相关文章RecycleView实现首页复杂布局效果(横向和竖向) 一.添加依赖 maven { url ‘https://jitpack.io’ } 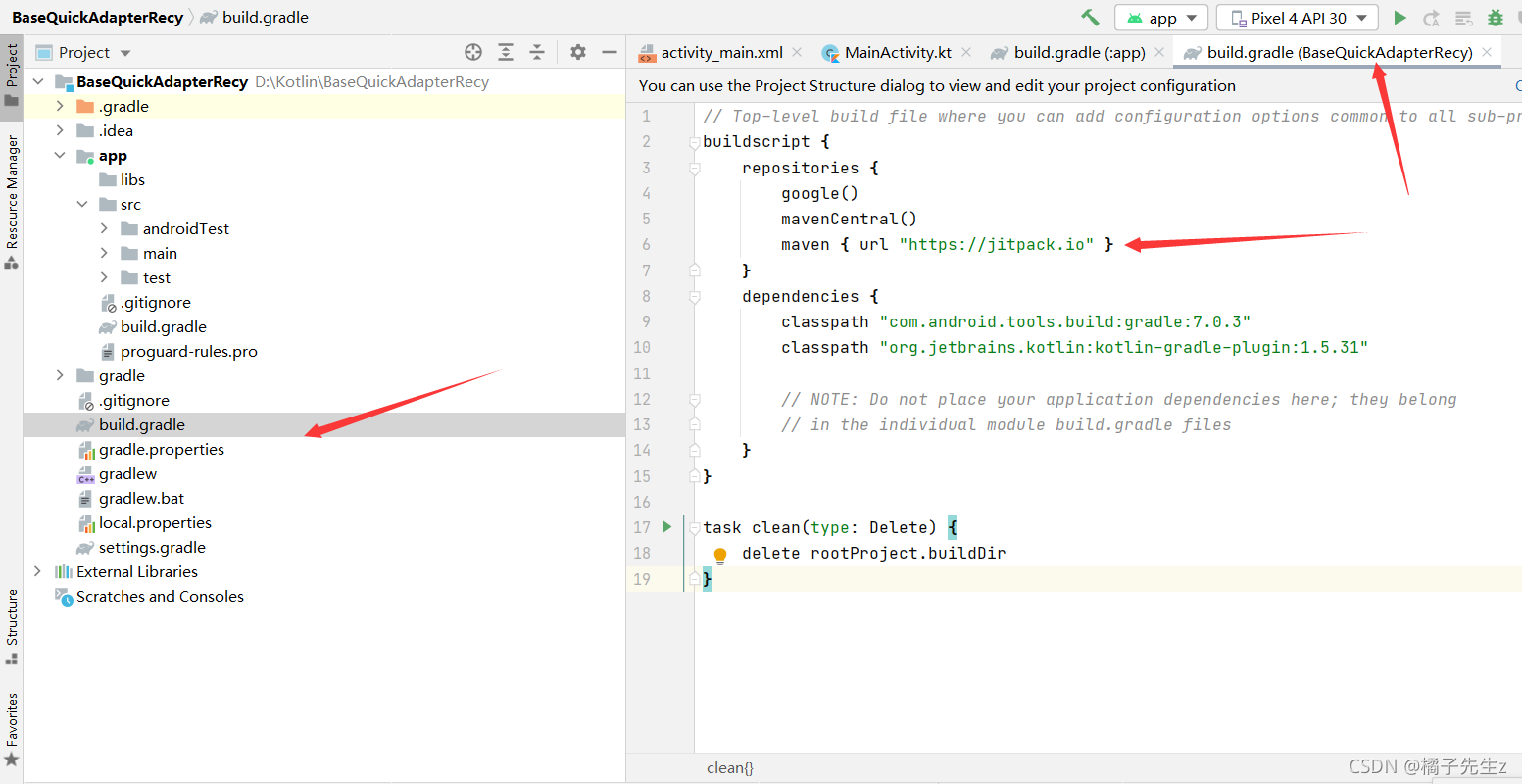 implementation ‘com.github.CymChad:BaseRecyclerViewAdapterHelper:3.0.1’ 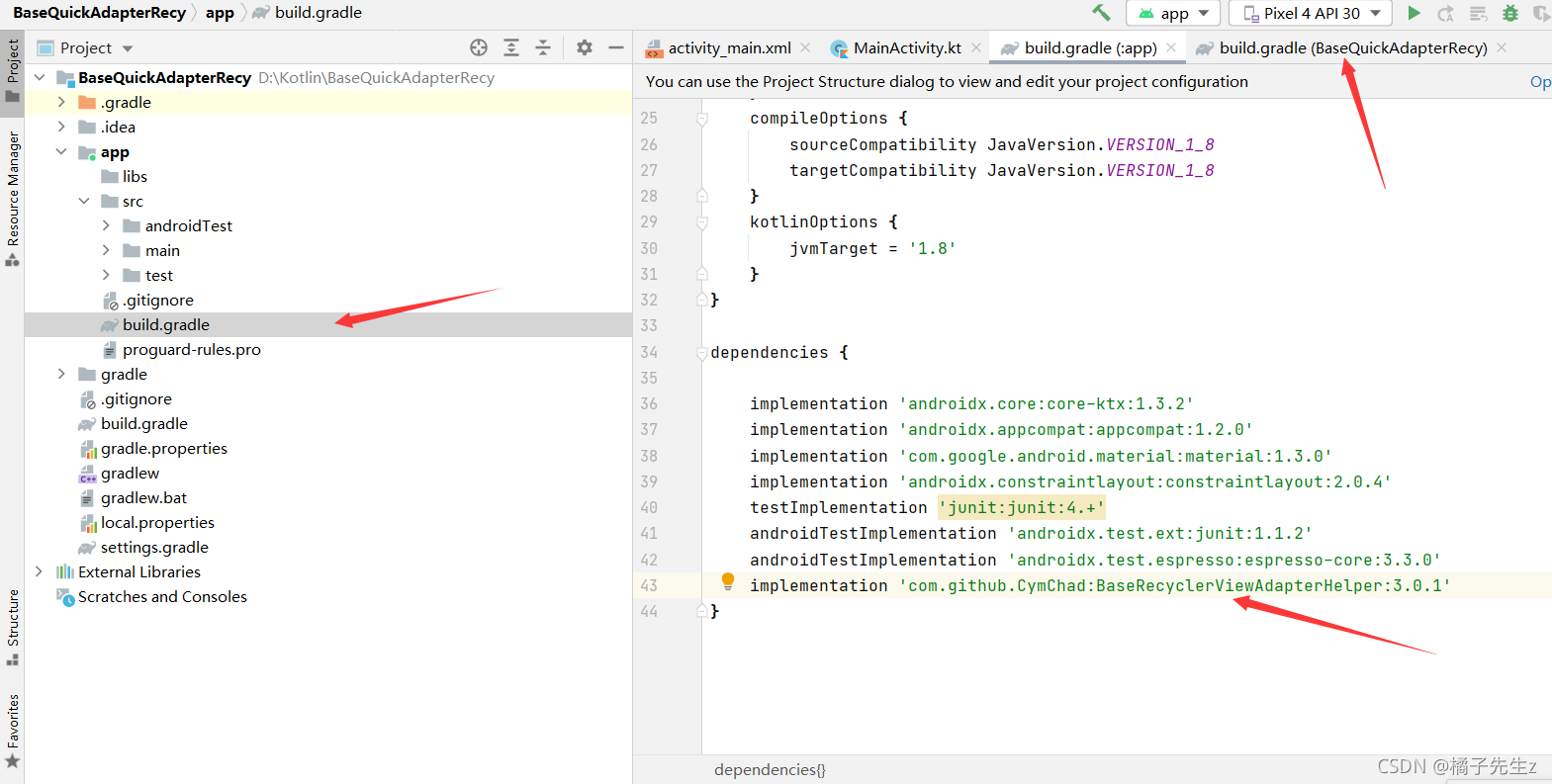 二.适配器
class BaseAdapter(
layoutId: Int,
data: MutableList<InfoBean>? = null
) : BaseQuickAdapter<Bean, BaseViewHolder>(layoutId, data) {
override fun convert(holder: BaseViewHolder, item: InfoBean?) {
val img = holder.getView<ImageView>(R.id.image)
val text = holder.getView<TextView>(R.id.text)
text.setText(item?.data)
item?.let {
holder.setText(R.id.name, item?.data)
.addOnClickListener(R.id.image)
}
}
}
三.数据类
class InfoBean {
var url:String?=null
var image:Int?= 0
var data:String?=null
}
如果想实现多布局就让数据类实现MultiItemEntity接口同时需要实现getItemType方法,这个方法是用来返回item类型的。
四.主程序
val recyclerView=findViewById<RecyclerView>(R.layout.Base_recyclerView)
var dataList = mutableListOf<InfoBean>()
recyclerView.layoutManager = GridLayoutManager(this,5)
val adapter=BaseAdapter(R.layout.item,dataList)
recyclerView.adapter = adapter
adapter.setOnItemChildClickListener {
adapter, view, position ->
}
}
五.布局
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/Base_recyclerView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
tools:ignore="MissingConstraints"
app:layoutManager="androidx.recyclerview.widget.GridLayoutManager"
app:spanCount="5"
tools:itemCount="6"
/>
</androidx.constraintlayout.widget.ConstraintLayout>
1.可以在XML中设置layoutManager app:layoutManager=“androidx.recyclerview.widget.GridLayoutManager” 2.app:spanCount=“5”:设置列数为5 3.tools:itemCount=“6”:设置总的item个数 六. MultiItemEntity实现多布局
class Bean(var type: Int, var url: String) : MultiItemEntity {
override fun getItemType(): Int {
return type
}
companion object {
const val ITEM1 = 1
const val ITEM2 = 2
}
}
class MultiColumnAdapter(data: MutableList<Bean?>?) :
BaseMultiItemQuickAdapter<MultiItemImgBean?, BaseViewHolder?>(data) {
init {
addItemType(MultiItemImgBean.ITEM1, R.layout.item1)
addItemType(MultiItemImgBean.ITEM2, R.layout.item2)
}
override fun convert(helper: BaseViewHolder?, item: Bean?) {
helper ?: return
item ?: return
when (helper.itemViewType) {
Bean.ITEM1 ->{}
Bean.ITEM2 -> {}
else -> {}
}
}
}
|