17.键值对数据存储SharedPreferences介绍
(1)介绍
SharedPreferences是一种轻量级的数据存储方式,采用键值对的存储方式。
SharedPreferences只能存储少量数据,大量数据不能使用该方式存储,支持存储的数据类型有booleans, floats, ints, longs, and strings。
SharedPreferences存储到一个XML文件中的
(2)向 Shared preferences 文件写入数据
? 1)首先必须获得 SharedPreferences.Editor 对象;
? 2)通过 putInt()、putString() 等方式写入键值对数据;
? 3)△最后通过调用 apply() 或 commit() 方法保存数据
(3)从 Shared preferences 文件读取数据
首先获得文件对应的 SharedPreferences 对象;
通过 getInt()、getString() 等方法指定 key 获取数据;
(4)学习使用 Shared preferences:登录时记住密码
登录布局源代码如下:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="48dp"
>
<ImageView
android:layout_width="150dp"
android:layout_height="150dp"
android:layout_centerInParent="true"
android:src="@drawable/ic_logo2"
/>
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp"
android:layout_marginTop="48dp">
<ImageView
android:layout_width="32dp"
android:layout_height="32dp"
android:layout_marginBottom="4dp"
android:layout_alignParentBottom="true"
android:src="@drawable/ic_baseline_person_outline_24"/>
<EditText
android:id="@+id/et_Account"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_gravity="center"
android:layout_marginStart="48dp"
android:layout_marginEnd="48dp"
android:layout_marginBottom="2dp"
android:hint="Email/Account"
android:inputType="text"
android:maxLines="1" />
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp"
android:layout_marginTop="20dp">
<ImageView
android:layout_width="32dp"
android:layout_height="32dp"
android:layout_marginBottom="4dp"
android:layout_alignParentBottom="true"
android:src="@drawable/ic_baseline_lock_24"/>
<EditText
android:id="@+id/et_pwd"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_gravity="center"
android:layout_marginStart="48dp"
android:layout_marginEnd="48dp"
android:layout_marginBottom="2dp"
android:hint="Password"
android:inputType="textPassword"
android:maxLines="1" />
<ImageView
android:layout_width="24dp"
android:layout_height="24dp"
android:src="@drawable/ic_baseline_visibility_off_24"
android:layout_marginBottom="6dp"
android:id="@+id/iv_pwd_switch"
android:layout_centerInParent="true"
android:layout_alignParentEnd="true"
/>
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="40dp"
android:layout_marginTop="16dp">
<CheckBox
android:id="@+id/cb_remember_pwd"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentStart="true"
android:layout_alignParentBottom="true"
android:layout_marginLeft="8dp"
android:layout_marginBottom="4dp"
android:text="remeber password"
android:textColor="@color/teal_700" />
<TextView
android:text="sign up"
android:textColor="@color/teal_700"
android:id="@+id/tv_sign_up"
android:layout_alignBaseline="@+id/cb_remember_pwd"
android:layout_marginEnd="8dp"
android:layout_width="wrap_content"
android:layout_alignParentEnd="true"
android:layout_alignParentBottom="true"
android:layout_height="wrap_content"/>
</RelativeLayout>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
android:layout_marginTop="48dp">
<Button
android:id="@+id/bt_login"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="Login"
android:textColor="@color/white" />
</RelativeLayout>
</LinearLayout>
MainActivity.java
package com.example.code05;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Context;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.text.InputType;
import android.text.TextUtils;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.Toast;
public class MainActivity extends AppCompatActivity implements View.OnClickListener{
private EditText etPwd;
private Boolean bPwdSwitch = false;
private EditText etAccount;
private CheckBox cbRemeberPwd;
private Button btLogin;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
final ImageView ivpwdswitch = findViewById(R.id.iv_pwd_switch);
etPwd = findViewById(R.id.et_pwd);
etAccount = findViewById(R.id.et_Account);
cbRemeberPwd = findViewById(R.id.cb_remember_pwd);
String spFileName = getResources().getString(R.string.shared_preferences_file_name);
String accountKey = getResources().getString(R.string.login_account_name);
String passwordKey = getResources().getString(R.string.login_password);
String rememberPasswordKey = getResources().getString(R.string.login_remember_password);
SharedPreferences spFile = getSharedPreferences(spFileName, MODE_PRIVATE);
String account = spFile.getString(accountKey,null);
String password = spFile.getString(passwordKey,null);
boolean rememberPassword = spFile.getBoolean(rememberPasswordKey,false);
if(account != null && !TextUtils.isEmpty(account)){
etAccount.setText(account);
}
if(password != null && !TextUtils.isEmpty(password)){
etPwd.setText(password);
}
cbRemeberPwd.setChecked(rememberPassword);
ivpwdswitch.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
bPwdSwitch = !bPwdSwitch;
if(bPwdSwitch){
ivpwdswitch.setImageResource(R.drawable.ic_baseline_visibility_24);
etPwd.setInputType(InputType.TYPE_TEXT_VARIATION_VISIBLE_PASSWORD);
}
else {
ivpwdswitch.setImageResource(R.drawable.ic_baseline_visibility_off_24);
etPwd.setInputType(InputType.TYPE_TEXT_VARIATION_PASSWORD |
InputType.TYPE_CLASS_TEXT);
}
}
});
btLogin = findViewById(R.id.bt_login);
if (btLogin!=null) {
btLogin.setOnClickListener(this);
}
}
@Override
public void onClick(View v) {
String spFileName = getResources().getString(R.string.shared_preferences_file_name);
String accountKey = getResources().getString(R.string.login_account_name);
String passwordKey = getResources().getString(R.string.login_password);
String rememberPasswordKey = getResources().getString(R.string.login_remember_password);
SharedPreferences spFile = getSharedPreferences(spFileName ,Context.MODE_PRIVATE);
SharedPreferences.Editor editor = spFile.edit();
if(cbRemeberPwd.isChecked()){
String password = etPwd.getText().toString();
String account = etAccount.getText().toString();
editor.putString(accountKey,account);
editor.putString(passwordKey,password);
editor.putBoolean(rememberPasswordKey,true);
editor.apply();
}else {
editor.remove(accountKey);
editor.remove(passwordKey);
editor.remove(rememberPasswordKey);
editor.apply();
}
}
}
18.布尔bool转变成String类
Bollean.getBollean(String s)
19.运行项目时总是出现Waiting for all target devices to come online
翻译成中文的意思是:等待所有目标设备上线
刚开始还以为是网络连接不通畅,后来百度一下发现不是。
可用的方法有:
(1).换一台运行模拟器
(2).打开AVD Manager,对相应的模拟器进行数据删除 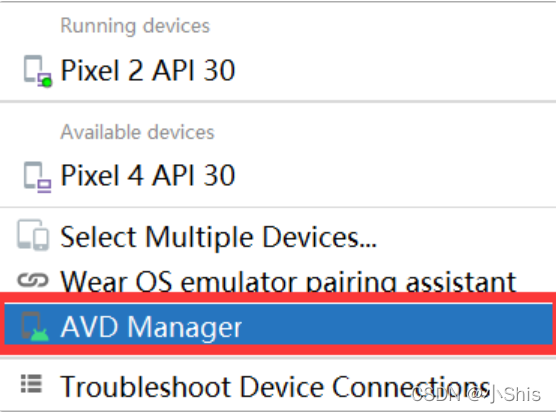
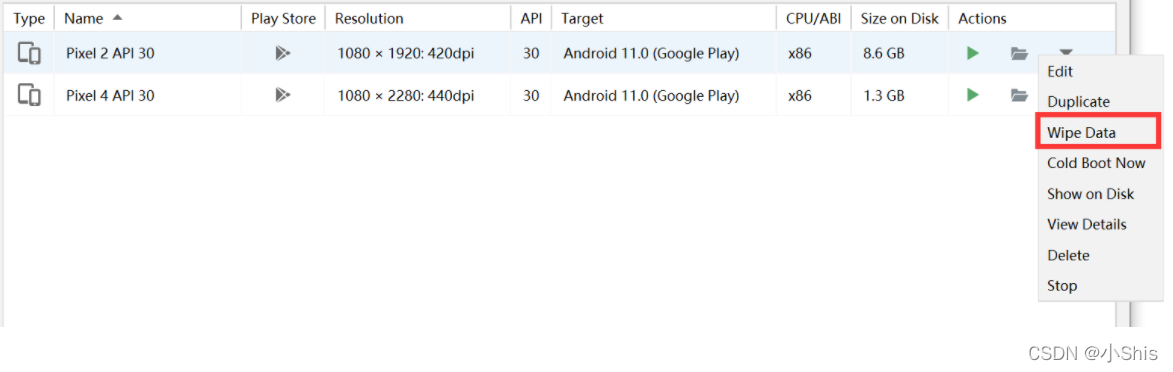
|