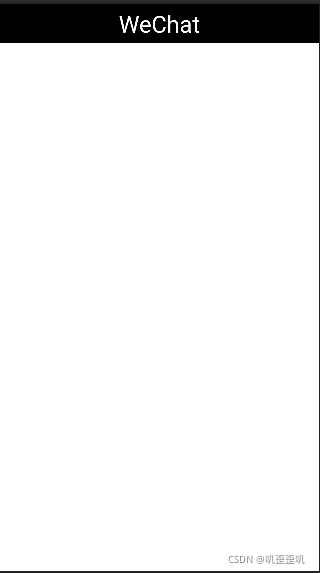
三、bottom布局
1.将图标文件夹粘贴到drawable中; 2.在res文件夹的layout中新建bottom.xml,拖入TextView拖入ImageButton后会调用drawable,选取所需的图标; 3.复制粘贴四个并调整水平平铺布局
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="80dp">
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:background="@color/black"
android:orientation="horizontal">
<LinearLayout
android:id="@+id/LinearLayout_weixin"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:orientation="vertical">
<ImageButton
android:id="@+id/id_tab_wechat_image"
android:layout_width="match_parent"
android:layout_height="53dp"
android:background="#000000"
android:clickable="false"
android:contentDescription="@string/app_name"
app:srcCompat="@drawable/p1" />
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="129dp"
android:layout_weight="1"
android:gravity="center"
android:text="聊天"
android:textColor="@color/white"
android:textSize="20dp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:id="@+id/LinearLayout_lianxiren"
android:gravity="center"
android:orientation="vertical">
<ImageButton
android:id="@+id/id_tab_friend_image"
android:layout_width="match_parent"
android:layout_height="53dp"
android:background="#000000"
android:clickable="false"
android:contentDescription="@string/app_name"
android:src="@drawable/p2" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="155dp"
android:layout_weight="1"
android:text="联系人"
android:gravity="center"
android:textColor="@color/white"
android:textSize="20dp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:id="@+id/LinearLayout_pengyouquan"
android:gravity="center"
android:orientation="vertical">
<ImageButton
android:id="@+id/id_tab_contact_image"
android:layout_width="match_parent"
android:layout_height="53dp"
android:background="#000000"
android:clickable="false"
android:contentDescription="@string/app_name"
android:src="@drawable/p3" />
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="朋友圈"
android:gravity="center"
android:textColor="@color/white"
android:textSize="20dp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:id="@+id/LinearLayout_shezhi"
android:gravity="center"
android:orientation="vertical">
<ImageButton
android:id="@+id/id_tab_settings_image"
android:layout_width="match_parent"
android:layout_height="53dp"
android:background="#000000"
android:contentDescription="@string/app_name"
android:clickable="false"
android:src="@drawable/p4" />
<TextView
android:id="@+id/textView4"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_weight="1"
android:text="设置"
android:gravity="center"
android:textColor="@color/white"
android:textSize="20dp" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
四、中间布局
四个tab.xml分别对应四个界面的中间内容,此处以微信界面为例。
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".weixinFragment">
<!-- TODO: Update blank fragment layout -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:text="这是微信聊天界面!"
android:textSize="40sp" />
</LinearLayout>
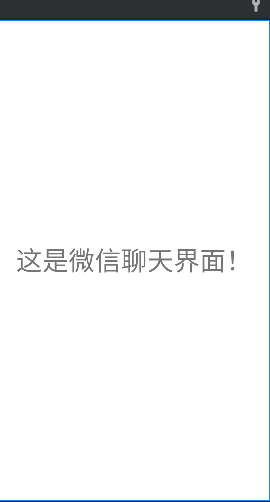
五、activity_main.xml
activity_main.xml里面需要加上FragmentLayout(重叠布局)和include
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<include layout="@layout/top"/>
<FrameLayout
android:id="@+id/id_content"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1">
</FrameLayout>
<include layout="@layout/buttom" />
</LinearLayout>
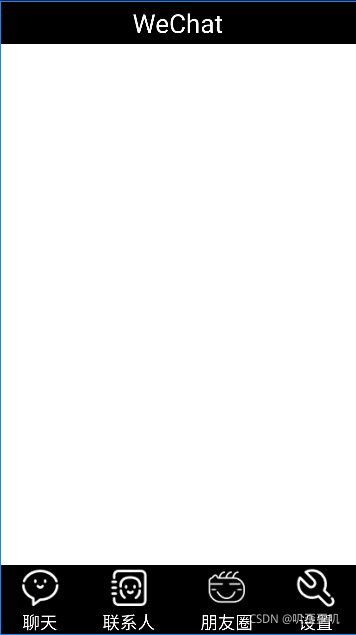
六、MainActivity
实现fragment的切换、图标颜色的改变、隐藏未使用界面等功能
package com.example.mywork;
import android.app.Activity;
import android.os.Bundle;
import android.view.Window;
import android.view.View;
import android.app.FragmentManager;
import android.app.Fragment;
import android.app.FragmentTransaction;
import android.widget.ImageButton;
import android.widget.LinearLayout;
public class MainActivity extends Activity implements View.OnClickListener{
private Fragment mTab01=new weixinFragment();
private Fragment mTab02=new lianxirenFragment();
private Fragment mTab03=new pengyouquanFragment();
private Fragment mTab04=new shezhiFragment();
private FragmentManager fm;
LinearLayout mTabWechat;
LinearLayout mTabFriend;
LinearLayout mTabContact;
LinearLayout mTabSettings;
ImageButton mimgWechat;
ImageButton mimgFriend;
ImageButton mimgContact;
ImageButton mimgSettings;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
setContentView(R.layout.activity_main);
initView();
initEvent();
initFragment();
selectfragment(0);
}
private void initEvent(){
mTabWechat.setOnClickListener(this);
mTabFriend.setOnClickListener(this);
mTabContact.setOnClickListener(this);
mTabSettings.setOnClickListener(this);
}
private void initFragment(){
fm = getFragmentManager();
FragmentTransaction transaction=fm.beginTransaction();
transaction.add(R.id.id_content,mTab01);
transaction.add(R.id.id_content,mTab02);
transaction.add(R.id.id_content,mTab03);
transaction.add(R.id.id_content,mTab04);
transaction.commit();
}
private void initView(){
mTabWechat=findViewById(R.id.LinearLayout_weixin);
mTabFriend = findViewById(R.id.LinearLayout_lianxiren);
mTabContact = findViewById(R.id.LinearLayout_pengyouquan);
mTabSettings = findViewById(R.id.LinearLayout_shezhi);
mimgWechat = findViewById(R.id.id_tab_wechat_image);
mimgFriend = findViewById(R.id.id_tab_friend_image);
mimgContact = findViewById(R.id.id_tab_contact_image);
mimgSettings = findViewById(R.id.id_tab_settings_image);
}
private void hideFragment(FragmentTransaction transaction){
transaction.hide(mTab01);
transaction.hide(mTab02);
transaction.hide(mTab03);
transaction.hide(mTab04);
}
private void resetImgs(){
mimgWechat.setImageResource(R.drawable.p1);
mimgFriend.setImageResource(R.drawable.p2);
mimgContact.setImageResource(R.drawable.p3);
mimgSettings.setImageResource(R.drawable.p4);
}
private void selectfragment(int i){
FragmentTransaction transaction=fm.beginTransaction();
hideFragment(transaction);
switch (i){
case 0:
transaction.show(mTab01);
mimgWechat.setImageResource(R.drawable.p1_press);
break;
case 1:
transaction.show(mTab02);
mimgFriend.setImageResource(R.drawable.p2_press);
break;
case 2:
transaction.show(mTab03);
mimgContact.setImageResource(R.drawable.p3_press);
break;
case 3:
transaction.show(mTab04);
mimgSettings.setImageResource(R.drawable.p4_press);
break;
default:
break;
}
transaction.commit();
}
public void onClick(View v){
resetImgs();
switch (v.getId()){
case R.id.LinearLayout_weixin:
selectfragment(0);
break;
case R.id.LinearLayout_lianxiren:
selectfragment(1);
break;
case R.id.LinearLayout_pengyouquan:
selectfragment(2);
break;
case R.id.LinearLayout_shezhi:
selectfragment(3);
break;
}
}
}
七、其余文件
1、weixinFragment
package com.example.mywork;
import android.os.Bundle;
import android.app.Fragment;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
public class weixinFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
public weixinFragment() {
}
public static weixinFragment newInstance(String param1, String param2) {
weixinFragment fragment = new weixinFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_weixin, container, false);
}
}
2、lianxirenFragment
package com.example.mywork;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.app.Fragment;
public class lianxirenFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
public lianxirenFragment() {
}
public static weixinFragment newInstance(String param1, String param2) {
weixinFragment fragment = new weixinFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_lianxiren, container, false);
}
}
3、pengyouquanFragment
package com.example.mywork;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.app.Fragment;
public class pengyouquanFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
public pengyouquanFragment() {
}
public static weixinFragment newInstance(String param1, String param2) {
weixinFragment fragment = new weixinFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_pengyouquan, container, false);
}
}
4、shezhiFragment
package com.example.mywork;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.app.Fragment;
public class shezhiFragment extends Fragment {
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
private String mParam1;
private String mParam2;
public shezhiFragment() {
}
public static weixinFragment newInstance(String param1, String param2) {
weixinFragment fragment = new weixinFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_shezhi, container, false);
}
}
八、运行界面
 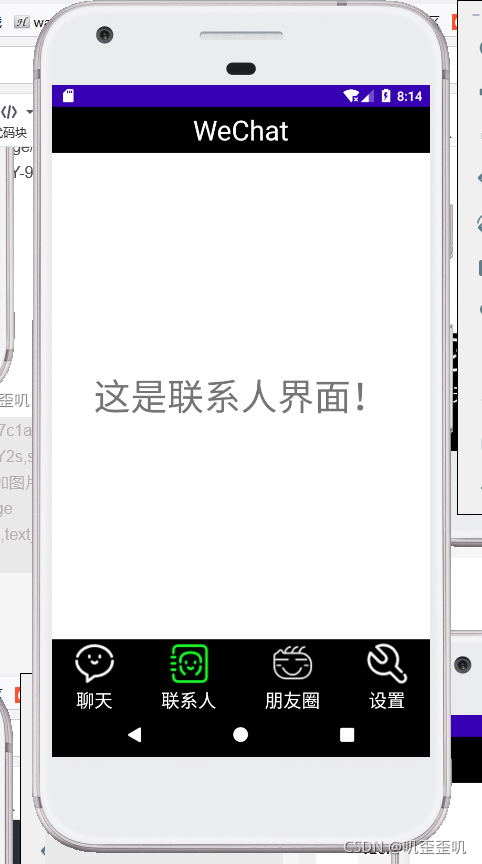
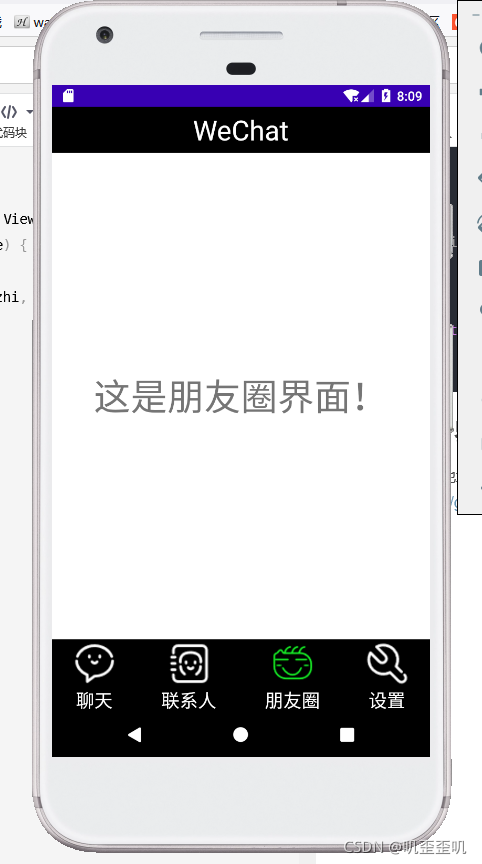
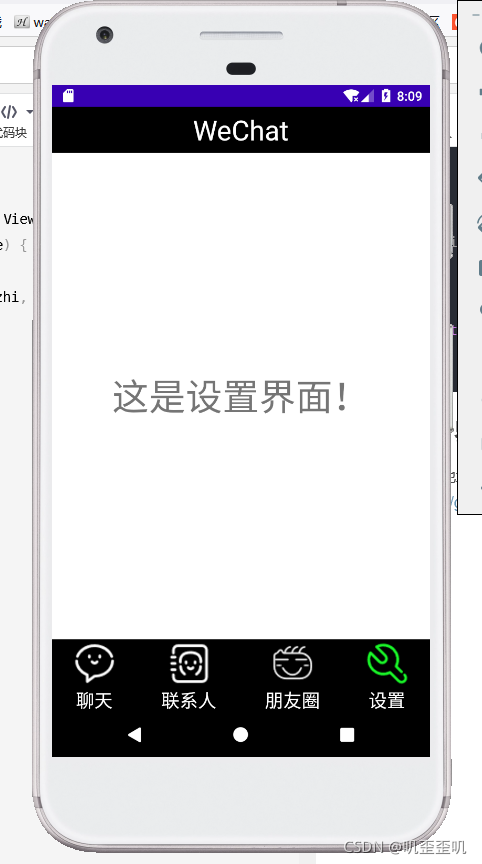
源码GitHub地址: 链接: https://github.com/Tsundere12/Mywork01.git
|