示例: 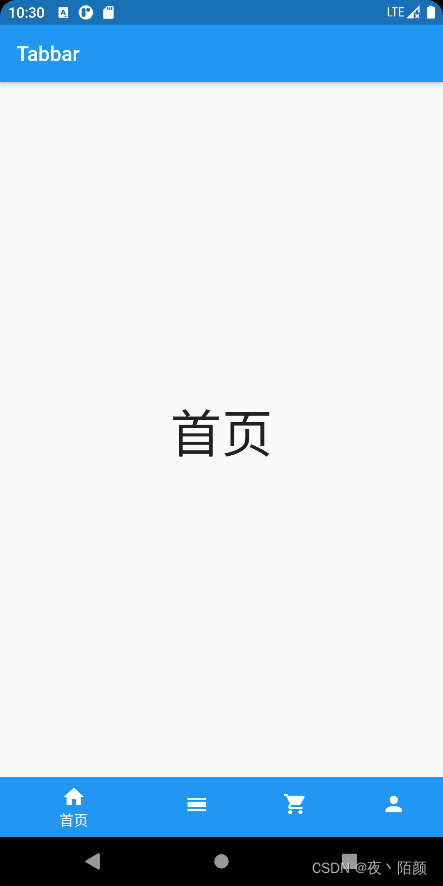
import 'package:flutter/material.dart';
class Home extends StatefulWidget {
Home({Key key}) : super(key: key);
@override
_HomeState createState() => _HomeState();
}
class _HomeState extends State<Home> {
final List<BottomNavigationBarItem> bottomNavigationItems = [
BottomNavigationBarItem(
icon: Icon(Icons.home),
backgroundColor: Colors.blue,
label: '首页',
),
BottomNavigationBarItem(
icon: Icon(Icons.calendar_view_day_sharp),
backgroundColor: Colors.yellow,
label: '分类',
),
BottomNavigationBarItem(
icon: Icon(Icons.shopping_cart),
backgroundColor: Colors.cyan,
label: '购物车',
),
BottomNavigationBarItem(
icon: Icon(Icons.person),
backgroundColor: Colors.purple,
label: '我的',
)
];
final page = [
Center(child: Text('首页', style: TextStyle(fontSize: 50))),
Center(child: Text('分类', style: TextStyle(fontSize: 50))),
Center(child: Text('购物车', style: TextStyle(fontSize: 50))),
Center(child: Text('我的', style: TextStyle(fontSize: 50)))
];
int index;
void changePage(currentIndex) {
setState(() {
index = currentIndex;
});
}
@override
void initState() {
// TODO: implement initState
super.initState();
index = 0;
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Tabbar'),
),
bottomNavigationBar: BottomNavigationBar(
items: bottomNavigationItems,
currentIndex: index,
type: BottomNavigationBarType.shifting,
onTap: (index) {
changePage(index);
},
),
body: page[index],
);
}
}
|