一、开发软件:Android studio
二、内容:
1、掌握UI设计中的layout布局(约束布局)与基本控件(button、text、imageview等);
2、掌握复杂控件与adapter的使用;
3、对有recycleView的页面进行点击跳转设计;
要实现列表,在上次实验的基础上,选择在 fragment_weixin上添加 recyclerview。将原来的 TextView 删除,再添加一个 recyclerview
在 myadapter 中需要重写三个方法:
重写 onCreateViewHolder :获取 view,并传入 MyViewHolder,最后返回一个 MyViewHolder
重写 onBindViewHolder :设置 holder 中的数据
重写 getItemCount :用于返回条目个数
添加 recyclerview 后,新建一个对应的 item_down.xml 文件,设计 recyclerview 内元素的 xml 样 式
创建适配器 RecyclerView.Adapter
完成适配器后,修改对应的 weixinFragment.java 文件
至此列表完成,接下来是进行跳转设计
在适配器里我们要加入点击监听事件,然后修改weixinFragment.java
在点击第一个事件时,会跳转到Main2Activity,点击其余事件则显示“在线”
然后写activity_main2.xml文件进行布局
在AndroidManifest.xml中添加
<activity android:name=".Main2Activity" />
部分代码如下:
myadapter.java
package com.example.mywork;
import android.content.Context;
import android.content.Intent;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.ImageView;
import android.widget.TextView;
import androidx.annotation.NonNull;
import androidx.collection.CircularArray;
import androidx.recyclerview.widget.RecyclerView;
import java.util.List;
public class myadapter extends RecyclerView.Adapter<myadapter.Myviewholder> {
private static OnRecyclerItemClickListener onItemClickListener;
private List<chat> mList;
private Context context;
public myadapter(Context context,List<chat> list) {
this.mList= list;
this.context=context;
}
public myadapter() {
}
@NonNull
@Override
public Myviewholder onCreateViewHolder(@NonNull ViewGroup parent, int viewType){
View view=(View)LayoutInflater.from(parent.getContext()).inflate(R.layout.item_down,parent,false);
Myviewholder myviewholder= new Myviewholder((view));
return myviewholder;
}
@Override
public void onBindViewHolder(@NonNull Myviewholder holder, int position) {
chat chat = mList.get(position);
holder.Image.setImageResource(chat.getImageid());
holder.Name.setText(chat.getName());
holder.Message.setText(chat.getMessage());
// holder.itemView.setOnClickListener(new View.OnClickListener() {
// @Override
// public void onClick(View v) {
// Intent intent=new Intent(context,frdFragment.class);
// context.startActivities(new Intent[]{intent});
// }
// });
}
@Override
public int getItemCount() {
return mList.size();
}
public class Myviewholder extends RecyclerView.ViewHolder{
private ImageView Image;
private TextView Name;
private TextView Message;
public Myviewholder(@NonNull View itemView) {
super(itemView);
Image = itemView.findViewById(R.id.item_img);
Name = itemView.findViewById(R.id.item_name);
Message = itemView.findViewById(R.id.item_message);
itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(onItemClickListener!=null){
onItemClickListener.onRecyclerItemClick(getAdapterPosition());
}
}
});
}
}
public static void setRecyclerItemClickListener(OnRecyclerItemClickListener listener){
onItemClickListener=listener;
}
public interface OnRecyclerItemClickListener{
void onRecyclerItemClick(int position);
}
}
weixinFragment.java
package com.example.mywork;
import android.app.Activity;
import android.content.Context;
import android.content.Intent;
import android.os.Bundle;
import android.app.Fragment;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.Toast;
import androidx.recyclerview.widget.DividerItemDecoration;
import androidx.recyclerview.widget.LinearLayoutManager;
import androidx.recyclerview.widget.RecyclerView;
import java.util.ArrayList;
import java.util.List;
public class weixinFragment extends Fragment {
private Context context;
private List<chat> mList = new ArrayList<chat>();
// TODO: Rename parameter arguments, choose names that match
// the fragment initialization parameters, e.g. ARG_ITEM_NUMBER
private static final String ARG_PARAM1 = "param1";
private static final String ARG_PARAM2 = "param2";
// TODO: Rename and change types of parameters
private String mParam1;
private String mParam2;
public weixinFragment() {
// Required empty public constructor
}
// TODO: Rename and change types and number of parameters
public static weixinFragment newInstance(String param1, String param2) {
weixinFragment fragment = new weixinFragment();
Bundle args = new Bundle();
args.putString(ARG_PARAM1, param1);
args.putString(ARG_PARAM2, param2);
fragment.setArguments(args);
return fragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (getArguments() != null) {
mParam1 = getArguments().getString(ARG_PARAM1);
mParam2 = getArguments().getString(ARG_PARAM2);
}
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
View view= inflater.inflate(R.layout.fragment_weixin, container, false);
context = (Activity) view.getContext();
InitData();
RecyclerView recyclerView = view.findViewById(R.id.recyclerview);
myadapter adapter = new myadapter(context,mList);
recyclerView.setAdapter(adapter);
LinearLayoutManager manager = new LinearLayoutManager(context);
manager.setOrientation(LinearLayoutManager.VERTICAL);
recyclerView.setLayoutManager(manager);
recyclerView.addItemDecoration(new DividerItemDecoration(context,LinearLayoutManager.VERTICAL ));
myadapter.setRecyclerItemClickListener(new myadapter.OnRecyclerItemClickListener() {
@Override
public void onRecyclerItemClick(int position) {
Toast.makeText(getActivity(),"在线",Toast.LENGTH_LONG).show();
if (position==0){
Intent intent=new Intent(getActivity(),Main2Activity.class);
startActivity(intent);}
}
});
return view;
}
private void InitData() {
for (int i = 0; i < 10; i++) {
chat friend_1 = new chat(i, R.drawable.friend_1, "刘婧","我们一起出去玩吧");
mList.add(friend_1);
chat friend_2= new chat(i, R.drawable.friend_2, "高妍","你想去看电影吗");
mList.add(friend_2);
chat friend_3 = new chat(i, R.drawable.friend_3, "占明润","可好看了");
mList.add(friend_3);
chat friend_4 = new chat(i, R.drawable.friend_4, "陈飞宇","哈哈哈哈笑死了");
mList.add(friend_4);
chat friend_5 = new chat(i, R.drawable.friend_5, "徐洲","冲冲冲");
mList.add(friend_5);
chat friend_6 = new chat(i, R.drawable.friend_6, "黄林海","哎哟");
mList.add(friend_6);
chat friend_7 = new chat(i, R.drawable.friend_7, "胡凯","那就行");
mList.add(friend_7);
chat friend_8 = new chat(i, R.drawable.friend_8, "陈聪颖","去打球吧");
mList.add(friend_8);
chat friend_9 = new chat(i, R.drawable.friend_9, "余畅","我也觉得");
mList.add(friend_9);
chat friend_10 = new chat(i, R.drawable.friend_10, "余梦珂","莫?");
mList.add(friend_10);
}
}
}
activity_main2.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#ffffff">
<!--左侧的图片布局盒子-->
<LinearLayout
android:id="@+id/ll_1"
android:layout_width="wrap_content"
android:layout_height="125dp"
android:gravity="center">
<ImageView
android:id="@+id/item_img"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_margin="10dp"
android:src="@drawable/friend_1"
android:background="#DFDFDF"/>
</LinearLayout>
<!--右侧文字盒子布局-->
<LinearLayout
android:id="@+id/ll_2_name"
android:layout_width="match_parent"
android:layout_height="125dp"
android:orientation="vertical">
<!--名字TextView所在盒子布局-->
<LinearLayout
android:id="@+id/ll_2_name1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_marginBottom="10dp">
<TextView
android:id="@+id/item_name"
android:layout_width="190dp"
android:layout_height="25dp"
android:text="刘婧"
android:textSize="20sp"
android:textColor="#000000" />
</LinearLayout>
<LinearLayout
android:id="@+id/ll_2_message"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp">
<TextView
android:id="@+id/item_message"
android:layout_width="190dp"
android:layout_height="25dp"
android:text="我们一起出去玩吧"
android:textSize="20sp"
android:textColor="#000000" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
fragment_weixin.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="horizontal">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerview"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="8dp" />
</LinearLayout>
item_dowm.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="#ffffff">
<!--左侧的图片布局盒子-->
<LinearLayout
android:id="@+id/ll_1"
android:layout_width="wrap_content"
android:layout_height="125dp"
android:gravity="center">
<ImageView
android:id="@+id/item_img"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_margin="10dp"
android:src="@drawable/friend_a"
android:background="#DFDFDF"/>
</LinearLayout>
<!--右侧文字盒子布局-->
<LinearLayout
android:id="@+id/ll_2_name"
android:layout_width="match_parent"
android:layout_height="125dp"
android:orientation="vertical">
<!--名字TextView所在盒子布局-->
<LinearLayout
android:id="@+id/ll_2_name1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_marginBottom="10dp">
<TextView
android:id="@+id/item_name"
android:layout_width="190dp"
android:layout_height="25dp"
android:text=""
android:textSize="20sp"
android:textColor="#000000" />
</LinearLayout>
<LinearLayout
android:id="@+id/ll_2_message"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp">
<TextView
android:id="@+id/item_message"
android:layout_width="190dp"
android:layout_height="25dp"
android:text=""
android:textSize="20sp"
android:textColor="#000000" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
三、运行界面展示
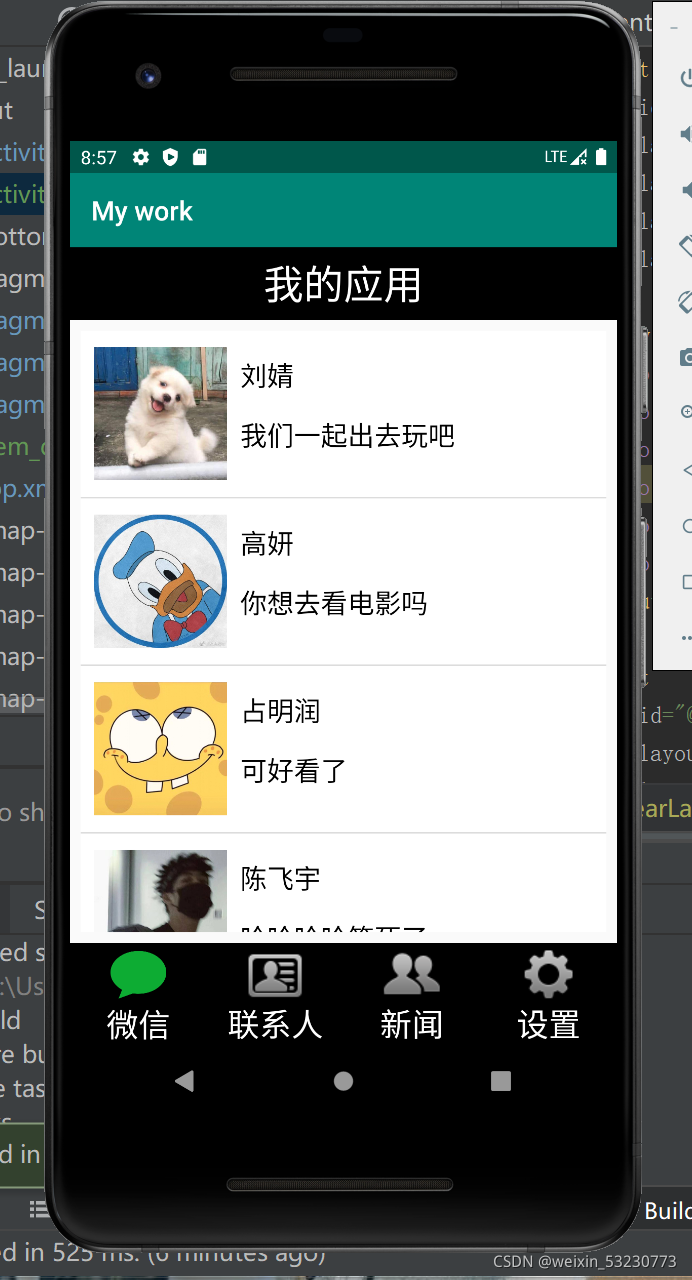
?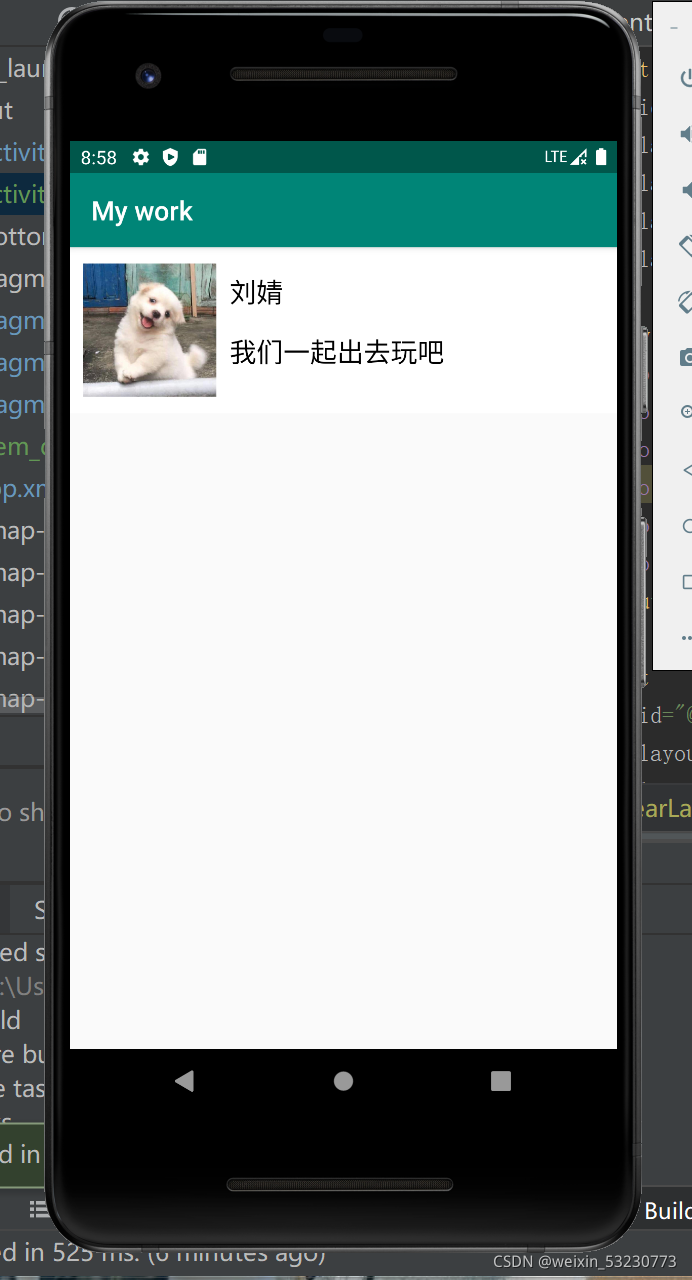
?四、源码的代码仓库地址
代码仓库
|