线性布局-LinearLayout
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<LinearLayout
android:id="@+id/11_1"
android:layout_width="200dp" 宽度
android:layout_height="200dp" 高度
android:orientation="vertical" 排列方式
android:background="#000000" 背景颜色
android:paddingLeft="20dp" 内部元素排列方式-留白 内边距
android:paddingRight="20dp"
android:paddingBottom="10dp"
android:paddingTop="50dp"
android:layout_marginEnd="20dp"> 外部留白
<View
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#FF0033">
</View>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="200dp"
android:orientation="horizontal"
android:background="#0066FF"
android:layout_marginTop="20dp"
android:layout_marginLeft="15dp"
android:layout_marginRight="15dp">
<View
android:layout_width="0dp"
android:layout_height="match_parent"
android:background="#000000"
android:layout_weight="1"> 除却原来的宽度剩余空间的比例
</View>
<View
android:layout_width="0dp"
android:layout_height="match_parent"
android:background="#FF0033"
android:layout_weight="1">
</View>
<View
android:layout_width="0dp"
android:layout_height="match_parent"
android:background="#55AA99"
android:layout_weight="1">
</View>
</LinearLayout>
</LinearLayout>
相对布局-RelativeLayout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<View
android:id="@+id/view_1"
android:layout_width="100dp"
android:layout_height="100dp"
android:background="#000000">
</View>
<View
android:id="@+id/view_2"
android:layout_width="100dp"
android:layout_height="100dp"
android:background="#800000"
android:layout_toRightOf="@id/view_1"> 相对位置
</View>
<View
android:id="@+id/view_3"
android:layout_width="100dp"
android:layout_height="100dp"
android:background="#800000"
android:layout_below="@id/view_1"> 相对位置
</View>
<LinearLayout
android:id="@+id/ll_1"
android:layout_width="match_parent"
android:layout_height="200dp"
android:layout_below="@id/view_3"
android:orientation="horizontal"
android:background="#0066FF"
android:padding="15dp"> 内部组件留白
<View
android:layout_width="100dp"
android:layout_height="match_parent"
android:background="#FF0033">
</View>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#000000"
android:padding="15dp">
<View
android:id="@id/view_1"
android:layout_width="100dp"
android:layout_height="match_parent"
android:background="#FF9900">
</View>
<View
android:id="@id/view_2"
android:layout_width="100dp"
android:layout_height="match_parent"
android:layout_toRightOf="@id/view_1"
android:background="#FF9900"
android:layout_marginLeft="10dp"> 组件间的间隔
</View>
</RelativeLayout>
</LinearLayout>
</RelativeLayout>
TextView
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<TextView
android:id="@+id/tv_1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/tv_test1"
android:textColor="#000000" 颜色
android:textSize="24sp"> 大小
</TextView>
<TextView
android:id="@+id/tv_2"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:maxLines="1" 省略号 最多一行
android:ellipsize="end"
android:text="@string/tv_test1"
android:textColor="#000000"
android:textSize="24sp"
android:layout_marginTop="10dp">
</TextView>
<TextView
android:id="@+id/tv_3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="筛选"
android:drawableRight="@drawable/dog" 字+icon
android:drawablePadding="5dp"
android:textColor="#000000"
android:textSize="24sp"
android:layout_marginTop="10dp">
</TextView>
<TextView
android:id="@+id/tv_4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/tv_test1" 中划线
android:textColor="#000000"
android:textSize="24sp"
android:layout_marginTop="10dp">
</TextView>
<TextView
android:id="@+id/tv_5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/tv_test1"
android:textColor="#000000" 下划线
android:textSize="24sp"
android:layout_marginTop="10dp">
</TextView>
<TextView
android:id="@+id/tv_6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:textColor="#000000" 下划线
android:textSize="24sp"
android:layout_marginTop="10dp">
</TextView>
<TextView
android:id="@+id/tv_7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="张赟天下第一张赟天下第一张赟天下第一" 跑马灯
android:textColor="#000000"
android:textSize="24sp"
android:singleLine="true"
android:ellipsize="marquee"
android:marqueeRepeatLimit="marquee_forever"
android:focusable="true"
android:focusableInTouchMode="true"
android:clickable="true">
</TextView>
</LinearLayout>
package com.example.helloworld;
import androidx.appcompat.app.AppCompatActivity;
import android.graphics.Paint;
import android.os.Bundle;
import android.text.Html;
import android.widget.TextView;
public class TextViewActivity extends AppCompatActivity {
private TextView mTv4,mTv5,mTv6,mTv7;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_text_view);
mTv4 =(TextView) findViewById(R.id.tv_4);
mTv4.getPaint().setFlags(Paint.STRIKE_THRU_TEXT_FLAG);
mTv4.getPaint().setAntiAlias(true);
mTv5=(TextView) findViewById(R.id.tv_5);
mTv5.getPaint().setFlags(Paint.UNDERLINE_TEXT_FLAG);
mTv6=(TextView) findViewById(R.id.tv_6);
mTv5.setText(Html.fromHtml("<u>张赟天下第一</u>"));
mTv7=(TextView) findViewById(R.id.tv_7);
mTv7.setSelected(true);
}
}
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<Button
android:id="@+id/btn_textview"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="TextView">
</Button>
</LinearLayout>
package com.example.helloworld;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
private Button mBtnTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mBtnTextView=(Button) findViewById(R.id.btn_textview);
mBtnTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(MainActivity.this, TextViewActivity.class);
startActivity(intent);
}
});
}
}
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.helloworld">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.HelloWorld">
<activity
android:name=".TextViewActivity"
android:exported="false" />
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
- 运行结果
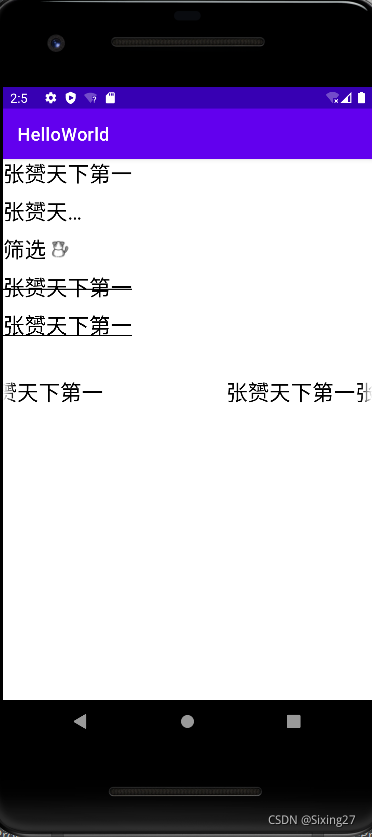
Button
简单登录界面
|