1. 单子布局组件
1.1. Align组件
1.1.1. Align介绍
const Align({
Key key,
this.alignment: Alignment.center, // 对齐方式,默认居中对齐
this.widthFactor, // 宽度因子,不设置的情况,会尽可能大
this.heightFactor, // 高度因子,不设置的情况,会尽可能大
Widget child // 要布局的子Widget
})
alignment :
- 范围是(-1, 1),设置位置;
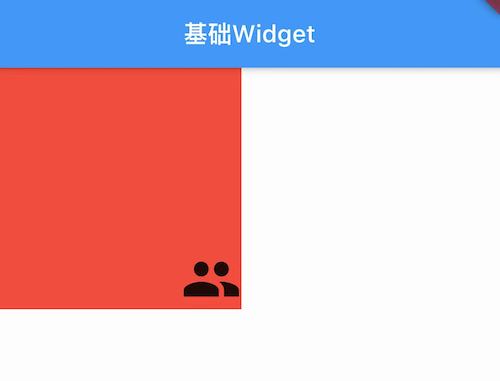
@override
Widget build(BuildContext context) {
return Container(
width: 200,
height: 200,
color: Colors.red,
child: Align(
child: Icon(Icons.people, size: 50,),
alignment: Alignment(1, 1),
),
);
widthFactor 和heightFactor 作用:
- 因为子组件在父组件中的对齐方式必须有一个前提,就是父组件得知道自己的范围(宽度和高度);
- 如果widthFactor和heightFactor不设置,那么默认Align会尽可能的大(尽可能占据自己所在的父组件);
- 如果widthFactor设置为3,那么相对于Align的宽度是子组件跨度的3倍;
1.2 Center组件
1.2.1. Center介绍
Center组件继承自Align,只是将alignment设置为Alignment.center。
class Center extends Align {
const Center({
Key key,
double widthFactor,
double heightFactor,
Widget child
}) : super(key: key, widthFactor: widthFactor, heightFactor: heightFactor, child: child);
}
class MyHomeBody extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Center(
child: Icon(Icons.people, size: 36, color: Colors.red),
widthFactor: 3,
heightFactor: 3,
);
}
}
1.3. Padding组件
1.3.1. Padding 介绍
Padding通常用于设置子Widget到父Widget的边距(父组件的内边距或子Widget的外边距)。
const Padding({
Key key,
@required this.padding, // EdgeInsetsGeometry类型(抽象类),使用EdgeInsets
Widget child,
})
1.3.2. 实例
class _YZHomeContentState extends State<YZHomeContent> {
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(8.0),
//padding: const EdgeInsets.fromLTRB(10, 5, 8, 20),
child: Text("Hello word", style: TextStyle(fontSize: 20, backgroundColor: Colors.red)),
);
}
}
1.4. Container组件
Container组件类似于其他Android中的View,iOS中的UIView。
1.4.1. Container介绍
Container在开发中被使用的频率是非常高的,特别是我们经常会将其作为容器组件。
Container({
this.alignment,
this.padding, //容器内补白,属于decoration的装饰范围
Color color, // 背景色
Decoration decoration, // 背景装饰
Decoration foregroundDecoration, //前景装饰
double width,//容器的宽度
double height, //容器的高度
BoxConstraints constraints, //容器大小的限制条件
this.margin,//容器外补白,不属于decoration的装饰范围
this.transform, //变换
this.child,
})
1.4.2 实例
class _YZHomeContentState extends State<YZHomeContent> {
@override
Widget build(BuildContext context) {
return Container(
color: Colors.red,
width: 200,
height: 200,
alignment: Alignment(-1, -1),
padding: EdgeInsets.all(20),
margin: EdgeInsets.all(20),
child: Icon(Icons.favorite, size: 50, color: Colors.white,),
// transform: Matrix4.rotationZ(100),//旋转
);
}
}
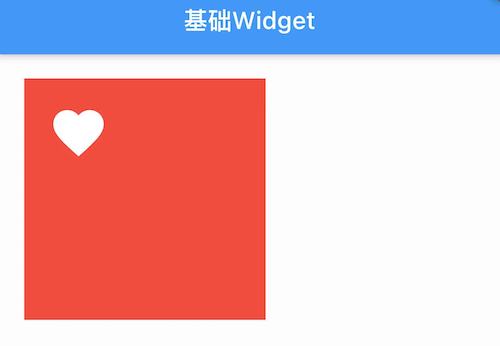
1.4.3 BoxDecoration
Container有一个非常重要的属性:decoration ;
- 在开发中,我们经常使用它的实现类
BoxDecoration 来进行实例化; - 源码:
const BoxDecoration({
this.color, // 颜色,会和Container中的color属性冲突
this.image, // 背景图片
this.border, // 边框,对应类型是Border类型,里面每一个边框使用BorderSide
this.borderRadius, // 圆角效果
this.boxShadow, // 阴影效果
this.gradient, // 渐变效果
this.backgroundBlendMode, // 背景混合
this.shape = BoxShape.rectangle, // 形变
})
示例: -添加边框,圆角,阴影;
- 注意:如果在
decoration 中添加颜色,需要把外部的颜色赋值去掉;
class _YZHomeContentState extends State<YZHomeContent> {
@override
Widget build(BuildContext context) {
return Container(
// color: Colors.red,
width: 200,
height: 200,
alignment: Alignment(-1, -1),
padding: EdgeInsets.all(20),
margin: EdgeInsets.all(20),
child: Icon(Icons.favorite, size: 50, color: Colors.white,),
// transform: Matrix4.rotationZ(100),//旋转
decoration: BoxDecoration (
color: Colors.red,
border: Border.all(
width: 5,
color: Colors.blue,
),
borderRadius: BorderRadius.circular(20),
boxShadow: [
BoxShadow(color: Colors.black,offset: Offset(10,10), spreadRadius: 5, blurRadius: 100),
],
),
);
}
}
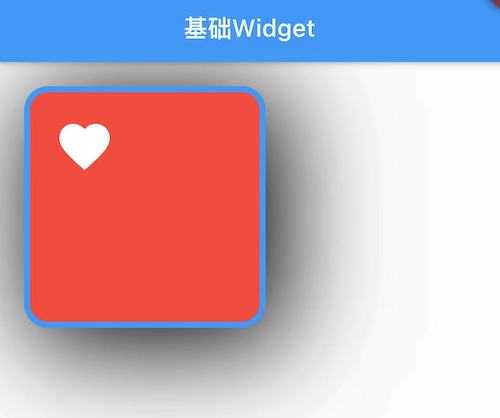
|