SimpelDialog:
用于设置简单的提示信息,可以显示附加的提示或操作
属性表:
属性 | 作用 |
---|
title | 对话框标题 | titlePadding | 标题间隔 | titletextStyle | 标题文字样式 | children | 子组件选项 | contentPadding | 子选项间隔 | backgroundColor | 对话框背景颜色 | shap | 对话框形状 | elevation | 对话框阴影 | semanticLabel | 对话框语义标签,用来给读屏软件识别 |
子组件一般配合SimpleDialog选项使用,用于将组件包装,相当于textButton,文本是左对齐的,并且padding较小
实现代码如下:
?void main() => runApp(MyApp());
??
?class MyApp extends StatelessWidget
?{
??
? ?@override
? ?Widget build(BuildContext context) {
? ? ?return MaterialApp(
? ? ? ?title: "简单对话框",
? ? ? ?home: Scaffold(
? ? ? ? ?appBar: AppBar(
? ? ? ? ? ?title:const Text("简单对话框"),
? ? ? ? ),
? ? ? ? ?body: Container(
? ? ? ? ? ?child: SimpleDialog(
? ? ? ? ? ? ?//列表标题
? ? ? ? ? ? ?title: const Text("对话框标题"),
? ? ? ? ? ? ?children: <Widget>[
? ? ? ? ? ? ? ?//列表项
? ? ? ? ? ? ? ?SimpleDialogOption(
? ? ? ? ? ? ? ? ?child: const Text("第一行信息"),
? ? ? ? ? ? ? ? ?//监听事件
? ? ? ? ? ? ? ? ?onPressed: (){},
? ? ? ? ? ? ? ),
? ? ? ? ? ? ? ?SimpleDialogOption(
? ? ? ? ? ? ? ? ?child: const ?Text("第二行信息"),
? ? ? ? ? ? ? ? ?onPressed: (){},
? ? ? ? ? ? ? )
? ? ? ? ? ? ],
? ? ? ? ? ),
? ? ? ? ),
? ? ? ),
? ? );
? }
?}
?
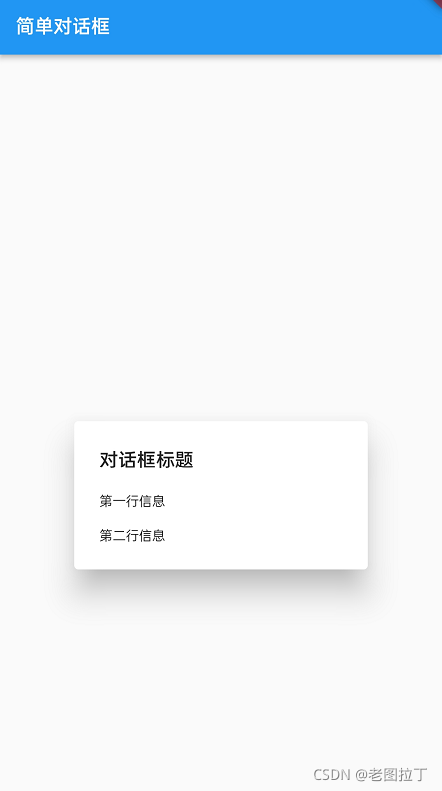
AlertDialog:
一般带有确定和取消的提示框
参数:
属性名 | 类型 | 说明 |
---|
title | Widget | 对话框标题,通常是一个文本 | action | List<Widget> | 对话框底部的操作按钮,比如确定、取消等 |
?AlertDialog(
? ?//标题
? ?title: const Text("提示"),
? ?//一个可滚动组件
? ?content: SingleChildScrollView(
? ? ?//ListBody是一个不常直接使用的控件,一般都会配合ListView或者Column等控件使用。ListBody的作用是按给定的轴方向,按照顺序排列子节点。,与listView相似
? ? ?child: ListBody(
? ? ? ?//列表
? ? ? ?children: const <Widget>[
? ? ? ? ?Text("是否要删除?"),
? ? ? ? ?Text("一旦删除数据不可恢复"),
? ? ? ],
? ? ),
? ),
? ?//按钮选项
? ?actions: <Widget>[
? ? ?TextButton(onPressed: () {
? ? ? ?//关闭
? ? ? ?Navigator.of(context).pop();
? ? }, child: const Text("取消")),
? ? ?TextButton(onPressed: () {
? ? ? ?//关闭并返回true
? ? ? ?Navigator.of(context).pop(true);
? ? }, child: const Text("确定"))
? ],
?);
和SimpleDialog基本一致,Navigator.of(context).pop();用来关闭弹窗,那么如何弹出弹窗呢?,只需要将Dialog嵌套在showDialog()中即可,showDialog()两个必要参数:
属性名 | 类型 | 说明 |
---|
context | BuildContext | 上下文 | builder | WidgetBuilder | 对话框UI的创建方法 | barrierDismissible | bool | 点击对话框barrier(遮罩)时是否关闭它 |
builder方法返回一个Future用于接收对话框选项的返回值,如果我们通过点击对话框外部退出,那么返回值为null,如果通过Navigator.of(context).pop(true)退出那么返回值为pop中传入的值。
完整代码:
?
void main() => runApp(MyApp());
??
?//RunApp不能直接接受StatefulWidget
?class MyApp extends StatelessWidget
?{
? ?@override
? ?Widget build(BuildContext context) {
? ? ?return MaterialApp(
? ? ? ?title: "对话框",
? ? ? ?home: Scaffold(
? ? ? ? ?appBar: AppBar(
? ? ? ? ? ?title: const Text("对话框"),
? ? ? ? ),
? ? ? ? ?body: MyHome(),
? ? ? ),
? ? );
? }
?}
??
?class MyHome extends StatefulWidget
?{
? ?@override
? ?Dialog createState() => Dialog();
?}
??
?class Dialog extends State<MyHome>{
? ?@override
? ?Widget build(BuildContext context) {
? ? ?return Center(
? ? ? ? ? ?//按钮的监听和子选是必须填的
? ? ? ? ? ?child: ElevatedButton(
? ? ? ? ? ? ?//async异步函数
? ? ? ? ? ? ?onPressed: () async {
? ? ? ? ? ? ? ?bool? delete = await showDeleteConfirmDialog(context);
? ? ? ? ? ? ? ?if(delete == null)
? ? ? ? ? ? ? {
? ? ? ? ? ? ? ? ? ?print("取消删除");
? ? ? ? ? ? ? }else
? ? ? ? ? ? ? {
? ? ? ? ? ? ? ? ? ?print("确定删除");
? ? ? ? ? ? ? }
? ? ? ? ? ? },
? ? ? ? ? ? ?child: const Text("点击弹出对话框"),
? ? ? ? ? )
? ? ? ? );
? }
?}
?Future<bool?> showDeleteConfirmDialog(BuildContext context) {
? ?return showDialog<bool>(
? ? ?context: context,
? ? ?builder: (context) {
? ? ? ?return AlertDialog(
? ? ? ? ?//标题
? ? ? ? ?title: const Text("提示"),
? ? ? ? ?//一个可滚动组件
? ? ? ? ?content: SingleChildScrollView(
? ? ? ? ? ?//ListBody是一个不常直接使用的控件,一般都会配合ListView或者Column等控件使用。ListBody的作用是按给定的轴方向,按照顺序排列子节点。,与listView相似
? ? ? ? ? ?child: ListBody(
? ? ? ? ? ? ?//列表
? ? ? ? ? ? ?children: const <Widget>[
? ? ? ? ? ? ? ?Text("是否要删除?"),
? ? ? ? ? ? ? ?Text("一旦删除数据不可恢复"),
? ? ? ? ? ? ],
? ? ? ? ? ),
? ? ? ? ),
? ? ? ? ?//按钮选项
? ? ? ? ?actions: <Widget>[
? ? ? ? ? ?TextButton(onPressed: () {
? ? ? ? ? ? ?//关闭
? ? ? ? ? ? ?Navigator.of(context).pop();
? ? ? ? ? }, child: const Text("取消")),
? ? ? ? ? ?TextButton(onPressed: () {
? ? ? ? ? ? ?//关闭并返回true
? ? ? ? ? ? ?Navigator.of(context).pop(true);
? ? ? ? ? }, child: const Text("确定"))
? ? ? ? ],
? ? ? );
? ? },
? );
?}
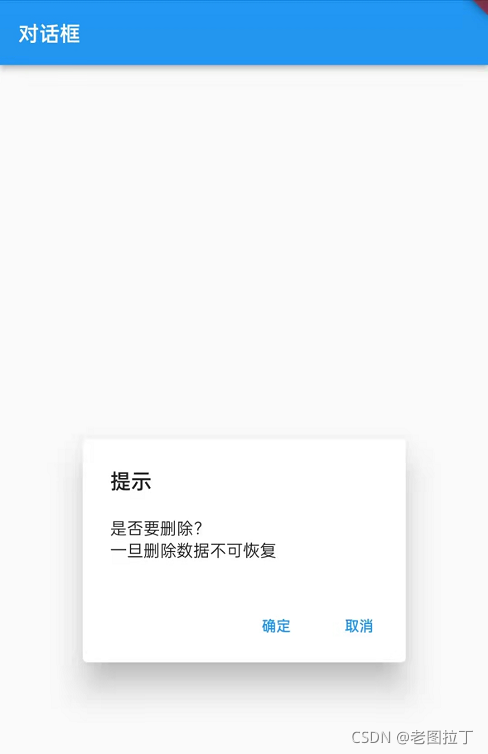
?
|