介绍
笔者自己封装的一个flutter组件,用于展示网格数据。可以传入默认展示的数目,网格的列数,展开和收起时显示的文字等。需注意的是,该组件需要传入一个itemBuilder 函数,这个函数有context和index两个参数,即网格的子项构造函数。还需要传入一个包含所有网格数据的数组。网格的子项宽高比childAspectRatio 也是可以传入的。
效果图
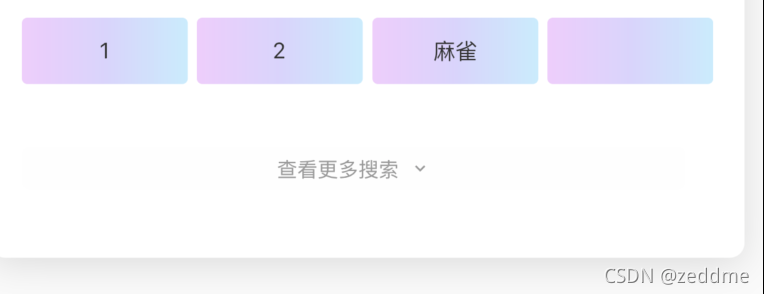 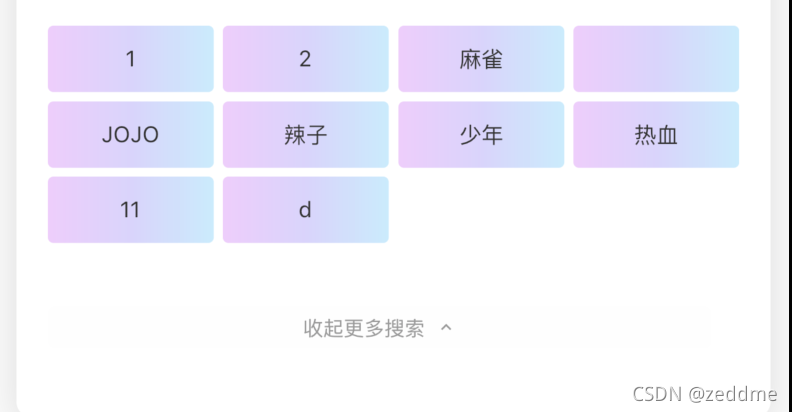
源码
import 'package:flutter/material.dart';
class MoreData extends StatefulWidget {
final List<dynamic> list;
final int firstShowCount;
final int crossCount;
final double childAspectRatio;
final IndexedWidgetBuilder itemBuilder;
final String expandedText;
final String tightText;
const MoreData({
Key? key,
required this.list,
required this.firstShowCount,
required this.itemBuilder,
this.childAspectRatio = 2.5,
this.crossCount = 4,
this.expandedText = '收起',
this.tightText = '查看更多',
}) : super(key: key);
@override
_MoreDataState createState() => _MoreDataState();
}
class _MoreDataState extends State<MoreData> {
int currentListLength = 0;
bool isExpanded = false;
@override
void initState() {
super.initState();
currentListLength = widget.list.length > widget.firstShowCount
? widget.firstShowCount
: widget.list.length;
}
@override
Widget build(BuildContext context) {
return getMoreData();
}
Widget getMoreData() {
if (widget.list.length > widget.firstShowCount) {
return Column(
children: [
getList(),
getButton(),
],
);
}
return getList();
}
Widget getList() {
return GridView.builder(
physics: const NeverScrollableScrollPhysics(),
shrinkWrap: true,
itemCount: currentListLength,
itemBuilder: widget.itemBuilder,
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
childAspectRatio: widget.childAspectRatio,
crossAxisCount: widget.crossCount,
mainAxisSpacing: 5,
crossAxisSpacing: 5,
),
);
}
Widget getButton() {
return GestureDetector(
onTap: () {
if (isExpanded) {
setState(() {
currentListLength = widget.firstShowCount;
});
} else {
setState(() {
currentListLength = widget.list.length;
});
}
setState(() {
isExpanded = !isExpanded;
});
},
child: Container(
height: 23,
margin: const EdgeInsets.only(right: 15, bottom: 20),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(4),
color: const Color(0xfffefefe),
),
alignment: Alignment.center,
child: Row(
mainAxisSize: MainAxisSize.min,
children: [
Text(
isExpanded ? widget.expandedText : widget.tightText,
style: const TextStyle(
fontSize: 11,
color: Color(0xff999999),
),
),
const SizedBox(width: 6),
Icon(
isExpanded ? Icons.keyboard_arrow_up : Icons.keyboard_arrow_down,
size: 11,
color: const Color(0xff999999),
),
],
),
),
);
}
}
|