Kotlin 不是 Swift on JVM
- Swift 有 sum type, Kotlin 用 sealed class 来模拟, 但易用性真的不敢恭维, 写起来要加不少莫名其妙的字符.
// Kotlin
sealed class Expr
data class Const(val number: Double) : Expr()
data class Sum(val e1: Expr, val e2: Expr) : Expr()
object NotANumber : Expr()
fun eval(expr: Expr): Double = when(expr) {
is Const -> expr.number
is Sum -> eval(expr.e1) + eval(expr.e2)
NotANumber -> Double.NaN
}
fun main() {
println(eval(Sum(Const(1.0), Const(2.0)))) // --> 3.0
}
这是 Swift 的写法 :
// Swift
indirect enum Expr {
case nan
case const(Double)
case sum(Expr, Expr)
}
func eval(_ expr: Expr) -> Double {
switch (expr) {
case .nan:
return Double.nan
case let .const(x):
return x
case let .sum(x, y):
return eval(x) + eval(y)
}
}
print(eval(.sum(.const(1.0), .const(2.0)))) // --> 3.0
// Kotlin
val (state, msg) = 404 to "not found."
val (state, msg) = Pair(404, "not found.")
println("error: $state ($msg)")
这是 Swift (以及所有正常语言) 的写法 :
// Swift
let (state, msg) = (404, "not found.")
print("error: \(state) (\(msg))")
// Kotlin
val cube = arrayListOf(
arrayListOf(1, 2, 3),
arrayListOf(4, 5, 6),
arrayListOf(7, 8, 9)
)
Swift 里是这样的
// Swift
let cube = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
- Swift 能自定义运算符 (包括前缀, 中缀和后缀, 优先级), Kotlin 不知道是不是觉得这个东西复杂度太高, 只能允许中缀表示法.
// Kotlin
infix fun Double.sumOfSquare(rhs: Double): Double {
return this * this + rhs * rhs
}
val x = 2.0 sumOfSquare 3.0
Swift 中是这样的
// Swift
infix operator *+*
func *+*(lhs: Double, rhs: Double) -> Double {
return lhs * lhs + rhs * rhs
}
2.0 *+* 3.0
- Swift 有 inout 参数, 可以直接改变函数的参数, 而 Kotlin (很多语言也) 没有
// Swift
infix operator <->
func <-><T>(lhs: inout T, rhs: inout T) {
return swap(&lhs, &rhs)
}
var (a, b) = (1, 2)
a <-> b
print("a, b = \(a), \(b)") // a, b = 2, 1
// Kotlin
val sum = arrayListOf(1, 4, 5, 2, 3).reduce { x, y -> x + y } // 15
Swift 里可以直接用裸的 +
// Swift
let sum = [1, 4, 5, 2, 3].reduce(0, +) // 15
而 Kotlin 里那些语法特性, Swift 里当然有 :
extension Int {
func times(_ fn: () -> Void) {
(1...self).forEach { _ in fn() }
}
}
3.times {
print("Hi!")
}
(1...100).map { $0 * $0 }
.filter { $0 % 2 == 1 }
let x: Int? = nil
x?.distance(to: 5) // nil
let y = x ?? 0 // 默认值
if let it = x { // if let
// ...
}
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? 需要更多教程,微信扫码即可
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ??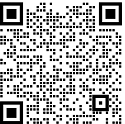
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?👆👆👆
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? 别忘了扫码领资料哦【高清Java学习路线图】
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?和【全套学习视频及配套资料】 ?
|