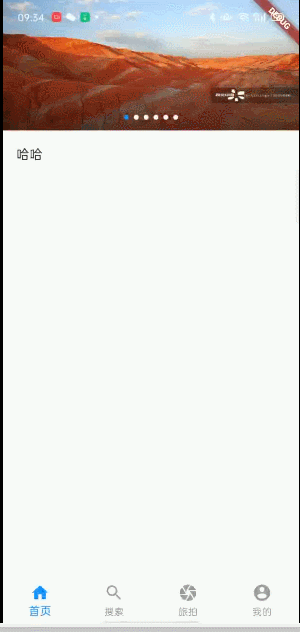 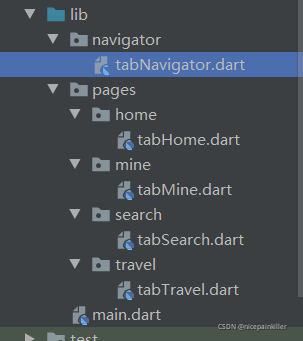
移除 状态栏底色
main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_trip/navigator/tabNavigator.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// SystemUiOverlayStyle uiStyle = SystemUiOverlayStyle.light.copyWith(
// statusBarColor: Colors.transparent,
// );
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
//设置状态栏背景透明
SystemChrome.setSystemUIOverlayStyle(SystemUiOverlayStyle.light.copyWith(
statusBarColor: Colors.transparent,
));
//强制竖屏
SystemChrome.setPreferredOrientations(
[DeviceOrientation.portraitUp, DeviceOrientation.portraitDown],
);
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: TabNavigator(),
);
}
}
bottomNavigationBar 配置
?tabNavigator.dart
import 'package:flutter/material.dart';
import 'package:flutter_trip/pages/home/tabHome.dart';
import 'package:flutter_trip/pages/mine/tabMine.dart';
import 'package:flutter_trip/pages/search/tabSearch.dart';
import 'package:flutter_trip/pages/travel/tabTravel.dart';
class TabNavigator extends StatefulWidget {
@override
_TabNavigatorState createState() => _TabNavigatorState();
}
class _TabNavigatorState extends State<TabNavigator> {
final List<Map> navigationBarArray = [
{
'title': '首页',
'icon': Icons.home,
'colorDefault': Colors.grey,
'colorSelect': Colors.blue
},
{
'title': '搜索',
'icon': Icons.search,
'colorDefault': Colors.grey,
'colorSelect': Colors.blue
},
{
'title': '旅拍',
'icon': Icons.camera,
'colorDefault': Colors.grey,
'colorSelect': Colors.blue
},
{
'title': '我的',
'icon': Icons.account_circle,
'colorDefault': Colors.grey,
'colorSelect': Colors.blue
}
];
int tabIndexCurrent = 0;
final PageController pageController = PageController(initialPage: 0);
// _TabNavigatorState({Key? key, required this.pageController})
// : super(key: key);
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
body: PageView(
controller: pageController,
children: [
TabHome(),
TabSearch(),
TabTravel(),
TabMine(),
],
),
bottomNavigationBar: BottomNavigationBar(
currentIndex: tabIndexCurrent,
type: BottomNavigationBarType.fixed,
onTap: (index) {
pageController.jumpToPage(index);
setState(() {
tabIndexCurrent = index;
});
},
items: navigationBarArray
.asMap()
.map(
(index, item) => MapEntry(
index,
BottomNavigationBarItem(
title: Text(
item['title'],
style: TextStyle(
color: index == tabIndexCurrent
? item['colorSelect']
: item['colorDefault']),
),
icon: Icon(
item['icon'],
color: item['colorDefault'],
),
activeIcon: Icon(
item['icon'],
color: item['colorSelect'],
))),
)
.values
.toList()),
);
}
}
上拉显示导航栏: swiper 插件
flutter_swiper_null_safety | Flutter Package
flutter_swiper_null_safety: ^1.0.2
tabHome.dart
import 'package:flutter/material.dart';
import 'package:flutter_swiper_null_safety/flutter_swiper_null_safety.dart';
class TabHome extends StatefulWidget {
@override
_TabHomeState createState() => _TabHomeState();
}
class _TabHomeState extends State<TabHome> {
final List imageArray = [
'https://tenfei05.cfp.cn/creative/vcg/800/new/VCG21gic13899040.jpg',
'https://tenfei05.cfp.cn/creative/vcg/800/new/VCG21gic17808815-ZKZ.jpg',
'https://alifei01.cfp.cn/creative/vcg/800/version23/VCG21gic5474093.jpg',
'https://tenfei03.cfp.cn/creative/vcg/800/version23/VCG41159337470.jpg',
'https://tenfei04.cfp.cn/creative/vcg/800/version23/VCG41162310340.jpg',
'https://tenfei04.cfp.cn/creative/vcg/800/version23/VCG41147366616.jpg'
];
double appBarOpacity = 0;
@override
Widget build(BuildContext context) {
// TODO: implement build
return Scaffold(
body: Stack(
children: [
MediaQuery.removePadding(
removeTop: true,
context: context,
child: NotificationListener(
onNotification: (ScrollNotification scrollNotification) {//不光监听父widget 还监听子widget监听
//列表滚动 并且只监听外部元素;
if (scrollNotification is ScrollUpdateNotification &&
scrollNotification.depth == 0) {
_onScroll(scrollNotification.metrics.pixels);
}
return true;
},
child: ListView(
children: [
Container(
height: 160,
child: Swiper(
autoplay: true,
itemCount: imageArray.length,
itemBuilder: (BuildContext content, int index) {
return Image.network(
imageArray[index],
fit: BoxFit.fill,
);
},
pagination: SwiperPagination(
builder: DotSwiperPaginationBuilder(
size: 6,
activeSize: 6,
),
),
),
),
Container(
height: 1888,
child: ListTile(
title: Text('哈哈'),
),
)
],
),
),
),
Opacity(
opacity: appBarOpacity,
child: Container(
height: 80,
decoration: BoxDecoration(
color: Colors.white,
),
child: Center(
child: Padding(
padding: EdgeInsets.only(top: 20),
child: Text('首页'),
),
),
),
)
],
),
);
}
bool _onScroll(double pixels) {
print('---)$pixels');
double opacity = pixels / 100;
if (opacity < 0) {
opacity = 0;
} else if (opacity > 1) {
opacity = 1;
}
setState(() {
appBarOpacity = opacity;
});
return true;
}
}
?
MediaQuery.removePadding(//移除安全区域
removeTop: true,
context: context,
child: NotificationListener(//可以监听滑动的组件
onNotification: (ScrollNotification scrollNotification) {//不光监听父widget 还监听子widget监听
//列表滚动 并且只监听外部元素;
if (scrollNotification is ScrollUpdateNotification &&
scrollNotification.depth == 0) {
_onScroll(scrollNotification.metrics.pixels);
}
return true;
},
|