实现效果
下拉刷新 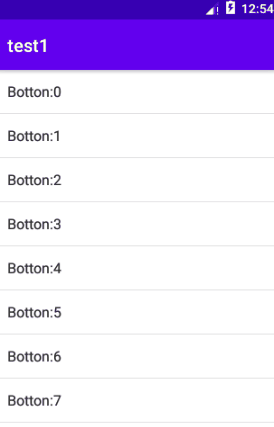 上拉加载 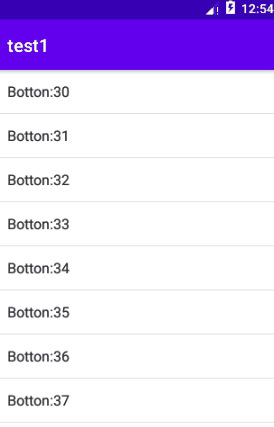
1. 准备环境
Android Studio 2020.3.1  (1) 给项目安装 androidx.swiperefreshlayout:swiperefreshlayout:1.1.0-beta01 布局组件 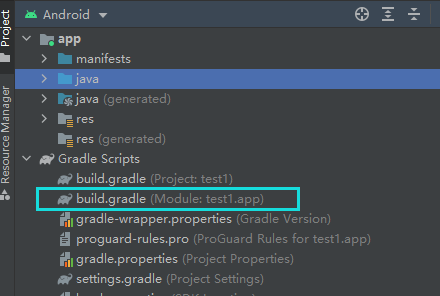 (2) 在 dependencies依赖中添加一行
implementation 'androidx.swiperefreshlayout:swiperefreshlayout:1.1.0-beta01'
(3) 点击 Sync 同步,完成安装 笔者使用的是1.1.0 beta01版本,只要保证在这个版本之上应该都适用,更多关于这个布局的使用,请参考官方API Android开发文档,
2. 布局
2.1 foot_view
foot_view 用于显示上拉加载时,出现的画面提示,到时候会添加到列表框的底部
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
>
<TextView
android:id="@+id/foot_view_tv"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:text="加载更多..."
/>
</LinearLayout>
ftoo_view.xml 布局的效果图: 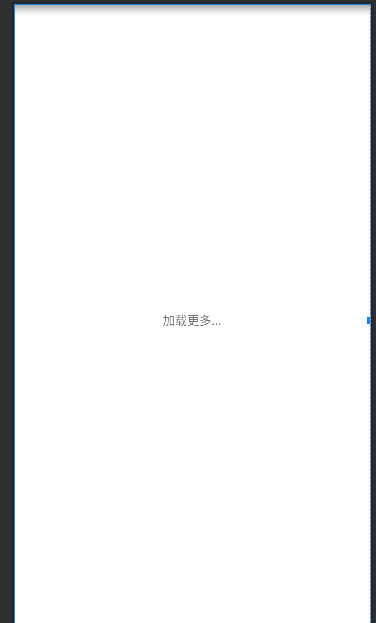
2.2 activity_main
activity_main.xml 应用主要的布局 ,在 SwipeRefreshLayout布局里嵌套了一个 ListView
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.swiperefreshlayout.widget.SwipeRefreshLayout
android:id="@+id/refresh"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
<ListView
android:id="@+id/listview"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
</androidx.swiperefreshlayout.widget.SwipeRefreshLayout>
</LinearLayout>
3.代码实现
3.1 全局变量
public class MainActivity extends AppCompatActivity implements SwipeRefreshLayout.OnRefreshListener, AbsListView.OnScrollListener{
ArrayList<String> list_all;
ArrayList<String> list_lv;
ArrayAdapter<String> adapter;
SwipeRefreshLayout refreshLayout;
ListView lv;
View foot;
final int MAX_PAGE = 5;
final int PER_PAGE = 15;
int page = 0;
}
3.2 OnCreate
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
lv = findViewById(R.id.listview);
refreshLayout = findViewById(R.id.refresh);
list_all = new ArrayList<>();
for (int i = 0; i < PER_PAGE * MAX_PAGE; i++)
list_all.add(String.format("Botton:%d", i));
list_lv = new ArrayList<>(list_all.subList(0, PER_PAGE));
adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, list_lv);
foot = LayoutInflater.from(this).inflate(R.layout.foot_view,null);
lv.setAdapter(adapter);
lv.setOnScrollListener(this);
refreshLayout.setOnRefreshListener(this);
}
3.3 自定义的showInfo方法
public void showPageInfo(){
Toast.makeText(MainActivity.this, String.format("当前是第%d页",page), Toast.LENGTH_SHORT).show();
}
3.4 下拉刷新
@Override
public void onRefresh() {
page = ((page == MAX_PAGE - 1) ? 0 : page + 1);
list_lv.clear();
list_lv.addAll(list_all.subList(page * PER_PAGE, (page+1) * PER_PAGE - 1));
System.out.println(list_lv.toString());
adapter.notifyDataSetChanged();
showPageInfo();
refreshLayout.setRefreshing(false);
}
3.5 上拉加载
@Override
public void onScrollStateChanged(AbsListView absListView, int i) {
int last =lv.getLastVisiblePosition();
int first = lv.getFirstVisiblePosition();
int num = lv.getCount();
if(last == num - 1 && SCROLL_STATE_IDLE == i)
lv.addFooterView(foot);
if(num == PER_PAGE + 1){
lv.removeFooterView(foot);
onRefresh();
lv.setSelection(0);
}
}
@Override
public void onScroll(AbsListView absListView, int i, int i1, int i2) { }
4. 所有代码
package com.example.test1;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
import androidx.swiperefreshlayout.widget.SwipeRefreshLayout;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.AbsListView;
import android.widget.Adapter;
import android.widget.ArrayAdapter;
import android.widget.ListView;
import android.widget.TextView;
import android.widget.Toast;
import java.util.ArrayList;
public class MainActivity extends AppCompatActivity implements SwipeRefreshLayout.OnRefreshListener, AbsListView.OnScrollListener{
ArrayList<String> list_all;
ArrayList<String> list_lv;
ArrayAdapter<String> adapter;
SwipeRefreshLayout refreshLayout;
ListView lv;
View foot;
final int MAX_PAGE = 5;
final int PER_PAGE = 15;
int page = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
lv = findViewById(R.id.listview);
refreshLayout = findViewById(R.id.refresh);
list_all = new ArrayList<>();
for (int i = 0; i < PER_PAGE * MAX_PAGE; i++)
list_all.add(String.format("Botton:%d", i));
list_lv = new ArrayList<>(list_all.subList(0, PER_PAGE));
adapter = new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, list_lv);
foot = LayoutInflater.from(this).inflate(R.layout.foot_view,null);
lv.setAdapter(adapter);
lv.setOnScrollListener(this);
refreshLayout.setOnRefreshListener(this);
}
public void showPageInfo(){
Toast.makeText(MainActivity.this, String.format("当前是第%d页",page), Toast.LENGTH_SHORT).show();
}
@Override
public void onRefresh() {
page = ((page == MAX_PAGE - 1) ? 0 : page + 1);
list_lv.clear();
list_lv.addAll(list_all.subList(page * PER_PAGE, (page+1) * PER_PAGE - 1));
System.out.println(list_lv.toString());
adapter.notifyDataSetChanged();
showPageInfo();
refreshLayout.setRefreshing(false);
}
@Override
public void onScrollStateChanged(AbsListView absListView, int i) {
int last =lv.getLastVisiblePosition();
int first = lv.getFirstVisiblePosition();
int num = lv.getCount();
if(last == num - 1 && SCROLL_STATE_IDLE == i)
lv.addFooterView(foot);
if(num == PER_PAGE + 1){
lv.removeFooterView(foot);
onRefresh();
lv.setSelection(0);
}
}
@Override
public void onScroll(AbsListView absListView, int i, int i1, int i2) { }
}
|