动画
最基本的动画使用方式
[UIView beginAnimation:nil context:nil];
[UIView setAnimationDuration:2];
self.btnIcon.center=centerPoint;
[UIView commitAnimations];
[UIView animateWithDuration:0.5 animations:^{
self.btnIcon.center=centerPoint;
}];
核心动画
Core Animation ,中文翻译为核心动画,,它是一组非常强大的动画处理API,使用它能做出非常炫丽的动画效果,而且往往是事半功倍。也就是说,使用少量的代码就可以实现非常强大的功能。 Core Animation 可以用在 Mac OS X 和iOS 平台。 Core Animation 的动画执行过程都是在后台操作的,不会阻塞主线程。 要注意的是, Core Animation 是直接作用在CALayer 上的,并非UIView 。
- 继承关系 有基本动画、帧动画、组动画、转场动画
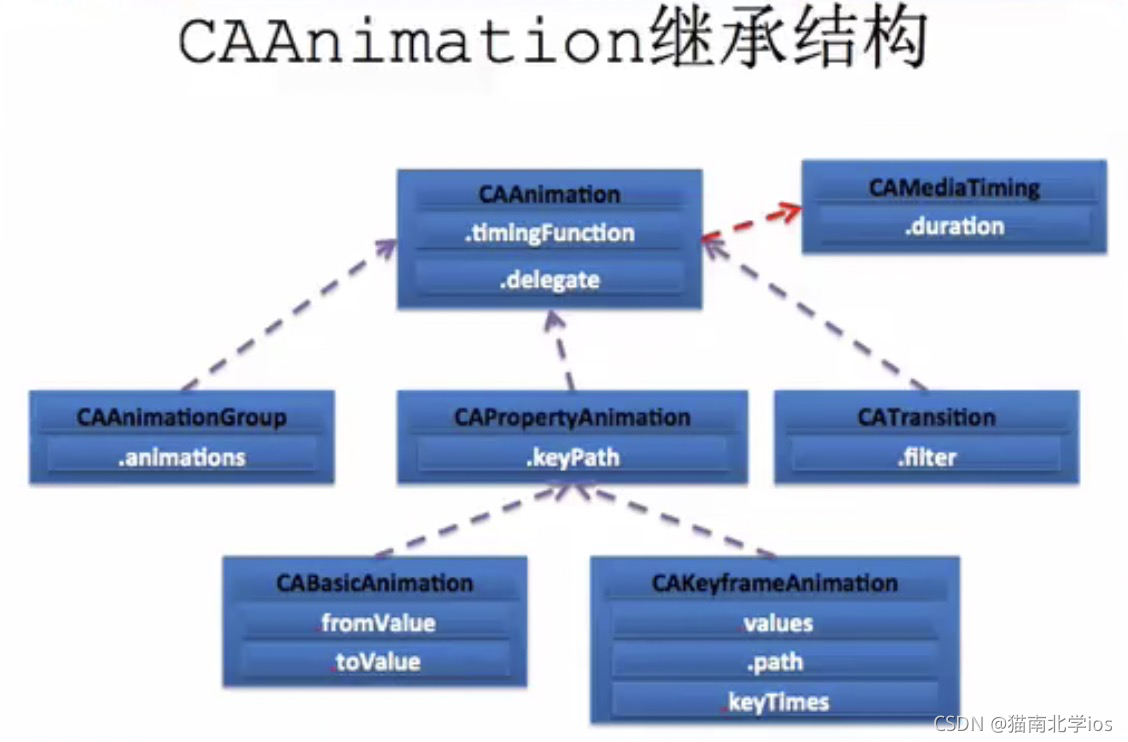
基本动画
- 核心动画结束后,默认会回到原来的位置,可以为根
layer 添加核心动画 - 属性:
keyPath 、fromValue 、toValue 、byValue
CABasicAnimation *basicAnim = [[CABasicAnimation alloc]init];
basicAnim.keyPath = @"position.x";
basicAnim.fromValue = @(10);
basicAnim.toValue = @(300);
basicAnim.fillMode = kCAFillModeForwards;
basicAnim.removedOnCompletion = NO;
[self.view.layer addAnimation:basicAnim forKey:nil];
关键帧动画
方式一:关键点
CAKeyframeAnimation *basicAnim = [[CAKeyframeAnimation alloc]init];
basicAnim.keyPath = @"position";
NSValue *v1 = [NSValue valueWithCGPoint:CGPointMake(100, 100)];
NSValue *v2 = [NSValue valueWithCGPoint:CGPointMake(150, 100)];
NSValue *v3 = [NSValue valueWithCGPoint:CGPointMake(100, 150)];
NSValue *v4 = [NSValue valueWithCGPoint:CGPointMake(150, 150)];
basicAnim.values = @[v1,v2,v3,v4];
basicAnim.duration = 5;
basicAnim.repeatCount = INT_MAX;
[self.view.layer addAnimation:basicAnim forKey:nil];
方式二:路径
CAKeyframeAnimation *basicAnim = [[CAKeyframeAnimation alloc]init];
basicAnim.keyPath = @"position";
UIBezierPath *path = [UIBezierPath bezierPathWithArcCenter:CGPointMake(150, 150) radius:100 startAngle:0 endAngle:2*M_PI clockwise:1];
basicAnim.path = path.CGPath;
basicAnim.duration = 2;
basicAnim.repeatCount = INT_MAX;
[self.view.layer addAnimation:basicAnim forKey:nil];
组动画
CABasicAnimation *basicAnim = [[CABasicAnimation alloc]init];
basicAnim.keyPath = @"position.rotation";
basicAnim.byValue = @(2*M_PI);
CAKeyframeAnimation *keyAnim = [[CAKeyframeAnimation alloc]init];
keyAnim.keyPath = @"position";
UIBezierPath *path = [UIBezierPath bezierPathWithArcCenter:CGPointMake(150, 150) radius:100 startAngle:0 endAngle:2*M_PI clockwise:1];
keyAnim.path = path.CGPath;
CAAnimationGroup *groupAnim = [[CAAnimationGroup alloc]init];
groupAnim.animations = @[basicAnim,keyAnim];
groupAnim.duration = 3;
groupAnim.repeatCount = INT_MAX;
[self.view.layer addAnimation:groupAnim forKey:nil];
转场动画
一般添加到UIImageView 里面
CATransition *anim = [[CATransition alloc]init];
anim.type = @"cube";
anim.subtype = kCATransitionFromLeft;
[self.imageView.layer addAnimation:anim forKey:nil];
动画效果:
fade:淡入淡出。不支持subType,设置无效。
moveIn:慢慢进入并覆盖原始。
push:推进效果。
reveal:揭开效果。
cube:立方体效果。
suckEffect:吮吸效果。不支持subType,设置无效。iOS13及以上版本不支持该动画了。
oglFlip:翻转效果。
rippleEffect:波纹效果。不支持subType,设置无效。iOS13及以上版本不支持该动画了。
pageCurl:翻页效果。
pageUnCurl:反翻页效果。
cameraIrisHollowOpen:开镜头效果。不支持subType,设置无效。iOS13及以上版本不支持该动画了。
cameraIrisHollowClose:关镜头效果。不支持subType,设置无效。iOS13及以上版本不支持该动画了。
|