MainActivity,java
package com.liuli.liebiao;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.TextView;
import java.text.SimpleDateFormat;
import java.util.Date;
public class MainActivity extends AppCompatActivity {
String[] title = null;
int[] image = null;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
title = new String[]{
"考试测试题1",
"考试测试题2",
"考试测试题3",
"考试测试题4"
};
image = new int[]{
R.drawable.a,
R.drawable.b,
R.drawable.c,
R.drawable.d,
};
ListView lv = findViewById(R.id.lv);
//加分块
View id1 = findViewById(R.id.b1);
View id2 = findViewById(R.id.b2);
id1.setOnClickListener(view ->{
lv.setAdapter(new shxian());
});
id2.setOnClickListener(view ->{
lv.setAdapter(null);
});
}
class shxian extends BaseAdapter{
@Override
public int getCount() {
return title.length;
}
@Override
public Object getItem(int i) {
return title[i];
}
@Override
public long getItemId(int i) {
return i;
}
@Override
public View getView(int i, View view, ViewGroup viewgroup) {
/*
普通加分
if (view == null) {
view = View.inflate(MainActivity.this,R.layout.lst_view_tem,null);
}
TextView titles = view.findViewById(R.id.title);
TextView time = view.findViewById(R.id.time);
ImageView img = view.findViewById(R.id.img);
titles.setText(title[i]);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-mm-dd");
time.setText(sdf.format(new Date()));
img.setImageResource(image[i]);
return view;*/
//多加分
Hold hold = null;
if (view == null) {
view = View.inflate(MainActivity.this,R.layout.lst_view_tem,null);
hold = new Hold();
hold.titles = view.findViewById(R.id.title);
hold.time = view.findViewById(R.id.time);
hold.img = view.findViewById(R.id.img);
view.setTag(hold);
}
hold = (Hold)view.getTag();
hold.titles.setText(title[i]);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-mm-dd");
hold.time.setText(sdf.format(new Date()));
hold.img.setImageResource(image[i]);
return view;
}
}
class Hold{
TextView titles;
TextView time;
ImageView img;
}
}
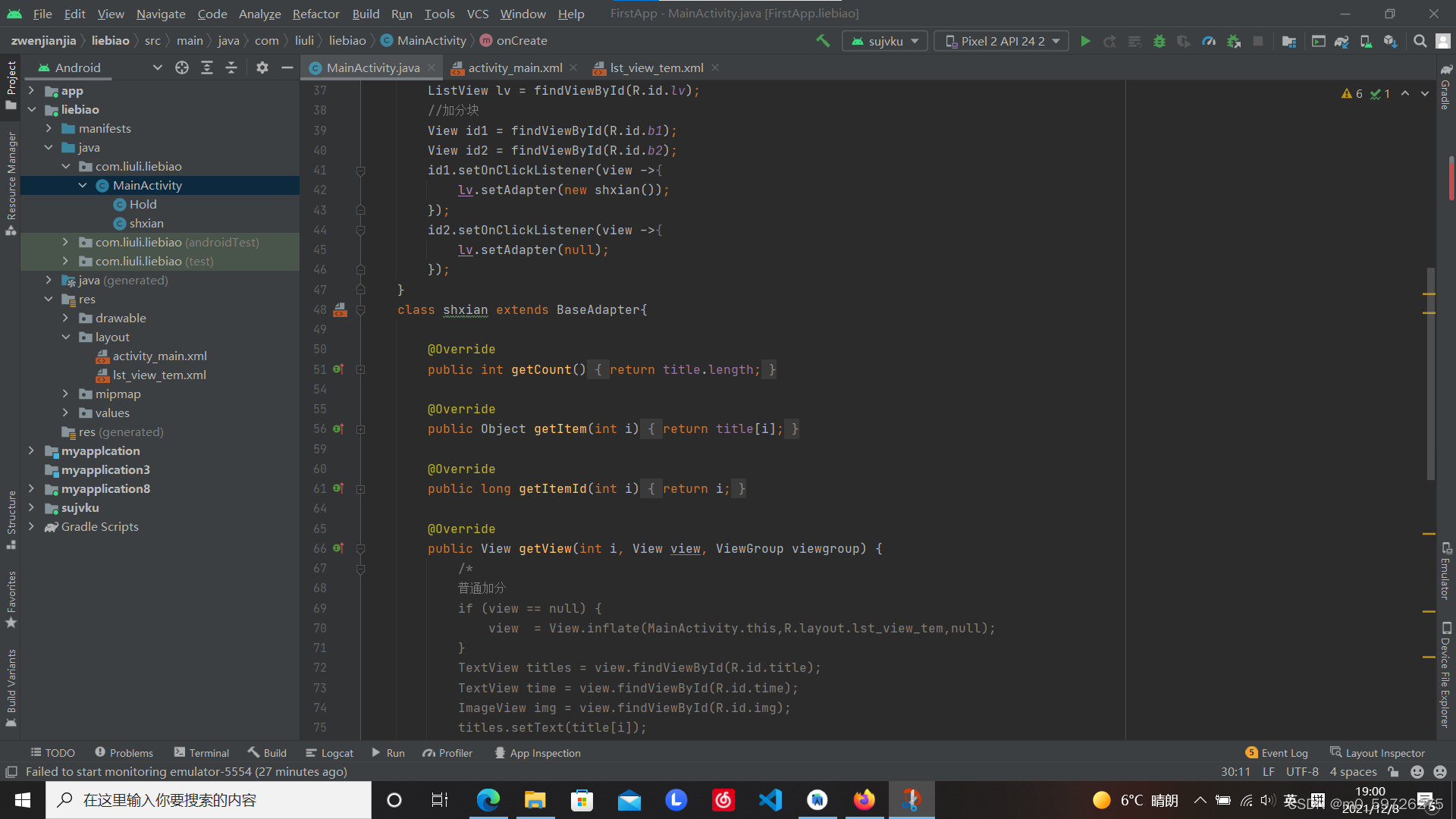
?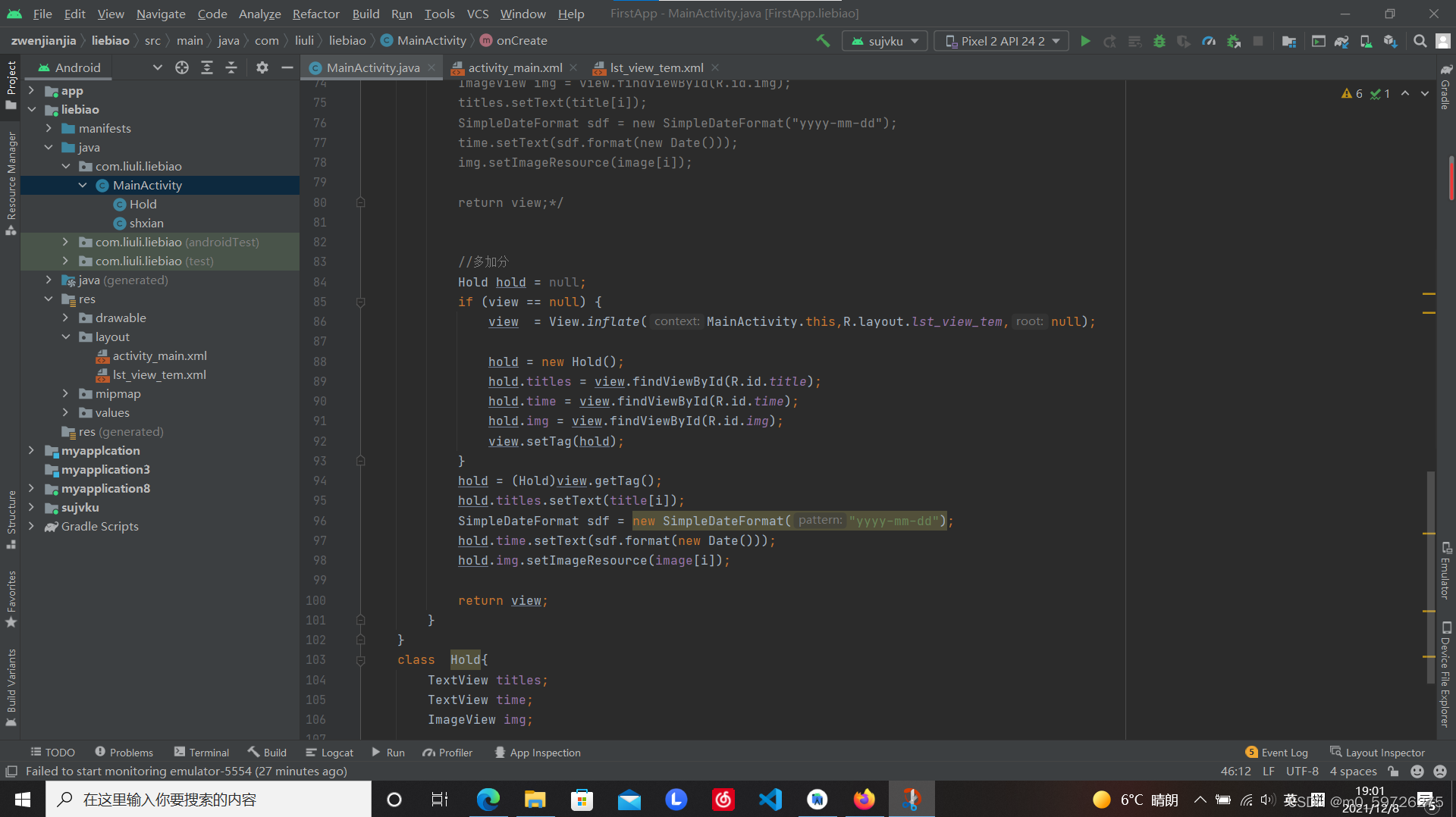
?
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/constraintLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ListView
android:id="@+id/lv"
android:layout_width="match_parent"
android:layout_height="0dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_weight="1"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toBottomOf="@+id/lv">
<Button
android:id="@+id/b1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="按钮1" />
<Button
android:id="@+id/b2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="按钮2" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="按钮3" />
<Button
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="按钮4" />
</LinearLayout>
</androidx.constraintlayout.widget.ConstraintLayout>
lst_view_tem
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="5dp"
>
<RelativeLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1">
<TextView
android:id="@+id/title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="考试测试题1"
/>
<TextView
android:id="@+id/time"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="2021-12-06"
android:layout_alignBottom="@id/img"
/>
<ImageView
android:id="@+id/img"
android:layout_width="160dp"
android:layout_height="120dp"
android:src="@drawable/a"
android:layout_alignParentRight="true"/>
</RelativeLayout>
</LinearLayout>
|