首先需要创建apk密钥:
执行命令:keytool -genkey -v -keystore /D:/key.jks -keyalg RSA -keysize 2048 -validity 10000 -alias key
根据提示输入密钥信息
输入密钥库口令:
密钥库口令太短 - 至少必须为 6 个字符
输入密钥库口令:
再次输入新口令:
您的名字与姓氏是什么?
[Unknown]: wanghf
您的组织单位名称是什么?
[Unknown]: vk
您的组织名称是什么?
[Unknown]: wx
您所在的城市或区域名称是什么?
[Unknown]: sh
您所在的省/市/自治区名称是什么?
[Unknown]: sh
该单位的双字母国家/地区代码是什么?
[Unknown]: china
CN=wanghf, OU=vk, O=wx, L=sh, ST=sh, C=china是否正确?
[否]: y
正在为以下对象生成 2,048 位RSA密钥对和自签名证书 (SHA256withRSA) (有效期为 10,000 天):
CN=wanghf, OU=vk, O=wx, L=sh, ST=sh, C=china
输入 <key> 的密钥口令
(如果和密钥库口令相同, 按回车):
再次输入新口令:
[正在存储/D:/key.jks]
创建密钥文件,名字可以随便取: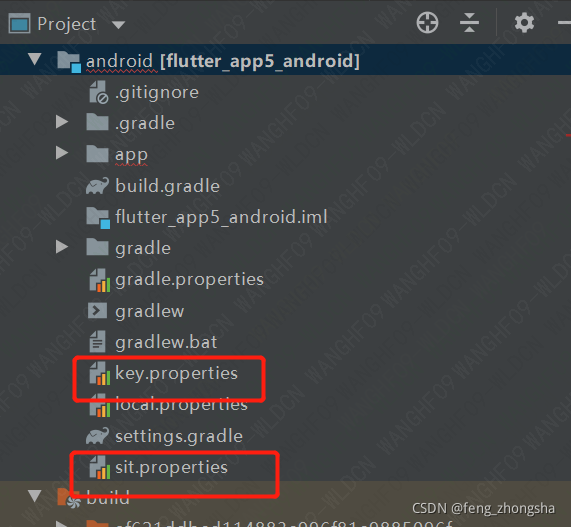
?配置密钥信息:
storePassword=12345
keyPassword=12345
keyAlias=key
storeFile=D:/sit.jks
build.gradle 配置密钥信息及打包渠道
def localProperties = new Properties()
def localPropertiesFile = rootProject.file('local.properties')
if (localPropertiesFile.exists()) {
localPropertiesFile.withReader('UTF-8') { reader ->
localProperties.load(reader)
}
}
def flutterRoot = localProperties.getProperty('flutter.sdk')
if (flutterRoot == null) {
throw new GradleException("Flutter SDK not found. Define location with flutter.sdk in the local.properties file.")
}
def flutterVersionCode = localProperties.getProperty('flutter.versionCode')
if (flutterVersionCode == null) {
flutterVersionCode = '1'
}
def flutterVersionName = localProperties.getProperty('flutter.versionName')
if (flutterVersionName == null) {
flutterVersionName = '1.0'
}
apply plugin: 'com.android.application'
apply plugin: 'kotlin-android'
apply from: "$flutterRoot/packages/flutter_tools/gradle/flutter.gradle"
//新增==============
def keystorePropertiesFile = rootProject.file("key.properties")
def keystoreProperties = new Properties()
keystoreProperties.load(new FileInputStream(keystorePropertiesFile))
def sitKeystorePropertiesFile = rootProject.file("sit.properties")
def sitKeystoreProperties = new Properties()
sitKeystoreProperties.load(new FileInputStream(sitKeystorePropertiesFile))
//============================================
android {
compileSdkVersion 30
sourceSets {
main.java.srcDirs += 'src/main/kotlin'
}
defaultConfig {
// TODO: Specify your own unique Application ID (https://developer.android.com/studio/build/application-id.html).
applicationId "com.example.flutter_app5"
minSdkVersion 16
targetSdkVersion 30
versionCode flutterVersionCode.toInteger()
versionName flutterVersionName
}
//新增==========================
signingConfigs {
sit {
keyAlias sitKeystoreProperties['keyAlias']
keyPassword sitKeystoreProperties['keyPassword']
storeFile file(sitKeystoreProperties['storeFile'])
storePassword sitKeystoreProperties['storePassword']
}
release {
keyAlias keystoreProperties['keyAlias']
keyPassword keystoreProperties['keyPassword']
storeFile file(keystoreProperties['storeFile'])
storePassword keystoreProperties['storePassword']
}
}
buildTypes {
debug {
minifyEnabled false
signingConfig signingConfigs.sit
ndk {
abiFilters "armeabi","armeabi-v7a","arm64-v8a", "x86"
}
}
release {
minifyEnabled true
zipAlignEnabled true
shrinkResources true
signingConfig signingConfigs.release
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
ndk {
abiFilters "armeabi","armeabi-v7a","arm64-v8a", "x86"
}
}
}
flavorDimensions "flutter_app5"
productFlavors {
sit{
dimension "flutter_app5"
applicationId "${defaultConfig.applicationId}.sit"
manifestPlaceholders = [
app_name: "flutter_sit"
]
}
uat{
dimension "flutter_app5"
applicationId "${defaultConfig.applicationId}.uat"
manifestPlaceholders = [
app_name: "flutter_uat"
]
}
production{
dimension "flutter_app5"
applicationId "${defaultConfig.applicationId}.production"
manifestPlaceholders = [
app_name: "flutter_production"
]
}
}
}
//==============
flutter {
source '../..'
}
dependencies {
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk7:$kotlin_version"
}
至此渠道已经完成
但是需求需要不同环境引入不同的URL,我们可以创建多个main.dart
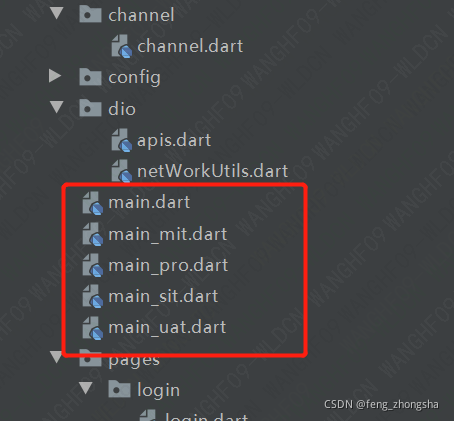
?在我们的main文件中不同的环境配置不同的URL,所以我们需要一个枚举类
enum APPChannel {
MIT,
SIT,
UAT,
PRO,
}
class Channel {
static APPChannel currentChannel;
static String get baseURL {
switch (currentChannel) {
case APPChannel.MIT:
return 'https://mit.111.com/';
case APPChannel.SIT:
return 'https://sit.222.com/';
case APPChannel.UAT:
return 'https://uat.333.com/';
case APPChannel.PRO:
return 'https://pro.444.com/';
default:
return 'https://sit.111.com/';
}
}
static String get baseAuthURL {
switch (currentChannel) {
case APPChannel.MIT:
return 'https://mitauth.111.com/';
case APPChannel.SIT:
return 'https://sitauth.222.com/';
case APPChannel.UAT:
return 'https://uatauth.333.com/';
case APPChannel.PRO:
return 'https://proauth.444.com/';
default:
return 'https://sitauth.111.com/';
}
}
}
在main函数中配置读取
Channel.currentChannel = APPChannel.MIT;
?不同环境配置不同的取值: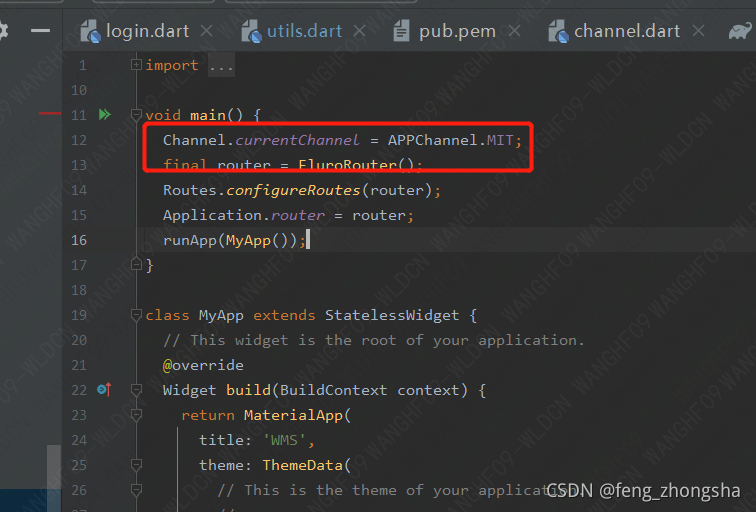
?在API文件获取对应的URL
import 'package:flutter_app2/channel/channel.dart';
class APIs{
static final String API_SERVER_URL = Channel.baseURL;
static final String API_SERVER_URL_AUTH = Channel.baseAuthURL;
static const String QUERYVERSION = "queryVersion";
static const String LOGIN = "login";
static const String CHANGE_OBJECT = "changeObject";
}
至此配置完成
命令运行不同环境的包
flutter build apk --flavor uat --debug -t lib/main_uat.dart
--flavor dev指定好渠道
--debug 指定好环境
-t lib/main_uat.dart? 指定好入口文件
至此就可以根据需求打相应的包了
参考文档:打包dev环境命令_解决Flutter在android端多渠道打包问题_蒙氏宝宝的博客-CSDN博客
|