要求:写一个网上测试小程序,包含填空题、多选题、单选题和判断题,最后进行评分。
jsp
设计一个表单,里面包含两道填空题、两道单选题、一道判断题、一道多选题及一个提交按钮。表单采用POST提交方法,将数据提交到test2中进行运算,得出得分。
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>基础知识在线测试</title>
<link rel="stylesheet" href="<%=request.getContextPath() %>/css/test2.css">
</head>
<body>
<div id="main">
<div id="title" style="margin:20px 200px;">
<h2>在线测试</h2>
</div>
<form action="test2" method="post">
<div class="test">
<label>1、面向对象语言的三大特性有多态、继承和</label>
<input type="text" value="" name="t1" class="input-text">(10分)
</div>
<div class="test">
<label>2、Java中修饰类或者属性为抽象的关键字是</label>
<input type="text" value="" name="t2" class="input-text">(10分)
</div>
<div class="test">
<label>3、Java属于下列哪种语言?(20分)</label><br>
<input type="radio" value="A" name="t3">汇编语言
<input type="radio" value="B" name="t3">机器语言
<input type="radio" value="C" name="t3">高级语言
<input type="radio" value="D" name="t3">自然语言
</div>
<div class="test">
<label>4、下列哪个是HTML中的行内元素?(20分)</label><br>
<input type="radio" value="A" name="t4">form
<input type="radio" value="B" name="t4">p
<input type="radio" value="C" name="t4">audio
<input type="radio" value="D" name="t4">button
</div>
<div class="test">
<label>5、一个负数的补码还是它本身。(10分)</label><br>
<input type="radio" value="true" name="t5">对
<input type="radio" value="false" name="t5">错
</div>
<div class="test">
<label>6、下列哪些属于面向对象的程序语言?(30分)</label><br>
<input type="checkbox" value="A" name="t6">C
<input type="checkbox" value="B" name="t6">Java
<input type="checkbox" value="C" name="t6">C++
<input type="checkbox" value="D" name="t6">C#
</div>
<div class="test" style="margin:20px 200px;">
<input type="submit" name="submit" value="提交" class="submit">
</div>
</form>
</div>
</body>
</html>
servlet
编写一个计算得分的servlet。获取表单提交的数据,计算出结果并输出到页面。
package com.bnuz;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/Test2")
public class Test2 extends HttpServlet {
private static final long serialVersionUID = 1L;
public Test2() {
super();
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
response.setContentType("text/html;charset=utf-8");
request.setCharacterEncoding("UTF-8");
int total_score = 0;
String[] answer = { "封装", "abstract", "C", "D", "false" };
int[] score = { 10, 10, 20, 20, 10 };
for (int i = 1; i <= answer.length; i++) {
String t = request.getParameter("t" + i);
if (answer[i - 1].equals(t)) {
total_score += score[i - 1];
}
}
String[] t6 = request.getParameterValues("t6");
if (t6.length == 3) {
if ("B".equals(t6[0]) && "C".equals(t6[1]) && "D".equals(t6[2])) {
total_score += 30;
}
}
PrintWriter out = response.getWriter();
out.print("您本次的得分为:" + total_score);
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
doGet(request, response);
}
}
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://xmlns.jcp.org/xml/ns/javaee" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd" id="WebApp_ID" version="4.0">
<display-name>Demo2</display-name>
<welcome-file-list>
<welcome-file>index.html</welcome-file>
<welcome-file>index.htm</welcome-file>
<welcome-file>index.jsp</welcome-file>
<welcome-file>default.html</welcome-file>
<welcome-file>default.htm</welcome-file>
<welcome-file>default.jsp</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>Test2</servlet-name>
<servlet-class>com.bnuz.Test2</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>Test2</servlet-name>
<url-pattern>/test2</url-pattern>
</servlet-mapping>
</web-app>
css
#main{
width:580px;
margin:0 auto;
height:600px;
}
.test{
margin-top:15px;
}
.input-text{
border:0;
border-bottom:1px solid black;
width:70px;
}
.submit{
width:100px;
height:35px;
background-color: rgb(145, 233, 255);
}
运行结果
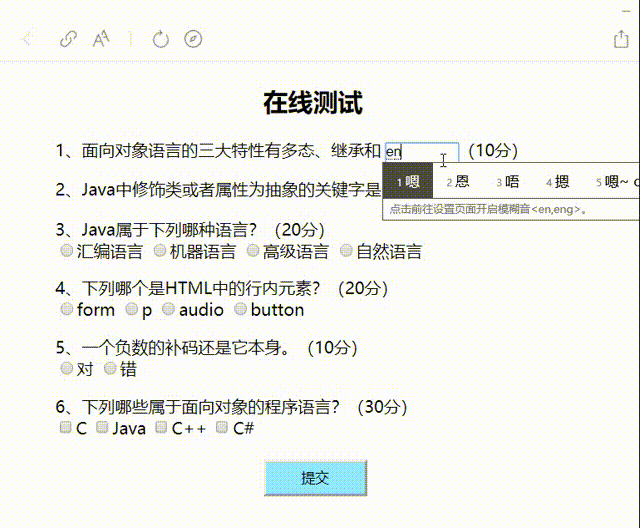 ━━( ̄ー ̄*|||━━菜鸟一枚,如有问题,欢迎指出。
|