Fragment
定义:
Fragment是一种嵌套在Activity中的片段,Activity主要负责一个界面,而Fragment是Activity中的片段,用于管理部分界面,可以在Activity中组合多个Fragment,或者在Activity中重复使用某个Fragment,Fragment具有自己的生命周期,能接收自己的输入事件,并且可以在运行时动态的添加或者删除Fragment。Fragment具有自己的生命周期,但是Fragment依托于Activity,所以生命周期受Activity生命周期的影响,Activity被称为宿主
在Activity中使用Fragment
在build.gradle 中导入依赖:
implementation 'androidx.navigation:navigation-fragment:2.2.1'
implementation 'androidx.navigation:navigation-ui:2.2.1'
1.静态添加:
1.宿主activity_main的布局
使用<androidx.fragment.app.FragmentContainerView>标签添加fragment,其中的name属性用于添加fragment的子布局:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="match_parent"
>
<androidx.fragment.app.FragmentContainerView
android:id="@+id/TopFragment"
android:layout_width="match_parent"
android:name="com.example.fragment.TopFragment"
android:layout_height="0dp"
android:layout_weight="1"
/>
<androidx.fragment.app.FragmentContainerView
android:id="@+id/BottomFragment"
android:name="com.example.fragment.BottomFragment"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
/>
</LinearLayout>
2.创建子布
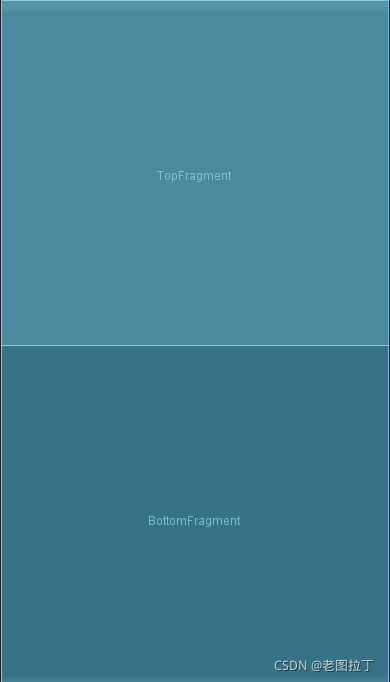
2.创建子布局
TopFragment的布局:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textColor="@color/white"
android:background="@color/purple_200"
android:text="Top"
/>
</LinearLayout>
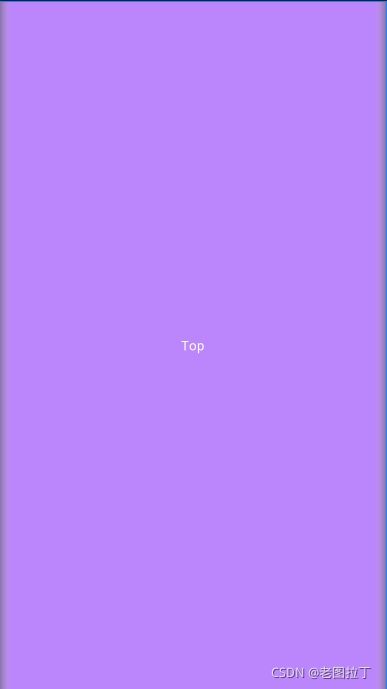
?
创建BottomFragment的布局:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:textColor="@color/white"
android:background="@color/teal_200"
android:text="bottom"
</LinearLayout>
3.创建片段类:
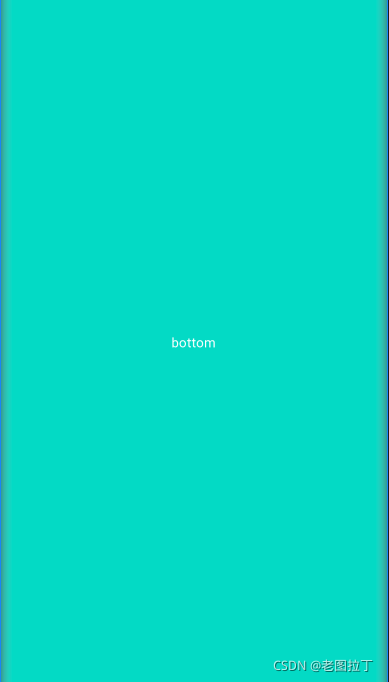
?
要创建Fragment必须扩展Fragment类,将布局传入给构造函数:
class TopFragment: Fragment(R.layout.top_fragment)
class BottomFragment:Fragment(R.layout.bottom_fragment)
4.将Fragment添加到Activity中
1.通过xml的方式,更新main_activity,要在布局中显示Fragment请使用<androidx.fragment.app.FragmentContainerView>标签,这是一种包括单个视图的布局:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="match_parent"
>
<androidx.fragment.app.FragmentContainerView
android:id="@+id/TopFragment"
android:layout_width="match_parent"
android:name="com.example.fragment.TopFragment"
android:layout_height="0dp"
android:layout_weight="1"
/>
<androidx.fragment.app.FragmentContainerView
android:id="@+id/BottomFragment"
android:name="com.example.fragment.BottomFragment"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
/>
</LinearLayout>
添加属性android:name 指定Gragment实例化的类名,当活动运行时,Fragment类将会被实例化。
2.通过程序的方式动态添加:删除掉其中的name属性取消name属性后程序将不会被自动实例化:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:orientation="vertical"
android:layout_height="match_parent"
>
<androidx.fragment.app.FragmentContainerView
android:id="@+id/TopFragment"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
/>
<androidx.fragment.app.FragmentContainerView
android:id="@+id/BottomFragment"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"
/>
</LinearLayout>
当活动运行时,可以进行FragmentManager对Fragment进行管理,在Activity中通过getSupportFragmentManager()获得该对象的实例,通过add()传入布局对象和需要布局到的容器片段id然后提交事务,事务详细内容后面会说到
kotlin:使用commit()方法必须导入依赖见:java - supportFragmentManager.commit in kotlin is not working - Stack Overflow
class MainActivity : AppCompatActivity(R.layout.activity_main)
{
override fun onCreate(savedInstanceState: Bundle?)
{
super.onCreate(savedInstanceState);
// savedInstanceState用于确保在首次创建Activity时仅添加了一次片段,当配置更改并重新创建活动时,savedInstanceState将不再是null
if (savedInstanceState == null) {
//https://stackoverflow.com/questions/64077250/supportfragmentmanager-commit-in-kotlin-is-not-working
supportFragmentManager.commit()
{
setReorderingAllowed(true);
add<TopFragment>(R.id.TopFragment);
add<BottomFragment>(R.id.BottomFragment);
}
}
}
}
java:
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
if (savedInstanceState == null) {
//beginTransaction()开启事务
getSupportFragmentManager().beginTransaction()
.setReorderingAllowed(true)
.add(R.id.fragment_container_view, ExampleFragment.class, null)
//提交事务
.commit();
}
}
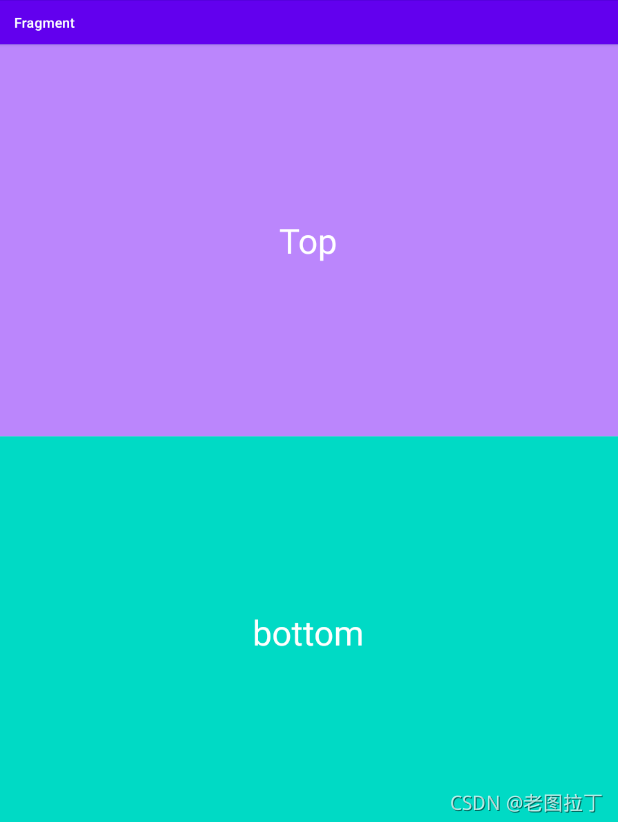
?
2.动态添加
点击返回键后将原本的BottomFragment替换为NewFragment,创建一个新的fragment和上面步骤一致,设置返回键监听事件,按下返回见后,将BottomFragment替换为NewFragment:
class MainActivity : AppCompatActivity(R.layout.activity_main)
{
override fun onCreate(savedInstanceState: Bundle?)
{
super.onCreate(savedInstanceState);
// savedInstanceState用于确保在首次创建Activity时仅添加了一次片段,当配置更改并重新创建活动时,savedInstanceState将不再是null
if (savedInstanceState == null) {
//https://stackoverflow.com/questions/64077250/supportfragmentmanager-commit-in-kotlin-is-not-working
supportFragmentManager.commit()
{
setReorderingAllowed(true);
add<TopFragment>(R.id.TopFragment);
add<BottomFragment>(R.id.BottomFragment);
}
}
}
//
// /**
// * 返回键监听事件
// */
override fun onBackPressed()
{
// 按下返回键改变布局
replaceFragment(NewFragment());
}
//
fun replaceFragment(fragment: Fragment)
{
// 创建Fragment布局管理器管理动态布局
val fragmentManager = supportFragmentManager;
// 开启事务
val transaction = fragmentManager.beginTransaction();
transaction.replace(R.id.BottomFragment,fragment);
// 提交事务
transaction.commit();
}
}
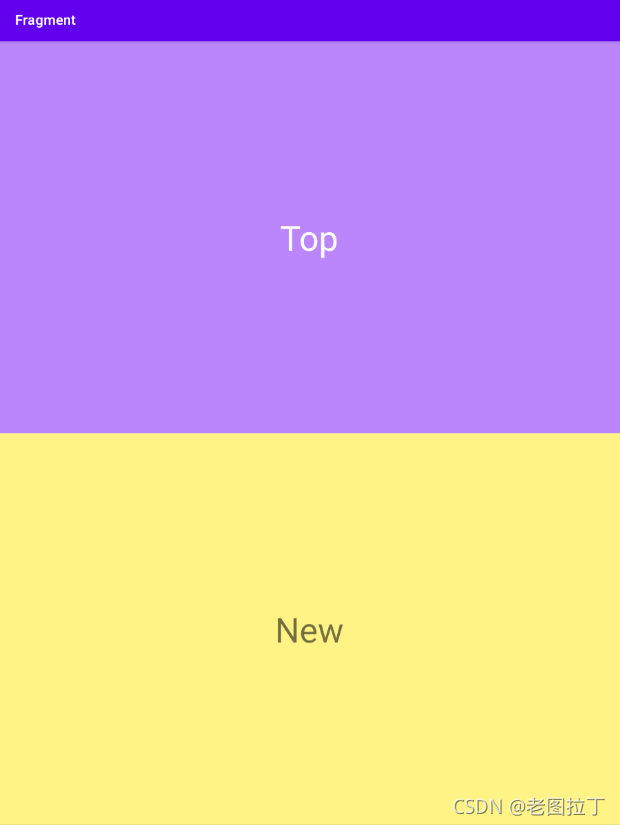
?
更新中
|