1、GreenDao简介:
greenDAO是一个开源的Android ORM,它让SQLite数据库的开发再次变得有趣。它使开发人员免于处理低级数据库要求,同时节省了开发时间。SQLite是一个很棒的嵌入式关系数据库。尽管如此,编写SQL和解析查询结果仍然是非常繁琐且耗时的任务。通过将Java对象映射到数据库表(称为ORM,“对象/关系映射”),greenDAO使您摆脱了这些麻烦。这样,您可以使用简单的面向对象的API来存储,更新,删除和查询Java对象。 ?
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ?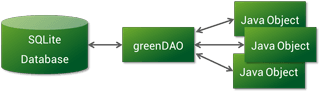
?????????????????????????????????????????????????????????????????ORM图
2、GreenDao优点:
- 最佳性能 (可能是 Android 中最快的 ORM) ,基准测试也是开源的;
- 易于使用的功能强大的 api,涵盖关系和连接;
- 最小的内存消耗;
- 小型库大小(< 100KB) ,以保持较低的构建时间,并避免65k 方法限制;
- 数据库加密:greenDAO 支持 SQLCipher 来保证用户数据的安全;
- 强大而活跃的社区交流支持,相关资料比较完善;
- 许多顶级的Android应用程序都依赖greenDAO,其中一些应用程序的安装量超过1000万,更证明了其可靠性。
GreenDao使用:
首先在项目目录下的build.gradle中配置
repositories {
? ?// GreenDao仓库
? ?mavenCentral()
}
dependencies {
? ?// GreenDao插件
? ?classpath 'org.greenrobot:greendao-gradle-plugin:3.2.2'
}
然后在app目录下的build.gradle中配置
//GreenDao插件
apply plugin: 'org.greenrobot.greendao'
dependencies {
? ? implementation 'org.greenrobot:greendao:3.2.2'
}
android {
? ? ? ? greendao {
?
? ? ? ? schemaVersion 1 //数据库版本号 每次升级数据库都需要改变版本,只能增加
?
? ? ? ? daoPackage 'com...green' ?//设置DaoMaster、 ? ? ? DaoSession、Dao包名
?
? ? ? ? targetGenDir 'src/main/java' //设置DaoMaster、DaoSession、Dao目录
? ? }
}
创建一个表:
@Entity(nameInDb = "logData")
public class LogData implements Serializable {
private static final long serialVersionUID = 8418246610166499523L;
@Id(autoincrement = true)
public Long id;
@Property(nameInDb = "username")
public String username; // 用户名
// 公司设置
@Property(nameInDb = "company_name")
public String companyName; // 企业名称
@Property(nameInDb = "server_address")
public String serverAddress; // 服务器地址
@Property(nameInDb = "message")
public String message; // 日志信息
@Property(nameInDb = "timestamp")
public long timestamp; // 时间
@Property(nameInDb = "barcode")
public String barcode; // 条码
@Property(nameInDb = "order_no")
public String orderNo; // 单号
@Property(nameInDb = "type")
public String type; // 类型
@Property(nameInDb = "status")
public int status; // 状态 1-成功,2-失败
@Property(nameInDb = "date")
public String date; // 时间2021-08-24
@Property(nameInDb = "customer")
public String customer; // 客户
@Property(nameInDb = "product")
public String product; // 产品
@Property(nameInDb = "is_delete")
public int isDelete; // 是否删除 0正常 1删除
@Transient
public boolean isSelected; // 是否选择
public LogData(String username, String companyName,
String serverAddress, String message, long timestamp, String barcode,
String orderNo, String type, int status,
String date, String customer) {
this.username = username;
this.companyName = companyName;
this.serverAddress = serverAddress;
this.message = message;
this.timestamp = timestamp;
this.barcode = barcode;
this.orderNo = orderNo;
this.type = type;
this.status = status;
this.date = date;
this.customer = customer;
}
@Generated(hash = 27898366)
public LogData(Long id, String username, String companyName, String serverAddress,
String message, long timestamp, String barcode, String orderNo, String type,
int status, String date, String customer, String product, int isDelete) {
this.id = id;
this.username = username;
this.companyName = companyName;
this.serverAddress = serverAddress;
this.message = message;
this.timestamp = timestamp;
this.barcode = barcode;
this.orderNo = orderNo;
this.type = type;
this.status = status;
this.date = date;
this.customer = customer;
this.product = product;
this.isDelete = isDelete;
}
@Generated(hash = 1020246481)
public LogData() {
}
public Long getId() {
return this.id;
}
public void setId(Long id) {
this.id = id;
}
public String getUsername() {
return this.username;
}
public void setUsername(String username) {
this.username = username;
}
public String getCompanyName() {
return this.companyName;
}
public void setCompanyName(String companyName) {
this.companyName = companyName;
}
public String getServerAddress() {
return this.serverAddress;
}
public void setServerAddress(String serverAddress) {
this.serverAddress = serverAddress;
}
public String getMessage() {
return this.message;
}
public void setMessage(String message) {
this.message = message;
}
public long getTimestamp() {
return this.timestamp;
}
public void setTimestamp(long timestamp) {
this.timestamp = timestamp;
}
public String getType() {
return this.type;
}
public void setType(String type) {
this.type = type;
}
public int getStatus() {
return this.status;
}
public void setStatus(int status) {
this.status = status;
}
public String getOrderNo() {
return this.orderNo;
}
public void setOrderNo(String orderNo) {
this.orderNo = orderNo;
}
public String getBarcode() {
return this.barcode;
}
public void setBarcode(String barcode) {
this.barcode = barcode;
}
public String getDate() {
return date;
}
public void setDate(String date) {
this.date = date;
}
public String getCustomer() {
return customer;
}
public void setCustomer(String customer) {
this.customer = customer;
}
public String getProduct() {
return this.product;
}
public void setProduct(String product) {
this.product = product;
}
public int getIsDelete() {
return this.isDelete;
}
public void setIsDelete(int isDelete) {
this.isDelete = isDelete;
}
}
自动生成的类(Dao,DaoMaster,DaoSession):后两个就不贴代码了
// THIS CODE IS GENERATED BY greenDAO, DO NOT EDIT.
/**
* DAO for table "logData".
*/
public class LogDataDao extends AbstractDao<LogData, Long> {
public static final String TABLENAME = "logData";
/**
* Properties of entity LogData.<br/>
* Can be used for QueryBuilder and for referencing column names.
*/
public static class Properties {
public final static Property Id = new Property(0, Long.class, "id", true, "_id");
public final static Property Username = new Property(1, String.class, "username", false, "username");
public final static Property CompanyName = new Property(2, String.class, "companyName", false, "company_name");
public final static Property ServerAddress = new Property(3, String.class, "serverAddress", false, "server_address");
public final static Property Message = new Property(4, String.class, "message", false, "message");
public final static Property Timestamp = new Property(5, long.class, "timestamp", false, "timestamp");
public final static Property Barcode = new Property(6, String.class, "barcode", false, "barcode");
public final static Property OrderNo = new Property(7, String.class, "orderNo", false, "order_no");
public final static Property Type = new Property(8, String.class, "type", false, "type");
public final static Property Status = new Property(9, int.class, "status", false, "status");
public final static Property Date = new Property(10, String.class, "date", false, "date");
public final static Property Customer = new Property(11, String.class, "customer", false, "customer");
public final static Property Product = new Property(12, String.class, "product", false, "product");
public final static Property IsDelete = new Property(13, int.class, "isDelete", false, "is_delete");
}
public LogDataDao(DaoConfig config) {
super(config);
}
public LogDataDao(DaoConfig config, DaoSession daoSession) {
super(config, daoSession);
}
/** Creates the underlying database table. */
public static void createTable(Database db, boolean ifNotExists) {
String constraint = ifNotExists? "IF NOT EXISTS ": "";
db.execSQL("CREATE TABLE " + constraint + "\"logData\" (" + //
"\"_id\" INTEGER PRIMARY KEY AUTOINCREMENT ," + // 0: id
"\"username\" TEXT," + // 1: username
"\"company_name\" TEXT," + // 2: companyName
"\"server_address\" TEXT," + // 3: serverAddress
"\"message\" TEXT," + // 4: message
"\"timestamp\" INTEGER NOT NULL ," + // 5: timestamp
"\"barcode\" TEXT," + // 6: barcode
"\"order_no\" TEXT," + // 7: orderNo
"\"type\" TEXT," + // 8: type
"\"status\" INTEGER NOT NULL ," + // 9: status
"\"date\" TEXT," + // 10: date
"\"customer\" TEXT," + // 11: customer
"\"product\" TEXT," + // 12: product
"\"is_delete\" INTEGER NOT NULL );"); // 13: isDelete
}
/** Drops the underlying database table. */
public static void dropTable(Database db, boolean ifExists) {
String sql = "DROP TABLE " + (ifExists ? "IF EXISTS " : "") + "\"logData\"";
db.execSQL(sql);
}
@Override
protected final void bindValues(DatabaseStatement stmt, LogData entity) {
stmt.clearBindings();
Long id = entity.getId();
if (id != null) {
stmt.bindLong(1, id);
}
String username = entity.getUsername();
if (username != null) {
stmt.bindString(2, username);
}
String companyName = entity.getCompanyName();
if (companyName != null) {
stmt.bindString(3, companyName);
}
String serverAddress = entity.getServerAddress();
if (serverAddress != null) {
stmt.bindString(4, serverAddress);
}
String message = entity.getMessage();
if (message != null) {
stmt.bindString(5, message);
}
stmt.bindLong(6, entity.getTimestamp());
String barcode = entity.getBarcode();
if (barcode != null) {
stmt.bindString(7, barcode);
}
String orderNo = entity.getOrderNo();
if (orderNo != null) {
stmt.bindString(8, orderNo);
}
String type = entity.getType();
if (type != null) {
stmt.bindString(9, type);
}
stmt.bindLong(10, entity.getStatus());
String date = entity.getDate();
if (date != null) {
stmt.bindString(11, date);
}
String customer = entity.getCustomer();
if (customer != null) {
stmt.bindString(12, customer);
}
String product = entity.getProduct();
if (product != null) {
stmt.bindString(13, product);
}
stmt.bindLong(14, entity.getIsDelete());
}
@Override
protected final void bindValues(SQLiteStatement stmt, LogData entity) {
stmt.clearBindings();
Long id = entity.getId();
if (id != null) {
stmt.bindLong(1, id);
}
String username = entity.getUsername();
if (username != null) {
stmt.bindString(2, username);
}
String companyName = entity.getCompanyName();
if (companyName != null) {
stmt.bindString(3, companyName);
}
String serverAddress = entity.getServerAddress();
if (serverAddress != null) {
stmt.bindString(4, serverAddress);
}
String message = entity.getMessage();
if (message != null) {
stmt.bindString(5, message);
}
stmt.bindLong(6, entity.getTimestamp());
String barcode = entity.getBarcode();
if (barcode != null) {
stmt.bindString(7, barcode);
}
String orderNo = entity.getOrderNo();
if (orderNo != null) {
stmt.bindString(8, orderNo);
}
String type = entity.getType();
if (type != null) {
stmt.bindString(9, type);
}
stmt.bindLong(10, entity.getStatus());
String date = entity.getDate();
if (date != null) {
stmt.bindString(11, date);
}
String customer = entity.getCustomer();
if (customer != null) {
stmt.bindString(12, customer);
}
String product = entity.getProduct();
if (product != null) {
stmt.bindString(13, product);
}
stmt.bindLong(14, entity.getIsDelete());
}
@Override
public Long readKey(Cursor cursor, int offset) {
return cursor.isNull(offset + 0) ? null : cursor.getLong(offset + 0);
}
@Override
public LogData readEntity(Cursor cursor, int offset) {
LogData entity = new LogData( //
cursor.isNull(offset + 0) ? null : cursor.getLong(offset + 0), // id
cursor.isNull(offset + 1) ? null : cursor.getString(offset + 1), // username
cursor.isNull(offset + 2) ? null : cursor.getString(offset + 2), // companyName
cursor.isNull(offset + 3) ? null : cursor.getString(offset + 3), // serverAddress
cursor.isNull(offset + 4) ? null : cursor.getString(offset + 4), // message
cursor.getLong(offset + 5), // timestamp
cursor.isNull(offset + 6) ? null : cursor.getString(offset + 6), // barcode
cursor.isNull(offset + 7) ? null : cursor.getString(offset + 7), // orderNo
cursor.isNull(offset + 8) ? null : cursor.getString(offset + 8), // type
cursor.getInt(offset + 9), // status
cursor.isNull(offset + 10) ? null : cursor.getString(offset + 10), // date
cursor.isNull(offset + 11) ? null : cursor.getString(offset + 11), // customer
cursor.isNull(offset + 12) ? null : cursor.getString(offset + 12), // product
cursor.getInt(offset + 13) // isDelete
);
return entity;
}
@Override
public void readEntity(Cursor cursor, LogData entity, int offset) {
entity.setId(cursor.isNull(offset + 0) ? null : cursor.getLong(offset + 0));
entity.setUsername(cursor.isNull(offset + 1) ? null : cursor.getString(offset + 1));
entity.setCompanyName(cursor.isNull(offset + 2) ? null : cursor.getString(offset + 2));
entity.setServerAddress(cursor.isNull(offset + 3) ? null : cursor.getString(offset + 3));
entity.setMessage(cursor.isNull(offset + 4) ? null : cursor.getString(offset + 4));
entity.setTimestamp(cursor.getLong(offset + 5));
entity.setBarcode(cursor.isNull(offset + 6) ? null : cursor.getString(offset + 6));
entity.setOrderNo(cursor.isNull(offset + 7) ? null : cursor.getString(offset + 7));
entity.setType(cursor.isNull(offset + 8) ? null : cursor.getString(offset + 8));
entity.setStatus(cursor.getInt(offset + 9));
entity.setDate(cursor.isNull(offset + 10) ? null : cursor.getString(offset + 10));
entity.setCustomer(cursor.isNull(offset + 11) ? null : cursor.getString(offset + 11));
entity.setProduct(cursor.isNull(offset + 12) ? null : cursor.getString(offset + 12));
entity.setIsDelete(cursor.getInt(offset + 13));
}
@Override
protected final Long updateKeyAfterInsert(LogData entity, long rowId) {
entity.setId(rowId);
return rowId;
}
@Override
public Long getKey(LogData entity) {
if(entity != null) {
return entity.getId();
} else {
return null;
}
}
@Override
public boolean hasKey(LogData entity) {
return entity.getId() != null;
}
@Override
protected final boolean isEntityUpdateable() {
return true;
}
}
GreenDaoManager管理类:
public class GreenDaoManager {
private static DaoMaster daoMasterEcmc;
private static DaoMaster daoMasterOut;
// 默认DB
private static DaoSession daoSessionDefault;
// 拷贝的db
private static DaoSession daoOutSession;
/**
* 默认数据库名称:localdata
*/
public static final String DATABASE_NAME = "hy_gather_app.db";
private static DaoMaster mDaoMaster;
private static volatile GreenDaoManager mInstance = null;
public static final String DB_NAME = "hy_gather_app.db";
private static MySQLiteOpenHelper helper;
private GreenDaoManager() {
}
public static void init(Context context) {
QueryBuilder.LOG_SQL = true;
QueryBuilder.LOG_VALUES = true;
// DaoMaster.DevOpenHelper devOpenHelper = new
// DaoMaster.DevOpenHelper(context, DB_NAME);
// mDaoMaster = new DaoMaster(devOpenHelper.getWritableDatabase());
//如果你想查看日志信息,请将DEBUG设置为true
MigrationHelper.DEBUG = true;
MySQLiteOpenHelper helper = new MySQLiteOpenHelper(context, GreenDaoManager.DB_NAME,
null);
mDaoMaster = new DaoMaster(helper.getWritableDatabase());
daoSessionDefault = mDaoMaster.newSession();
}
public static void init(Context context, String dbName) {
QueryBuilder.LOG_SQL = true;
QueryBuilder.LOG_VALUES = true;
// DaoMaster.DevOpenHelper devOpenHelper = new
// DaoMaster.DevOpenHelper(context, DB_NAME);
//如果你想查看日志信息,请将DEBUG设置为true
MigrationHelper.DEBUG = true;
helper = new MySQLiteOpenHelper(context, dbName,
null);
mDaoMaster = new DaoMaster(helper.getWritableDatabase());
}
public static GreenDaoManager getInstance() {
if (mInstance == null) {
synchronized (GreenDaoManager.class) {
if (mInstance == null) {
mInstance = new GreenDaoManager();
}
}
}
return mInstance;
}
public AppConfigDao getAppConfigDao() {
return getDaoSession().getAppConfigDao();
}
public PDADataDao getPDADataDao() {
return getDaoSession().getPDADataDao();
}
public PDADataDao getPDADataDao(String dbName) {
// clearOutsideDao();
return getDaoSession(dbName).getPDADataDao();
}
public LogDataDao getLogDataDao(String dbName) {
// clearOutsideDao();
return getDaoSession(dbName).getLogDataDao();
}
public ScanDataDao getScanDataDao(String dbName) {
// clearOutsideDao();
return getDaoSession(dbName).getScanDataDao();
}
public CheckedCompanyDao getCheckedCompanyDao() {
return getDaoSession().getCheckedCompanyDao();
}
public BarcodeDataDao getBarcodeDataDao(String dbName) {
// clearOutsideDao();
return getDaoSession(dbName).getBarcodeDataDao();
}
/**
* 查询某个公司配置
*
* @param serverAddress
* @param account
* @return
*/
public AppConfig getAppConfig(AppConfigDao appConfigDao, String serverAddress, String account) {
return appConfigDao.queryBuilder()
.where(AppConfigDao.Properties.ServerAddress.eq(serverAddress) /*,
AppConfigDao.Properties.Username.eq(account)*/)
.build().unique();
}
/**
* 获取总数据条数
*
* @return
*/
public static int getLogCount(LogDataDao logDataDao, String username, String serverAddress) {
long time = System.currentTimeMillis();
// Database db = logDataDao.getDatabase();
//
long count = logDataDao.queryBuilder().where(LogDataDao.Properties.ServerAddress.eq(serverAddress),
LogDataDao.Properties.Username.eq(username))
.count();
// String sql = "select * from " + LogDataDao.TABLENAME + " where username=? and serverAddress=?";
// Cursor rec = db.rawQuery(sql, new String[]{username, serverAddress});
// rec.moveToLast();
count = rec.getInt(0);//取得总数
// count = rec.getCount(); //取得总数
//
// rec.close();
// db.close();
LogUtils.d("查询完成:" + (System.currentTimeMillis() - time) + " ms");
return (int) count;
}
public long getPDACount(AppConfig appConfig, String dbName, String scanType) {
long time = System.currentTimeMillis();
long count = 0;
// clearOutsideDao();
PDADataDao pdaDataDao = getDaoSession(dbName).getPDADataDao();
count = pdaDataDao.queryBuilder()
.where(PDADataDao.Properties.ServerAddress.eq(appConfig.serverAddress),
PDADataDao.Properties.Type.eq(scanType),
PDADataDao.Properties.Username.eq(appConfig.username))
.count();
LogUtils.d("查询完成 " + scanType + " PDACount: " + count + " it took " + (System.currentTimeMillis() - time) + " ms");
return count;
}
public static boolean insertPDABySql(AppConfig appConfig, String dbName, List<PDAData> list) {
long time = System.currentTimeMillis();
if (list == null || list.size() == 0) return false;
String sql = "INSERT INTO " + PDADataDao.TABLENAME + " (timestamp,username,serverAddress,Key,Value,Type) VALUES (?,?,?,?,?,?)";
// clearOutsideDao();
PDADataDao pdaDataDao = getDaoSession(dbName).getPDADataDao();
Database db = null;
try {
db = pdaDataDao.getDatabase();
DatabaseStatement stat = db.compileStatement(sql);
db.beginTransaction();
for (PDAData pdaData : list) {
stat.bindString(1, pdaData.timestamp);
stat.bindString(2, pdaData.username);
stat.bindString(3, pdaData.serverAddress);
stat.bindString(4, pdaData.Key);
stat.bindString(5, pdaData.Value);
stat.bindString(6, pdaData.Type);
long result = stat.executeInsert();
if (result < 0) {
return false;
}
}
db.setTransactionSuccessful();
} catch (Exception e) {
e.printStackTrace();
return false;
} finally {
if (db != null) {
db.close();
}
}
LogUtils.d("插完成:" + (System.currentTimeMillis() - time) + " ms");
return true;
}
public static Boolean fastInsertMany(PDADataDao pdaDataDao, List<PDAData> list) {
Database db = pdaDataDao.getDatabase();
Boolean flag = false;
String sql = "INSERT INTO " + PDADataDao.TABLENAME + " (timestamp,username,serverAddress,Key,Value,Type) VALUES (?,?,?,?,?,?)";
if (list != null && list.size() > 0) {
List<Object[]> bindArgs = new ArrayList<Object[]>();
for (int i = 0; i < list.size(); i++) {
bindArgs.add(new Object[]{list.get(i).getTimestamp(), list.get(i).getUsername(),
list.get(i).getServerAddress(), list.get(i).getKey(), list.get(i).getValue(), list.get(i).getType()});
}
flag = fastInsertMany(sql, bindArgs);
} else {
flag = false;
}
db.close();
return flag;
}
public static boolean fastInsertMany(String sql, List<Object[]> bindArgs) {
boolean flag = false;
SQLiteDatabase db = helper.getWritableDatabase();
if (db == null) {
helper.getWritableDatabase();
}
db.beginTransaction(); //手动设置开始事务
//数据插入操作循环
try {
for (int i = 0; i < bindArgs.size(); i++) {
db.execSQL(sql, bindArgs.get(i));
//LogUtil.i(TAG, "sql:" + sql+" args:"+bindArgs.toString());
}
db.setTransactionSuccessful(); //设置事务处理成功,不设置会自动回滚不提交
flag = true;
} catch (Exception ex) {
flag = false;
} finally {
db.endTransaction(); //处理完成
}
return flag;
}
public static boolean deletePDAData2(PDADataDao pdaDataDao, String type, String serverAddress) {
LogUtils.d("deletePDAData");
long time = System.currentTimeMillis();
// 删除旧数据
pdaDataDao.queryBuilder().
where(PDADataDao.Properties.ServerAddress.eq(serverAddress),
PDADataDao.Properties.Type.eq(type))
.buildDelete().executeDeleteWithoutDetachingEntities();
LogUtils.d("数据删除完成:" + (System.currentTimeMillis() - time) + " ms");
return true;
}
@Deprecated
public static boolean deletePDAData(PDADataDao pdaDataDao, String Type, String serverAddress) {
LogUtils.d("查询完成 deletePDAData start");
long time = System.currentTimeMillis();
List<PDAData> list = pdaDataDao.queryBuilder()
.where(PDADataDao.Properties.ServerAddress.eq(serverAddress)
, PDADataDao.Properties.Type.eq(Type))
.build().list();
long endTime = System.currentTimeMillis();
LogUtils.d("查询完成 deletePDAData :" + (endTime - time) + " ms");
if (list != null && list.size() > 0) {
pdaDataDao.deleteInTx(list);
}
LogUtils.d("删除完成:" + (System.currentTimeMillis() - time) + " ms");
return true;
}
public List<PDAData> getPDADataList(PDADataDao pdaDataDao, String serverAddress, String account, String type, int offset) {
if (pdaDataDao == null) {
pdaDataDao = getPDADataDao(serverAddress);
}
return pdaDataDao.queryBuilder()
.where(PDADataDao.Properties.ServerAddress.eq(serverAddress),
PDADataDao.Properties.Username.eq(account), PDADataDao.Properties.Type.eq(type))
.offset(offset * 100)
.limit(100)
.build().list();
}
/**
* 拷贝数据库名称:school
*/
private static DaoMaster obtainMaster(Context context, String dbName) {
return new DaoMaster(new DaoMaster.DevOpenHelper(context, dbName, null).getWritableDatabase());
}
private static DaoMaster getDaoMaster(Context context, String dbName) {
if (dbName == null)
return null;
if (daoMasterEcmc == null) {
daoMasterEcmc = obtainMaster(context, dbName);
}
return daoMasterEcmc;
}
private static DaoMaster getOutSideDaoMaster(Context context, String dbName) {
if (dbName == null)
return null;
if (daoMasterOut == null) {
daoMasterOut = obtainMaster(context, dbName);
}
return daoMasterOut;
}
/**
* 取得DaoSession
*
* @return
*/
public static DaoSession getDaoSession(String dbName) {
// clearOutsideDao();
if (daoOutSession == null) {
daoOutSession = getOutSideDaoMaster(App.getInstance(), dbName).newSession();
}
return daoOutSession;
}
/**
* 默认操作localdata数据库
*/
public static DaoSession getDaoSession() {
// clearOutsideDao();
if (daoSessionDefault == null) {
daoSessionDefault = getDaoMaster(App.getInstance(), DATABASE_NAME).newSession();
}
return daoSessionDefault;
}
/**
* 链接不同的外部数据库时需要每次都清掉之前的操作
*/
public static void clearOutsideDao() {
if (daoOutSession != null) {
daoOutSession.clear();
daoOutSession = null;
}
if (daoMasterOut != null) {
daoMasterOut = null;
}
}
/**
* 将一个list均分成n个list,主要通过偏移量来实现的
*
* @param source
* @param n
* @param <T>
* @return
*/
public static <T> List<List<T>> averageAssign(List<T> source, int n) {
List<List<T>> result = new ArrayList<List<T>>();
int remaider = source.size() % n; //(先计算出余数)
int number = source.size() / n; //然后是商
int offset = 0;//偏移量
for (int i = 0; i < n; i++) {
List<T> value = null;
if (remaider > 0) {
value = source.subList(i * number + offset, (i + 1) * number + offset + 1);
remaider--;
offset++;
} else {
value = source.subList(i * number + offset, (i + 1) * number + offset);
}
result.add(value);
AppConfigDao appConfigDao = getDaoSession("").getAppConfigDao();
}
return result;
}
public ScanDataDetailDao getScanDataDetailDao(String dbName) {
// clearOutsideDao();
return getDaoSession(dbName).getScanDataDetailDao();
}
/**
* 关闭所有的操作
* 注:数据库开启之后,使用完毕必须要关闭
*/
public void closeConnection() {
closeHelper();
closeDaoSession();
}
private void closeHelper() {
if (helper != null) {
helper.close();
helper = null;
}
}
private void closeDaoSession() {
if (daoSessionDefault != null) {
daoSessionDefault.clear();
daoSessionDefault = null;
}
if (daoOutSession != null) {
daoOutSession.clear();
daoOutSession = null;
}
}
}
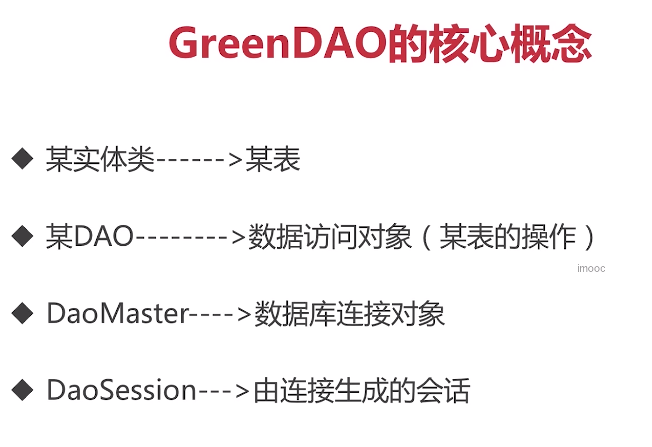
?
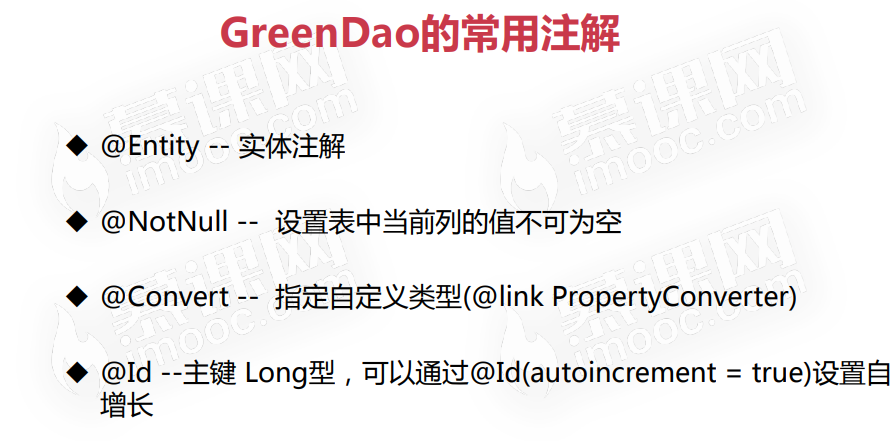
?
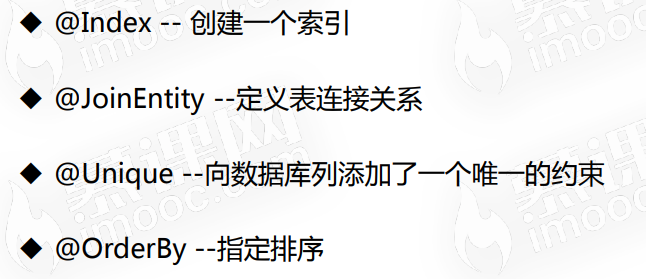
?
简单使用:
QueryBuilder<LogData> queryBuilder = GreenDaoManager.getDaoSession(StringUtils.getDatabaseName(appConfig.serverAddress)).getLogDataDao().queryBuilder();
List<LogData> searchList = queryBuilder
.where(LogDataDao.Properties.ServerAddress.eq(appConfig.serverAddress),
LogDataDao.Properties.Username.eq(appConfig.username), LogDataDao.Properties.OrderNo.like("%" + searchKey + "%"))
.offset(offset * limit)
.limit(limit)
.build().list();
?
|