百度SDK流程
1、下载开发包
访问百度地图开发平台,依次选择:开发文档->Android 定位SDK->产品下载->自定义下载,勾选百度定位包后解压、下载 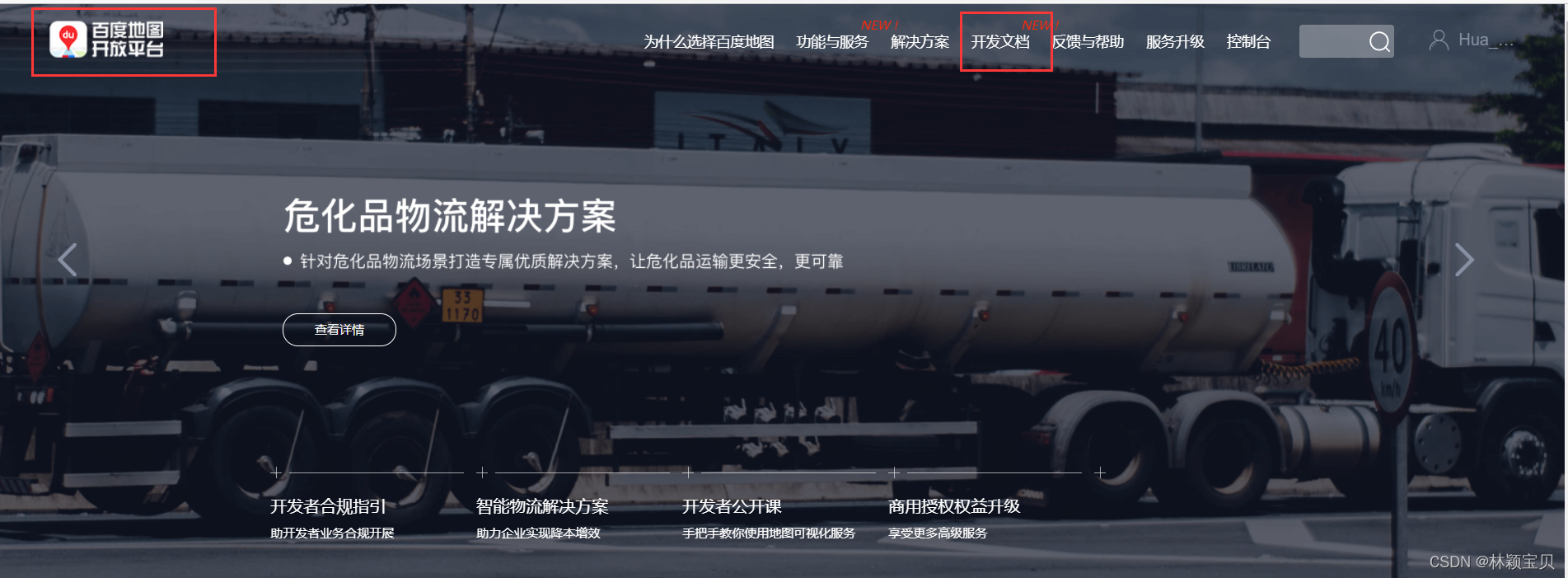 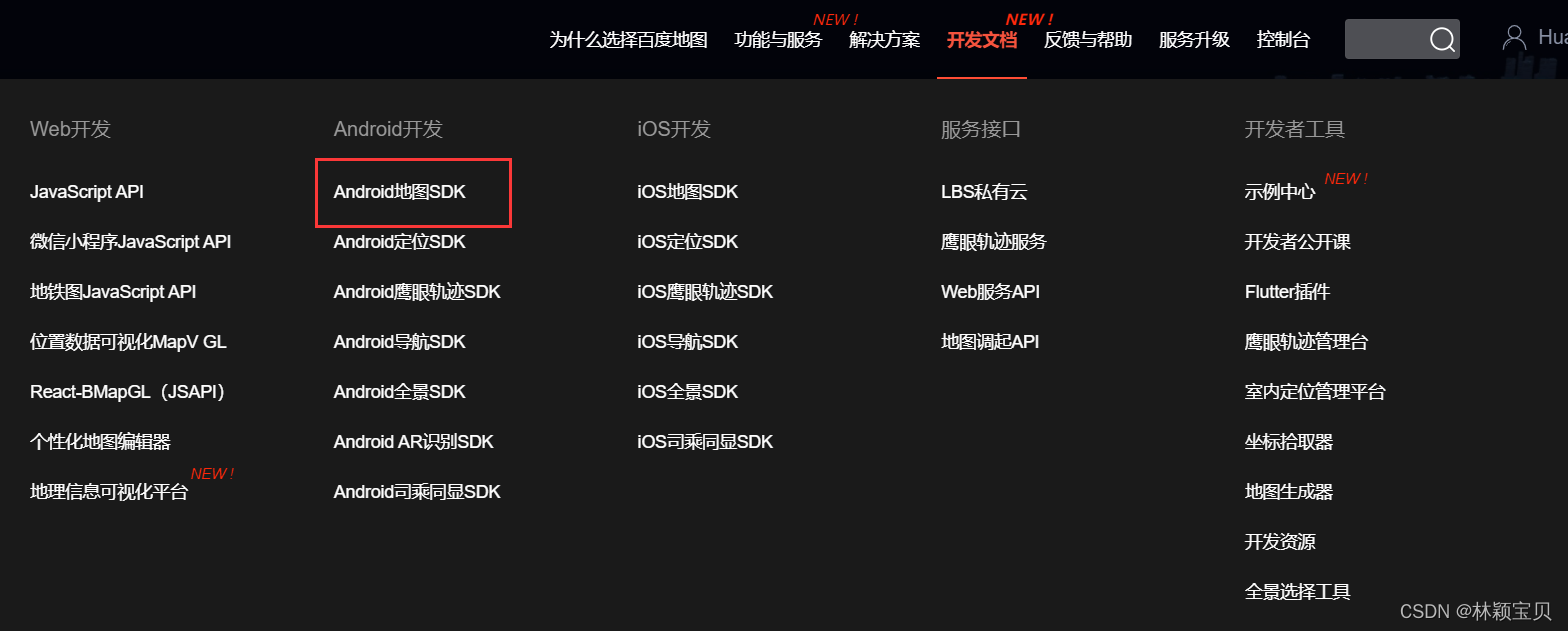 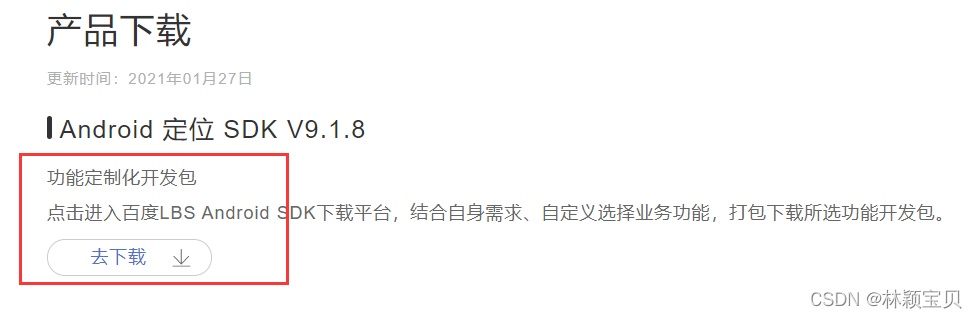 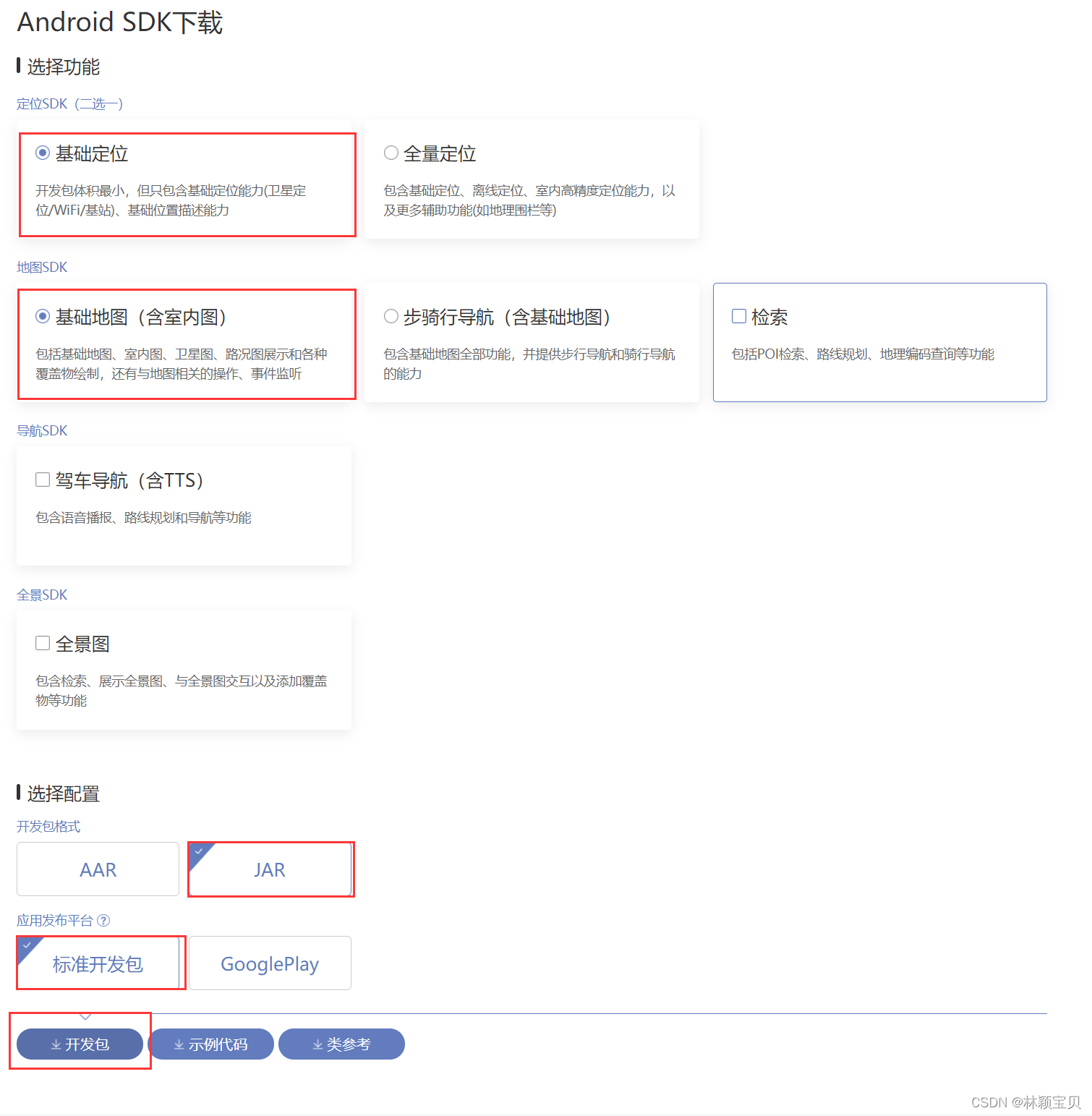 
2、Android Studio配置
在项目里,新建名为MyLBS的模块 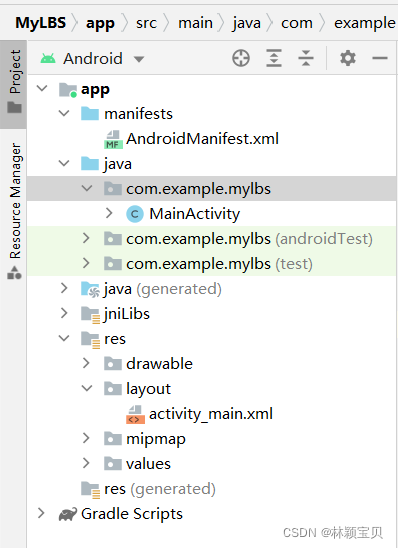 选择模块视图为Project,复制定位包BaiduLBS_Android.jar至模块MyLBS的libs文件夹里,然后右键jar文件,选择“Add As Library” 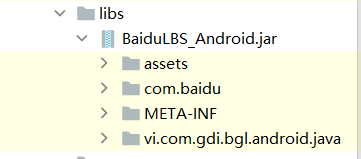 在main文件夹下新建名为jniLibs的文件夹,复制存放.so文件(share object)的多个文件夹至jniLibs文件夹 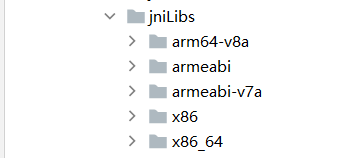
3、注册百度开发者账号并登录
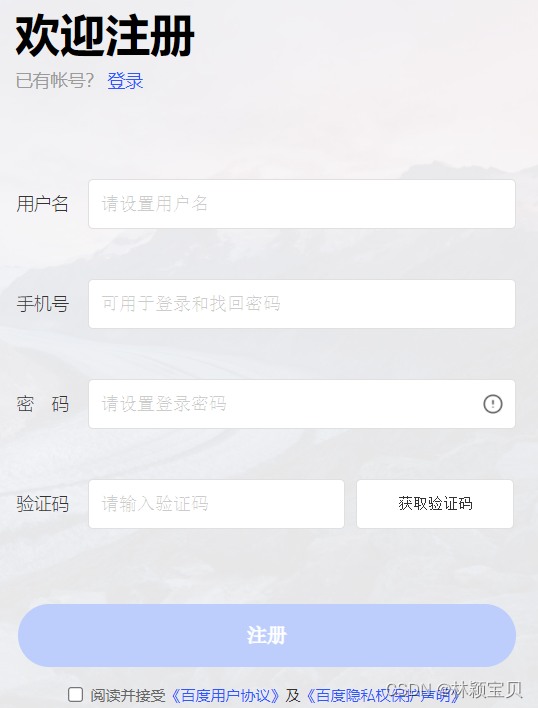
4、申请应用的Key
登录百度账号后,访问网站进入应用的控制台,输入正确的SHA1码和包名 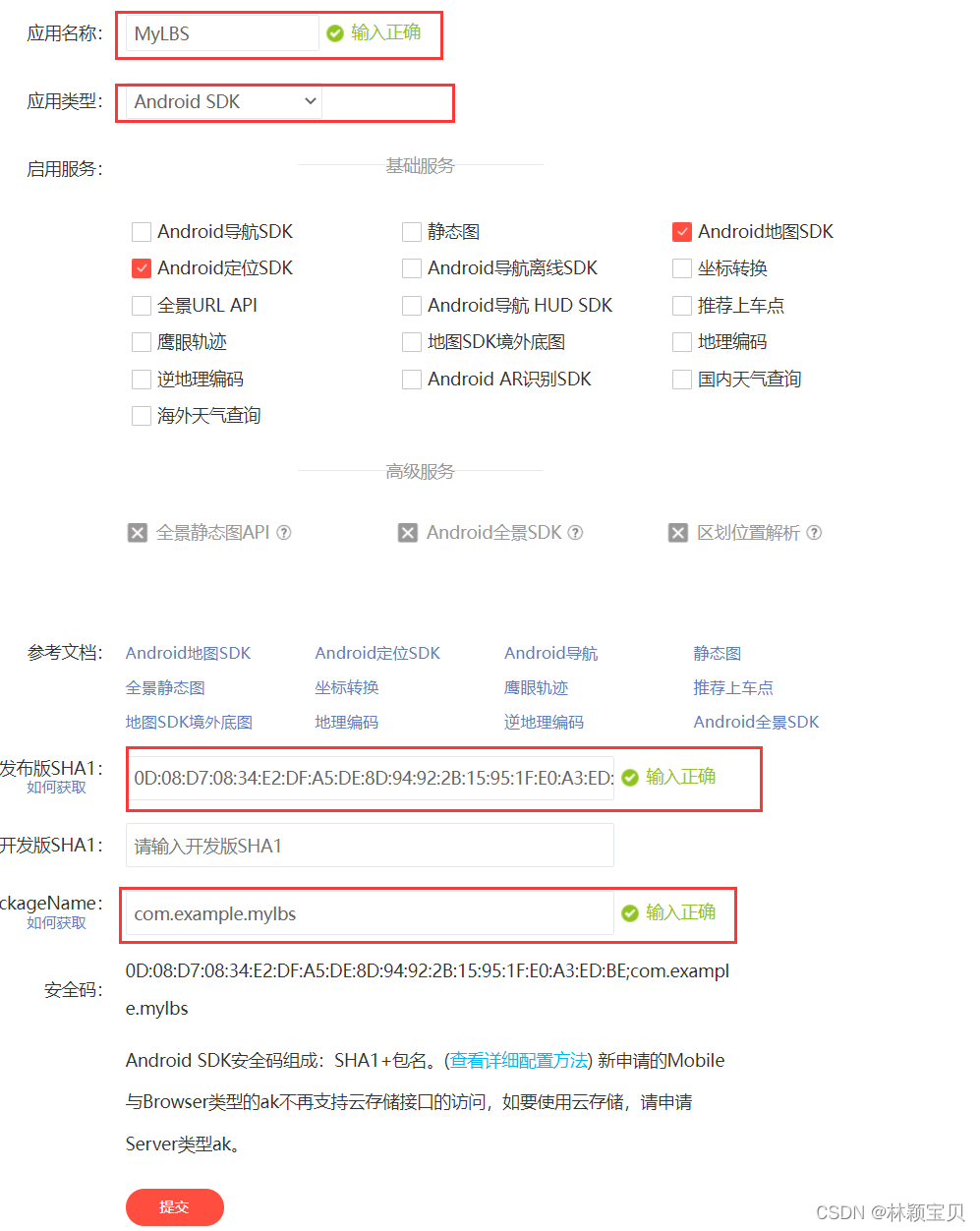
获取SHA1码
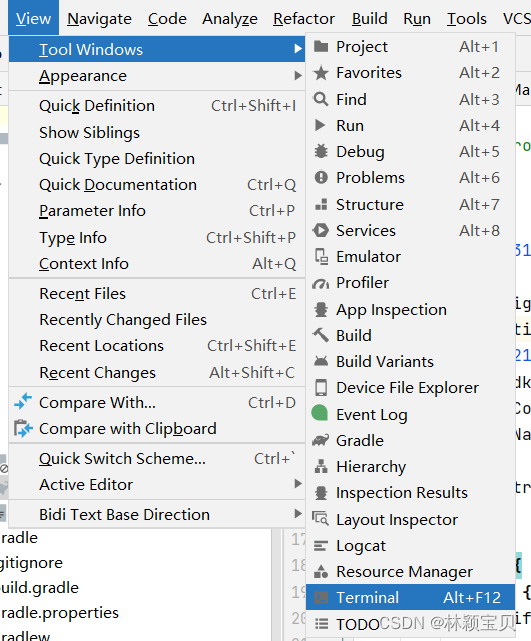 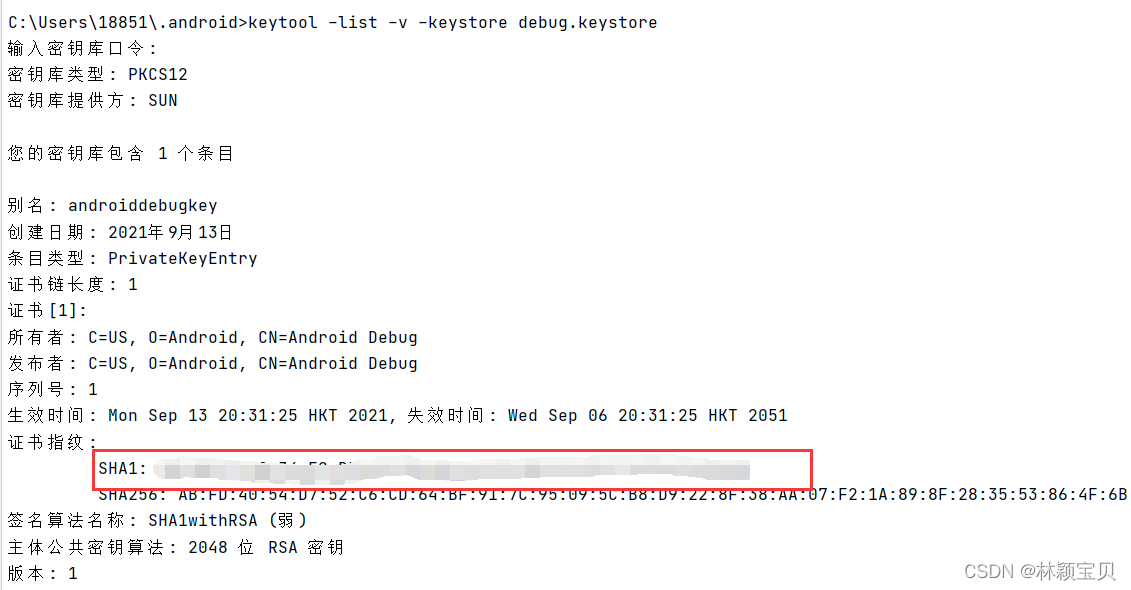
获取包名
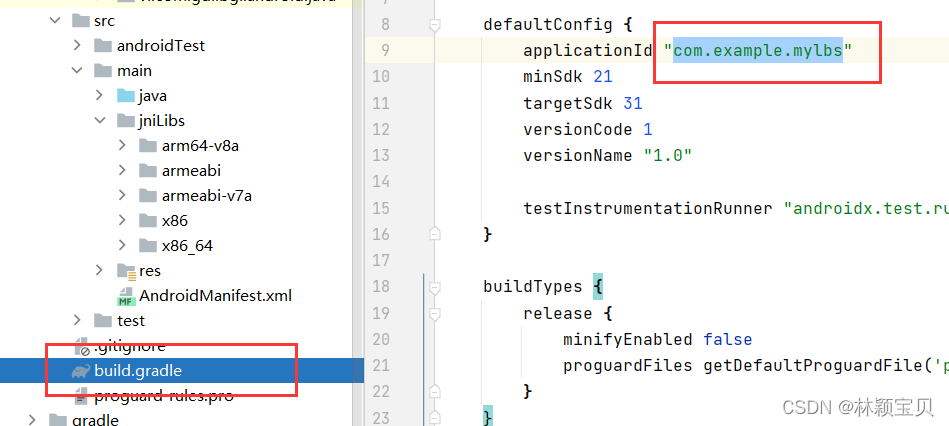
百度定位
1、配置清单
百度定位应用开发,在清单文件里,需要注册的权限如下(共6个):
<!--百度定位所需要权限,前面2个是LOCATE权限组的2个危险权限-->
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/>
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/>
<!--百度定位所需要的普通权限-->
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.CHANGE_WIFI_STATE"/>
<uses-permission android:name="android.permission.INTERNET"/> <!--因为程序要与百度云服务交互-->
在清单文件里声明自己的service组件
<service
android:name="com.baidu.location.f"
android:enabled="true"
android:process=":remote" />
在清单文件的登记百度位置应用的Key 
<meta-data
android:name="com.baidu.lbsapi.API_KEY"
android:value="bZnzosMhymU7GgnHGRZ7GeV42CZogIRN"/><!--应用Key是在百度开发者页面里生成的,需要替换-->
2、编写MainActivity.java文件
动态请求用户允许使用该权限
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 1);
}else {
requestLocation();
}
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
switch (requestCode) {
case 1:
if (grantResults[0] != PackageManager.PERMISSION_GRANTED) {
Toast.makeText(this, "没有定位权限!", Toast.LENGTH_LONG).show();
finish();
} else {
requestLocation();
}
}
}
对定位进行初始化
private void initLocation() {
mLocationClient = new LocationClient(getApplicationContext());
mLocationClient.registerLocationListener(new MyLocationListener());
SDKInitializer.initialize(getApplicationContext());
setContentView(R.layout.activity_main);
mapView = findViewById(R.id.bmapView);
baiduMap = mapView.getMap();
tv_Lat = findViewById(R.id.tv_Lat);
tv_Lon = findViewById(R.id.tv_Lon);
tv_Add = findViewById(R.id.tv_Add);
LocationClientOption option = new LocationClientOption();
option.setScanSpan(1000);
option.setLocationMode(LocationClientOption.LocationMode.Hight_Accuracy);
option.setIsNeedAddress(true);
mLocationClient.setLocOption(option);
}
百度位置监听器
private class MyLocationListener implements BDLocationListener {
@Override
public void onReceiveLocation(BDLocation bdLocation) {
tv_Lat.setText(bdLocation.getLatitude()+"");
tv_Lon.setText(bdLocation.getLongitude()+"");
tv_Add.setText(bdLocation.getAddrStr());
if(bdLocation.getLocType()==BDLocation.TypeGpsLocation || bdLocation.getLocType()==BDLocation.TypeNetWorkLocation){
navigateTo(bdLocation);
}
}
}
3、设计界面
编写activity_main.xml文件
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!--百度地图控件-->
<com.baidu.mapapi.map.MapView
android:id="@+id/bmapView"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:clickable="true" />
<!--位置文本布局的背景色代码的前2位代码为透明度-->
<LinearLayout
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:background="#e0000000"
android:orientation="vertical" >
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="12dp"
android:layout_marginTop="20dp"
android:orientation="horizontal" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="纬度:"
android:textColor="#ffffff"
android:textSize="15dp" />
<TextView
android:id="@+id/tv_Lat"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:textColor="#ffffff"
android:textSize="15dp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="12dp"
android:layout_marginTop="10dp"
android:orientation="horizontal" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="经度:"
android:textColor="#ffffff"
android:textSize="15dp" />
<TextView
android:id="@+id/tv_Lon"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:textColor="#ffffff"
android:textSize="15dp" />
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="10dp"
android:layout_marginLeft="12dp"
android:layout_marginTop="10dp"
android:orientation="horizontal" >
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="地址:"
android:textColor="#ffffff"
android:textSize="15dp" />
<TextView
android:id="@+id/tv_Add"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:textColor="#ffffff"
android:textSize="15dp" />
</LinearLayout>
</LinearLayout>
</FrameLayout>
效果展示
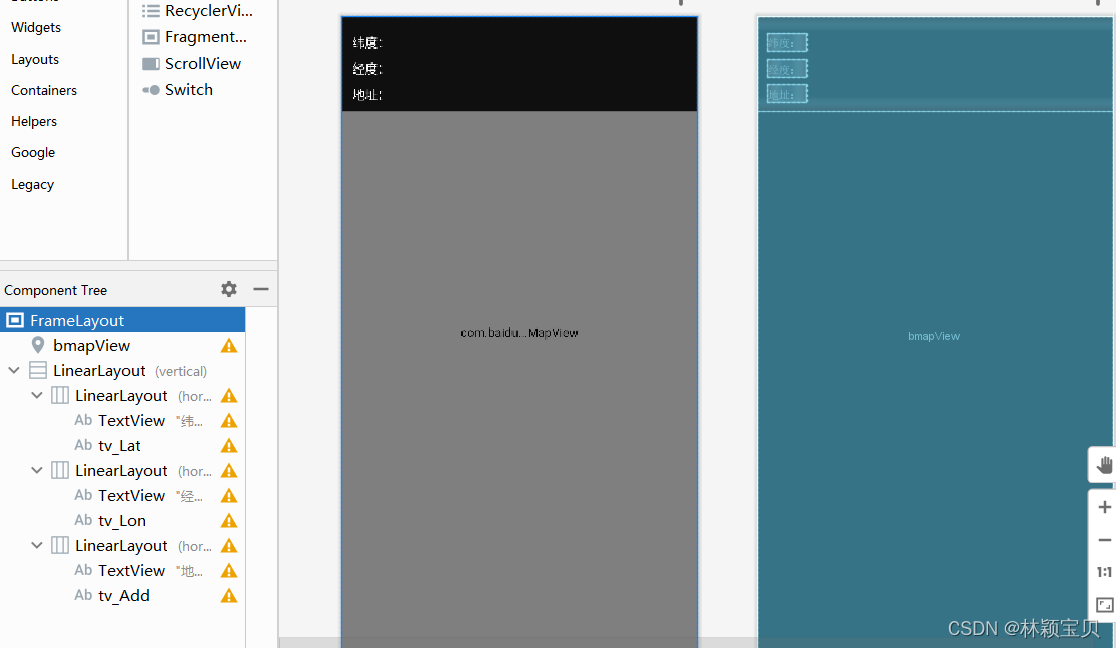
效果展示
1、未成功结果展示
当代码编写好之后,用AVD Manager运行没有报错,但定位一直在美国 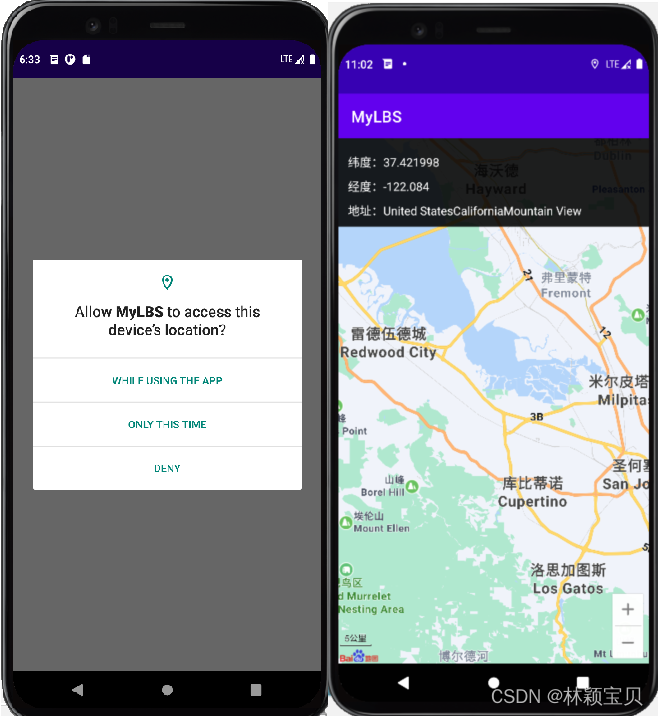
2、成功结果展示
经过百度查询,发现并不是代码的问题,而是要用真机测试具体步骤如下 首先进入开发者模式,开启USB调试 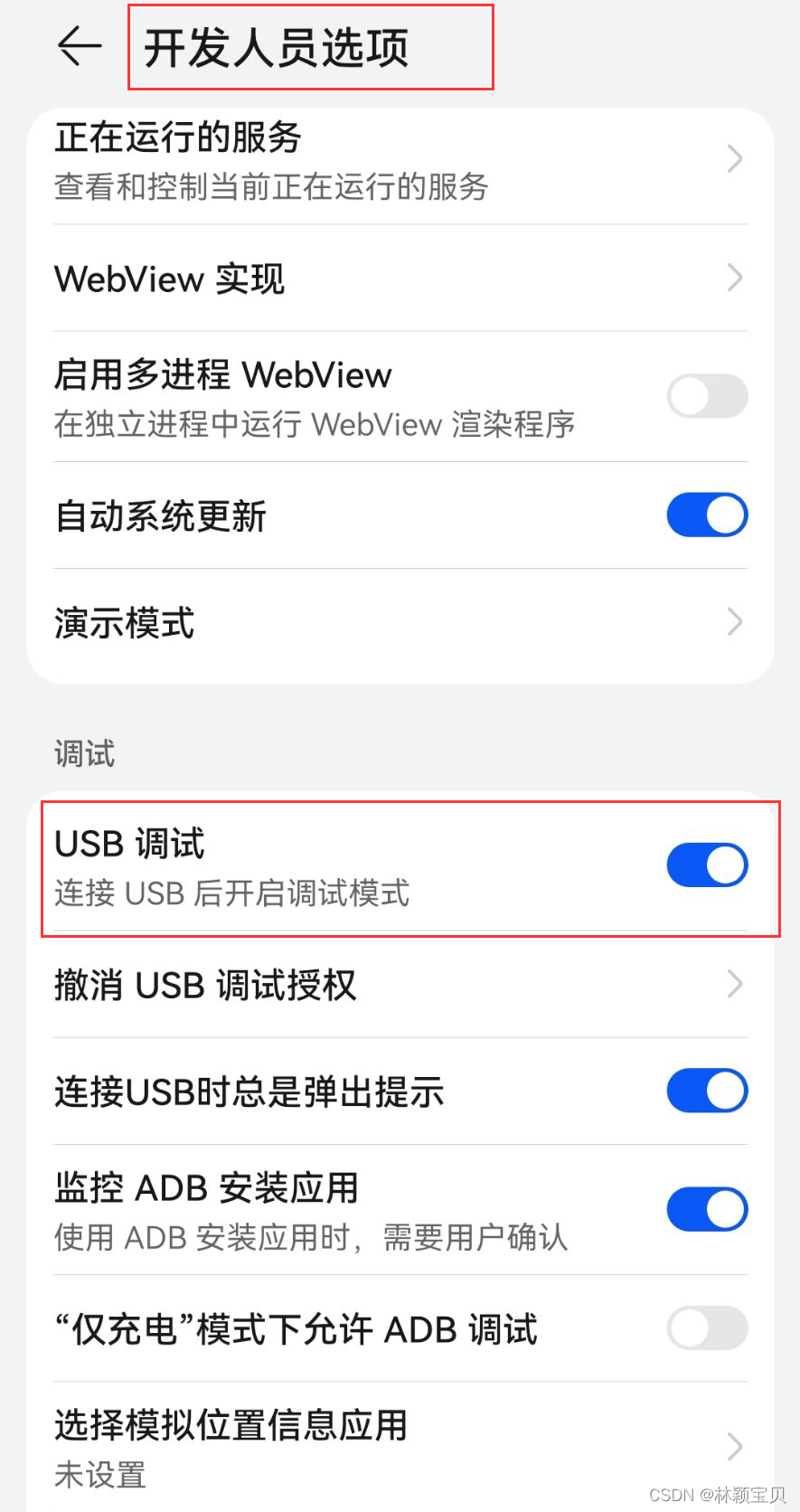 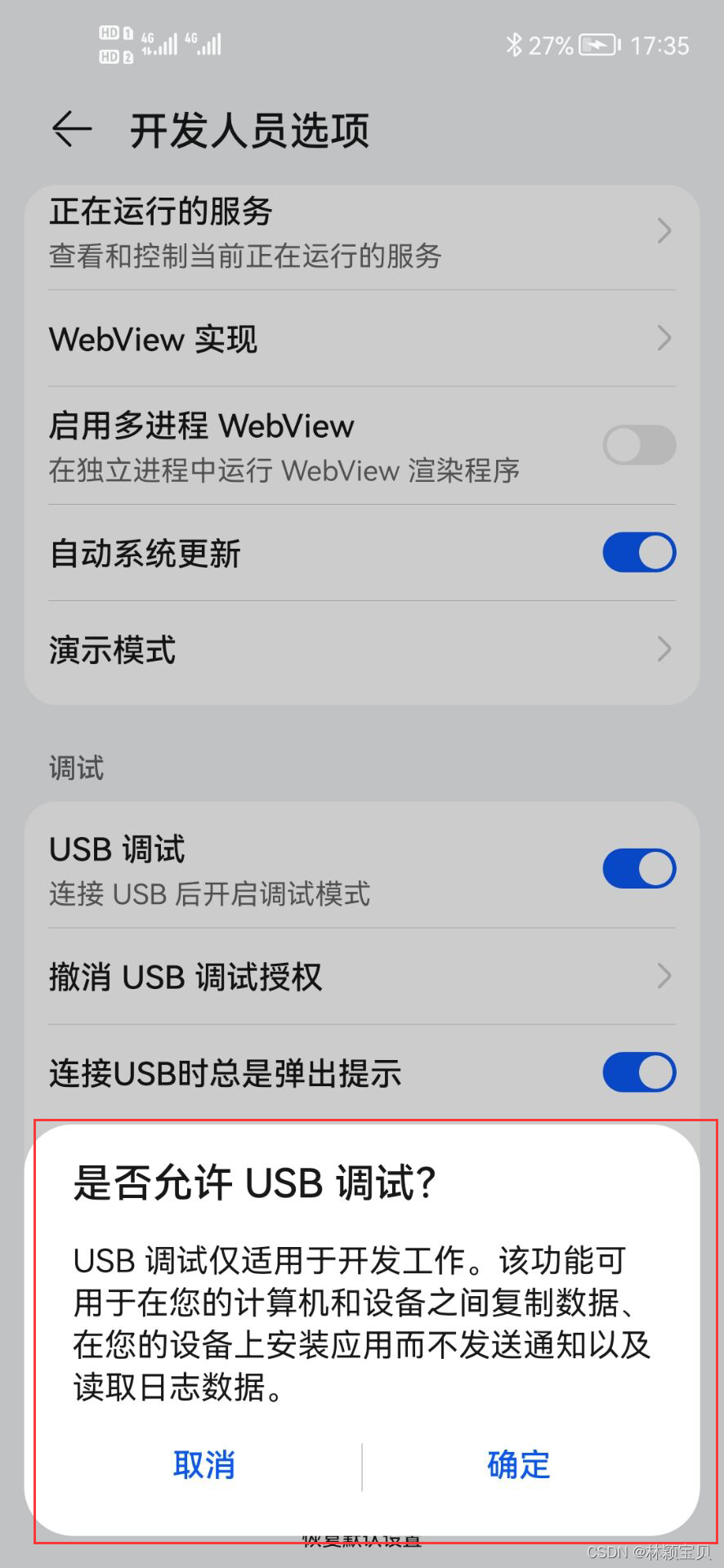 使用数据线连接到电脑,选择传输文件,并且允许USB调试 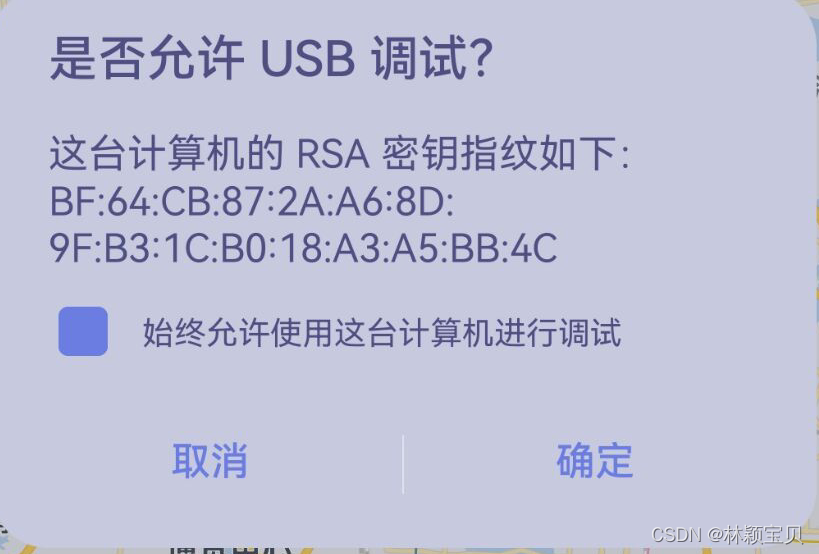 在运行时选择所连接的设备并运行 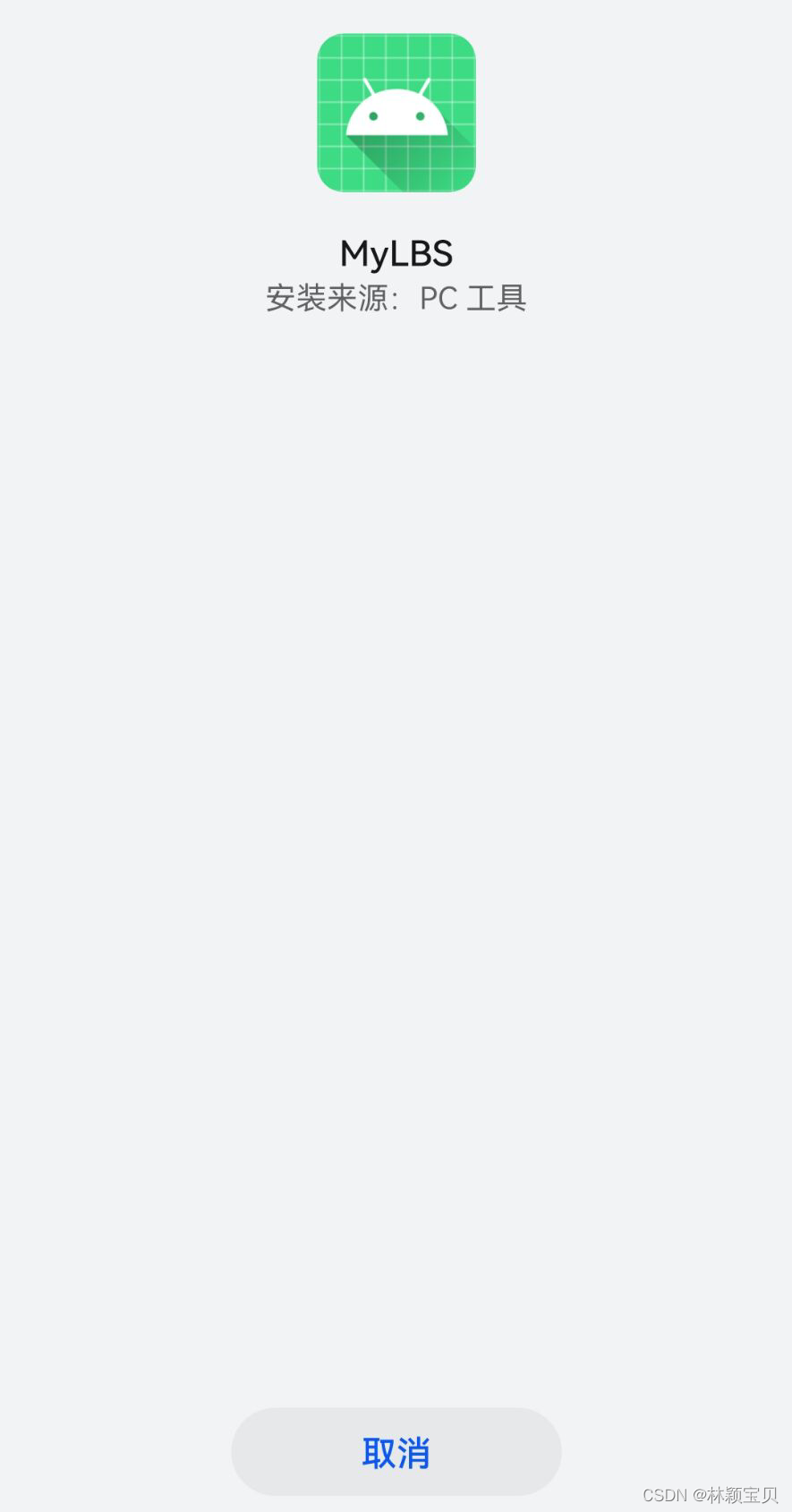 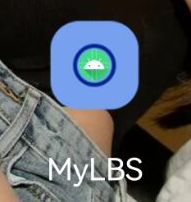 安装成功,打开 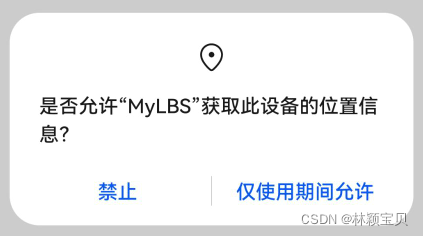 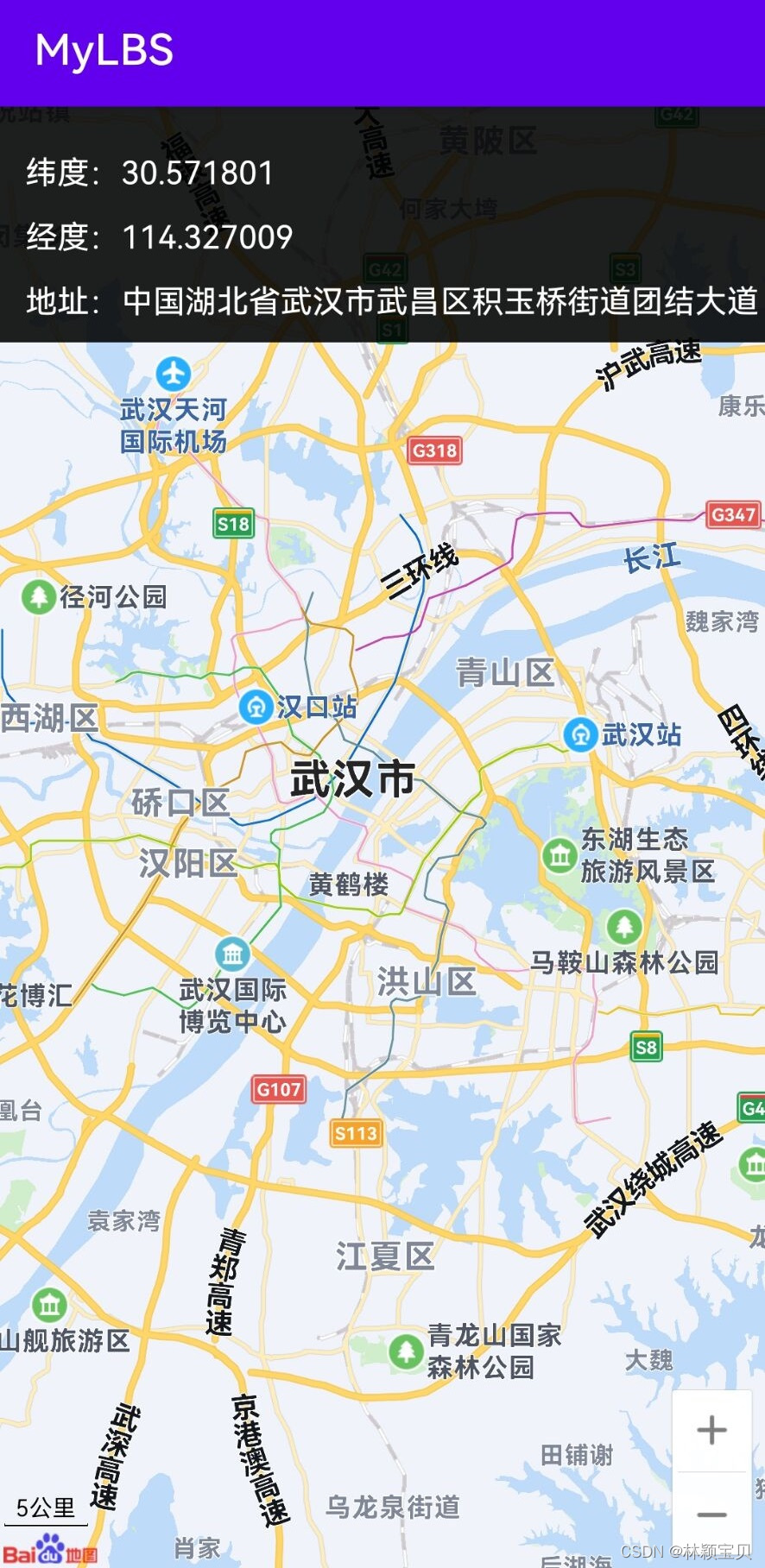
参考博客
https://blog.csdn.net/qq_44489836/article/details/106318639
Gitee源码
链接
|