一、消息提示框和对话框
1.使用Toast显示消息提示框
消息提示框之前都有接触,最主要的应用就是
Toast.makeText().show
接下来详细学习
Toast类的对象有以下常用方法
setDuration(int duration)设置消息的持续时间Toast.LENGTH_LONG/SHORT
setGravity(int gravity,int xOffset,int yOffset)设置位置,参数分别是对齐方式和偏移量
setMargin(float horizontalMargin,float verticalMargin)设置消息提示框页边距
setText(CharSequence s)设置显示文本内容
setView(View view)显示的视图位置
java代码如下
package com.thundersoft.session2;
import android.annotation.SuppressLint;
import android.app.Activity;
import android.os.Bundle;
import android.view.Gravity;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import androidx.annotation.Nullable;
public class ToastActivity extends Activity {
@SuppressLint("ResourceAsColor")
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
LinearLayout linearLayout = new LinearLayout(ToastActivity.this);
Toast.makeText(this, "Toast的第一种创建方式", Toast.LENGTH_SHORT).show();
setContentView(linearLayout);
LinearLayout linearLayout1 = new LinearLayout(ToastActivity.this);
ImageView imageView = new ImageView(ToastActivity.this);
imageView.setImageResource(R.drawable.gif1);
imageView.setPadding(0,0,5,0);
imageView.setLayoutParams(new LinearLayout.LayoutParams(300,300));
TextView textView = new TextView(ToastActivity.this);
textView.setText("Toaset的第二种创建方式");
Toast toast = new Toast(this);
toast.setDuration(Toast.LENGTH_LONG);
toast.setGravity(Gravity.CENTER,0,0);
linearLayout1.addView(imageView);
linearLayout1.addView(textView);
linearLayout1.setBackgroundColor(R.color.purple_200);
toast.setView(linearLayout1);
toast.show();
}
}
代码中的两个布局,第一个布局是总布局,也就是第一个页面,第二个布局是应用在Toaset中的布局
2.使用Notification在状态栏上显示通知
Android8以后,API26
安卓的通知管理类是Notification和NotiffcationManager类
Notification主要是代表全局效果的类,而Manager主要管理发送Notification,是系统服务
下面是一般通知实现的方法
package com.thundersoft.session2;
import android.app.Activity;
import android.app.Notification;
import android.app.NotificationChannel;
import android.app.NotificationManager;
import android.content.Context;
import android.graphics.BitmapFactory;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
import androidx.annotation.Nullable;
public class NotificationActivity extends Activity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
LinearLayout linearLayout = new LinearLayout(this);
setContentView(linearLayout);
Button button = new Button(this);
linearLayout.addView(button);
final NotificationManager manager = (NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
String id = "channel_1";
String description = "channel_description";
int NOTIFY_ID_1=0x1;
int NOTIFY_ID_2=0x2;
int importance = NotificationManager.IMPORTANCE_HIGH;
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
NotificationChannel channel = new NotificationChannel(id, "123", importance);
channel.setDescription(description);
channel.enableLights(true);
channel.enableVibration(true);
channel.setVibrationPattern(new long[]{100,200,300,400,500,400,300,200,400});
manager.createNotificationChannel(channel);
Notification builder = new Notification.Builder(NotificationActivity.this, id)
.setContentTitle("通知标题")
.setSmallIcon(R.drawable.gif1)
.setLargeIcon(BitmapFactory.decodeResource(getResources(),R.drawable.gif1))
.setContentText("通知栏内容")
.setAutoCancel(true)
.build();
manager.notify(NOTIFY_ID_1,builder);
}
});
}
}
通知首先需要定义几个值,一是通知通道的id,二是它的描述属性,三是它的编号
定义好值后,先获取系统服务,new一个通知管理对象
接着new通知通道对象,设置它的各种属性,将通道给通知管理对象管理
通道设置好了就开始new通知对象了,调用类的Builder方法构建,设置属性
最后把通知也交给管理对象管理即可
一般的通知点击会自动跳转到一个页面,这里写了个intent来实现点击通知跳转的功能
Intent intent = new Intent(Notification2Activity.this, NotificationActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(Notification2Activity.this, 0, intent, 0);
Notification builder2 = new Notification.Builder(Notification2Activity.this, id)
.setContentTitle("通知标题")
.setSmallIcon(R.drawable.gif1)
.setLargeIcon(BitmapFactory.decodeResource(getResources(),R.drawable.gif1))
.setContentText("通知栏内容")
.setAutoCancel(true)
.setContentIntent(pendingIntent)
.build()
;
manager.notify(NOTIFY_ID_2,builder2);
需要new一个intent接着用它pendingintent来传递意图,后面会详细讲解intent
通知的取消可以有以下代码控制
manager.cancel(NOTIFY_ID_1);
manager.cancelAll();
3.使用AlertDialog创建对话框
下面介绍了常用的四种对话框
对话框的运用步骤为:通过new AlertDialog.Builder,再设置它的属性和按钮等内容,最后别忘了create和show
新建四个按钮对应四种对话框,写第一个最基础的对话框,代码如下
package com.thundersoft.session2;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
import androidx.annotation.Nullable;
public class AlertDialogActivity extends Activity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
LinearLayout linearLayout = new LinearLayout(this);
setContentView(linearLayout);
Button button1 = new Button(this);
Button button2 = new Button(this);
Button button3 = new Button(this);
Button button4 = new Button(this);
button1.setText("按钮对话框");
button2.setText("列表对话框");
button3.setText("列表+按钮对话框");
button4.setText("多选+按钮对话框");
linearLayout.addView(button1);
linearLayout.addView(button2);
linearLayout.addView(button3);
linearLayout.addView(button4);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AlertDialog alertDialog = new AlertDialog.Builder(AlertDialogActivity.this).create();
alertDialog.setIcon(R.drawable.gif1);
alertDialog.setTitle("提示一:");
alertDialog.setMessage("取消、中立和确定");
alertDialog.setButton(DialogInterface.BUTTON_NEGATIVE, "取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Log.i("对话框","点击了取消");
}
});
alertDialog.setButton(DialogInterface.BUTTON_POSITIVE, "确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Log.i("对话框","点击了确定");
}
});
alertDialog.setButton(DialogInterface.BUTTON_NEUTRAL, "中立", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Log.i("对话框","点击了中立");
}
});
alertDialog.show();
}
});
二三四种如下
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] strings = {"第一条", "第二条", "第三条", "第四条"};
AlertDialog.Builder alertDialog = new AlertDialog.Builder(AlertDialogActivity.this);
alertDialog.setTitle("提示二:");
alertDialog.setItems(strings, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Log.i("对话框", "onClick: "+strings[which]);
}
});
alertDialog.create().show();
}
});
button3.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String[] strings = {"第一条", "第二条", "第三条", "第四条"};
AlertDialog.Builder alertDialog = new AlertDialog.Builder(AlertDialogActivity.this);
alertDialog.setTitle("提示三:");
alertDialog.setSingleChoiceItems(strings, 0,new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
Log.i("对话框", "onClick: "+strings[which]);
}
});
alertDialog.setPositiveButton("确定",null);
alertDialog.create().show();
}
});
button4.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
boolean[] items = {false,false,true,true};
String[] strings = {"第一条", "第二条", "第三条", "第四条"};
AlertDialog.Builder alertDialog = new AlertDialog.Builder(AlertDialogActivity.this);
alertDialog.setTitle("提示三:");
alertDialog.setMultiChoiceItems(strings, items, new DialogInterface.OnMultiChoiceClickListener() {
@Override
public void onClick(DialogInterface dialog, int which, boolean isChecked) {
items[which] = isChecked;
}
});
alertDialog.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String result = "";
for (int i = 0; i < items.length; i++) {
if (items[i]){
result+=strings[i]+"、";
}
}
if (!"".equals(result)){
result = result.substring(0,result.length()-1);
Log.i("对话框", result);
}
}
});
alertDialog.create().show();
}
});
}
}
第四种中,增加了按钮直接再按钮里new DialogInterface.OnClickListener()来新建点击事件
下面的代码演示了退出确认的对话框提示
package com.thundersoft.session2;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
import androidx.annotation.Nullable;
public class Test1Activity extends Activity {
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
LinearLayout linearLayout = new LinearLayout(this);
setContentView(linearLayout);
Button button = new Button(this);
button.setText("点击退出");
linearLayout.addView(button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
AlertDialog.Builder builder = new AlertDialog.Builder(Test1Activity.this);
builder.setIcon(R.drawable.gif1);
builder.setTitle("是否退出?");
builder.setMessage("是否真的要退出码?");
builder.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
finish();
}
});
builder.create().show();
}
});
}
}
二、实践
1.要求
实现一个登录页面,提供记住密码和用户名功能,登陆后显示列表视图必须包含登录用户名,且点击列表会显示点击那一项
(以上是Android 几 当中的实践要求)
在上一次的实践项目之上,登录后主界面列表选择第一个选项后,显示一组图片,图片加载完成前需要显示进度条,加载完成后,长按某一个图片弹出提示框是否删除,如果确定就不再显示该图片。
2.代码实现
image.xml显示图片的页面
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<GridView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:stretchMode="columnWidth"
android:numColumns="3"
android:id="@+id/gridview"/>
</LinearLayout>
items.xml每一个gridview里面的内容
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:id="@+id/imageview"
android:scaleType="fitXY"
android:paddingLeft="10px"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="5px"
android:layout_gravity="center"
android:id="@+id/titleview"/>
</LinearLayout>
title.xml自定义标题栏
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="图像预览"
android:textStyle="bold"
android:textColor="#FFFF0000"
/>
<ProgressBar
android:id="@+id/bartest"
android:layout_width="match_parent"
android:layout_height="wrap_content"
style="?android:attr/progressBarStyleHorizontal"
android:max="80"/>
</LinearLayout>
在上一次的实践中,需要更改MainActivity.java以下代码
@Override
protected void onListItemClick(ListView l, View v, int position, long id) {
super.onListItemClick(l, v, position, id);
int index = position+1;
if (index == 1){
Intent intent = new Intent(MainActivity.this, ImageActivity.class);
startActivity(intent);
}
Toast.makeText(this,"您选择了第"+ index +"项",Toast.LENGTH_SHORT).show();
}
最主要的部分ImageActivity
package com.thundersoft.login;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.AdapterView;
import android.widget.GridView;
import android.widget.ProgressBar;
import android.widget.SimpleAdapter;
import androidx.annotation.Nullable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Map;
public class ImageActivity extends Activity {
int[] image = new int[]{R.drawable.jpg1,R.drawable.jpg2,R.drawable.jpg3,R.drawable.jpg4,R.drawable.jpg5};
String[] title = {"头像1", "头像2", "头像3", "头像4", "头像5"};
ArrayList<Map<String, Object>> list = new ArrayList<>();
private ProgressBar progressBar;
private GridView gridView;
private SimpleAdapter simpleAdapter;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_CUSTOM_TITLE);
setContentView(R.layout.image);
getWindow().setFeatureInt(Window.FEATURE_CUSTOM_TITLE,R.layout.title);
progressBar = findViewById(R.id.bartest);
gridView = findViewById(R.id.gridview);
new MyTack().execute();
gridView.setOnItemLongClickListener(new AdapterView.OnItemLongClickListener() {
@Override
public boolean onItemLongClick(AdapterView<?> parent, View view, int position, long id) {
AlertDialog.Builder builder = new AlertDialog.Builder(ImageActivity.this);
builder.setTitle("是否删除?");
builder.setMessage("是否真的要删除吗?");
builder.setNegativeButton("取消", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
}
});
builder.setPositiveButton("确定", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
list.remove(position);
simpleAdapter.notifyDataSetChanged();
}
});
builder.create().show();
return true;
}
});
}
class MyTack extends AsyncTask<Void,Integer,SimpleAdapter>{
@Override
protected SimpleAdapter doInBackground(Void... params) {
for (int i = 0; i < image.length; i++) {
HashMap<String, Object> map = new HashMap<>();
map.put("imageview",image[i]);
map.put("titleview",title[i]);
try {
list.add(map);
Thread.sleep(600);
} catch (InterruptedException e) {
e.printStackTrace();
}
publishProgress(i);
}
simpleAdapter = new SimpleAdapter(ImageActivity.this,
list,
R.layout.items,
new String[]{"titleview", "imageview"},
new int[]{R.id.titleview, R.id.imageview});
return simpleAdapter;
}
@Override
protected void onProgressUpdate(Integer... values) {
progressBar.setProgress(values[0]*20);
super.onProgressUpdate(values);
}
@Override
protected void onPostExecute(SimpleAdapter result) {
progressBar.setVisibility(View.GONE);
gridView.setAdapter(result);
super.onPostExecute(result);
}
}
}
详细说下代码的运行过程
通过上一个页面的intent传递,到这个页面,开始oncreate方法
加载自定义标题栏,设置页面,找到控件等基本操作
接下来进行线程操作,写Tack继承AsyncTask,注意result参数应该是SimpleAdapter,实现三个方法
? doInBackground方法返回为SimpleAdapter,在这个里面,new一个SimpleAdapter对象,将图片和文字装载进这个适配器中,返回这个适配器
? onProgressUpdate方法显示更新操作,展示给用户查看进度
? onPostExecute方法参数,result应该是SimpleAdapter对象,通过gridView.setAdapter(result);来将适配器加载给gridview
线程操作完成后,显示gridview,给每一个元素设置长按点击操作,展示对话框询问删除,删除通过对list的操作在重载适配器完成操作
3.实现效果
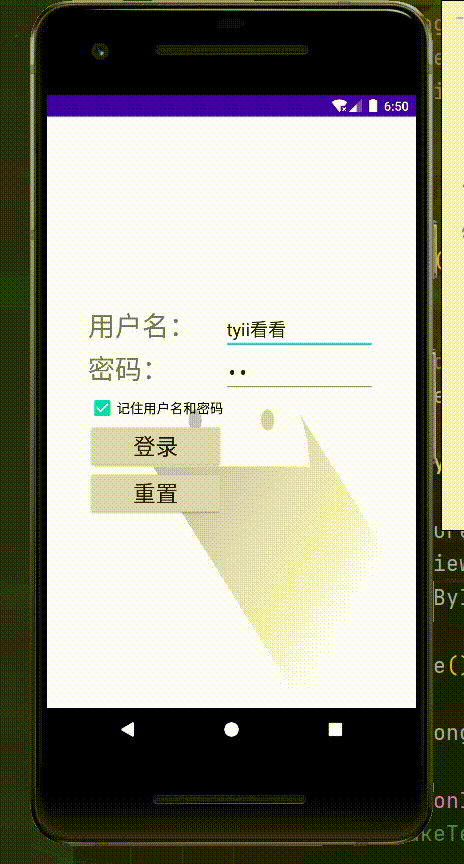
|