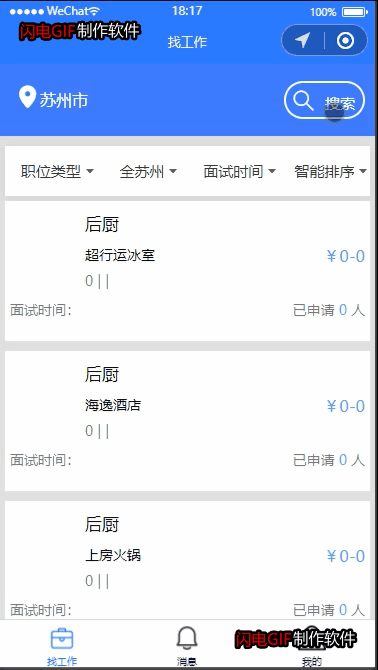
search.js
// pages/job/search/search.js
var util = require('../../../utils/util.js');
Page({
/**
* 页面的初始数据
*/
data: {
searchValue: '',
searchList: [],
isShow: true, //控制标签的是否可删除
isTag: true, //控制标签的显示与隐藏
isBtn: true, //控制删除按钮的显示与隐藏
list: [], //查询列表
showList: false,
},
//点击tag标签删除
tagClick(e) {
var a = e.currentTarget.dataset.index
let searchList = this.data.searchList;
searchList.splice(a, 1);
this.setData({
searchList: searchList
})
wx.setStorageSync('searchList', searchList)
},
giveInput(e) {
let newIndex = e.currentTarget.dataset.index;
let newSearch = this.data.searchList[newIndex];
this.setData({
searchValue: newSearch
})
},
onChange(e) {
this.setData({
searchValue: e.detail
})
},
//搜索
onSearch() {
let page = this;
var inputValue = this.data.searchValue;
var searchList = this.data.searchList || [];
if (inputValue !== "") {
//示例通用接口 获取列表信息
wx.request({
url: '此处省略哈/tag/getlist',//列表接口
data: {
table: 'job_position', //表名
keywords: inputValue,
fields: 'name'
},
method: 'GET',
success(res) {
console.log(res.data.data);
let returnData = res.data.data;
page.setData({
list: returnData,
})
if (returnData.length > 0) {
page.setData({
showList: true,
})
} else {
page.setData({
showList: false,
})
}
}
})
//若是输入的值不存在数组中则添加
if (!searchList.includes(inputValue)) {
//将搜索值放入历史记录中,只能放前五条
if (searchList.length < 5) {
searchList.unshift(inputValue)
} else {
searchList.pop() //删掉旧的时间最早的第一条
searchList.unshift(inputValue)
}
//将历史记录数组整体储存到缓存中
wx.setStorageSync('searchList', searchList)
}
} else {
wx.showToast({
title: '请输入搜索内容',
icon: 'error',
duration: 1500,
})
page.setData({
showList: false,
})
}
},
//前往职位详情页面
toJobDetail: util.throttle(function (e) {
let id = e.currentTarget.dataset.id;
wx.navigateTo({
url: "/pages/job/jobDetail/jobDetail?id="+id,
})
}, 1000),
// 删除图标
searchDelete() {
this.setData({
isShow: false,
isBtn: false
})
},
//完成按钮
finishClick() {
this.setData({
isShow: true,
isBtn: true
})
},
//全部删除按钮
deleteAll() {
wx.clearStorageSync('searchList')
this.setData({
searchList: []
})
},
/**
* 生命周期函数--监听页面加载
*/
onLoad: function (options) {
let localData = wx.getStorageSync('searchList');
this.setData({
searchList: localData
})
},
})
search.html
<view>
<van-search background="rgb(49, 121, 255)" value="{{searchValue}}" bind:change="onChange" shape="round"
placeholder="输入职位 / 公司 / 标签" bind:search="onSearch" use-action-slot>
<view slot="action" bind:tap="onSearch" class="search_color">搜索</view>
</van-search>
<!-- 查询列表 -->
<view wx:for="{{list}}" wx:key="index" wx:if="{{showList}}" class="margin_top">
<view class="job_list" bindtap="toJobDetail" data-id="{{item.id}}">
<view class="job_list_top">
<view class="job_list_top_left">
<image class="job_list_top_left_image" src="{{item.logo}}"></image>
</view>
<view class="job_list_top_right">
<view class="job_flex job_list_top_right_out">
<view class="job_list_top_right_job">{{item.jobName}}</view>
<!-- <view class="job_list_top_right_zhaopin">{{item.jobFair}}</view> -->
<view></view>
</view>
<view class="job_flex job_list_top_center">
<view class="job_list_top_right_name">{{item.name}}</view>
<view class="job_list_top_right_xinzi">¥{{item.min}}-{{item.max}}</view>
</view>
<view class="job_list_top_bottom job_list_top_right_name"><text>{{item.area}}</text> | <text>{{item.education}}</text> | <text>{{item.experience}}</text></view>
</view>
</view>
<view class="job_list_footer job_flex">
<view class="job_list_footer_time">
<text>面试时间:</text><text class="job_list_footer_time_color">{{item.face}}</text>
<!-- <text>面试时间:</text><text class="job_list_footer_time_color">短信通知</text> -->
</view>
<view>已申请 <text class="job_list_top_right_xinzi">{{item.applyNum}}</text> 人</view>
<!-- 若是申请后,则显示 -->
<!-- <view class="job_list_footer_applied">已申请</view> -->
</view>
</view>
</view>
<view class="search_history" wx:if="{{searchList.length>0 && !showList}}">
<view>历史搜索</view>
<view wx:if="{{isBtn}}">
<van-icon name="delete-o" size="45rpx" bindtap="searchDelete" />
</view>
<view wx:else class="search_btn"><text bindtap="deleteAll">全部删除 </text>|<text class="search_btn_active" bindtap="finishClick"> 完成</text></view>
</view>
<view class="search_list" wx:for="{{searchList}}" wx:key="index">
<van-tag class="search_list_tag" size="large" plain wx:if="{{isShow}}" bindtap="giveInput" data-index="{{index}}">{{item}}</van-tag>
<van-tag class="search_list_tag" size="large" closeable wx:else plain bindtap="tagClick" data-index="{{index}}">{{item}}</van-tag>
</view>
</view>
search.wxss
page {
background-color: rgba(204, 204, 204, 0.596078431372549);
}
.search_color{
color: #fff;
}
.search_history {
display: flex;
justify-content: space-between;
margin: 20rpx;
}
.search_list {
margin: 20rpx;
}
.search_list_tag {
float: left;
margin: 10rpx;
}
.search_btn{
font-size: 13px;
}
.search_btn_active{
color:rgb(49, 121, 255)
}
.margin_top{
margin-top:10px;
}
.job_list {
margin: 0 auto;
box-sizing: border-box;
margin-bottom: 20rpx;
width: calc(100% - 20rpx);
/* border-radius: 10rpx; */
background-color: #fff;
height: 280rpx;
padding-left: 10rpx;
padding-right: 10rpx;
box-sizing: border-box;
}
.job_list_top {
display: flex;
justify-content: space-between;
}
.job_list_top_left_image {
width: 140rpx;
height: 140rpx;
}
.job_list_top_right {
width: 560rpx;
}
.job_flex {
display: flex;
justify-content: space-between;
}
.job_list_top_right_out {
margin-top: 20rpx;
}
.job_list_top_right_job {
font-size: 34rpx;
height: 48rpx;
line-height: 48rpx;
width: 440rpx;
Text-overflow: ellipsis;
white-space: nowrap;
overflow: hidden;
}
.job_list_top_right_zhaopin {
font-size: 24rpx;
text-align: center;
height: 48rpx;
line-height: 48rpx;
color: #429EFD;
border: 2rpx solid #429EFD;
width: 90rpx;
}
.job_list_top_right_name {
font-size: 28rpx;
}
.job_list_top_right_xinzi {
color: #429EFD;
}
.job_list_top_center {
margin-top: 20rpx;
}
.job_list_top_bottom {
margin-top: 10rpx;
color: rgba(51, 51, 51, 0.690196078431373);
}
.job_list_footer {
margin-top: 20rpx;
font-size: 28rpx;
color: rgba(51, 51, 51, 0.690196078431373);
}
.job_list_footer_time_color {
color: #FFA535;
}
.job_list_footer_applied {
color: #fff;
background-color: rgba(153, 153, 153, 1);
width: 132rpx;
height: 52rpx;
border-radius: 30rpx;
text-align: center;
line-height: 52rpx;
}
search.json
{
"usingComponents": {
"van-search": "@vant/weapp/search/index",
"van-icon":"@vant/weapp/icon/index",
"van-tag":"@vant/weapp/tag/index"
},
"navigationBarTitleText": "搜索"
}
util.js
//节流,防止短时间内点击多次导致页面跳转多次
const throttle = (fn, delay) => {
let oldDate = Date.now();
return function () {
let args = arguments;
let newDate = Date.now();
let that = this;
if (newDate - oldDate > delay) {
fn.apply(that, args);
//倘若时间差大于延长时间 就更新一次旧时间
oldDate = Date.now();
}
}
}
module.exports = {
throttle: throttle,
}
|