ObjectAnimator动画使用
实现如图效果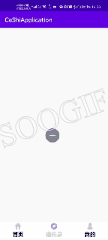
- 首先现在xml中定义布局
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
tools:context=".fragment.AddressFragment">
<ImageView
android:id="@+id/iv_show_menu"
android:layout_width="48dp"
android:layout_height="47dp"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="10dp"
android:layout_marginRight="10dp"
android:minHeight="40dp"
android:minWidth="40dp"
android:src="@drawable/home_menu_add" />
<ImageView
android:id="@+id/iv_add_one"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="10dp"
android:layout_marginRight="14dp"
android:background="@android:color/transparent"
android:minHeight="40dp"
android:minWidth="40dp"
android:src="@mipmap/home_menu_photo"
android:visibility="invisible"
/>
<ImageView
android:id="@+id/iv_scan"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_alignParentRight="true"
android:layout_marginBottom="10dp"
android:layout_marginRight="14dp"
android:background="@android:color/transparent"
android:minHeight="40dp"
android:minWidth="40dp"
android:src="@mipmap/home_menu_scan"
android:visibility="invisible"
/>
</RelativeLayout>
2.java代码实现 我是在一个fragment当中实现
package com.example.ceshiapplication.fragment;
import android.animation.Animator;
import android.animation.ObjectAnimator;
import android.animation.PropertyValuesHolder;
import android.nfc.Tag;
import android.os.Bundle;
import androidx.fragment.app.Fragment;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import com.example.ceshiapplication.R;
public class AddressFragment extends Fragment implements View.OnClickListener {
private ImageView iv_show_menu;
private ImageView iv_add_one;
private ImageView iv_scan;
private boolean isShow = false;
private PropertyValuesHolder translationY1;
private PropertyValuesHolder translationY2;
private PropertyValuesHolder scaleX;
private PropertyValuesHolder scaleY;
private PropertyValuesHolder translationY3;
private PropertyValuesHolder translationY4;
private PropertyValuesHolder scaleX_;
private PropertyValuesHolder scaleY_;
private PropertyValuesHolder rotation;
private PropertyValuesHolder rotation_;
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container,
Bundle savedInstanceState) {
// Inflate the layout for this fragment
View view = inflater.inflate(R.layout.fragment_address, container, false);
initView(view);
return view;
}
private void initView(View view) {
iv_show_menu = view.findViewById(R.id.iv_show_menu);
iv_show_menu.setOnClickListener(this);
iv_add_one = view.findViewById(R.id.iv_add_one);
iv_scan = view.findViewById(R.id.iv_scan);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.iv_show_menu:
showView();
break;
}
}
private void showView() {
//设置其它两个的位置
int measuredHeight = iv_show_menu.getMeasuredHeight();
int transV1 = (int) (measuredHeight + measuredHeight * 0.2 + 10);
int transV2 = (int)(measuredHeight +measuredHeight*0.2)*2+10;
//使用PropertyValuesHolder来保存动画过程中所需要的操作的属性和属性所对应的值,通过ofFloat(Object target, String propertyName, float… values)构造的动画,ofFloat()的内部实现其实就是将传进来的参数封装成PropertyValuesHolder实例来保存动画状态。
//平移
translationY1 = PropertyValuesHolder.ofFloat("translationY", -transV1);
translationY2 = PropertyValuesHolder.ofFloat("translationY", -transV2);
//缩放
scaleX = PropertyValuesHolder.ofFloat("scaleX", 0f, 1f);
scaleY = PropertyValuesHolder.ofFloat("scaleY", 0f, 1f);
translationY3 = PropertyValuesHolder.ofFloat("translationY", transV1);
translationY4 = PropertyValuesHolder.ofFloat("translationY", transV2);
scaleX_ = PropertyValuesHolder.ofFloat("scaleX", 1f, 0f);
scaleY_ = PropertyValuesHolder.ofFloat("scaleY", 1f,0f);
//旋转
rotation = PropertyValuesHolder.ofFloat("rotation", 45);
rotation_ = PropertyValuesHolder.ofFloat("rotation", 0);
if (isShow) {
//隐藏
//ObjectAnimator给我们提供了一个设置PropertyValuesHolder实例的入口ofPropertyValuesHolder
ObjectAnimator showMenu = ObjectAnimator.ofPropertyValuesHolder(iv_show_menu,rotation_);
showMenu.setDuration(200);
showMenu.start();
ObjectAnimator addOne = ObjectAnimator.ofPropertyValuesHolder(iv_add_one,translationY3,scaleX_,scaleY_);
addOne.setDuration(200);
addOne.start();
ObjectAnimator scan = ObjectAnimator.ofPropertyValuesHolder(iv_scan,translationY4,scaleX_,scaleY_);
scan.setDuration(200);
scan.start();
scan.addListener(new Animator.AnimatorListener() {
@Override
public void onAnimationStart(Animator animation) {
}
@Override
public void onAnimationEnd(Animator animation) {
iv_add_one.setVisibility(View.INVISIBLE);
iv_scan.setVisibility(View.INVISIBLE);
}
@Override
public void onAnimationCancel(Animator animation) {
}
@Override
public void onAnimationRepeat(Animator animation) {
}
});
isShow = false;
} else {
//显示
iv_add_one.setVisibility(View.VISIBLE);
iv_scan.setVisibility(View.VISIBLE);
ObjectAnimator showMenu = ObjectAnimator.ofPropertyValuesHolder(iv_show_menu, rotation);
showMenu.setDuration(200);
showMenu.start();
ObjectAnimator addOne = ObjectAnimator.ofPropertyValuesHolder(iv_add_one, translationY1, scaleX, scaleY);
addOne.setDuration(200);
addOne.start();
ObjectAnimator scan = ObjectAnimator.ofPropertyValuesHolder(iv_scan, translationY2, scaleX, scaleY);
scan.setDuration(200);
scan.start();
isShow = true;
}
}
}
参数解释 调用接口
public static ObjectAnimator ofPropertyValuesHolder(Object target,PropertyValuesHolder... values)
- target:指需要执行动画的控件
- values:一个可变长参数,可以传进去一个或多个PropertyValuesHolder实例,因为每个PropertyValuesHolder实例都会针对一个属性做动画,所以如果传进去多个PropertyValuesHolder实例,将会对控件的多个属性同时做动画操作
|