创建MainActivity使用navigation
底部导航+navigation
<?xml version="1.0" encoding="utf-8"?>
<layout>
<data>
<variable
name="bottomStyleBean"
type="com.zqq.h5shell.activity.jectpackdemo.bean.BottomStyleBean" />
</data>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.fragment.app.FragmentContainerView
android:id="@+id/fragmentview"
android:layout_width="match_parent"
android:layout_height="0dp"
android:name="androidx.navigation.fragment.NavHostFragment"
app:navGraph="@navigation/nav_jectpack"
app:defaultNavHost="false"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintBottom_toTopOf="@id/bottom_view"
app:layout_constraintVertical_weight="1"
/>
<com.google.android.material.bottomnavigation.BottomNavigationView
android:id="@+id/bottom_view"
android:layout_width="match_parent"
android:layout_height="45dp"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@id/fragmentview"
app:layout_constraintBottom_toBottomOf="parent"
/>
</androidx.constraintlayout.widget.ConstraintLayout>
</layout>
nav_jectpack
创建MainFragment、CenterFragment、MeFragment
<?xml version="1.0" encoding="utf-8"?>
<navigation xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto" android:id="@+id/nav_jectpack"
app:startDestination="@id/centerFragment">
<fragment
android:id="@+id/centerFragment"
android:name="com.zqq.h5shell.activity.jectpackdemo.fragment.CenterFragment"
android:label="CenterFragment" />
<fragment
android:id="@+id/mainFragment2"
android:name="com.zqq.h5shell.activity.jectpackdemo.fragment.MainFragment"
android:label="MainFragment" />
<fragment
android:id="@+id/meFragment2"
android:name="com.zqq.h5shell.activity.jectpackdemo.fragment.MeFragment"
android:label="MeFragment" />
</navigation>
BottomBtnBean 用于设置导航的按钮信息
package com.zqq.h5shell.activity.jectpackdemo.bean;
import androidx.databinding.BaseObservable;
import androidx.databinding.Bindable;
import androidx.databinding.PropertyChangeRegistry;
import com.zqq.h5shell.BR;
public class BottomBtnBean extends BaseObservable {
public int icon_id;
public String name;
public int width;
public int height;
private transient PropertyChangeRegistry propertyChangeRegistry = new PropertyChangeRegistry();
@Bindable
public int getIcon_id() {
return icon_id;
}
public void setIcon_id(int icon_id) {
this.icon_id = icon_id;
notifyChange(BR.icon_id);
}
@Bindable
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
notifyChange(BR.name);
}
@Bindable
public int getWidth() {
return width;
}
public void setWidth(int width) {
this.width = width;
notifyChange(BR.width);
}
@Bindable
public int getHeight() {
return height;
}
public void setHeight(int height) {
this.height = height;
notifyChange(BR.height);
}
private synchronized void notifyChange(int propertyId) {
if (propertyChangeRegistry == null) {
propertyChangeRegistry = new PropertyChangeRegistry();
}
propertyChangeRegistry.notifyChange(this, propertyId);
}
@Override
public synchronized void addOnPropertyChangedCallback(OnPropertyChangedCallback callback) {
if (propertyChangeRegistry == null) {
propertyChangeRegistry = new PropertyChangeRegistry();
}
propertyChangeRegistry.add(callback);
}
@Override
public synchronized void removeOnPropertyChangedCallback(OnPropertyChangedCallback callback) {
if (propertyChangeRegistry != null) {
propertyChangeRegistry.remove(callback);
}
}
}
创建ViewModel
package com.zqq.h5shell.activity.jectpackdemo;
import android.graphics.Color;
import androidx.lifecycle.LiveData;
import androidx.lifecycle.MutableLiveData;
import androidx.lifecycle.ViewModel;
import com.zqq.h5shell.R;
import com.zqq.h5shell.activity.jectpackdemo.bean.BottomBtnBean;
import com.zqq.h5shell.activity.jectpackdemo.bean.BottomStyleBean;
import java.util.ArrayList;
import java.util.List;
public class MyViewModel extends ViewModel {
private MutableLiveData<List<BottomBtnBean>> bottomBeans;
private MutableLiveData<BottomStyleBean> bottomStyleBean;
public MutableLiveData<BottomStyleBean> getBottomStyleBean() {
if(bottomStyleBean == null){
bottomStyleBean = new MutableLiveData<>();
initBottomStyle();
}
return bottomStyleBean;
}
private void initBottomStyle() {
if(bottomStyleBean!=null){
BottomStyleBean bottomStyle=new BottomStyleBean();
bottomStyle.setBg_color(R.drawable.bottom_bg);
bottomStyle.setNomal_color(Color.GRAY);
bottomStyle.setSelect_color(Color.BLUE);
bottomStyleBean.setValue(bottomStyle);
}
}
public void setBottomStyleBean(MutableLiveData<BottomStyleBean> bottomStyleBean) {
this.bottomStyleBean = bottomStyleBean;
}
public LiveData<List<BottomBtnBean>> getBottomBeans() {
if(bottomBeans == null){
bottomBeans = new MutableLiveData<>();
initBottomBeans();
}
return bottomBeans;
}
private void initBottomBeans() {
//自定义数据
List<BottomBtnBean> bottomBtnBeanList=new ArrayList<>();
BottomBtnBean bottomBtnBean=new BottomBtnBean();
bottomBtnBean.setName("首页");
bottomBtnBean.setIcon_id(R.drawable.home);
bottomBtnBeanList.add(bottomBtnBean);
BottomBtnBean bottomBtnBean1=new BottomBtnBean();
bottomBtnBean1.setName("中心");
bottomBtnBean1.setIcon_id(R.drawable.center);
bottomBtnBeanList.add(bottomBtnBean1);
BottomBtnBean bottomBtnBean2=new BottomBtnBean();
bottomBtnBean2.setName("我的");
bottomBtnBean2.setIcon_id(R.drawable.me);
bottomBtnBeanList.add(bottomBtnBean2);
bottomBeans.setValue(bottomBtnBeanList);
}
public void setBottomBeans(MutableLiveData<List<BottomBtnBean>> bottomBeans) {
this.bottomBeans = bottomBeans;
}
public void changeBottomBeans(){
if(bottomBeans!=null){
List<BottomBtnBean> value = bottomBeans.getValue();
for (BottomBtnBean bottomBean: value
) {
bottomBean.setName(bottomBean.getName()+"胡辣汤");
}
bottomBeans.setValue(value);
}
}
//改变底部导航选择按钮
public void changeBottomColor(int color){
if(bottomStyleBean!=null){
BottomStyleBean value = bottomStyleBean.getValue();
value.setSelect_color(color);
bottomStyleBean.setValue(value);
}
}
}
BottomStyleBean 用来修改导航的颜色信息
package com.zqq.h5shell.activity.jectpackdemo.bean;
import androidx.databinding.BaseObservable;
import androidx.databinding.Bindable;
import androidx.databinding.PropertyChangeRegistry;
import com.zqq.h5shell.BR;
public class BottomStyleBean extends BaseObservable {
public int bg_color;
public int nomal_color;
public int select_color;
private transient PropertyChangeRegistry propertyChangeRegistry = new PropertyChangeRegistry();
@Bindable
public int getBg_color() {
return bg_color;
}
public void setBg_color(int bg_color) {
this.bg_color = bg_color;
notifyChange(BR.bg_color);
}
@Bindable
public int getNomal_color() {
return nomal_color;
}
public void setNomal_color(int nomal_color) {
this.nomal_color = nomal_color;
notifyChange(BR.nomal_color);
}
@Bindable
public int getSelect_color() {
return select_color;
}
public void setSelect_color(int select_color) {
this.select_color = select_color;
notifyChange(BR.select_color);
}
private synchronized void notifyChange(int propertyId) {
if (propertyChangeRegistry == null) {
propertyChangeRegistry = new PropertyChangeRegistry();
}
propertyChangeRegistry.notifyChange(this, propertyId);
}
@Override
public synchronized void addOnPropertyChangedCallback(OnPropertyChangedCallback callback) {
if (propertyChangeRegistry == null) {
propertyChangeRegistry = new PropertyChangeRegistry();
}
propertyChangeRegistry.add(callback);
}
@Override
public synchronized void removeOnPropertyChangedCallback(OnPropertyChangedCallback callback) {
if (propertyChangeRegistry != null) {
propertyChangeRegistry.remove(callback);
}
}
}
MainActivity
package com.zqq.h5shell.activity.jectpackdemo;
import android.content.res.ColorStateList;
import android.content.res.XmlResourceParser;
import android.graphics.Color;
import android.graphics.ColorFilter;
import android.graphics.PorterDuff;
import android.graphics.PorterDuffColorFilter;
import android.graphics.drawable.Drawable;
import android.os.Build;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.annotation.RequiresApi;
import androidx.appcompat.app.AppCompatActivity;
import androidx.databinding.DataBindingUtil;
import androidx.lifecycle.MutableLiveData;
import androidx.lifecycle.Observer;
import androidx.lifecycle.ViewModelProvider;
import androidx.navigation.NavController;
import androidx.navigation.fragment.NavHostFragment;
import com.alibaba.android.arouter.facade.annotation.Route;
import com.google.android.material.bottomnavigation.BottomNavigationView;
import com.zqq.h5shell.ARouterContent;
import com.zqq.h5shell.R;
import com.zqq.h5shell.activity.jectpackdemo.bean.BottomBtnBean;
import com.zqq.h5shell.activity.jectpackdemo.bean.BottomStyleBean;
import com.zqq.h5shell.databinding.ActivityJectpackMainBinding;
import java.util.List;
@Route(path = ARouterContent.MainActivity)
public class MainActivity extends AppCompatActivity {
private ActivityJectpackMainBinding binding;
private MyViewModel myViewModel;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding = DataBindingUtil.setContentView(this, R.layout.activity_jectpack_main);
initView();
}
private void initView() {
myViewModel = new ViewModelProvider(this).get(MyViewModel.class);
binding.setBottomStyleBean(myViewModel.getBottomStyleBean().getValue());
//监听底部数据变化,设置按钮和文字
myViewModel.getBottomBeans().observe(this, new Observer<List<BottomBtnBean>>() {
@Override
public void onChanged(List<BottomBtnBean> bottomBtnBeans) {
setMenuItem(bottomBtnBeans);
}
});
//监听变化后修改导航显示
myViewModel.getBottomStyleBean().observe(this, new Observer<BottomStyleBean>() {
@Override
public void onChanged(BottomStyleBean bottomStyleBean) {
Menu menu = binding.bottomView.getMenu();
setMenuBg(menu);
}
});
//navigation
NavHostFragment navHostFragment = (NavHostFragment) getSupportFragmentManager().findFragmentById(R.id.fragmentview);
NavController navController = navHostFragment.getNavController();
binding.bottomView.setOnNavigationItemSelectedListener(new BottomNavigationView.OnNavigationItemSelectedListener() {
@RequiresApi(api = Build.VERSION_CODES.O)
@Override
public boolean onNavigationItemSelected(@NonNull MenuItem menuItem) {
switch (menuItem.getItemId()){
case 0:
navController.navigate(R.id.mainFragment2);
break;
case 1:
navController.navigate(R.id.centerFragment);
break;
case 2:
navController.navigate(R.id.meFragment2);
break;
}
return true;
}
});
}
private void setMenuItem(List<BottomBtnBean> bottomBtnBeans) {
//设置bottom的menu的item信息
Menu menu = binding.bottomView.getMenu();
menu.clear();
for(int i=0;i<bottomBtnBeans.size();i++){
BottomBtnBean btnBean = bottomBtnBeans.get(i);
menu.add(0,i,i,btnBean.getName());
menu.getItem(i).setIcon(btnBean.getIcon_id());
}
setMenuBg(menu);
}
private void setMenuBg(Menu menu) {
MutableLiveData<BottomStyleBean> bottomStyleBean = myViewModel.getBottomStyleBean();
BottomStyleBean value = bottomStyleBean.getValue();
// 设置颜色
int[][] states = new int[2][1];
states[0][0]=-android.R.attr.state_checked;
states[1][0]=android.R.attr.state_checked;
int[] colors =new int[]{value.getNomal_color(),value.getSelect_color()};
ColorStateList colorStateList = new ColorStateList(states,colors);
binding.bottomView.setItemTextColor(colorStateList);
binding.bottomView.setItemIconTintList(colorStateList);
binding.bottomView.setBackgroundResource(value.getBg_color());
menu.getItem(0).setChecked(true);
}
}
MeFragment 代码更新viewmodel
package com.zqq.h5shell.activity.jectpackdemo.fragment;
import android.app.StatusBarManager;
import android.graphics.Color;
import android.os.Build;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.view.WindowManager;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.databinding.DataBindingUtil;
import androidx.fragment.app.Fragment;
import androidx.lifecycle.ViewModelProvider;
import com.bumptech.glide.Glide;
import com.bumptech.glide.request.RequestOptions;
import com.zqq.h5shell.R;
import com.zqq.h5shell.activity.jectpackdemo.MyViewModel;
import com.zqq.h5shell.databinding.FragmentDemoMeBinding;
public class MeFragment extends Fragment {
FragmentDemoMeBinding binding;
MyViewModel myViewModel;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
binding = DataBindingUtil.inflate(inflater, R.layout.fragment_demo_me,container,false);
initView();
return binding.getRoot();
}
private void initView() {
setStatusBar();
RequestOptions requestOptions=new RequestOptions()
.circleCrop();
Glide.with(requireActivity())
.load(R.drawable.head)
.apply(requestOptions)
.into(binding.ivHead);
binding.tvName.setText("东门吹冰");
myViewModel = new ViewModelProvider(requireActivity()).get(MyViewModel.class);
binding.tvRed.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
myViewModel.changeBottomColor(Color.RED);
}
});
binding.tvBlue.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
myViewModel.changeBottomColor(Color.BLUE);
}
});
binding.tvGreen.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
myViewModel.changeBottomColor(Color.GREEN);
}
});
}
/*沉浸式*/
protected void setStatusBar() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {//5.0及以上
View decorView = requireActivity().getWindow().getDecorView();
int option = View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN
| View.SYSTEM_UI_FLAG_LAYOUT_STABLE;
decorView.setSystemUiVisibility(option);
//根据上面设置是否对状态栏单独设置颜色
requireActivity(). getWindow().setStatusBarColor(Color.TRANSPARENT);
} else if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.KITKAT) {//4.4到5.0
WindowManager.LayoutParams localLayoutParams = requireActivity().getWindow().getAttributes();
localLayoutParams.flags = (WindowManager.LayoutParams.FLAG_TRANSLUCENT_STATUS | localLayoutParams.flags);
}
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {//android6.0以后可以对状态栏文字颜色和图标进行修改
requireActivity().getWindow().getDecorView().setSystemUiVisibility(View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN | View.SYSTEM_UI_FLAG_LIGHT_STATUS_BAR);
}
}
}
实现效果
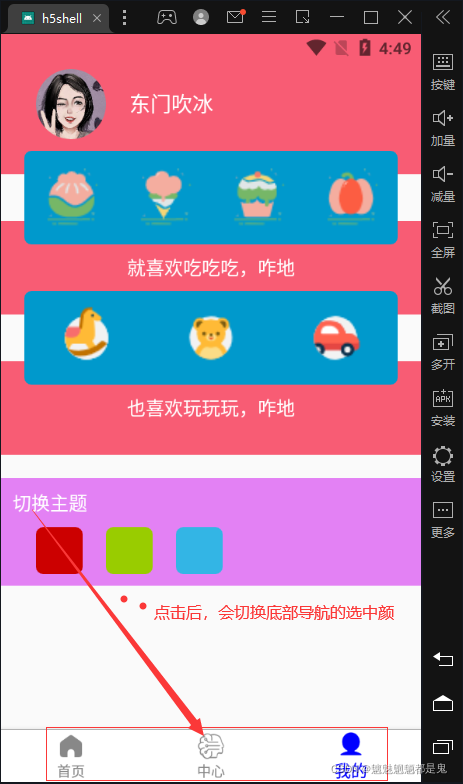 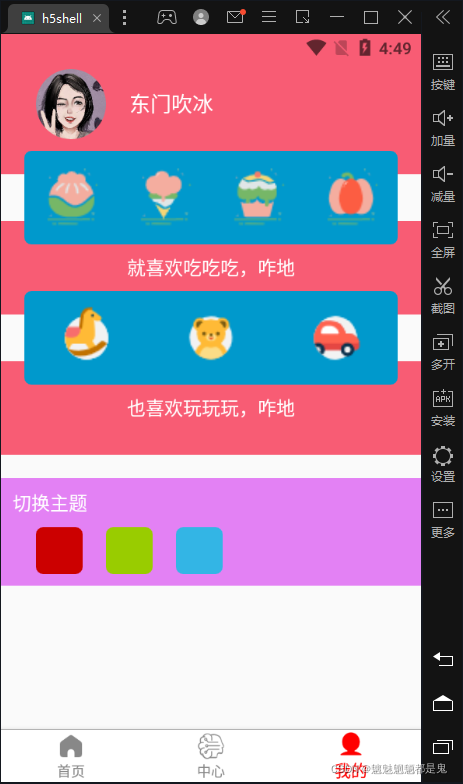
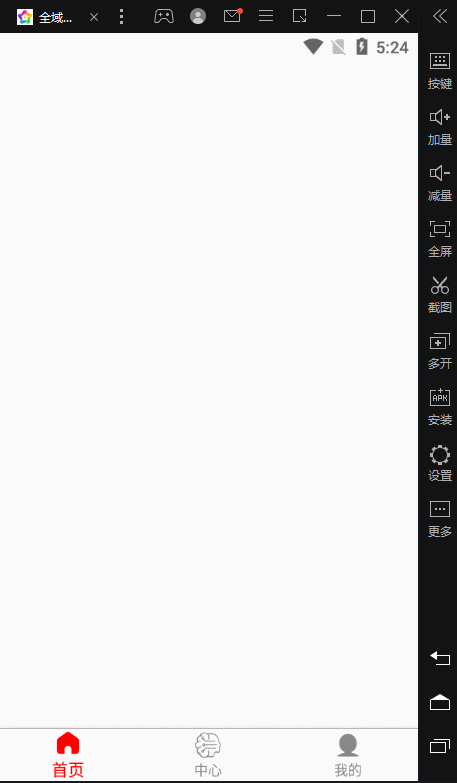
|